Common Pitfalls When Saving Data in DynamoDB with Lambda
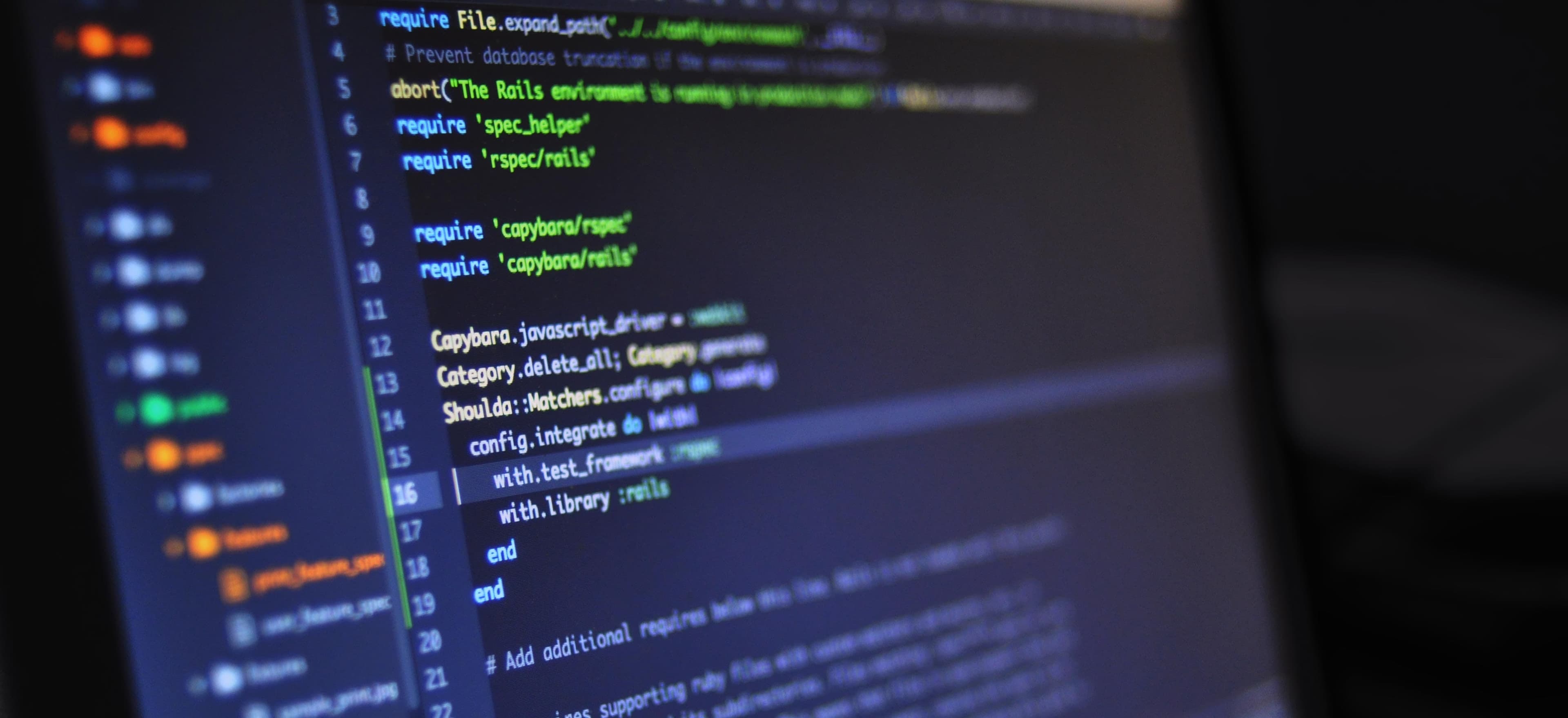
- Published on
Common Pitfalls When Saving Data in DynamoDB with Lambda
Amazon DynamoDB is a fully managed NoSQL database service designed for high availability and durability. When used alongside AWS Lambda, it can create powerful serverless applications. However, developers frequently encounter common pitfalls that can lead to inefficient code or even data loss.
In this blog post, we’ll explore these pitfalls and provide guidance on how to navigate them. We will also offer code snippets with explanations addressing the "why" behind them.
1. Not Understanding Provisioned Throughput vs. On-Demand
One of the first decisions to make when setting up DynamoDB is choosing between provisioned throughput and on-demand capacity mode.
Provisioned Throughput:
In this mode, you specify the number of reads and writes per second. It is essential to monitor usage closely. If your DynamoDB consumption exceeds your provisioned throughput, you will encounter "throttling," where write operations fail, and your Lambda function will throw errors.
On-Demand Capacity Mode:
This mode automatically adjusts to accommodate workload changes. It is ideal for unpredictable workloads, such as those that a Lambda function might generate.
Tip: Choose the mode that best fits your use case. For a serverless architecture with unpredictable usage, on-demand is often the safer choice.
Code Example
// Import necessary DynamoDB libraries
import com.amazonaws.services.dynamodbv2.*;
import com.amazonaws.services.dynamodbv2.model.*;
AWSLambda client = AWSLambdaClientBuilder.standard().build();
String tableName = "YourTable-name";
CreateTableRequest request = new CreateTableRequest()
.withTableName(tableName)
.withProvisionedThroughput(new ProvisionedThroughput()
.withReadCapacityUnits(5L)
.withWriteCapacityUnits(5L));
try {
client.createTable(request);
} catch (ResourceInUseException e) {
System.out.println("Table already exists. Specify a different name.");
}
2. Overlooking Error Handling
When deploying applications with Lambda, error handling is crucial. DynamoDB return codes can be misleading, and without robust error handling, you might miss critical failures.
