Mastering Security: Crafting Strong App Requirements
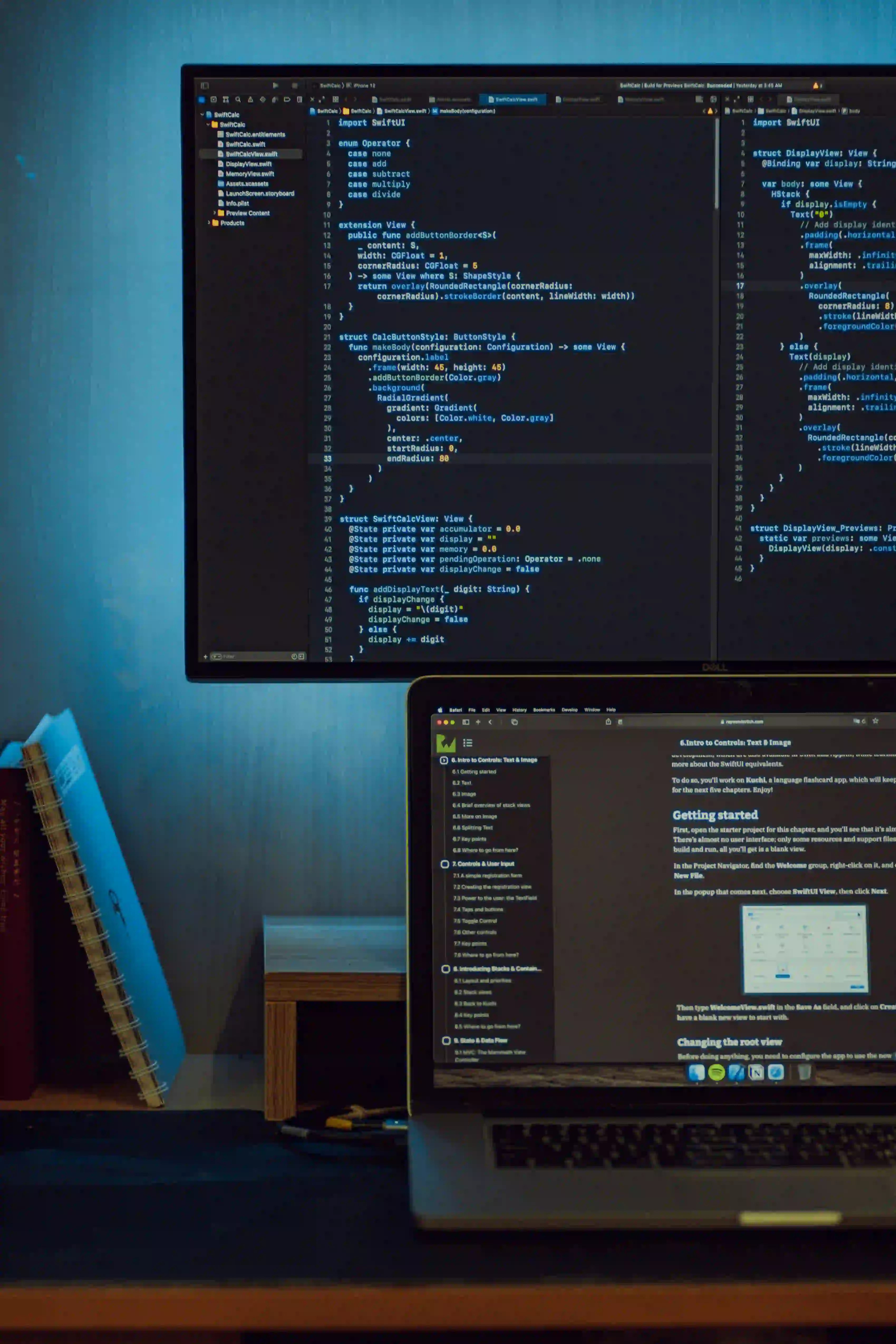
Mastering Security: Crafting Strong App Requirements in Java
In the ever-evolving landscape of technology, security remains a significant concern for developers. As apps become more complex and interconnected, how do we ensure that they are resistant to threats and vulnerabilities? The answer lies in crafting robust application requirements that prioritize security from the very beginning. In this blog post, we will explore the essential aspects of writing secure application requirements, aimed specifically at Java developers.
