DataWeave vs. Java: Solving Common Mapping Errors
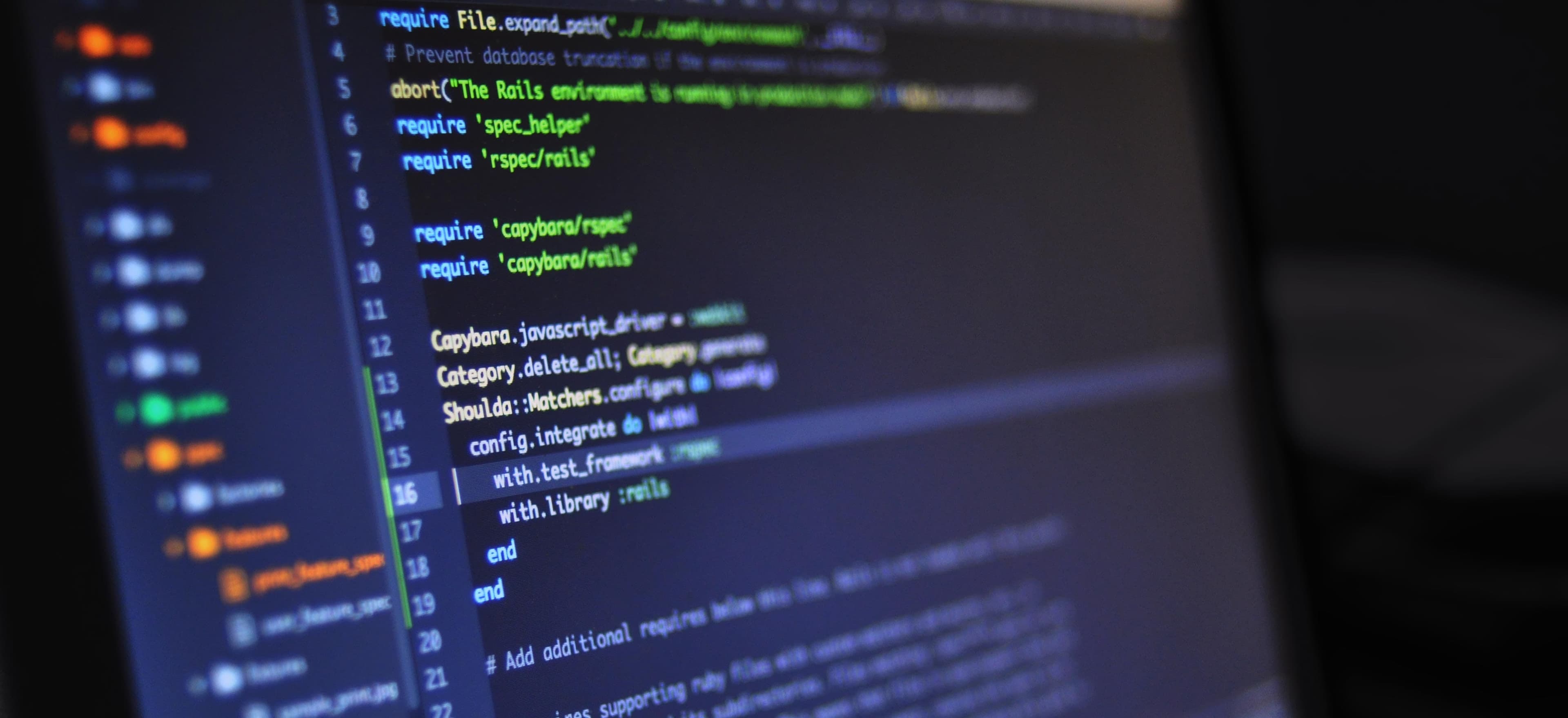
- Published on
DataWeave vs. Java: Solving Common Mapping Errors
In the era of data-driven decisions, transforming and mapping data efficiently is critical. Whether you're integrating data from APIs, databases, or flat files, you’ll often run into scenarios where data needs manipulation or transformation before it's usable. Two programming muscles come into play here: DataWeave, the powerful data transformation language used in MuleSoft, and Java, a widely-used programming language known for its versatility.
In this blog post, we will explore common mapping errors encountered in both DataWeave and Java, discussing how to solve them while highlighting key differences in their approaches.
Understanding Mapping Context
Before we dive into specific errors, it's crucial to understand the context of data mapping. Mapping involves taking data from a source format and transforming it into a target format. This may involve changing data types, modifying structures, or even filtering unnecessary information. Below is a brief explanation of both languages' roles in this mapping process:
DataWeave
DataWeave is inherently designed for data transformation. Its syntax is concise and geared towards making data manipulation straightforward, especially when dealing with JSON, XML, CSV and other formats.
