Common Java Practices That Can Wreak Havoc on Your Code
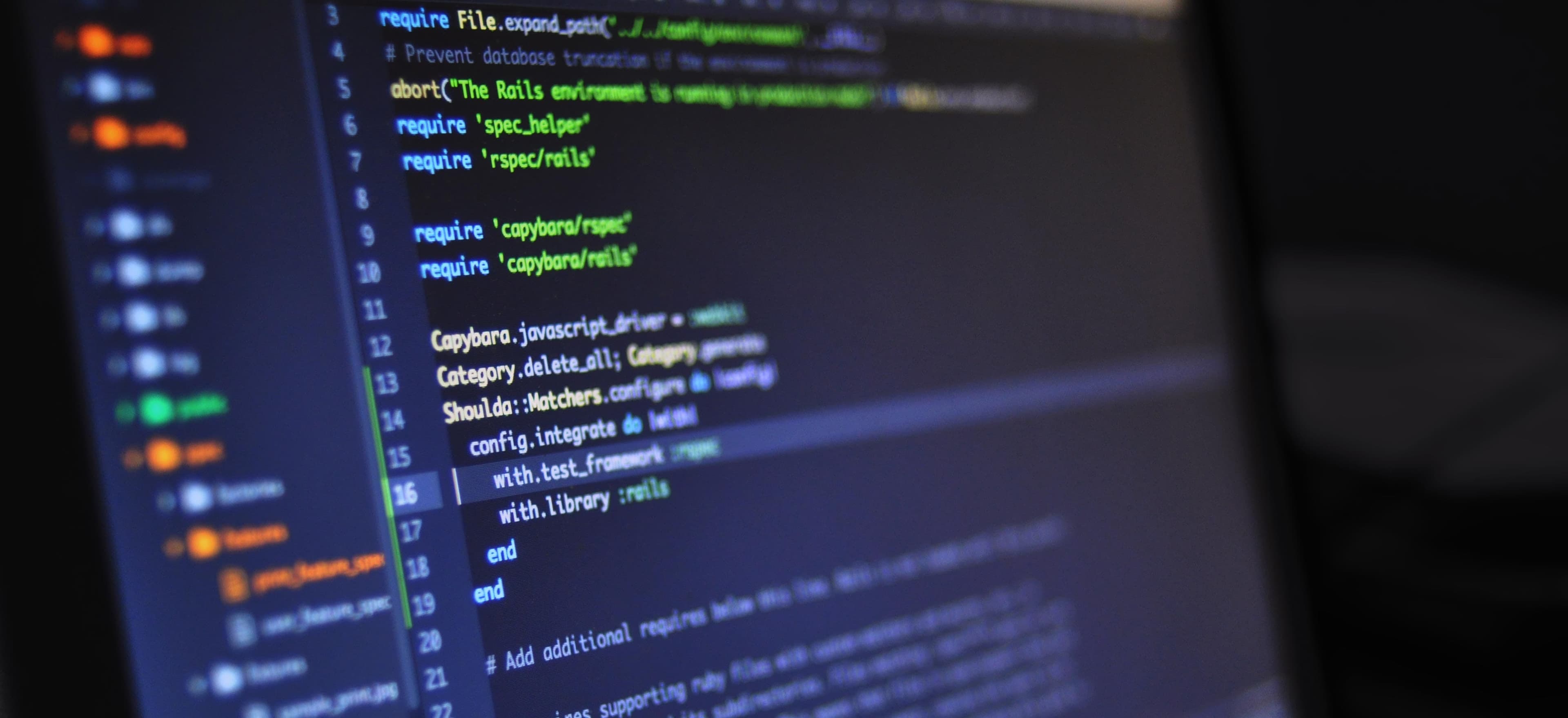
- Published on
Common Java Practices That Can Wreak Havoc on Your Code
Java is a widely-used, robust programming language cherished for its platform independence and versatility. However, even experienced developers can fall into bad habits that can lead to maintainability issues, performance degradation, or worse — catastrophic failures in production. In this post, we will explore common Java practices that developers should avoid to keep their code clean, efficient, and manageable.
1. Ignoring Exceptions
Why It's a Problem
Effective error handling is a crucial part of Java programming. When exceptions are ignored, they can lead to unpredictable behavior and make debugging significantly harder.
