Common Pitfalls When Daemonizing JVM Applications
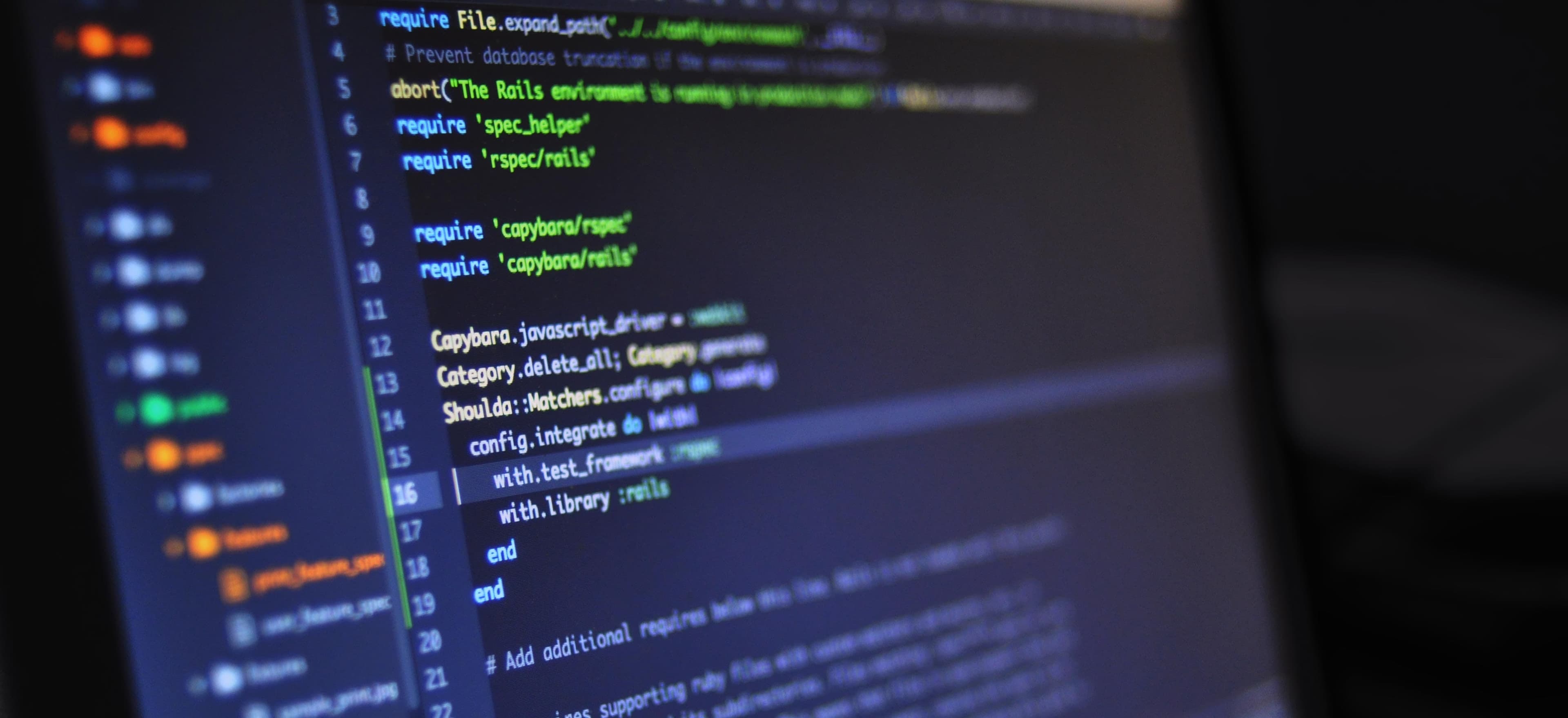
- Published on
Common Pitfalls When Daemonizing JVM Applications
Daemonizing Java Virtual Machine (JVM) applications is an essential aspect of modern software development. It allows your applications to run as background processes, freeing up system resources and enabling persistent operations. However, this process is fraught with pitfalls that developers often face. This blog post aims to shed light on the common challenges you might encounter when daemonizing your JVM applications and provide solutions to avoid these issues.
Understanding Daemonization
Daemonization refers to the method of running an application as a daemon, which is a background service that performs tasks without direct user interaction. In Java, this is often done using the class java.lang.Process
, along with Java libraries designed for background tasks.
Common Pitfalls
1. Incorrect Handling of Standard I/O Streams
When daemonizing a JVM application, it is crucial to manage standard input, output, and error streams correctly. If these streams are not redirected, any output from the application could end up in a terminal that no longer exists.
