Unlocking Clarity: The Case for Named Parameters in Java
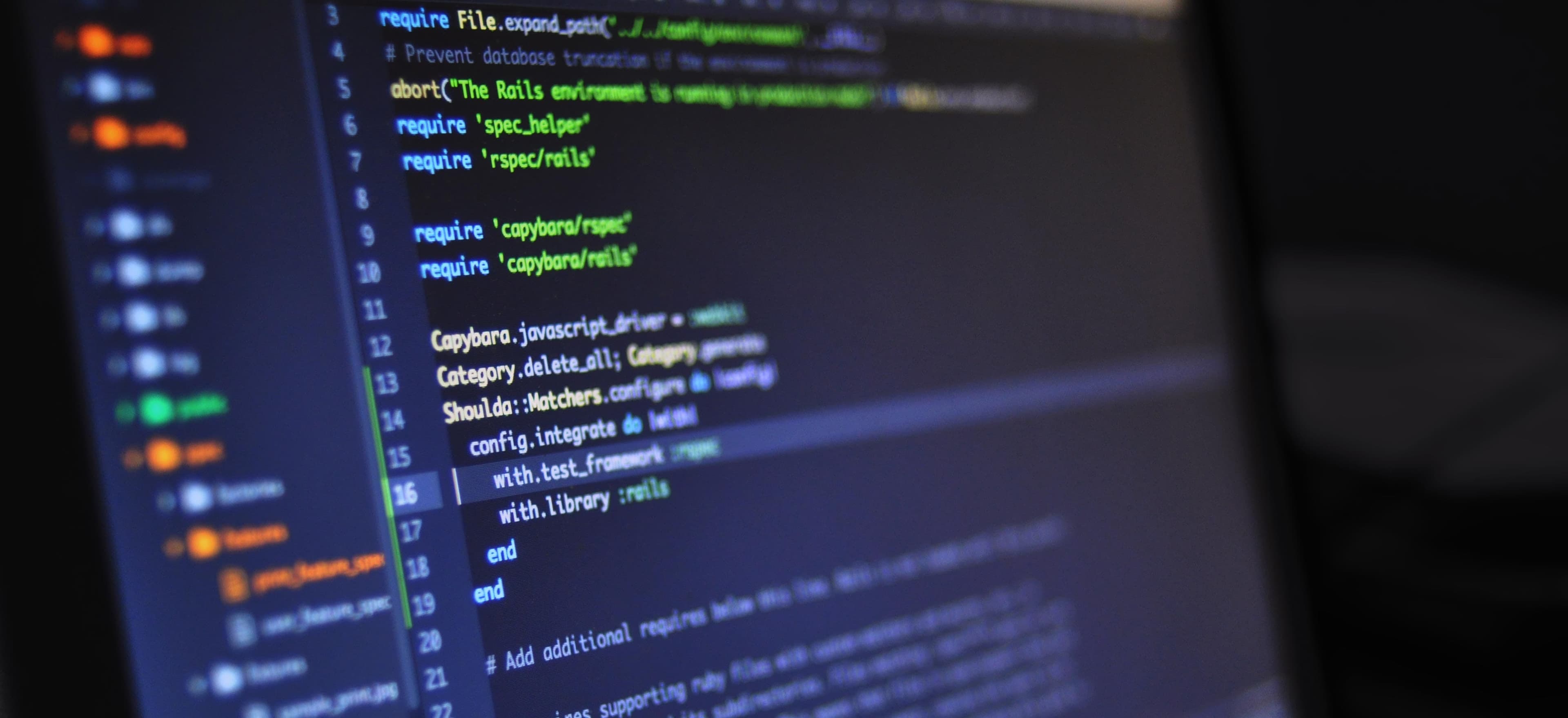
- Published on
Unlocking Clarity: The Case for Named Parameters in Java
In the world of programming, clarity and readability are paramount. Understanding how parameters are passed to methods can significantly affect how easy or hard it is to maintain code. In this blog post, we're going to explore the concept of named parameters in Java—an approach that, while not natively supported by the language itself, can still be emulated effectively for better code clarity.
