Streamline Your Build: Renaming Ant Tasks in Gradle
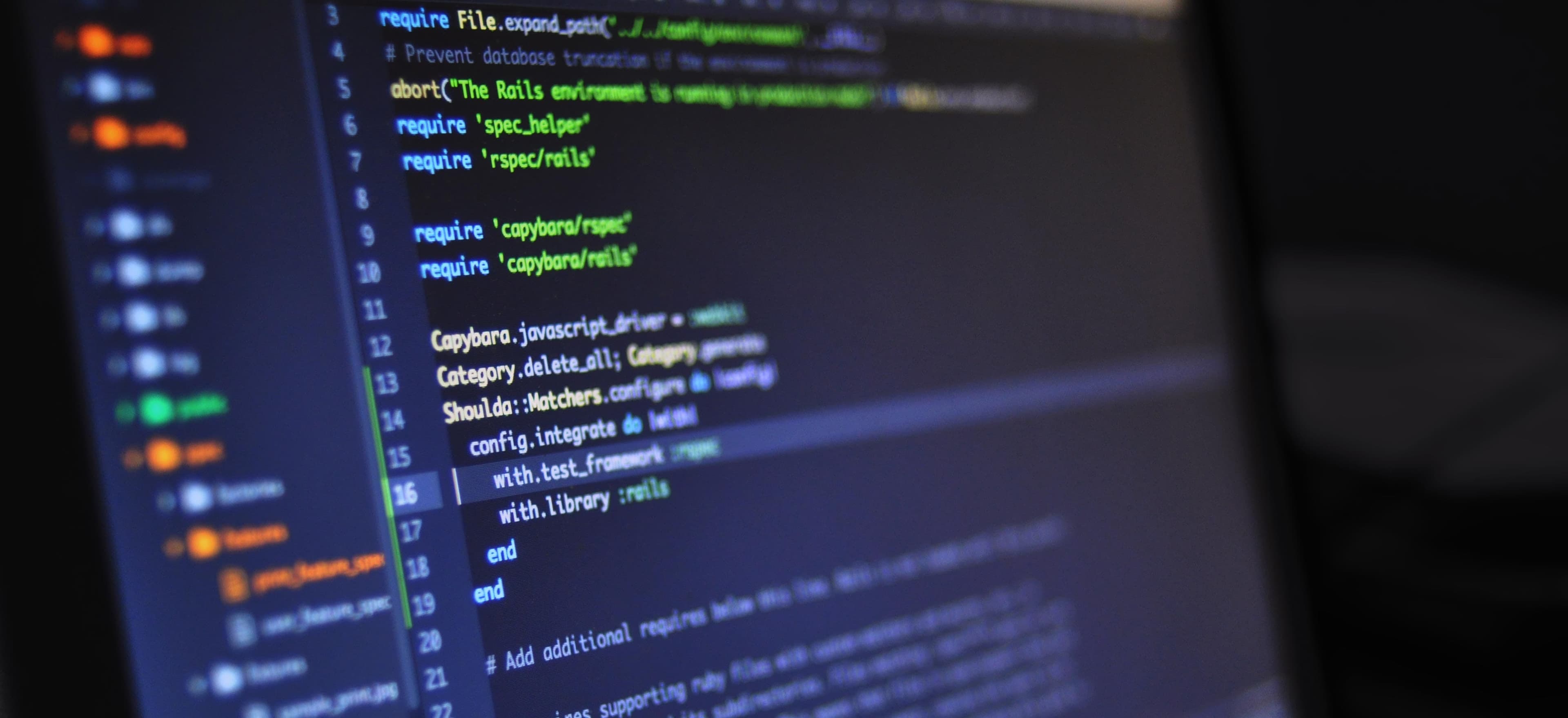
- Published on
Streamline Your Build: Renaming Ant Tasks in Gradle
Gradle is a powerful build automation tool that has gained traction in the development community due to its flexibility and efficiency. While primarily known for its ability to manage Java projects, Gradle also supports integration with Ant, allowing developers to leverage existing Ant scripts within a Gradle workflow. One of the ways to maximize efficiency is by renaming Ant tasks in Gradle. This blog post delves into what this process entails, its benefits, and how to implement it with examples.
