Maximize Java EE Performance: TinyLog's Hidden Logging Issues
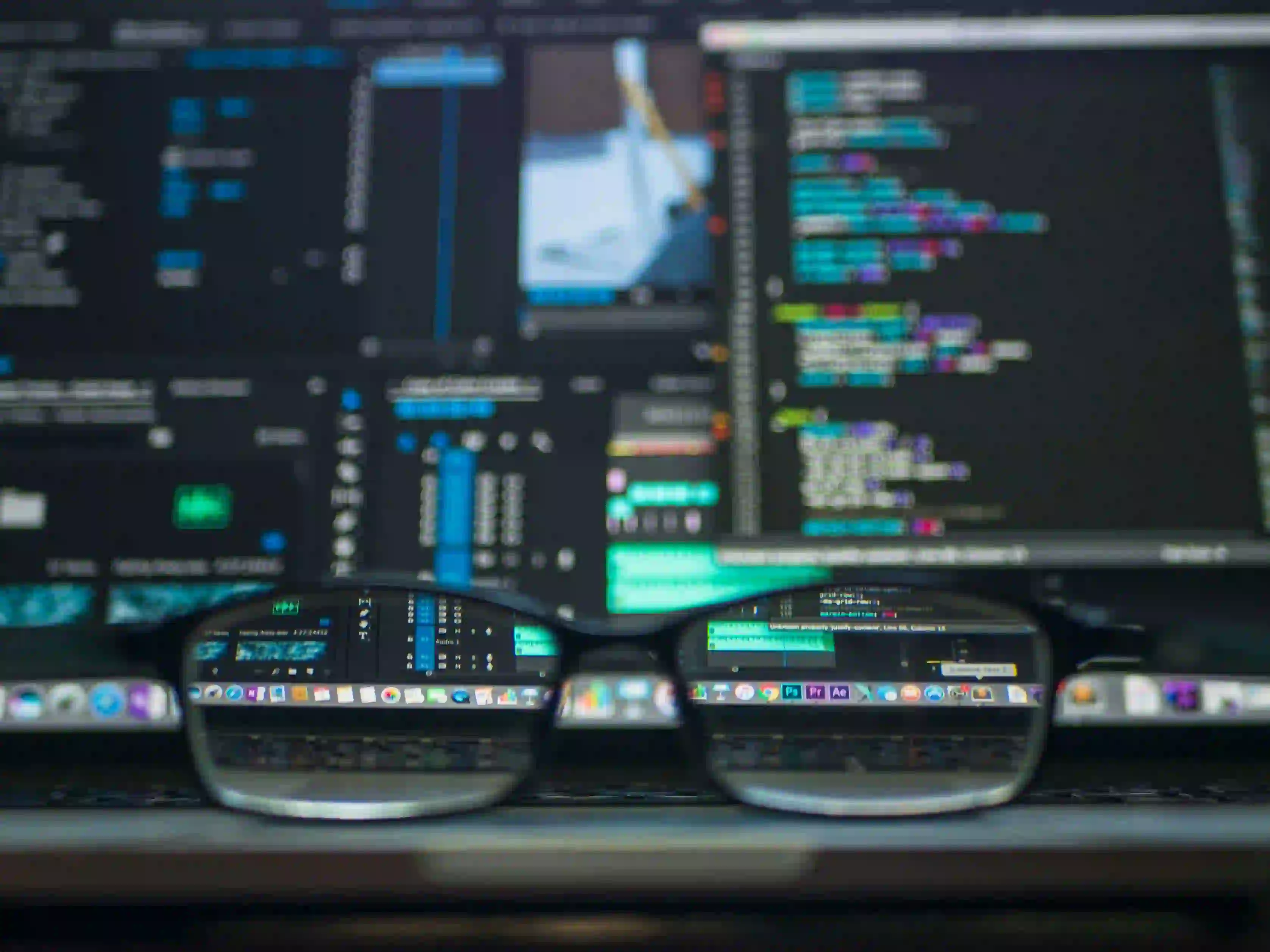
Maximize Java EE Performance: TinyLog's Hidden Logging Issues
Logging is a crucial element of any Java EE application. It not only helps in debugging but also plays a significant role in performance optimization. In this article, we'll explore logging in Java EE, specifically focusing on TinyLog—a lightweight, simple logging library that's often used but may harbor hidden issues impacting application performance.
Understanding TinyLog
TinyLog is known for its user-friendliness and fast performance. Designed to be lightweight, its simplicity allows developers to quickly integrate it into their applications. However, like any tool, it comes with its own set of challenges, especially in high-demand environments typical of Java EE applications.
Key Features of TinyLog
- Simplicity: The API is straightforward, making it easy to configure and use.
- High Performance: TinyLog claims to have minimal overhead, which is why many developers choose it for production systems.
