Solving Data Loading Issues in ZK’s MVVM Architecture
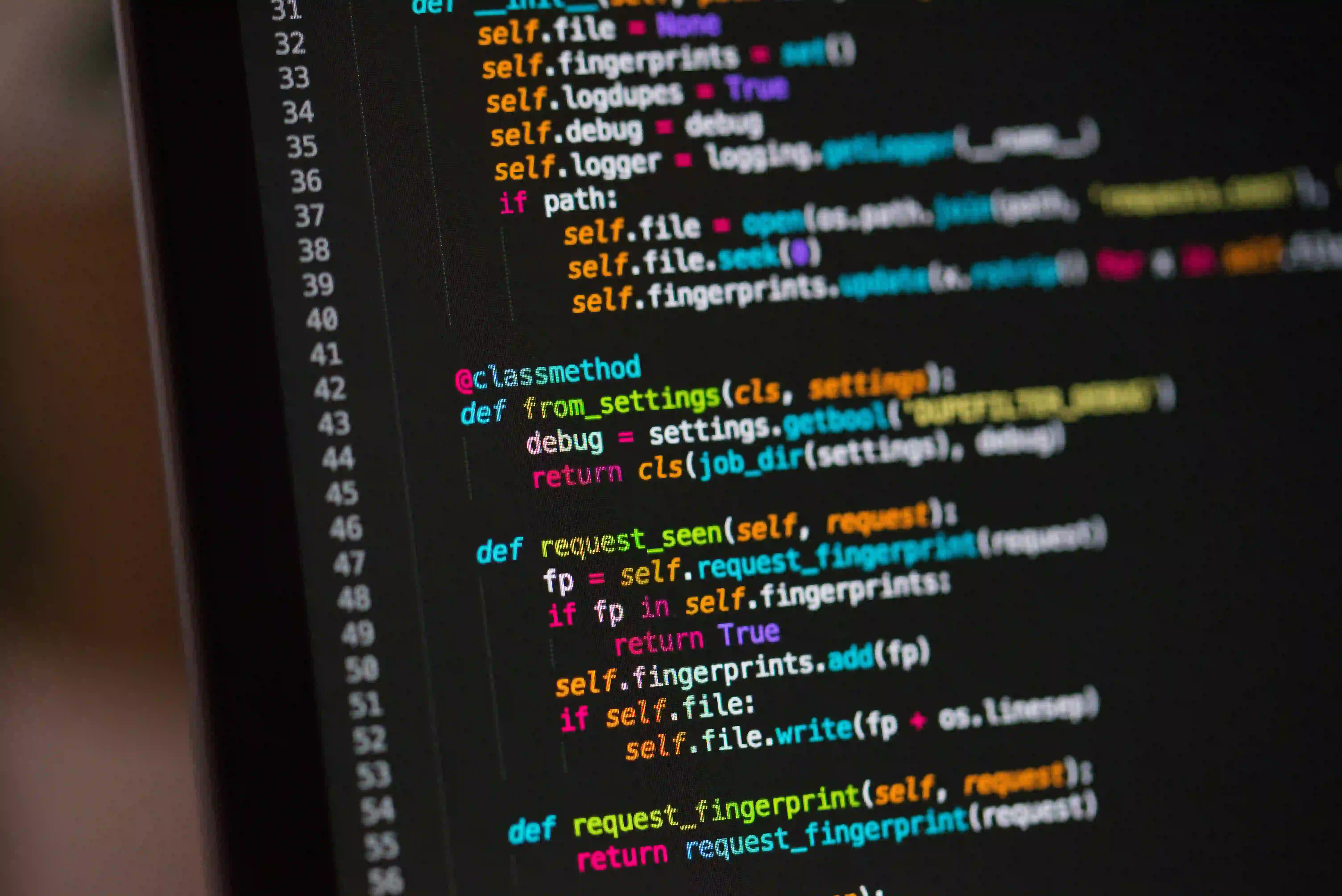
Solving Data Loading Issues in ZK's MVVM Architecture
In today’s fast-paced digital world, web applications need to be efficient, responsive, and capable of handling large volumes of data seamlessly. ZK Framework, known for its rich user interface, leverages the Model-View-ViewModel (MVVM) architectural pattern to achieve this goal. However, developers often encounter data loading issues while working with the MVVM architecture in ZK. In this post, we will explore these issues, understand the underlying reasons, and discover potential solutions.
Understanding MVVM Architecture in ZK
Before diving into the specific data loading issues, let's quickly recap what the MVVM architecture entails.
