Mastering the Chain of Responsibility Pattern in Modern Apps
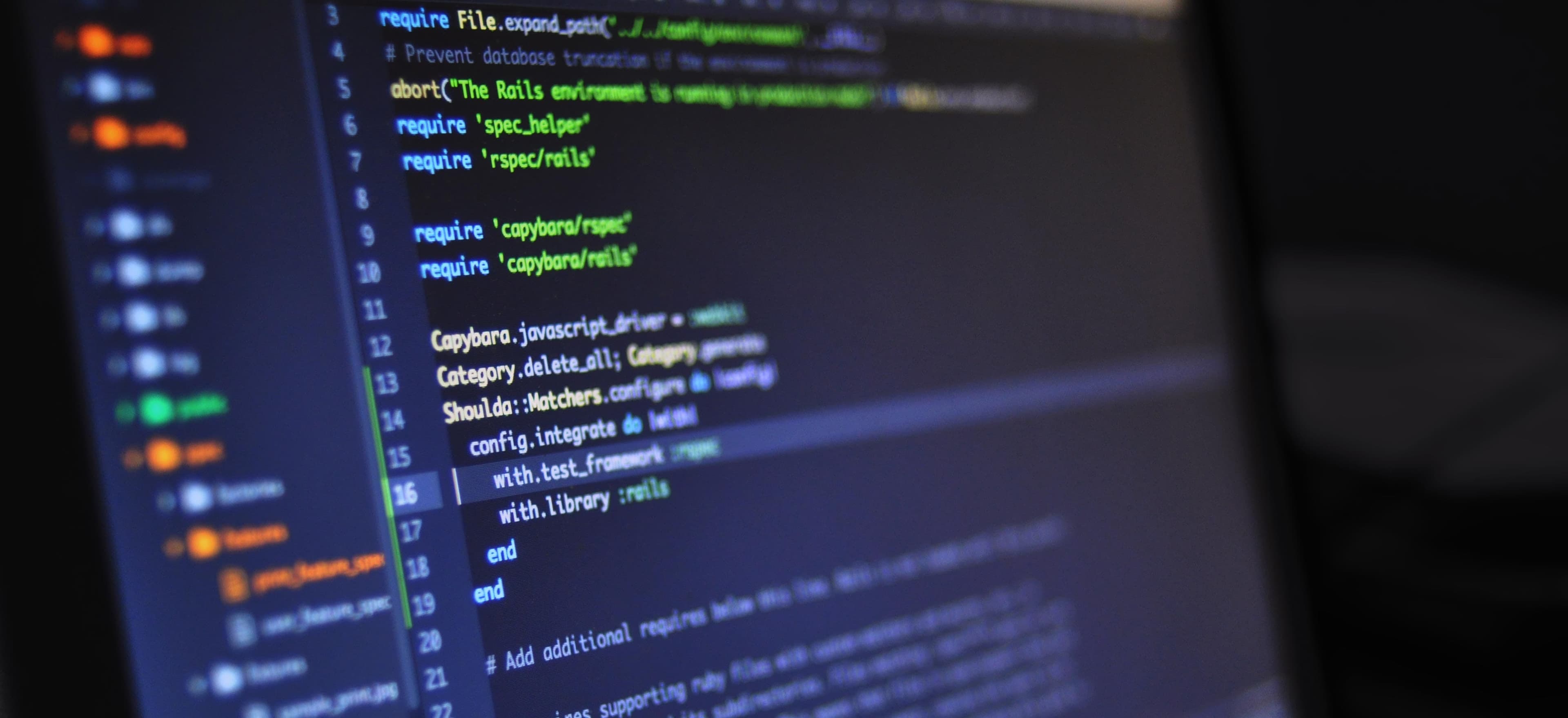
- Published on
Mastering the Chain of Responsibility Pattern in Modern Apps
In the evolving landscape of software design, patterns play a crucial role in creating clean, maintainable, and scalable applications. One such design pattern is the Chain of Responsibility (CoR) pattern. It facilitates handling requests in a systematic way, allowing multiple handlers to respond to a request. In this article, we will explore the CoR pattern in depth, its implementation in Java, and its practical applications in modern applications.