Understanding Ant and Gradle
Apache Ant has been around since the early 2000s and is a Java-based build tool that uses XML configuration files for specifying tasks. However, managing complex build environments can become cumbersome with Ant, leading to the rise of Gradle.
Gradle uses a Groovy or Kotlin-based DSL (Domain Specific Language) for configuration, promoting better readability and maintainability. But why would you want to mix the two tools?
- Legacy Support: Many organizations still have a substantial amount of legacy Ant scripts that are hard to rewrite.
- Familiarity: Gradle's flexibility allows you to maintain familiar Ant tasks while enhancing your build process.
- Incremental Upgrades: You can upgrade your build system incrementally without needing a complete rewrite.
Renaming Ant Tasks in Gradle
When you integrate Ant tasks into your Gradle build script, you might find it beneficial to rename these tasks to align with your project's naming conventions or simply for clarity. Renaming tasks might seem like a minor adjustment, but it can greatly enhance your team's understanding and usability of the build process.
The Basic Syntax
The basic approach to renaming an Ant task in Gradle involves:
- Importing the Ant task.
- Specifying the task under a new name using Gradle's syntax.
Example: Renaming an Ant Task
Let's consider an example where we have an Ant task named compile
. We want to rename this task to compileJava
in our Gradle build script.
Step 1: Adding the Ant Task
First, we will import the Ant task using the ant
property provided by Gradle. It gives us the ability to call any Ant tasks directly from our Gradle script.
task compileAnt {
doLast {
ant.taskdef(name: 'compile', classname: 'org.apache.tools.ant.taskdefs.Javac')
ant.compile(srcdir: 'src', destdir: 'bin') {
include(name: '**/*.java')
}
}
}
Explanation:
task compileAnt {}
: Defines a new Gradle task namedcompileAnt
.doLast {}
: Indicates that the enclosed code should be executed at the end of the task.ant.taskdef
: Registers thecompile
Ant task.ant.compile(...)
: Calls the compile task, specifying source and destination directories.
Step 2: Renaming the Ant Task
Now let’s create a new task within our Gradle build file that wraps the existing Ant task but with a new name.
task compileJava(dependsOn: compileAnt) {
doLast {
println 'Renamed task compileAnt to compileJava successfully!'
}
}
Explanation:
task compileJava {...}
: This defines a new task namedcompileJava
that depends on thecompileAnt
task.dependsOn
: Indicates thatcompileJava
will execute thecompileAnt
task first.println
: Outputs a message to the console indicating a successful run.
Running the Tasks
You can now run the newly renamed task in Gradle using:
gradle compileJava
This command will execute the Ant task under the new name, rendering the build process more intuitive.
Benefits of Renaming Ant Tasks
- Clarity: Having well-named tasks enhances readability and aids new team members in understanding the build process.
- Consistency: Aligning Ant task names with Gradle conventions helps in maintaining consistency across the build scripts.
- Ease of Maintenance: As your build scripts evolve, maintaining intuitive naming can reduce confusion when adding or removing tasks.
Additional Considerations
While renaming tasks, there are some considerations to keep in mind:
- Dependency Management: Ensure that renamed tasks do not cause dependency issues with other tasks.
- Documentation: Update documentation and comments in your build scripts to reflect any changes made.
- Testing: Test the renamed tasks thoroughly to ensure they behave as expected.
Lessons Learned
Integrating and renaming Ant tasks in Gradle can greatly streamline your build process, particularly in environments using both tools. By following the examples and guidelines laid out in this post, you can make your build scripts clearer and more intuitive.
If you're intrigued by Gradle and looking for more advanced use cases, consider checking out the official Gradle documentation for a deeper dive into its capabilities.
By taking advantage of Gradle's flexibility, you can breathe new life into your build processes, ensuring they remain effective and maintainable as your projects evolve. Implement these strategies today and witness a smoother, more coherent workflow within your development team!
Checkout our other articles