Java
Java, while a general-purpose programming language, requires a more manual approach to data mapping. Java provides extensive libraries, such as Jackson for JSON processing, that facilitate transformation but demand more overhead in terms of setup and coding.
Common Mapping Errors: DataWeave vs. Java
1. Missing Fields in Output
DataWeave Example:
When a required field is not present in the input, DataWeave might throw an error. For instance:
%dw 2.0
output application/json
var input = { "name": "John" }
---
{
name: input.name,
age: input.age // This will throw an error because 'age' does not exist
}
Solution: Safeguard the fields using the ?
operator, which checks for the field’s existence.
%dw 2.0
output application/json
var input = { "name": "John" }
---
{
name: input.name,
age: input.age ? input.age : "unknown" // Now it defaults to "unknown" if age is missing
}
2. Type Mismatch Errors
Java Example:
The type mismatch is a common error when mapping different data types, such as converting a String to an Integer.
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataMapping {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
String jsonString = "{\"name\":\"John\", \"age\":\"Twenty\"}"; // The age is a String
try {
JsonNode jsonNode = mapper.readTree(jsonString);
int age = jsonNode.get("age").asInt(); // This will throw an error
} catch (Exception e) {
e.printStackTrace();
}
}
}
Solution: Convert the string to an integer safely by handling potential parsing errors:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataMapping {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
String jsonString = "{\"name\":\"John\", \"age\":\"Twenty\"}";
try {
JsonNode jsonNode = mapper.readTree(jsonString);
int age = 0; // Default value if parsing fails
try {
age = Integer.parseInt(jsonNode.get("age").asText());
} catch (NumberFormatException nfe) {
System.out.println("Invalid age format");
}
System.out.println("Age: " + age);
} catch (Exception e) {
e.printStackTrace();
}
}
}
3. Null Values Handling
DataWeave Example:
Handling null values can be tricky. In DataWeave, if a value is null and is attempted to be accessed directly, it will produce an error.
%dw 2.0
output application/json
var input = { "name": "John", "age": null }
---
{
name: input.name,
age: input.age.toString() // This will throw an error because 'age' is null
}
Solution: Use defaults or checks to handle null values gracefully:
%dw 2.0
output application/json
var input = { "name": "John", "age": null }
---
{
name: input.name,
age: input.age ?? "unknown" // Using '??' to provide a default value
}
4. Mismatched Structures
Java Example:
When your source and target structures don't align, mapping might cause runtime exceptions. Here’s how Java handles mismatched structures using a straightforward example:
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataMapping {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
String jsonString = "{\"firstName\":\"John\", \"lastName\":\"Doe\"}"; // JSON structure
try {
JsonNode jsonNode = mapper.readTree(jsonString);
String name = jsonNode.get("name").asText(); // This will throw an error since "name" doesn't exist
} catch (Exception e) {
e.printStackTrace();
}
}
}
Solution: Always check the expected keys or utilize a mapping structure where names match:
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataMapping {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
String jsonString = "{\"firstName\":\"John\", \"lastName\":\"Doe\"}";
try {
JsonNode jsonNode = mapper.readTree(jsonString);
String firstName = jsonNode.has("firstName") ? jsonNode.get("firstName").asText() : "Unknown";
String lastName = jsonNode.has("lastName") ? jsonNode.get("lastName").asText() : "Unknown";
System.out.println(firstName + " " + lastName);
} catch (Exception e) {
e.printStackTrace();
}
}
}
5. Performance Issues
Lastly, performance can vary significantly between DataWeave and Java, particularly as the size of the data increases.
DataWeave:
DataWeave is optimized for performance behind the scenes, managing large datasets efficiently through streaming and lazy evaluation. When handling substantial data, DataWeave can process it in chunks without loading it entirely into memory.
Java:
Performance in Java can be improved by using streams and efficient data structures. Below is an example utilizing streams to enhance performance on a collection of data:
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class DataMapping {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe", "Alice");
Optional<String> foundName = names.stream()
.filter(name -> name.startsWith("J"))
.findFirst();
foundName.ifPresent(System.out::println); // prints "John"
}
}
In Conclusion, Here is What Matters
In conclusion, while both DataWeave and Java serve important functions for data mapping, each has its own strengths and common pitfalls. DataWeave excels in expressive syntax and built-in data transformation capabilities, while Java offers robustness and extensive libraries.
By being aware of the common errors—such as missing fields, type mismatches, null values handling, mismatched structures, and performance considerations—you can craft more reliable and efficient data transformation solutions, bridging the gap between disparate systems effectively.
For further reading on this topic, consider exploring these resources:
By mastering both DataWeave and Java, you will broaden your data manipulation toolkit, allowing you to tackle complex data transformation challenges confidently.