Understanding CDI Misconceptions in EJB Contexts
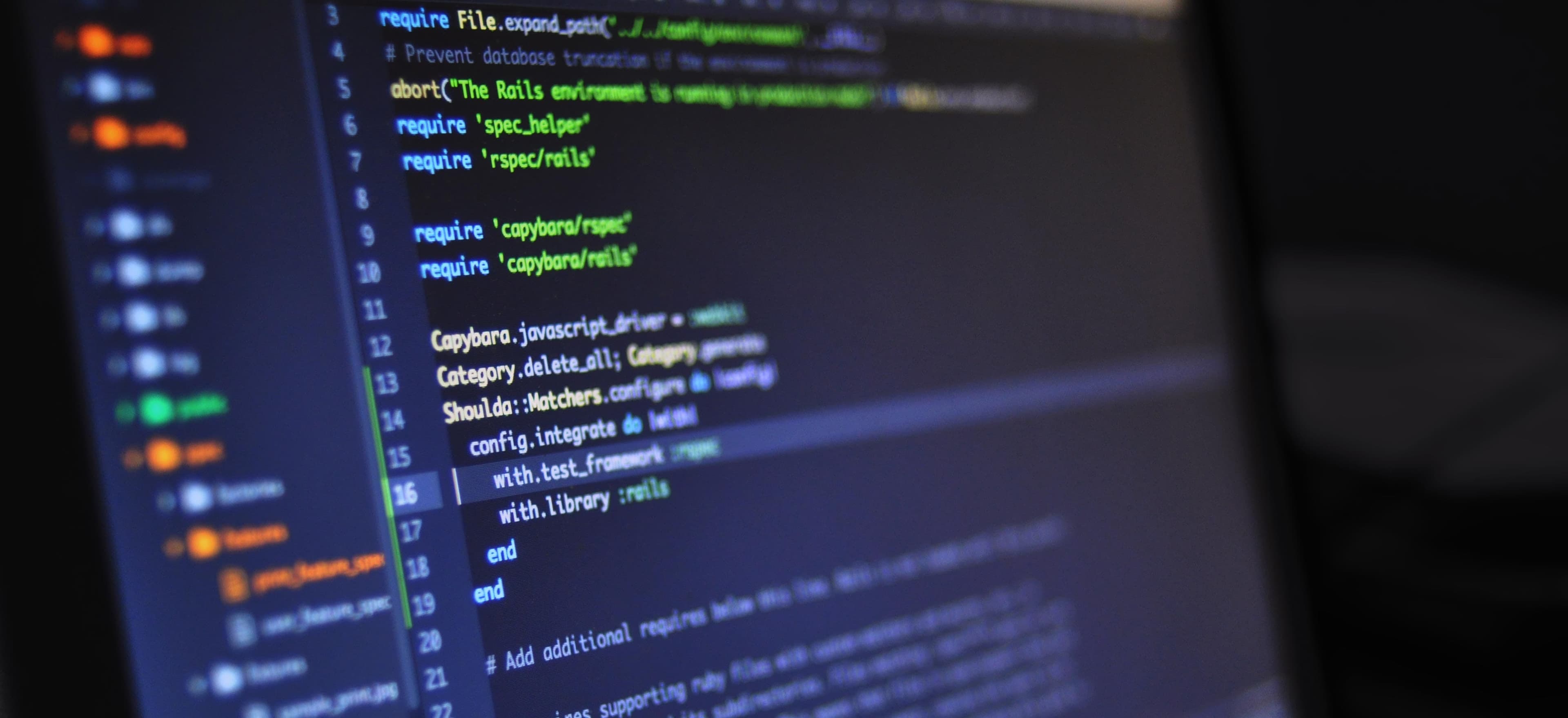
- Published on
Understanding CDI Misconceptions in EJB Contexts
Java EE offers a rich ecosystem for enterprise applications, blending various technologies to make developers' lives easier. Among its robust offerings are Contexts and Dependency Injection (CDI) and Enterprise JavaBeans (EJB). While both are powerful, misconceptions often arise regarding how they interact. This blog post aims to clarify these misconceptions, diving into the nuances of CDI within EJB contexts.
What is CDI?
Context and Dependency Injection (CDI) is a framework in Java EE that provides a powerful set of services for managing the lifecycle and interactions of stateful Java objects (beans). CDI uses dependency injection to allow developers to manage their components and decouple their business logic.
Key Features of CDI:
- Type-safe Dependency Injection: Allows injection of any object type.
- Scopes: Controls the lifecycle of CDI beans (e.g., request, session, application).
- Interceptors: Enables aspects-oriented programming for cross-cutting concerns.
If you want to delve deeper into CDI, the official documentation is a great place to start.
What are EJBs?
