How to Overcome Data Latency in Real-Time Uber Analytics
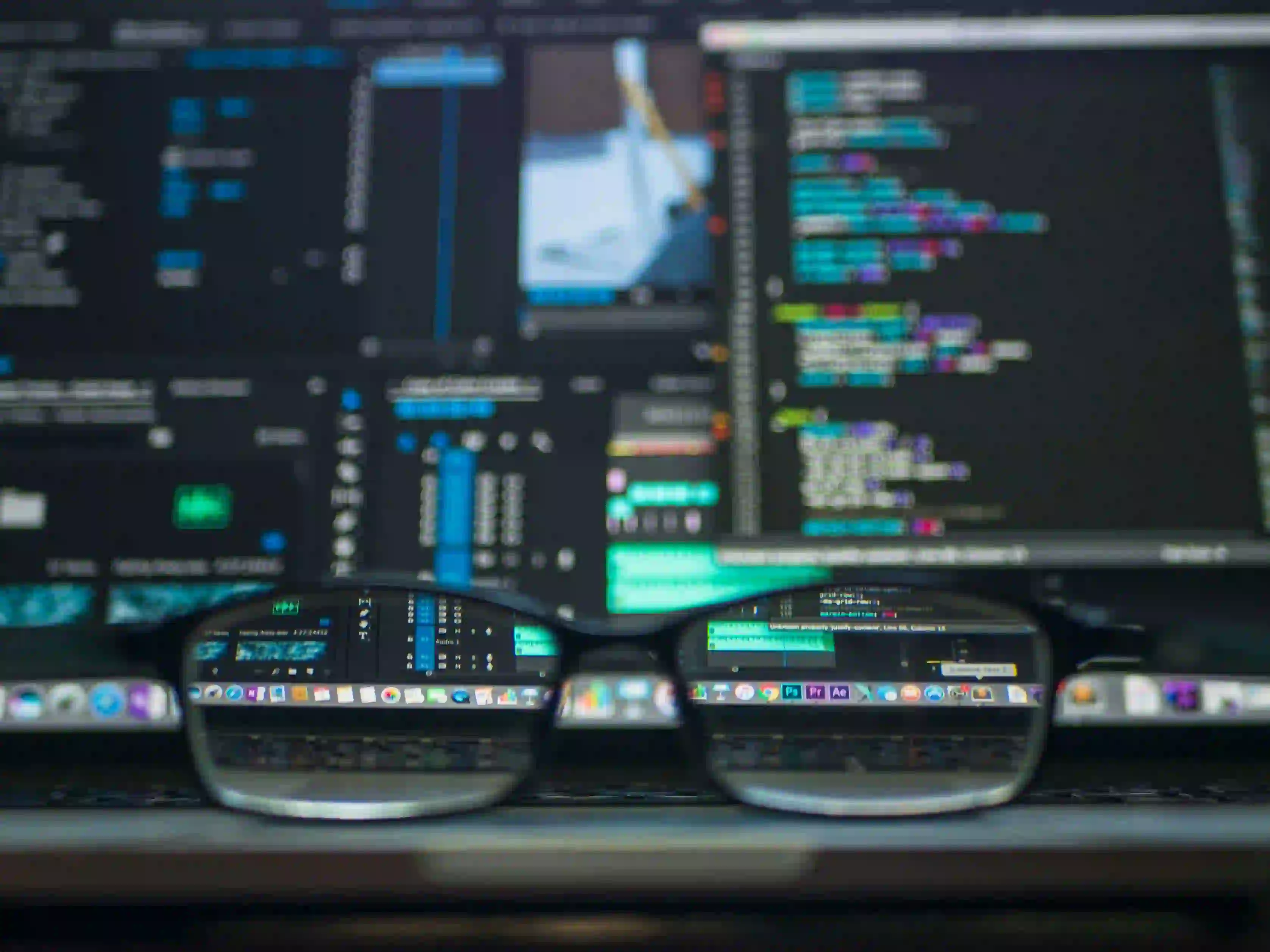
How to Overcome Data Latency in Real-Time Uber Analytics
In today’s fast-paced digital world, data latency can be a major bottleneck, particularly for companies reliant on real-time analytics, like Uber. As one of the largest ride-sharing platforms globally, Uber relies heavily on data to optimize user experience, ensure driver availability, and maintain operational excellence. In this blog post, we will discuss the challenges of data latency in real-time analytics and outline strategies to overcome these hurdles.
Understanding Data Latency
Data latency refers to the delay between the time data is generated and the time it is analyzed and utilized. This delay can stem from various sources, including network issues, data ingestion, processing time, or the time it takes to visualize the data. For an application like Uber, where decisions must be made in split seconds, even a fraction of a second can significantly impact user experience and operational efficiency.