Why Secure Application Requirements Matter
Often, security is treated as an afterthought, leading to vulnerabilities that can be exploited. Crafting strong app requirements reduces the risk of security flaws. It provides a clear roadmap for developers to follow during the software development lifecycle.
According to OWASP, a well-defined security requirement can mitigate at least 70% of security issues. More so, it fosters a culture of accountability among all team members involved in the development process.
Key Elements of Strong Security Requirements
To create strong security-focused application requirements, consider these essential elements:
- Identify Sensitive Data
- Access Control Requirements
- Common Security Standards
- Audit and Logging Requirements
- Error Handling and Security Notifications
Letβs break down each of these components.
1. Identify Sensitive Data
In modern applications, sensitive data can take many forms: personally identifiable information (PII), payment details, healthcare records, etc.
Example Requirement:
The application must encrypt all user data, including email, password, and payment details, using AES-256 encryption before storing it in the database.
Why This Is Important: Failing to protect sensitive data not only jeopardizes user privacy but could also lead to severe legal and compliance issues.
Java Implementation:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class EncryptionUtil {
public static String encrypt(String data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encrypted = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
}
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256);
return keyGen.generateKey();
}
}
// Sample usage
SecretKey secretKey = EncryptionUtil.generateKey();
String encryptedData = EncryptionUtil.encrypt("SensitiveData", secretKey);
2. Access Control Requirements
Defining roles and permissions is crucial. Ensure that only authorized users can access specific functionalities.
Example Requirement:
Users can only access their personal profiles and not the profiles of others unless they are part of the admin role.
Why This Is Important: Clear access control policies prevent unauthorized access, safeguarding user data.
Java Implementation:
public class User {
private String role;
public boolean canAccessProfile(User requestedUser) {
if (this.role.equals("admin")) {
return true; // Admin can access all profiles
}
return this.equals(requestedUser); // Regular user can only access personal profile
}
}
3. Common Security Standards
Align your application with recognized security practices, such as those outlined in the OWASP Top Ten.
Example Requirement:
The application must be designed to mitigate the risks of injections, such as SQL and XSS attacks, by using prepared statements and output encoding.
Why This Is Important: Adhering to industry standards creates a baseline for security, enabling a more systematic approach to risk management.
4. Audit and Logging Requirements
Implementing proper logging can help trace back any misuse and is often used for compliance purposes.
Example Requirement:
The application must log all user authentication attempts, including both successful and failed logins, while ensuring that sensitive information is not logged.
Why This Is Important: Robust logging practices can identify and resolve security breaches more effectively.
Java Implementation:
import java.util.logging.Logger;
public class LoginManager {
private static final Logger logger = Logger.getLogger(LoginManager.class.getName());
public void authenticate(String username, String password) {
boolean successfulLogin = checkCredentials(username, password);
if (successfulLogin) {
logger.info("User " + username + " successfully logged in.");
} else {
logger.warning("Failed login attempt for user " + username);
}
}
private boolean checkCredentials(String username, String password) {
// Credential verification logic here
return false; // Placeholder
}
}
5. Error Handling and Security Notifications
Handle errors gracefully while preventing the exposure of sensitive error information to the user.
Example Requirement:
The application must not expose stack traces or sensitive error details to end users, instead providing generic error messages while logging complete error details on the server-side.
Why This Is Important: Exposing error details can give attackers insight into potential vulnerabilities.
Java Implementation:
public class ErrorHandler {
public void handle(Exception e) {
System.err.println("A generic error occurred. Please try again."); // User-friendly error
logError(e); // Log complete error details for debugging
}
private void logError(Exception e) {
// Logging logic goes here
e.printStackTrace(); // Log complete stack trace
}
}
Testing the Requirements
Once the requirements have been established, the next step is to ensure they are adequately tested. Implementing security testing tools like SAST (Static Application Security Testing) and DAST (Dynamic Application Security Testing) can help identify vulnerabilities early in the development lifecycle.
Wrapping Up
Crafting strong application requirements focused on security is vital for any Java developer. Through clear and robust directives, we not only safeguard our applications against potential threats but also build a culture of security awareness. By focusing on elements like sensitive data protection, access control, security standards, logging, and error handling, we can create applications that stand the test of time in a challenging security landscape.
For further exploration of best practices in secure application development, consider checking out the OWASP Security Principles or Secure Coding Guidelines.
Stay secure, and happy coding!