Unlocking Java 7: Overcoming MethodHandle Complexity
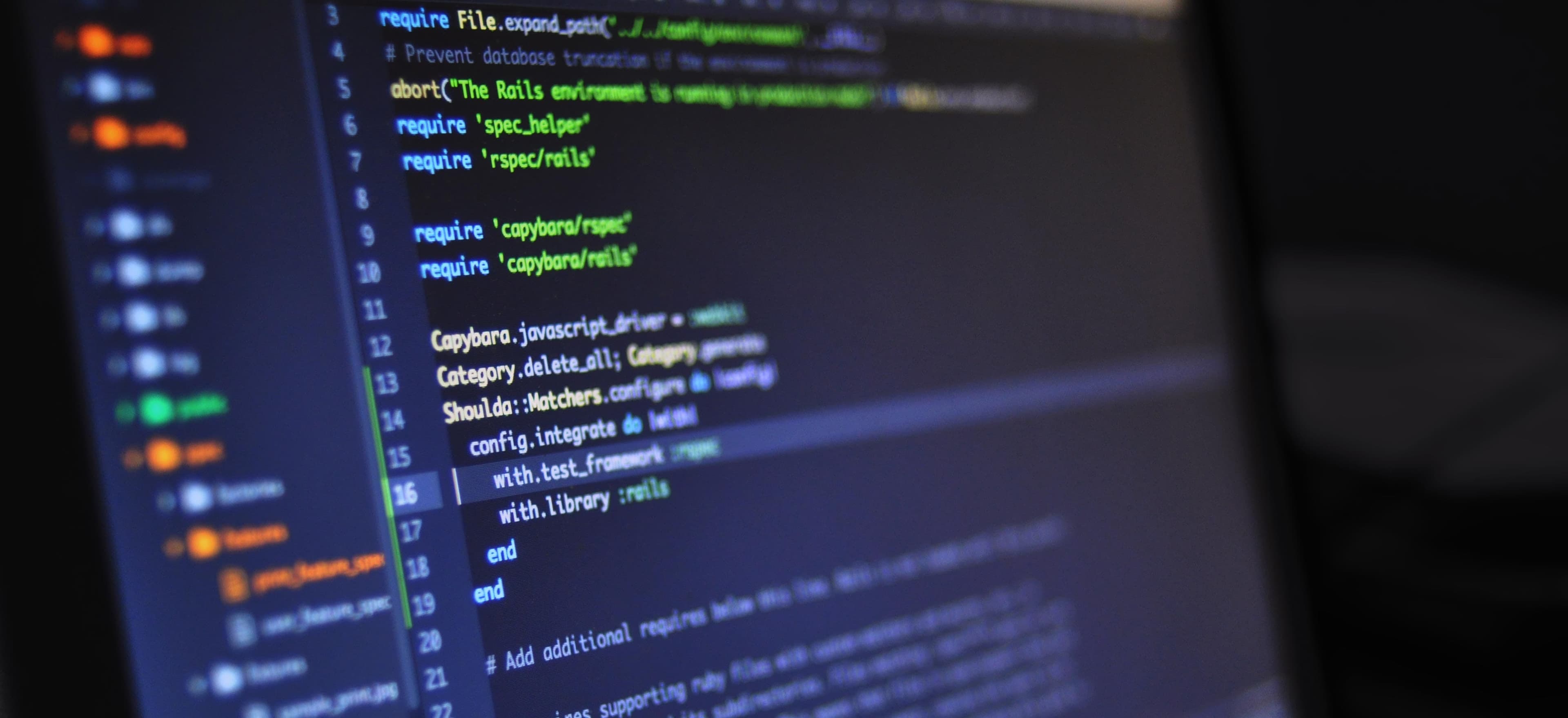
- Published on
Unlocking Java 7: Overcoming MethodHandle Complexity
Java, since its inception, has continually evolved. Among the many enhancements introduced, Java 7 brought a powerful new feature: MethodHandle
. However, as exciting as it is, many developers find themselves overwhelmed by its complexity. In this blog post, we will demystify MethodHandle
, explore its capabilities, and provide practical examples. By the end, you will see how to leverage these tools effectively in your Java applications.
What are MethodHandles?
MethodHandle
is part of the java.lang.invoke
package and serves as a dynamic method invocation mechanism. It allows developers to create flexible and reusable code that is somewhat analogous to reflection but with improved performance and type safety.
With MethodHandle
, you can invoke methods, constructors, and fields dynamically while avoiding the overhead associated with standard reflection. This makes it not just a replacement for traditional Java reflection but a more efficient alternative.
Why Use MethodHandles?
- Performance: MethodHandles are faster compared to traditional reflection due to stronger optimizations they allow by the JVM.
- Flexibility: They provide a way to create dynamic behaviors at runtime.
- Strongly Typed: Unlike traditional reflection, method handles are strongly typed, reducing the chances of runtime errors.