- Model: This represents the data and business logic of the application. It’s the backbone that interacts with databases or other data sources.
- View: The user interface that presents data (UI components).
- ViewModel: Acts as a bridge between the Model and the View, handling user inputs and updating the Model as needed.
This pattern promotes better separation of concerns, making the application more maintainable and scalable.
Why Use MVVM in ZK?
- Separation of Concerns: By splitting the application into distinct parts, developers can work on the Model, View, and ViewModel independently.
- Testability: The ViewModel is isolated from the View, making unit testing straightforward.
- Two-Way Data Binding: ZK supports automatic data synchronization between View and ViewModel, reducing boilerplate code.
Common Data Loading Issues in ZK’s MVVM
1. Latency in Data Retrieval
One of the most pressing issues is latency during data loading. This can occur due to several factors, such as:
- Poorly optimized database queries
- Large datasets being fetched entirely into memory
- Inefficient network calls
Solution: Optimize Data Queries
It's essential to ensure that database interactions are efficient. Use paging and lazy loading to minimize the amount of data retrieved at once. Here’s an example using ZK's paging feature:
@Command
public void loadPagedData(@BindingParam("page") int page) {
int pageSize = 20; // Number of records per page
List<MyData> data = myDataService.fetchData(page, pageSize);
this.myDataList.clear();
this.myDataList.addAll(data);
}
In this example, we fetch only a subset of data instead of loading everything at once, thus reducing latency.
2. Inconsistent Data State
Loading data asynchronously can lead to scenarios where the View might display outdated or inconsistent data. This usually happens when the ViewModel updates the Model but the View is still showing old data.
Solution: Implement Clear Data Binding
ZK provides an excellent way to ensure data consistency through its bindings. However, incorrect bindings can lead to issues.
Example:
<textbox value="@bind(myViewModel.myProperty)" />
<button label="Load Data" onClick="@command('loadData')" />
Ensure your ViewModel is set up properly and the data bindings are truly reflecting the current state of the Model. Always validate data integrity before rendering it in the UI.
3. UI Freezing During Data Load
When large amounts of data are loaded, it can lead to UI freezing or a poor user experience. This happens when the thread responsible for processing the data gets blocked.
Solution: Implement Background Data Loading
To prevent this, data should be loaded in a background thread, allowing the UI to remain responsive. Here's an example utilizing ZK's TaskExecutor:
@Command
public void loadDataAsync() {
new Thread(() -> {
List<MyData> data = myDataService.fetchAllData();
UI.getCurrent().access(() -> {
myDataList.clear();
myDataList.addAll(data);
});
}).start();
}
This way, the data is fetched in the background, and the UI is updated only once the data is fully ready.
4. Data Updates Not Reflected in the View
Sometimes, after data updates, changes may not be immediately reflected in the View due to incorrect binding or lack of proper event handling.
Solution: Use Notifiers
Using ZK's built-in listener framework can help to ensure the View is updated in response to changes in the Model.
public void updateModel() {
// Assume some data update logic
this.myDataList = myDataService.updateData();
firePropertyChange("myDataList", null, myDataList);
}
In this example, we explicitly notify the View of property changes, which allows it to stay in sync with the Model.
5. Error Handling During Data Loading
When loading data, especially from an external source, errors may occur (e.g., connection timeouts or data parsing issues).
Solution: Implement Robust Error Handling
Always implement proper error handling mechanisms when loading data. Display user-friendly messages in the UI so they are informed about issues rather than left in the dark.
@Command
public void loadDataSafely() {
try {
loadData();
} catch (Exception e) {
Clients.showNotification("Error loading data: " + e.getMessage(), "error", null, null, 3000);
}
}
This adds a layer of safety, improving overall user experience.
Closing Remarks
ZK’s MVVM architecture offers tremendous advantages in building scalable and maintainable web applications. However, data loading issues can hinder the user experience if not managed appropriately. By optimizing data queries, implementing clear data binding, loading data in the background, ensuring timely updates, and incorporating error handling, developers can enhance their applications' efficiency and responsiveness.
Creating a seamless data loading experience is crucial for engaging users. By following the strategies outlined in this article, you can address common pitfalls in ZK's MVVM architecture and elevate your applications to a new level of performance.
For more insights on ZK, you might find helpful resources in the official ZK documentation.
Further Reading
- Understanding MVVM with ZK
- ZK Data Binding Overview
- Optimizing Database Queries
Feel free to engage with us in the comments section below if you have any questions or experiences to share regarding data loading issues in ZK. Happy coding!