Common Pitfalls in Code Optimization Decisions
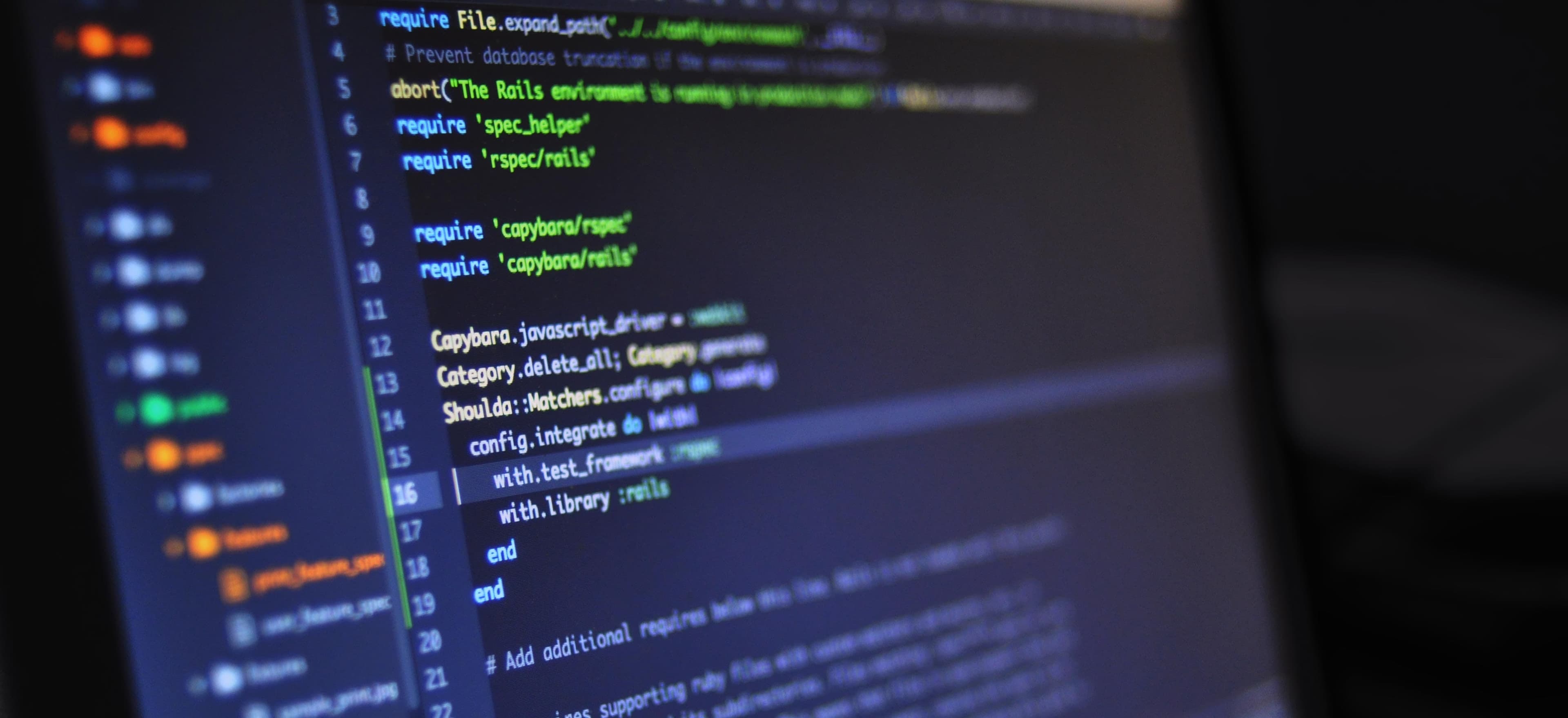
- Published on
Common Pitfalls in Code Optimization Decisions
In the ever-evolving world of software development, code optimization remains a critical area of focus. Enhancing the performance of your application can lead to faster execution times, reduced resource consumption, and an overall improved user experience. However, there are several common pitfalls developers encounter when optimizing their code. Understanding these pitfalls is crucial to make informed decisions that genuinely enhance your code's effectiveness without introducing new problems.
Table of Contents
- Understanding Optimization
- The Premature Optimization Trap
- Ignoring Code Readability
- Dismissing Algorithm Complexity
- Overlooking Bottleneck Analysis
- Neglecting the Power of Profiling
- Inconsistencies in Testing
- Conclusion
- Further Reading
Understanding Optimization
Code optimization involves making changes to your software to improve its performance. This can mean reducing execution time, memory usage, or improving throughput. That said, not every optimization effort yields tangible benefits, and sometimes these changes can introduce new issues.
For any optimization strategy to be effective, one must start with a solid understanding of the original code’s structure and functionality. Before diving into a series of optimizations, it's essential to analyze where the current inefficiencies lie.