Getting Started with MethodHandles
Before diving deep, let’s create a simple example that demonstrates how to create and invoke a MethodHandle
.
Step 1: Creating a MethodHandle
The first step is to obtain a MethodHandle
. You can do this using the MethodHandles.Lookup
class. This class provides the ability to look up and link method handles.
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
public class MethodHandleExample {
public void printMessage(String message) {
System.out.println(message);
}
public static void main(String[] args) {
try {
// Step 1: Obtain a MethodHandles.Lookup
MethodHandles.Lookup lookup = MethodHandles.lookup();
// Step 2: Define the type of the method we want to handle
MethodType methodType = MethodType.methodType(void.class, String.class);
// Step 3: Retrieve the MethodHandle for printMessage
MethodHandle methodHandle = lookup.findVirtual(MethodHandleExample.class, "printMessage", methodType);
// Step 4: Create an instance of MethodHandleExample
MethodHandleExample example = new MethodHandleExample();
// Step 5: Invoke the method handle
methodHandle.invoke(example, "Hello, MethodHandle!");
} catch (Throwable throwable) {
throwable.printStackTrace();
}
}
}
Why This Code Works:
- MethodHandles.Lookup: This object allows us to dynamically look up the methods we want to invoke.
- MethodType: Defines the method's return type and parameter types, ensuring the correct method is targeted.
- findVirtual: Locates the instance method and creates a
MethodHandle
that points to it. - invoke: Finally, you call the method with the handle, passing the instance and arguments.
Practical Use Cases
Now that you understand the basic mechanics, let’s explore some practical scenarios where using MethodHandles
shines.
Dynamic Method Invocation
Consider a framework where you need to invoke methods dynamically based on user input. Using MethodHandles
, you can achieve this without extensive boilerplate code.
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
public class DynamicInvocation {
public void greeting(String name) {
System.out.println("Hello, " + name + "!");
}
public void farewell(String name) {
System.out.println("Goodbye, " + name + "!");
}
public void invokeMethod(String methodName, String arg) {
try {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodType methodType = MethodType.methodType(void.class, String.class);
MethodHandle handle = lookup.findVirtual(DynamicInvocation.class, methodName, methodType);
handle.invoke(this, arg);
} catch (Throwable throwable) {
throwable.printStackTrace();
}
}
public static void main(String[] args) {
DynamicInvocation di = new DynamicInvocation();
di.invokeMethod("greeting", "Alice");
di.invokeMethod("farewell", "Alice");
}
}
Why This Code is Effective:
This example illustrates how to dynamically invoke different methods based on a string input. By using MethodHandles
, you can manage method calls efficiently at runtime, making the code more adaptable to changing requirements.
MethodHandles and Functional Interfaces
MethodHandles
can also be integrated with Java's functional programming features, such as lambdas. This opens up a world of possibilities for building event-driven systems or callback handlers.
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
import java.util.function.Consumer;
public class FunctionInterfaceDemo {
public void message(String msg) {
System.out.println(msg);
}
public Consumer<String> createConsumer(String methodName) {
try {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodType methodType = MethodType.methodType(void.class, String.class);
MethodHandle handle = lookup.findVirtual(FunctionInterfaceDemo.class, methodName, methodType);
return (msg) -> {
try {
handle.invoke(this, msg);
} catch (Throwable throwable) {
throwable.printStackTrace();
}
};
} catch (Throwable throwable) {
throwable.printStackTrace();
}
return null;
}
public static void main(String[] args) {
FunctionInterfaceDemo demo = new FunctionInterfaceDemo();
Consumer<String> consumer = demo.createConsumer("message");
consumer.accept("Hello from Consumer!");
}
}
Why This Implementation Works:
In this setup, MethodHandles
is used to create a Consumer
that can call the message
method dynamically. It demonstrates the synergy between dynamic method invocation and functional interfaces, expanding the flexibility and reusability of your code.
The Pitfalls to Avoid
Although MethodHandles
provides significant advantages, there are potential pitfalls:
- Complexity: The API can be convoluted for newcomers. Understanding the differences between
MethodHandles
and reflection is crucial. - Performance Misconceptions: While they offer improved performance, misunderstanding how they are optimized can lead to misuse.
- Accessibility Issues: Ensure that methods you wish to invoke are accessible to the
MethodHandle
.
Lessons Learned
Java 7's MethodHandle
feature represents a significant shift in how Java handles dynamic method invocation. While it can be intimidating at first due to its complexity, understanding its mechanics unlocks powerful capabilities and tremendous performance benefits.
By leveraging MethodHandles
, you can create more flexible, efficient, and reusable code. As Java continues to evolve, keeping abreast of such technologies will serve you well in your development journey.
For more comprehensive information on MethodHandles, refer to the official Java documentation or explore Java 7's features.
Happy Coding!
Checkout our other articles