- Flexible Configurations: It supports various output formats and destinations (console, files, etc.).
You can explore more about TinyLog here.
The Importance of Logging in Java EE
In Java EE environments, performance bottlenecks can arise from various sources, including poor logging practices. Good logging provides clear insights into the application flow, errors, and performance metrics. Here’s why effective logging matters:
- Debugging: Helps trace problems without significant overhead.
- Performance Monitoring: Allows developers to monitor critical application metrics, helping identify slow components.
- Auditing: Facilitates tracking user activity and changes in application state.
However, without careful management, logging may contribute to performance degradation.
The Hidden Logging Issues with TinyLog
Despite its advantages, there are hidden issues you may encounter while using TinyLog, especially in a Java EE context:
1. Synchronous Logging Overheads
While TinyLog is lightweight, synchronous logging can introduce latencies in high-throughput applications. Logging messages directly can fall behind in heavy traffic scenarios, resulting in performance bottlenecks.
Example of Synchronous Logging:
// Synchronous logging example
Logger logger = LoggerFactory.getLogger(MyClass.class);
public void processRequest() {
logger.info("Processing request..."); // This call blocks until the message is logged
// Business logic here
}
Why it Matters: The logger.info
line in a high-load scenario can introduce noticeable delays, especially under heavy loads.
Solution: Asynchronous Logging
To mitigate this, consider using an asynchronous logging approach. This can be implemented via a background thread that processes log messages, thus improving overall application throughput.
public class AsyncLogger {
private final BlockingQueue<String> loggingQueue = new LinkedBlockingQueue<>();
private final Logger logger = LoggerFactory.getLogger(AsyncLogger.class);
public AsyncLogger() {
new Thread(this::processQueue).start();
}
public void logAsync(String message) {
loggingQueue.offer(message);
}
private void processQueue() {
while (true) {
try {
String message = loggingQueue.take(); // Blocks until a message is available
logger.info(message);
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore interrupted status
}
}
}
}
// Usage in the application
AsyncLogger asyncLogger = new AsyncLogger();
asyncLogger.logAsync("Processing request asynchronously...");
Why it Matters: The asynchronous logger keeps your main application thread responsive. Although it requires careful management of thread lifecycle and error handling, the advantages in performance in a Java EE setting are often substantial. You'll prevent log statements from slowing down critical application paths.
2. Log Formatting Overhead
TinyLog's flexible log formatting capabilities might become a performance concern if not managed effectively. Complex formatting can consume additional CPU cycles, especially in situations where logging is frequent.
Example of a Complex Log Format:
logger.info("User {} from {} has accessed the resource at {}", userId, userIP, resource);
Why it Matters: If logger.info
is called frequently, this string formatting could introduce a substantial overhead.
Solution: Use Placeholders for Better Performance
Avoid unnecessary complexities in log formats. TinyLog supports string placeholders that optimize performance by only formatting messages that meet the log level criteria.
if (logger.isInfoEnabled()) {
logger.info("User {} from {} has accessed the resource at {}", userId, userIP, resource);
}
Why it Matters: This change optimizes performance by preventing unnecessary formatting calculations for log messages that will not actually be logged.
3. Volume of Log Data
The sheer volume of log data can overwhelm not only your storage but your processing capabilities. In Java EE applications, excessive logging during high-load situations can degrade performance significantly.
Solutions to Manage Log Volume:
- Log Level Configuration: Adjust log levels for different environments to keep only critical information in production.
- Log Rotation: Implement log rotation to manage log file sizes effectively.
Best Practices for Logging in Java EE with TinyLog
- Configure Appropriate Log Levels: Use error levels to ensure only critical information is logged during production.
- Centralized Logging: Consider using a logging aggregator like ELK Stack or Splunk to gather logs from multiple services. This way, you can alleviate the performance burden on a single service.
- Asynchronous Log Handling: Implement an asynchronous logging mechanism as demonstrated above.
- Profile Your Logging: Regularly profile your application to identify logging bottlenecks. Tools like JProfiler or VisualVM can help track performance metrics related to logging.
Closing the Chapter
Logging is an essential part of any Java EE application, but it should not come at the cost of performance. By being mindful of TinyLog's hidden issues—such as synchronous logging overheads, excessive formatting, and log volume—you can ensure that your application runs smoothly without sacrificing critical error and performance insights.
Applying the discussed solutions—such as asynchronous logging and optimized log formatting—will not only improve the performance of your application but also enhance your ability to troubleshoot issues when they arise.
For more in-depth strategies around logging in Java applications, check out Logging Best Practices for valuable insights.
By employing these best practices and solutions, you’re on your way to maximizing the performance of your Java EE applications while still enjoying the benefits of effective logging.
Remember, in the realm of adaptive software development, optimizing logging is as important as optimizing any other critical part of your stack.