The Premature Optimization Trap
One of the cardinal sins in programming is premature optimization. Often attributed to Donald Knuth, the phrase emphasizes that while optimizing is important, doing so before fully understanding the problem can lead you astray:
public int sum(int[] numbers) {
int total = 0;
for (int number : numbers) {
total += number; // Simple loop, easy to understand
}
return total;
}
In the example above, while you might consider rewriting the loop using parallel streams or other advanced techniques to speed up execution, the overhead of such an approach could outweigh the benefits, especially for small arrays. Focus on writing clean, understandable code first. Optimize later when you have identified performance bottlenecks.
Ignoring Code Readability
Code readability should never be sacrificed in the pursuit of performance. Optimized code can become complex and challenging to maintain, leading to potential bugs and increased technical debt. Consider this example:
public int calculateMax(int[] numbers) {
return Arrays.stream(numbers) // Stream API for elegance
.reduce(Integer.MIN_VALUE, Integer::max);
}
In this case, the use of the functional style with streams is both readable and concise. If you were to optimize by switching to a more complex algorithm for performance, you might inadvertently decrease the code's maintainability and understanding for other developers.
In software engineering, the principle of "Readability Over Everything Else" should be followed first, as clearer code tends to have fewer bugs.
Dismissing Algorithm Complexity
Another frequent pitfall is ignoring the algorithm's complexity. Not all fast implementations are efficient in every scenario. For example, complexity in algorithms is classified in Big O notation—failing to consider this can lead to undesirable performance in larger datasets.
Let's look at two approaches to searching an element in a list:
Linear Search
public boolean linearSearch(int[] numbers, int target) {
for (int number : numbers) {
if (number == target) return true; // O(n)
}
return false;
}
Binary Search
public boolean binarySearch(int[] numbers, int target) {
Arrays.sort(numbers); // O(n log n)
int left = 0;
int right = numbers.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (numbers[mid] == target) return true; // O(log n)
if (numbers[mid] < target) left = mid + 1;
else right = mid - 1;
}
return false;
}
In scenarios where the dataset is large and sorted, the binary search performs significantly better than the linear search—highlighting the importance of choosing the right algorithm based on use-case scenarios.
Overlooking Bottleneck Analysis
Failing to perform a bottleneck analysis can result in optimizing sections of code that do not contribute significantly to overall performance.
Using performance profiling tools helps you identify where the most time is being spent. Java provides the Java Flight Recorder and VisualVM, which can be invaluable in profiling applications:
- Profile the application: Identify which methods consume the most CPU or memory.
- Focus on time-costly methods: Once identified, Java methods that take up a significant amount of time should be optimized first.
Neglecting the Power of Profiling
Profiling is often overlooked but remains one of the best practices in optimization. Profiles reveal where your application spends most of its execution time, guiding your optimization efforts. Without profiling, you could easily engage in a misguided optimization venture.
For instance, let's say you improve a specific algorithm that barely impacts the execution time. Meanwhile, an unrelated function may be the true performance halt:
public void performHeavyCalculations() {
// Some calculations here
}
// HeavyCalculations runs much slower than anticipated...
profiler.start("HeavyCalculations");
// ...perform task...
profiler.stop();
Using the profiler early in development helps to prevent wasting time on premature optimizations.
Inconsistencies in Testing
Final but vital is the consistency in testing. When you modify your code for optimization, ensure that your tests reflect these changes. This helps you ascertain if performance improves, regresses, or has unintended side effects.
Utilizing a testing framework like JUnit in your Java applications is essential:
import org.junit.Assert;
import org.junit.Test;
public class OptimizationTests {
@Test
public void testLinearSearchWorks() {
int[] numbers = {1, 2, 3, 4, 5};
Assert.assertTrue(linearSearch(numbers, 3));
}
@Test
public void testBinarySearchWorks() {
int[] numbers = {1, 2, 3, 4, 5};
Assert.assertTrue(binarySearch(numbers, 3));
}
}
By rigorously testing after each optimization, you ensure continuous functionality and performance gains.
The Closing Argument
Optimization is essential but requires a thoughtful approach. By sidestepping the common pitfalls like premature optimization, neglecting readability, and ignoring algorithm complexities, you’ll not only improve performance but also maintain code quality.
Adhering to best practices in profiling and testing guarantees a smooth transition throughout your development cycle and extends the life of your application. Always prioritize maintainability without forgetting the goal of giving users a seamless experience.
Further Reading
For additional guidance on optimization practices in Java, explore:
- Effective Java by Joshua Bloch.
- Java Performance: The Definitive Guide by Scott Oaks.
These resources can deepen your understanding and proficiency in Java optimization techniques, ensuring that you navigate potential pitfalls effectively. Happy coding!