Example Code Snippet
public void readFile(String fileName) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
// Reading lines from the file...
} catch (FileNotFoundException e) {
// Ignoring the exception
}
}
Commentary
In this code, the FileNotFoundException
is being ignored. It is essential to handle exceptions properly. Logging the exception or rethrowing it can help you understand what went wrong, which is critical for maintaining code quality. Consider this improved version:
public void readFile(String fileName) {
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
// Reading lines from the file...
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
throw e; // Rethrowing the exception is often a good practice
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
2. Overusing static
Why It's a Problem
While static
members and methods can be helpful, overusing them goes against object-oriented principles and can lead to tightly coupled and hard-to-test code.
Example Code Snippet
public class Utility {
public static double calculateTax(double amount) {
return amount * 0.15;
}
}
Commentary
While the static method here works correctly, it makes testing harder. A better approach could be to use a service-based structure:
public class TaxService {
public double calculateTax(double amount) {
return amount * 0.15;
}
}
This allows for easier unit testing and promotes better encapsulation.
3. Using ==
Instead of .equals()
Why It's a Problem
In Java, ==
checks for reference equality, whereas .equals()
checks for logical equality. Using ==
on objects can lead to unintended results.
Example Code Snippet
String str1 = new String("Hello");
String str2 = new String("Hello");
if (str1 == str2) {
System.out.println("Strings are equal!");
}
Commentary
In this code, even though str1
and str2
contain the same value, the condition will evaluate to false because they reference different objects. Use .equals()
instead:
if (str1.equals(str2)) {
System.out.println("Strings are equal!");
}
4. Not Using Java Collections Properly
Why It's a Problem
Java's Collection Framework provides powerful data structures like List, Set, and Map. Not using them effectively can lead to poor performance and complex code.
Example Code Snippet
List<String> list = new ArrayList<String>();
list.add("one");
list.add("two");
list.add("three");
// Inefficient search operation
for (String item : list) {
if (item.equals("two")) {
System.out.println("Found two!");
}
}
Commentary
In the above example, a linear search is employed. While this suffices for small collections, larger ones can lead to performance issues. For a better approach, consider using a Set for faster lookups:
Set<String> set = new HashSet<String>();
set.add("one");
set.add("two");
set.add("three");
if (set.contains("two")) {
System.out.println("Found two!");
}
5. Hardcoding Values
Why It's a Problem
Hardcoding values can make the code less flexible and harder to maintain. Changes require code modifications, which increases the risk of introducing errors.
Example Code Snippet
public void printPi() {
System.out.println("Value of Pi: 3.14159");
}
Commentary
The hardcoded value of Pi can be stored in a constant or fetched from a configuration file, which is a more flexible way to handle such values:
public static final double PI = Math.PI;
public void printPi() {
System.out.println("Value of Pi: " + PI);
}
6. Poor Naming Conventions
Why It's a Problem
Naming conventions play a pivotal role in code readability. Poorly named variables and methods can confuse others (or yourself) trying to understand the code later.
Example Code Snippet
public class C {
public void m() {
// perform some action
}
}
Commentary
In the example above, the class and method names do not provide any context. A better version would clarify these roles:
public class UserService {
public void createUser() {
// perform user creation
}
}
7. Not Using Java 8 Features
Why It's a Problem
With the introduction of Java 8, features like lambda expressions, Streams, and Optional significantly simplify code and reduce boilerplate. Not leveraging these features can lead to outdated and verbose code.
Example Code Snippet
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
for (String name : names) {
if (name.startsWith("A")) {
System.out.println(name);
}
}
Commentary
Instead of using an explicit loop, consider leveraging the power of Streams:
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
Final Thoughts
Java is a powerful and flexible language, but poor practices can lead to headaches. By understanding and avoiding these common pitfalls, you can write cleaner, more efficient, and maintainable code.
For more information on best practices in Java, consider checking resources like Oracle's Java Documentation and Effective Java by Joshua Bloch.
Additional Resources
- Java Collections Framework
- Java Exception Handling
- Modern Java in Action
By being mindful of these practices and continuously seeking to improve your coding standards, you will significantly increase the quality of your Java applications and bolster your career as a developer.
Checkout our other articles