Common Errors in Google App Engine Task Queues and Fixes
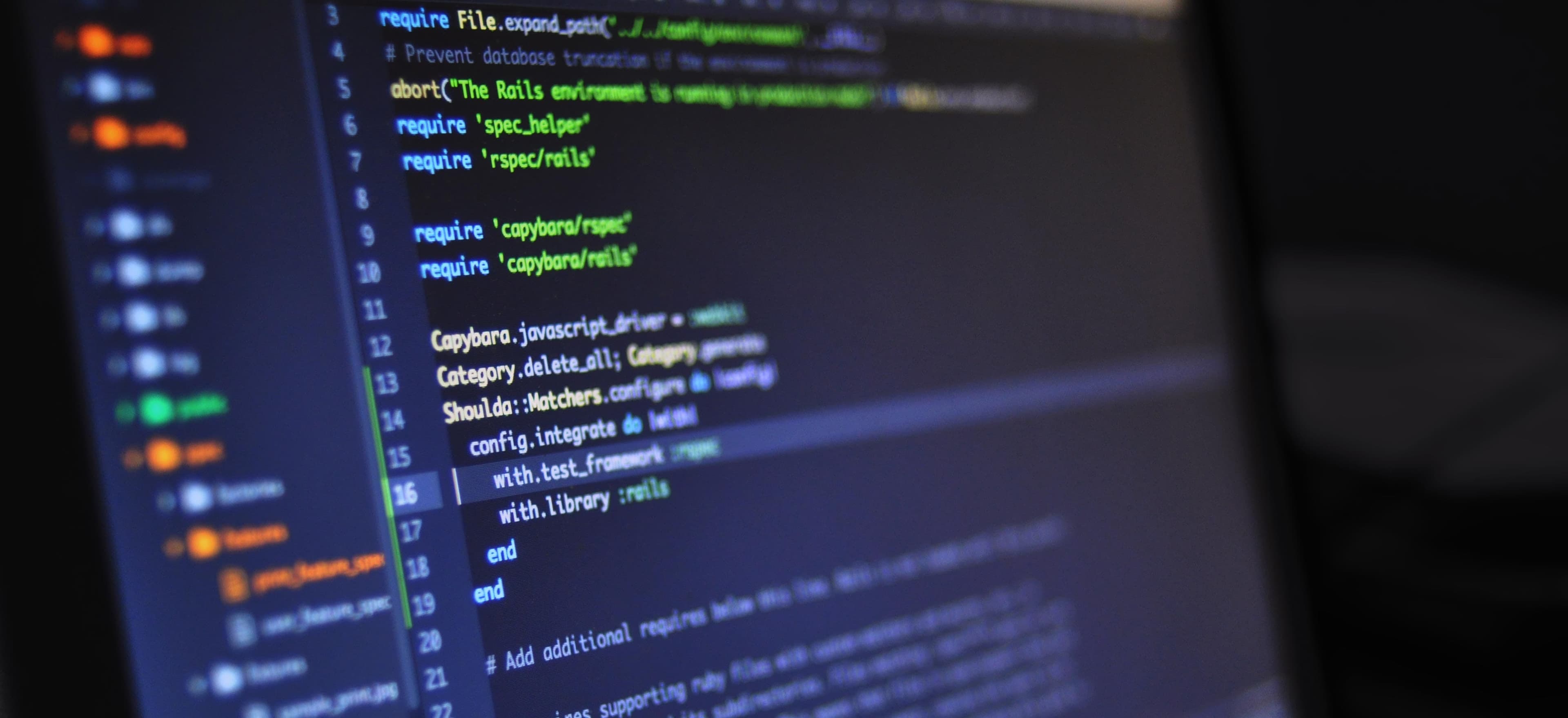
- Published on
Common Errors in Google App Engine Task Queues and Fixes
Google App Engine (GAE) provides a robust platform for deploying web applications, offering a myriad of services, including Task Queues. Task Queues facilitate the management and execution of asynchronous tasks, allowing developers to break heavy workloads into smaller, manageable tasks. However, working with Task Queues can sometimes lead to errors that can hinder the performance of your application. In this blog post, we will explore common errors encountered in Google App Engine Task Queues and provide effective solutions.
Understanding Task Queues
Before diving into common errors, it's crucial to understand what a Task Queue is and how it functions. In GAE, a Task Queue is a mechanism to manage background tasks. You can enqueue tasks that can later be processed independently from the main application. This decoupling allows for smoother user experiences, especially in high-load applications.
When you enqueue a task, you're effectively telling GAE to perform a certain action at a later time. The taskqueue
library in GAE makes these operations straightforward.