Enterprise JavaBeans (EJB) is a specification for developing scalable, transactional, and multi-user secure enterprise-level applications. EJB serves as a server-side component architecture, primarily focused on business logic.
Key Features of EJBs:
- Transaction Management: Handles transactions automatically.
- Concurrency Control: Manages concurrent access to beans.
- Security: Integrates with Java EE security for fine-grained control.
For more information on EJBs, check out the official EJB specifications.
Common Misconceptions About CDI and EJB
1. CDI and EJB are Mutually Exclusive
Misconception: Many believe that if you use CDI, you cannot use EJB and vice versa.
Truth: CDI and EJB can and often do complement each other. CDI beans can be injected into EJBs and vice-versa.
For instance, consider the following example where an EJB is injecting a CDI bean:
import javax.annotation.Stateless;
import javax.inject.Inject;
@Stateless
public class MyService {
@Inject
private MyCDIBean myCDIBean; // CDI Bean Injection
public void performService() {
myCDIBean.execute();
}
}
In this code snippet, MyCDIBean
is a CDI bean that is injected into an EJB (MyService
). Incorporating CDI beans allows you to take advantage of CDI's additional features while leveraging EJB's robust architecture.
2. All CDI Beans are Singleton
Misconception: There is a common belief that all CDI beans are singletons, similar to EJBs.
Truth: CDI provides multiple scopes, including request, session, and application scopes. Not all CDI beans are singletons; the default scope is @Dependent
, meaning the lifecycle of the bean is tied to the lifecycle of the bean that uses it.
Here is an example showing different scopes in CDI:
import javax.enterprise.context.RequestScoped;
import javax.enterprise.context.SessionScoped;
import javax.inject.Inject;
@RequestScoped
public class RequestScopedBean {
public String getRequestScopeMessage() {
return "This bean is request-scoped.";
}
}
@SessionScoped
public class SessionScopedBean {
public String getSessionScopeMessage() {
return "This bean is session-scoped.";
}
}
3. Dependencies Need to be Declared in both EJB and CDI
Misconception: Some developers think they must declare dependencies in both EJB and CDI contexts.
Truth: While you can declare a dependency in either context, you only need to define it in one. CDI allows seamless management of dependencies, so you can inject a CDI bean into an EJB without redundancy.
4. CDI Does Not Handle Transactions
Misconception: Another common myth is that CDI is not capable of managing transactions, leading developers to think they must stick solely to EJB for transactional needs.
Truth: CDI beans can participate in transactions when used in combination with EJB. Through EJB's built-in transaction management, you can assure that CDI beans called within an EJB context are transactional.
Here’s an example:
import javax.annotation.Stateless;
import javax.enterprise.context.Dependent;
import javax.inject.Inject;
import javax.transaction.Transactional;
@Stateless
public class TransactionService {
@Inject
private MyCDIBean myCDIBean;
@Transactional
public void executeTransaction() {
// transactional logic...
myCDIBean.performTask();
}
}
In this case, TransactionService
manages a transaction context while utilizing the functionality of a CDI bean.
5. CDI Cannot Interact with EJB Lifecycle Events
Misconception: Some developers mistakenly believe that CDI beans cannot react to lifecycle events of EJBs.
Truth: CDI introduces event-driven programming paradigms, enabling you to listen to events occurring in EJBs and respond to them appropriately.
For example:
import javax.ejb.Singleton;
import javax.enterprise.event.Event;
import javax.inject.Inject;
@Singleton
public class MyEJB {
@Inject
private Event<MyEvent> myEvent;
public void triggerEvent() {
myEvent.fire(new MyEvent());
}
}
6. CDI Breaks the EJB Statelessness
Misconception: There’s a belief that introducing CDI beans into your architecture compromises the statelessness of EJBs.
Truth: This is not the case. Using CDI does not alter the stateless nature of EJBs when they are designed to be stateless. Proper scope definition ensures that CDI beans respect the architectural boundaries.
Best Practices for Using CDI with EJBs
- Use CDI for Configuration and State Management: Leverage CDI to manage stateful components while keeping EJBs stateless where possible.
- Scope Appropriately: Choose appropriate scopes (request, session) for CDI beans to maximize performance.
- Event-Driven Programming: Utilize events to decouple components and promote a clean architecture.
Final Thoughts
Understanding the interplay between CDI and EJB allows developers to create more powerful and maintainable enterprise applications. By dispelling common misconceptions, you can harness the full potential of both technologies, developing scalable, secure, and maintainable solutions.
For additional resources, refer to Java EE Tutorial to deepen your understanding and exploration of CDI and EJB.
Whether you're an experienced Java EE developer or just embarking on your journey, understanding and leveraging CDI alongside EJB is not just beneficial; it's essential. Happy coding!
Checkout our other articles