What is the Chain of Responsibility Pattern?
The Chain of Responsibility is a behavioral design pattern that allows an object to pass a request along a chain of potential handlers until one of them handles the request. This pattern decouples the sender of the request from its receivers, promoting loose coupling and reducing dependencies between components in a system.
Key Benefits
-
Loose Coupling: The sender and the receiver are not tightly bound. You can add or remove handlers independently.
-
Reusable Handlers: Handlers can be reused and reconfigured dynamically, promoting flexibility in your architecture.
-
Easier Maintenance: Changes to the logic of request handling can often be made by simply altering the chain configuration.
How Does It Work?
The CoR pattern typically consists of three key components:
- Handler Interface: Defines a method for handling requests and an optional link to the next handler.
- Concrete Handlers: Implement the handler interface and decide either to process the request or pass it to the next handler in the chain.
- Client: Initiates the request and configures the chain of handlers.
Let's delve into a practical example using Java.
Implementing Chain of Responsibility in Java
Step 1: Define the Handler Interface
The first step in implementing the CoR pattern is defining the handler interface.
public interface Handler {
void setNext(Handler nextHandler);
void handleRequest(String request);
}
Commentary: This interface declares the methods for setting the next handler and processing the request. The setNext
method allows you to create a chain of handlers.
Step 2: Create Concrete Handlers
Next, we create concrete handler classes that implement the Handler
interface.
public class ConcreteHandlerA implements Handler {
private Handler nextHandler;
@Override
public void setNext(Handler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String request) {
if (request.equals("A")) {
System.out.println("Handler A processed request A");
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
public class ConcreteHandlerB implements Handler {
private Handler nextHandler;
@Override
public void setNext(Handler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String request) {
if (request.equals("B")) {
System.out.println("Handler B processed request B");
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
public class ConcreteHandlerC implements Handler {
private Handler nextHandler;
@Override
public void setNext(Handler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(String request) {
if (request.equals("C")) {
System.out.println("Handler C processed request C");
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
Commentary: Each handler processes requests according to its own logic. If a handler cannot handle the request, it forwards it to the next handler in the chain.
Step 3: Client Code
Now, let’s create a client that sets up and uses the chain.
public class Client {
public static void main(String[] args) {
Handler handlerA = new ConcreteHandlerA();
Handler handlerB = new ConcreteHandlerB();
Handler handlerC = new ConcreteHandlerC();
// Setting up the chain of responsibility
handlerA.setNext(handlerB);
handlerB.setNext(handlerC);
// Sending requests
handlerA.handleRequest("A"); // Handler A processes request A
handlerA.handleRequest("B"); // Handler B processes request B
handlerA.handleRequest("C"); // Handler C processes request C
handlerA.handleRequest("D"); // No handler processes request D
}
}
Commentary: The client initializes the chain, defining the order of the handlers. When a request is sent, it travels through the chain until a handler successfully processes it.
Practical Applications of the Chain of Responsibility Pattern
The Chain of Responsibility pattern has several practical applications in modern software development:
1. Event Handling Systems
In GUI frameworks or game engines, events (like mouse clicks, key presses) are often processed using the CoR pattern. Each component can react to the event without directly referencing other components.
2. HTTP Requests in Web Applications
Web applications often route requests through a series of filters or middleware before reaching the endpoint. The CoR pattern can help manage these interceptor-like layers to handle authentication, logging, or input validation.
3. Logging Systems
Logging frameworks can implement the CoR pattern allowing various log handlers (console, file, remote server) to process messages based on severity levels.
In Conclusion, Here is What Matters
The Chain of Responsibility pattern is a powerful tool in modern software design. It promotes loose coupling, enables flexibility, and simplifies request handling. The Java implementation we've explored illustrates how this pattern can be applied practically in various scenarios.
By mastering design patterns like the Chain of Responsibility, developers can build robust, maintainable, and efficient applications.
For more insights into software design patterns, you can check out Refactoring Guru and DZone.
With this understanding in hand, you can now leverage the CoR pattern to enhance your application's architecture and ensure a cleaner, more manageable codebase. Happy coding!