Debugging Common JAX-RS Issues in RESTEasy Services
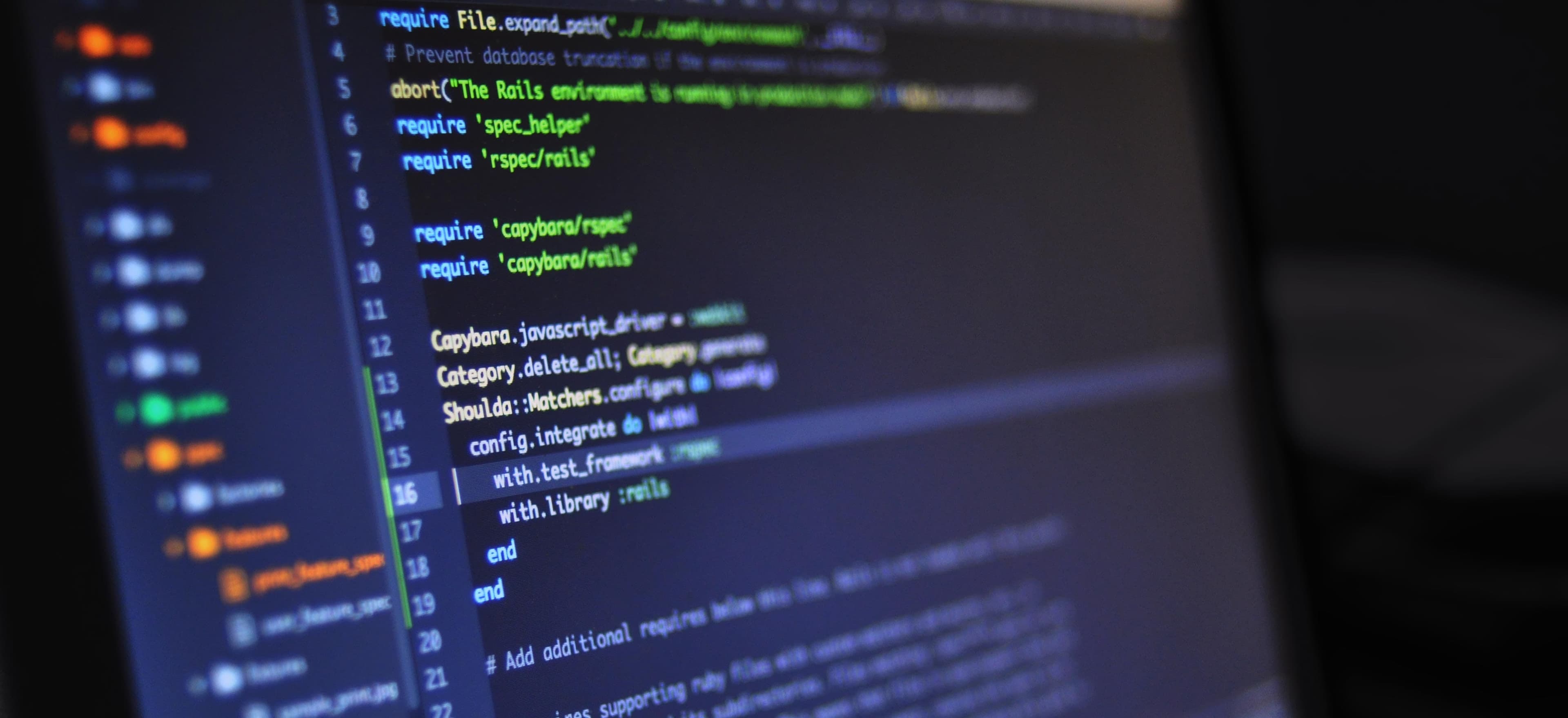
- Published on
Debugging Common JAX-RS Issues in RESTEasy Services
In the era of microservices and RESTful APIs, JAX-RS (Java API for RESTful Web Services) plays a crucial role in building scalable and maintainable applications. RESTEasy is a popular implementation of JAX-RS, providing developers with powerful features for creating RESTful web services in Java. However, like any technology, developers can encounter issues. This blog post will explore common debugging strategies for resolving typical issues faced when working with RESTEasy services.
Understanding RESTEasy and JAX-RS
RESTEasy is an application framework for building RESTful web services in Java. It implements the JAX-RS specification and offers a simple way to create, test, and deploy RESTful applications.
Before delving into debugging techniques, let's ensure we have a fundamental understanding of JAX-RS basics.
Basic JAX-RS Example
Below is a simple example of a JAX-RS resource that manages a list of books:
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import java.util.*;
@Path("/books")
public class BookResource {
private static List<String> books = new ArrayList<>();
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<String> getBooks() {
return books;
}
@POST
@Consumes(MediaType.APPLICATION_JSON)
public void addBook(String book) {
books.add(book);
}
}
Why Use JAX-RS?