The Cost of Data Latency
For ride-sharing services like Uber, high levels of data latency can lead to various problems:
- Poor User Experience: Delayed information on driver availability or estimated arrival times can frustrate users.
- Operational Inefficiencies: High latency can affect surge pricing, which relies on real-time analysis of rider demand and driver supply.
- Inaccurate Data Processing: Real-time fraud detection mechanisms could fail if the data analysis does not happen promptly.
Key Strategies to Mitigate Data Latency
1. Streamlining Data Ingestion
Efficient data ingestion is crucial for minimizing latency. One popular approach is to use Apache Kafka for real-time data streaming. Kafka allows for the real-time collection of data from various sources, enabling Uber to process it as it arrives.
Here is a simple example of a Kafka producer in Java:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class UberDataProducer {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(properties);
String topic = "uber-rides";
String key = "rideID_123";
String value = "User requested a ride";
ProducerRecord<String, String> record = new ProducerRecord<>(topic, key, value);
producer.send(record, (metadata, exception) -> {
if (exception != null) {
exception.printStackTrace();
} else {
System.out.println("Record sent to topic " + metadata.topic() + " partition " + metadata.partition());
}
});
producer.close();
}
}
Commentary on the Code:
- KafkaProducer Initialization: Properties define the broker and serialization methods.
- ProducerRecord: It packages the key, value, and topic together for sending.
- Callback Function: This handles completion or errors, allowing for better error monitoring.
2. Implementing Stream Processing
Once the data is ingested, it needs to be processed in real time. Utilizing a stream processing framework like Apache Flink or Apache Spark Streaming can help efficiently analyze the continuous flow of data.
Here’s a simple Spark streaming application in Java:
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaPairDStream;
import org.apache.spark.api.java.function.PairFunction;
import org.apache.spark.streaming.Durations;
import org.apache.spark.streaming.api.java.JavaDStream;
import org.apache.spark.streaming.api.java.JavaStreamingContext;
import scala.Tuple2;
public class UberStreamProcessor {
public static void main(String[] args) throws InterruptedException {
SparkConf conf = new SparkConf().setAppName("UberStreamProcessor").setMaster("local[*]");
JavaStreamingContext jssc = new JavaStreamingContext(conf, Durations.seconds(1));
JavaDStream<String> stream = jssc.socketTextStream("localhost", 9999);
JavaPairDStream<String, Integer> counts = stream.mapToPair(new PairFunction<String, String, Integer>() {
public Tuple2<String, Integer> call(String x) {
return new Tuple2<>(x, 1);
}
}).reduceByKey(Integer::sum);
counts.print();
jssc.start();
jssc.awaitTermination();
}
}
Commentary on the Code:
- Socket Text Stream: It simulates real-time data inputs.
- Pair Function: Maps inputs to key-value pairs for counting instances.
- Reduce Function: Aggregates counts based on keys, enabling quick insights on rider requests or driver statuses.
3. Caching and Data Storage Solutions
Utilizing an in-memory data grid like Redis or Apache Ignite can reduce access times for frequently requested data.
import redis.clients.jedis.Jedis;
public class UberRedisExample {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
// Caching ride data
jedis.set("rideID_123", "User requested a ride");
// Retrieving ride data quickly
String rideData = jedis.get("rideID_123");
System.out.println("Fetched ride data: " + rideData);
jedis.close();
}
}
Commentary on the Code:
- Quick Access to Data: The use of
set
andget
functions allows for fast storage and retrieval. - In-memory Advantage: Redis operates in memory, thereby significantly reducing latency compared to disk-based databases.
4. Monitoring and Alerting
Implementing robust monitoring and alerting systems can help proactively identify and address performance issues. Tools like Prometheus and Grafana can be utilized to visualize the data flow and quickly react to latency spikes.
# Sample Prometheus configuration
scrape_configs:
- job_name: 'uber-analytics'
static_configs:
- targets: ['localhost:9090']
Commentary on the Configuration:
- Job Name: It identifies the service being monitored.
- Targets: This specifies where Prometheus should scrape metrics from, enabling it to collect performance data.
Key Takeaways
Overcoming data latency in real-time analytics is crucial for ride-sharing platforms like Uber. By streamlining data ingestion, implementing effective stream processing, leveraging caching solutions, and maintaining robust monitoring systems, companies can significantly enhance their analytics capabilities.
These approaches not only improve responsiveness but also offer a competitive edge in a fast-paced environment. As data continues to grow exponentially, adopting these strategies will be imperative for staying ahead of the curve.
For more information on data analytics strategies, consider checking out resources from Apache Flink or Spark documentation.
By keeping an eye on latency and reacting swiftly to data dynamics, companies like Uber can ensure they provide an outstanding user experience and operational efficacy.