What Are Named Parameters?
Named parameters are a programming construct where the parameters passed to a function or method are prefixed with names. This provides a clear mapping between the method's parameters and the arguments that are being passed. While Java does not support named parameters out of the box, we can use several strategies to achieve similar results.
The Drawbacks of Traditional Parameter Passing
In traditional parameter passing, the order in which parameters are supplied to a method is crucial. If a method is defined to accept parameters in the order of "name", "age", and "address", it requires callers to always remember this order, leading to potential errors and reduced readability. Consider the following example:
public void createUser(String name, int age, String address) {
// Implementation here
}
// Calling the method
createUser("Alice", 25, "123 Main St");
Here, it is clear what each parameter means at first glance. However, if the code grows complex, especially with multiple method overloading or extensive parameter lists, it can quickly become difficult to remember what each argument corresponds to.
The Case for Named Parameters: Improved Readability
Using named parameters can significantly improve the readability and maintainability of your code. Let's dive into the strategies to emulate them in Java.
Strategy 1: Builder Pattern
The Builder Pattern is a widely used design pattern that allows for the creation of complex objects in a step-by-step approach. This is particularly beneficial when we have multiple parameters that can be optional.
Example: User Class with Builder
Let’s consider our User
class which accepts multiple parameters.
public class User {
private String name;
private int age;
private String address;
private User(Builder builder) {
this.name = builder.name;
this.age = builder.age;
this.address = builder.address;
}
public static class Builder {
private String name;
private int age;
private String address;
public Builder name(String name) {
this.name = name;
return this;
}
public Builder age(int age) {
this.age = age;
return this;
}
public Builder address(String address) {
this.address = address;
return this;
}
public User build() {
return new User(this);
}
}
}
Usage
With the builder pattern, creating a new User
instance becomes remarkably clearer:
User user = new User.Builder()
.name("Alice")
.age(25)
.address("123 Main St")
.build();
Why Use Builder Pattern?
The builder pattern provides a clear and readable way to construct complex objects without needing to remember the order of parameters, making it self-documenting. It is particularly advantageous in scenarios where you have optional parameters, which often leads to method overflow.
Strategy 2: Using Maps for Named Parameters
Another approach to achieve the effect of named parameters is to use a Map<String, Object>
. This technique is less strong-typed and more flexible, but it can increase the risk of runtime errors if not handled properly.
Example: Using a Map
In this example, we will create a method that accepts Map<String, Object>
as parameters:
public void createUser(Map<String, Object> params) {
String name = (String) params.get("name");
int age = (int) params.get("age");
String address = (String) params.get("address");
// Implementation here
}
// Usage
Map<String, Object> userParams = new HashMap<>();
userParams.put("name", "Alice");
userParams.put("age", 25);
userParams.put("address", "123 Main St");
createUser(userParams);
Why Use a Map?
Using a map provides flexibility in argument naming and allows for optional parameters without worrying about their order. However, it sacrifices compile-time checking against the wrong types, which could lead to runtime issues.
Strategy 3: Record Classes (Java 14+)
If you are using Java 14 or above, you can leverage record classes to manage named parameters neatly. Records provide a compact way to create data classes in Java.
Example: User Record
public record User(String name, int age, String address) {}
// Usage
User user = new User("Alice", 25, "123 Main St");
Why Use Record Classes?
Records are inherently clear as they provide named parameters. The declaring fields in the record serve as names themselves, making the code self-documenting and easy to understand.
Key Takeaways: Enhance Clarity and Maintainability
In summary, while Java does not support named parameters natively, various techniques like the Builder Pattern, using Maps, and employing Record Classes can enhance the clarity and maintainability of your code.
Further Reading
For those interested in learning more about the Builder pattern, you may visit Refactoring Guru for detailed insights.
Also, if you are curious about Java Records and their advantages, check out Oracle's official guide for a comprehensive overview.
By applying these strategies, not only can you unlock clarity in your Java code, but you can also create a programming environment that emphasizes readability, maintainability, and less cognitive load for yourself and your team.