For instance, you might encounter a ConditionalCheckFailedException
if a write operation does not meet your specified conditions. Failing to handle such exceptions can leave your data in an inconsistent state.
Code Example
try {
// Write to DynamoDB
Map<String, AttributeValue> itemValues = new HashMap<>();
itemValues.put("Id", new AttributeValue("1"));
itemValues.put("Name", new AttributeValue("Sample"));
PutItemRequest putItemRequest = new PutItemRequest("YourTable", itemValues);
client.putItem(putItemRequest);
} catch (ConditionalCheckFailedException e) {
System.out.println("Conditional check failed: " + e.getMessage());
} catch (DynamoDBException e) {
System.out.println("DynamoDB error: " + e.getMessage());
}
3. Inefficient Query Patterns
Designing efficient query patterns is vital for maintaining application performance. DynamoDB is designed for specific types of queries; failing to follow these can lead to performance degradation.
For instance, using a global secondary index (GSI) to improve read access patterns can significantly boost speed and efficiency. Nevertheless, using GSIs too liberally can also cause you to exceed limits and increase costs.
Code Example
// Define Global Secondary Index (GSI) for efficient querying
GlobalSecondaryIndex gsi = new GlobalSecondaryIndex()
.withIndexName("GSI-Name")
.withKeySchema(new KeySchemaElement()
.withAttributeName("SecondaryKey").withKeyType(KeyType.HASH))
.withProjection(new Projection()
.withProjectionType(ProjectionType.ALL))
.withProvisionedThroughput(new ProvisionedThroughput(5L, 5L));
CreateTableRequest request = new CreateTableRequest()
.withTableName("YourTable")
.withAttributeDefinitions(new AttributeDefinition("PrimaryKey", ScalarAttributeType.S))
.withKeySchema(new KeySchemaElement("PrimaryKey", KeyType.HASH))
.withGlobalSecondaryIndexes(gsi);
4. Not Using Batch Operations Efficiently
Batch write operations in DynamoDB allow you to write multiple items in a single call. This not only improves efficiency but also reduces costs by minimizing the number of requests to DynamoDB.
Lambda triggers do not support batch processing inherently, but using batch writes can still provide significant performance enhancements.
Code Example
List<WriteRequest> writeRequests = new ArrayList<>();
for (int i = 0; i < 25; i++) { // Maximum batch size is 25
Map<String, AttributeValue> itemValues = new HashMap<>();
itemValues.put("Id", new AttributeValue(String.valueOf(i)));
itemValues.put("Name", new AttributeValue("Name" + i));
writeRequests.add(new WriteRequest(new PutRequest().withItem(itemValues)));
}
BatchWriteItemRequest batchWriteItemRequest =
new BatchWriteItemRequest().withRequestItems(Collections.singletonMap("YourTable", writeRequests));
client.batchWriteItem(batchWriteItemRequest);
5. Ignoring Retry Strategies
In distributed systems, transient failures can occur. If a write to DynamoDB fails, it’s crucial to implement a robust retry logic. AWS SDK provides exponential backoff for retrying requests.
Exponential Backoff: This strategy delays successive retries exponentially, allowing your application a better chance to succeed on subsequent attempts.
Code Example
public void saveItemWithRetry(Map<String, AttributeValue> itemValues) {
int retries = 0;
boolean successful = false;
while (retries < 5 && !successful) {
try {
PutItemRequest putItemRequest = new PutItemRequest("YourTable", itemValues);
client.putItem(putItemRequest);
successful = true; // Exit loop if successful
} catch (ProvisionedThroughputExceededException e) {
retries++;
try {
Thread.sleep((long) Math.pow(2, retries) * 100); // Exponential backoff
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
if (!successful) {
System.out.println("Item could not be saved after 5 retries.");
}
}
6. Forgetting to Index Properly
Efficient retrieval is essential for any application. Proper indexing is key when working with DynamoDB. If your queries often involve certain attributes, create indexes for those.
Code Example
// When you find yourself querying by this specific attribute often, create a GSI
GlobalSecondaryIndex gsi = new GlobalSecondaryIndex()
.withIndexName("AttributeIndex")
.withKeySchema(new KeySchemaElement("YourAttribute", KeyType.HASH))
.withProjection(new Projection().withProjectionType(ProjectionType.ALL))
.withProvisionedThroughput(new ProvisionedThroughput(5L, 5L));
Always make sure you analyze your access patterns before deploying your indexes to avoid excessive costs.
Final Thoughts
Working with DynamoDB and AWS Lambda can be incredibly rewarding, but it's essential to navigate these common pitfalls effectively. By understanding the distinctions between provisioned and on-demand capacity, prioritizing error handling, utilizing batch operations, and implementing efficient query patterns, you can significantly enhance your application's performance and reliability.
Not only will mastering these best practices save you time and resources, but they will ultimately enable you to build robust, scalable applications that leverage the full power of serverless architecture.
For more detailed AWS insights and documentation regarding DynamoDB, refer to the official AWS DynamoDB Documentation and check out DynamoDB's Best Practices.
By avoiding these common mistakes, you set the stage for success with your serverless app built on DynamoDB and Lambda. Happy coding!