Solution: Redirect Output Streams
You can redirect the standard output and error streams to files or a logging system. Here’s an example of how to do this:
import java.io.*;
public class DaemonExample {
public static void main(String[] args) {
// Redirect output streams
try {
PrintStream out = new PrintStream(new File("daemon.log"));
System.setOut(out);
System.setErr(out);
// Your daemon logic here
} catch (FileNotFoundException e) {
System.err.println("Error setting up log file: " + e.getMessage());
}
}
}
Why? Redirecting output streams ensures that your application logs its activity correctly, making it easier for debugging.
2. Ignoring Shutdown Hooks
Another common pitfall is forgetting to register shutdown hooks. If your application receives a termination signal, it may not clean up resources properly, leading to potential data loss or corruption.
Solution: Use Shutdown Hooks
Java provides a straightforward way to add shutdown hooks using Runtime.getRuntime().addShutdownHook()
. Here’s an example:
public class DaemonWithShutdownHook {
public static void main(String[] args) {
// Register a shutdown hook
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
// Clean up resources here
System.out.println("Shutdown hook triggered.");
// Additional cleanup code
}));
// Daemon logic
while (true) {
// Simulate doing some work
try {
Thread.sleep(1000);
System.out.println("Working...");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
break;
}
}
}
}
Why? Using shutdown hooks helps ensure that your application exits gracefully, maintaining the integrity of your data.
3. Not Using a Suitable Threading Model
When building a daemon, improper utilization of threads can lead to performance bottlenecks or even application crashes. Some developers may use only the main thread for all tasks, leading to blocking operations and poor responsiveness.
Solution: Utilize Executor Services
Using ExecutorService
allows you to manage a pool of threads effectively, ensuring your application remains responsive while handling background tasks.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class DaemonWithExecutor {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(10);
// Submit tasks for execution
for (int i = 0; i < 100; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Task " + taskId + " is running.");
// Task logic here
});
}
executorService.shutdown();
}
}
Why? This approach allows for better management of threads and resources, improving the overall stability and performance of your application.
4. Failing to Handle Exceptions
In a daemon process, failing to handle exceptions can lead to unexpected termination of the application. Uncaught exceptions can be particularly damaging in long-running applications.
Solution: Implement Robust Exception Handling
Always utilize try-catch blocks to handle exceptions effectively. Here is an illustrative example:
public class DaemonWithExceptionHandling {
public static void main(String[] args) {
try {
while (true) {
// Simulated task
potentiallyHazardousOperation();
}
} catch (Exception e) {
System.err.println("An error occurred: " + e.getMessage());
// Additional error handling
}
}
private static void potentiallyHazardousOperation() throws Exception {
// Some risky logic
}
}
Why? Exception handling is essential for maintaining application stability. It prevents crashes and allows for graceful recovery.
5. Manual Resource Management
Failing to manage resources like database connections, sockets, or file handles can lead to resource leaks. Such issues can be particularly fatal in long-running daemons.
Solution: Use Try-With-Resources
Java introduced the try-with-resources statement, allowing automatic resource management. Here’s how you can use it:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DaemonWithResources {
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection("jdbc:yourdb", "user", "password")) {
// Use the connection
} catch (SQLException e) {
System.err.println("Database error: " + e.getMessage());
}
}
}
Why? Utilizing try-with-resources ensures that all resources are closed properly, reducing the likelihood of leaks.
6. Not Testing Daemon Behavior
Finally, one of the most significant oversights developers make is not thoroughly testing daemon behavior, especially in different environments. Failures can be opaque and hard to diagnose if not properly tested.
Solution: Implement Comprehensive Testing
Test your daemon under various scenarios, including high-load situations, unexpected signal interruptions, and network failures. Utilize frameworks like JUnit for unit testing and Mockito for mocking dependencies.
import org.junit.Test;
import static org.mockito.Mockito.*;
public class DaemonTesting {
@Test
public void testResourceCleanupOnShutdown() {
// Setup mock objects and validate cleanup logic
}
}
Why? Rigorous testing helps identify and rectify potential issues before they affect end-users, ensuring a more stable release.
To Wrap Things Up
Daemonizing JVM applications can greatly enhance their usability and performance. However, developers must navigate through various pitfalls to achieve this effectively. By understanding and mitigating common challenges such as improper I/O handling, absent shutdown hooks, inefficient threading, unhandled exceptions, resource mismanagement, and inadequate testing, you can create robust and reliable background processes.
For further reading on JVM application design, check out Oracle’s Java Tutorials and Effective Java by Joshua Bloch.
Remember, diligence and attention to detail in the daemonizing process will pay off in the long run, providing a reliable and efficient application that users can depend on. Happy coding!
Checkout our other articles