JAX-RS provides several benefits:
- Simplicity: The annotations and components simplify the task of creating services.
- Seamless Integration: It can easily integrate into existing Java EE standards.
- RESTful Principles: Supports REST principles, allowing for efficient resource handling.
Now, let’s dive into common issues and debugging techniques.
Common JAX-RS Issues
1. Resource Not Found (404 Error)
A common issue developers face is encountering a 404 error when trying to access an endpoint. This can happen for several reasons:
- Incorrect URL: Make sure that the URL corresponds to the @Path annotation defined in your resource class.
- Missing the Context Path: Ensure you include any context path your application may require when deploying to a servlet container.
Debugging Steps
- Check Deployment Descriptor: Confirm your application is properly deployed, especially if you're using WAR files.
- Logging: Use logging frameworks like SLF4J or Log4j to capture any runtime issues. Here’s an example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Path("/books")
public class BookResource {
private static final Logger logger = LoggerFactory.getLogger(BookResource.class);
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<String> getBooks() {
logger.info("Fetching books");
return books;
}
}
2. Unsupported Media Type (415 Error)
Another frequent problem is the "415 Unsupported Media Type" error, which usually occurs when the server cannot process the request's payload due to incorrect content type.
Debugging Steps
- Check Content-Type Headers: Ensure that your client request sends the relevant
Content-Type
header. For instance, when sending JSON data, it should beapplication/json
. - Correct Annotations: Ensure that your resource methods are using the correct @Consumes annotation.
Example of a correct POST
method in a resource:
@POST
@Consumes(MediaType.APPLICATION_JSON)
public void addBook(String book) {
books.add(book);
}
3. Method Not Allowed (405 Error)
A 405 error indicates that the HTTP method being used is not allowed for the specific resource. This commonly occurs when:
- An incorrect HTTP method (GET, POST, PUT, DELETE) is used on an endpoint.
- There’s a missing or incorrect @Path or @HttpMethod annotation.
Debugging Steps
- Check HTTP Method Usage: Ensure the client is using the right HTTP method defined in your resource method annotations.
- Confirm Annotations: Verify that each method has the correct annotations as shown in this example:
@DELETE
@Path("/{id}")
public void deleteBook(@PathParam("id") int id) {
// Logic to delete book
}
4. Serialization and Deserialization Issues
Often, you might encounter problems related to JSON serialization and deserialization, particularly when using frameworks like Jackson or Gson.
Debugging Steps
- Inspect Object Structure: Ensure that your Java objects are correctly annotated (e.g., with @JsonProperty for Jackson).
- Verify JSON Data: Check the JSON data being sent and ensure it matches your Java object's structure.
Example of a properly annotated object:
import com.fasterxml.jackson.annotation.JsonProperty;
public class Book {
@JsonProperty("title")
private String title;
@JsonProperty("author")
private String author;
// Getters and setters
}
5. Dependency Injection Issues
If you are using a dependency injection framework, such as CDI (Contexts and Dependency Injection) with JAX-RS, it can introduce challenges if not properly set up.
Debugging Steps
- Lifecycle Annotations: Ensure your resource lifecycle is managed correctly, using annotations such as @RequestScoped or @ApplicationScoped.
- Injection Points: Check that you are correctly injecting dependencies.
Example of dependency injection in RESTEasy:
import javax.inject.Inject;
@Path("/books")
public class BookResource {
@Inject
private BookService bookService;
// Resource methods...
}
Best Practices for Debugging JAX-RS Services
1. Use Logging Effectively
Incorporate logging throughout your application to help trace issues. Use various log levels (INFO, DEBUG, ERROR) to capture different severity of issues.
2. API Documentation
Consider using Swagger or OpenAPI to provide API documentation, which makes it easier to visualize your services and troubleshoot issues.
3. State Your Errors
When an error occurs, return meaningful error responses with helpful messages. This can be done using the @Provider annotation:
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Error Occurred: " + exception.getMessage())
.build();
}
}
4. Testing and Validation
Always validate your JSON payloads on the client-side before sending requests. Use testing frameworks like JUnit or RestAssured for unit testing your services.
Lessons Learned
Debugging issues in JAX-RS services can be challenging, but with a clear understanding of common problems and thoughtful debugging techniques, you can easily find solutions to most problems. By leveraging logging, embracing best practices, and utilizing the tools available, you can develop robust and error-free RESTful services with RESTEasy.
For detailed documentation or further reading, consider visiting JAX-RS Official Documentation, or check out the RESTEasy Documentation.
With these insights in hand, you are now better equipped to create and debug JAX-RS applications effectively. Happy coding!