Sample Code for Enqueuing a Task
Here’s how you can create and enqueue a simple task:
import com.google.appengine.api.taskqueue.TaskOptions;
import com.google.appengine.api.taskqueue.Queue;
import com.google.appengine.api.taskqueue.QueueFactory;
// Create or get a Queue instance
Queue queue = QueueFactory.getDefaultQueue();
// Create a task
queue.add(TaskOptions.Builder.withUrl("/backgroundtask"));
Why this code? The code above initializes a default task queue and enqueues a task that will be processed at the URL specified (/backgroundtask
). This enables your app to handle tasks in the background, improving user experience by offloading heavy processing.
Common Errors in Task Queues
Errors in Task Queues can arise from various sources, including coding mistakes, misconfiguration, and limitations imposed by Google Cloud services. Below are some of the common errors encountered, along with their solutions.
1. Task Not Found Error
Symptoms
You might see an error like "Task not found" if you attempt to retrieve or access a task that no longer exists.
Fix
Check if the task was successfully enqueued and hasn't been deleted. Ensure you are not retrieving a task that has already been executed or deleted due to time expiration. Implement logging to confirm task creation.
2. Over Quota Error
Symptoms
This error indicates that you've exceeded your task queue quota, leading to delays or failures in task processing.
Fix
- Optimize Task Usage: Review your application to see if there are redundant tasks that can be merged or eliminated.
- Increase Quota: For users with a high volume of tasks, it's recommended to request an increase in quota through the Google Cloud Console.
3. Timeout Error
Symptoms
If a task runs longer than the maximum execution time (10 minutes for HTTP tasks), it will time out and fail.
Fix
- Break Down Tasks: Split up longer tasks into smaller, shorter tasks that can finish within the time limit.
- Use Pull Queues: Consider using a pull queue approach, which allows more control over task processing.
Sample Code for Break Down Tasks
Here’s a code snippet illustrating how to create smaller tasks:
import com.google.appengine.api.taskqueue.TaskOptions;
import com.google.appengine.api.taskqueue.QueueFactory;
// Logic to break a large task into smaller ones
for (int i = 0; i < numberOfSmallTasks; i++) {
Queue queue = QueueFactory.getDefaultQueue();
queue.add(TaskOptions.Builder.withUrl("/smalltask").param("taskNum", String.valueOf(i)));
}
Why this is important? By dividing tasks into smaller chunks, each task has a much higher chance of completing successfully within the allotted time, thus optimizing resource usage.
4. Incorrect Task URL
Symptoms
If the URL specified in your task options is incorrect or unreachable, the task will fail to execute.
Fix
Double-check the task URL, ensuring:
- The URL is registered and can handle the request.
- The HTTP method is appropriate (GET or POST).
5. Missing Permissions/Error 403
Symptoms
You may encounter an "Access Denied (403)" error if your task is trying to access a resource for which it lacks permissions.
Fix
Adjust the permissions by ensuring that the service account executing the task has the necessary IAM roles to access the particular resource.
Sample Code to Check Permissions
import com.google.cloud.tasks.v2.CloudTasksClient;
import com.google.cloud.tasks.v2.QueueName;
CloudTasksClient client = CloudTasksClient.create();
QueueName queueName = QueueName.of(projectId, location, queueId);
// Ensure proper permissions are in place
if (!checkUserPermissions(user)) {
throw new SecurityException("User lacks permissions to access this queue");
}
Why implement permission checks? Ensuring only authorized users or services can perform task operations helps protect against any potential security vulnerabilities.
Best Practices for Task Queues
-
Use Retry Policies: Implement retry strategies to handle transient failures. Customize the retry parameters according to your application's needs.
-
Monitor Queue Metrics: Use Google Cloud Monitoring to observe metrics for task execution. This will help you pinpoint issues quickly.
-
Test Thoroughly: Testing your task implementations is vital. Simulate various scenarios to ensure your queues behave as expected.
-
Documentation: Familiarize yourself with Google Cloud Task Queues documentation for updated best practices and changes.
-
Use Proper Exception Handling: Utilize try-catch blocks to handle exceptions gracefully and log useful information during errors.
Final Thoughts
While Google App Engine's Task Queues are powerful, knowing how to troubleshoot common errors ensures a smoother development experience. Understanding potential pitfalls and the necessary preventive measures allows developers to focus on building robust, scalable applications without being bogged down by common issues.
By applying best practices, thoroughly understanding task durations, and continually monitoring your queues, you can leverage the full potential of Google App Engine Task Queues.
For more extensive resources and insights, check out:
Feel free to share your experiences with Task Queues or any trouble you have faced in the comments below!