Common Misconceptions in Multithreading Interviews
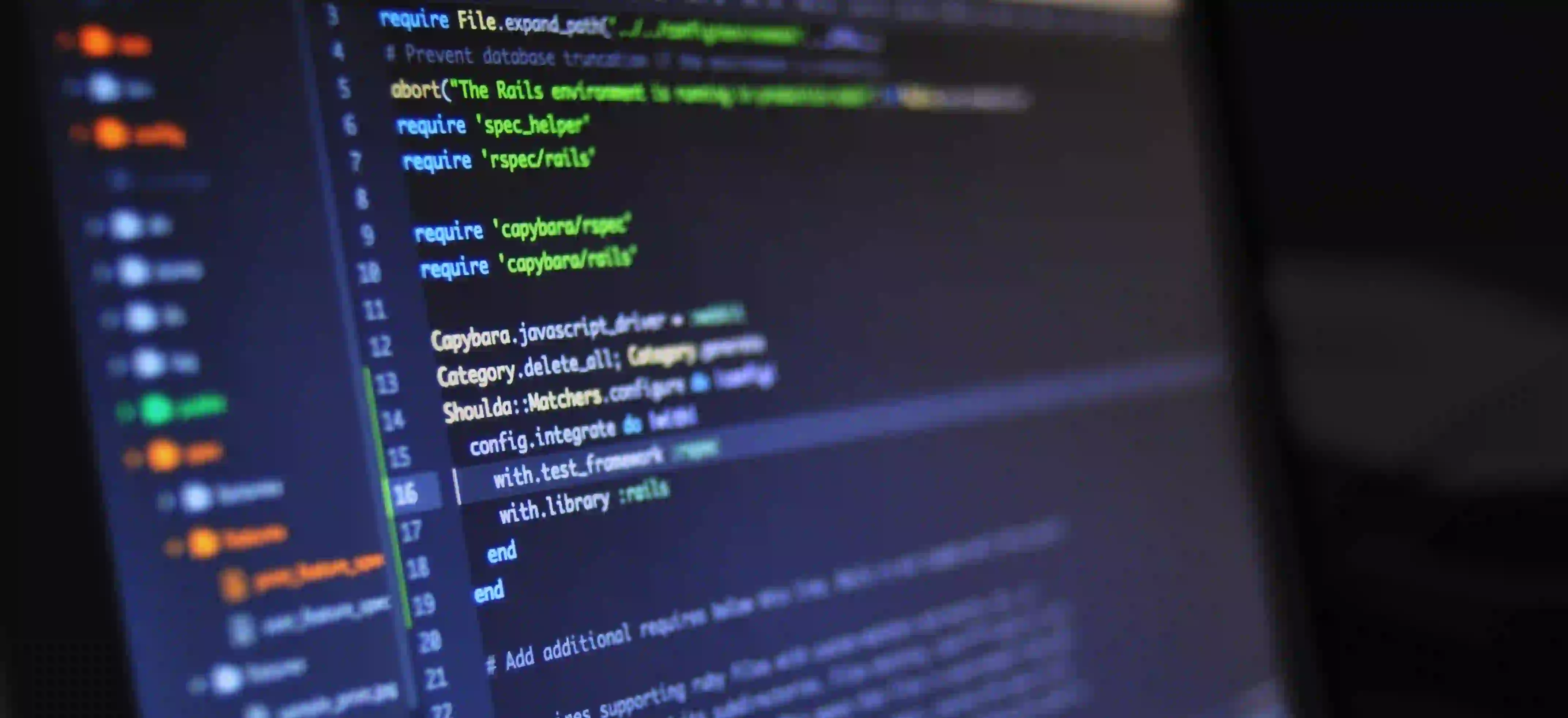
Common Misconceptions in Multithreading Interviews
Multithreading is an essential concept in computer science, particularly in Java programming. It allows multiple threads to run concurrently, providing efficient execution and responsive applications. However, during interviews, candidates often face various misconceptions regarding multithreading. In this blog post, we aim to clarify these misunderstandings and provide a robust understanding of multithreading principles in Java.
Understanding Multithreading
Before diving into the common misconceptions, let’s briefly discuss what multithreading is. In Java, multithreading refers to the concurrent execution of two or more threads—the smallest sequence of programmed instructions. It allows for better resource utilization and improved application performance. For an in-depth understanding of threads in Java, consider checking the official Java tutorials on Threads.
Misconception 1: All Threads Share the Same Memory
Reality: While all threads in a Java application share the same memory space, that does not mean they share data safely. Each thread can access shared variables but needs proper synchronization to avoid issues such as race conditions.
Example Code
public class Counter {
private int count = 0;
public void increment() {
count++; // Not thread-safe
}
public int getCount() {
return count;
}
}
Here, the increment
method is not thread-safe. If multiple threads call this method simultaneously, it may lead to inconsistent counts due to race conditions. To prevent this, synchronization will be necessary.
Solution with Synchronization
public synchronized void increment() {
count++;
}
By adding the synchronized
keyword, you ensure that only one thread can execute the increment
method at any given time, thus protecting the shared resource.
Misconception 2: Multithreading Always Improves Performance
Reality: While multithreading can improve performance, it is not always the case. Creating and managing multiple threads incurs overhead. If the tasks are too lightweight or if there is excessive context switching, performance can degrade.
When to Use Multithreading
-
Long-Running tasks: Use multithreading for tasks that take a significant amount of time, such as I/O operations, network calls, or complex computations.
-
CPU-bound tasks: If your application is CPU-intensive, dividing the workload among multiple threads affords better CPU utilization.
Example of Efficient vs. Inefficient Multithreading
public class Task implements Runnable {
@Override
public void run() {
// Simulate a long running task
System.out.println("Starting task in thread: " + Thread.currentThread().getName());
try {
Thread.sleep(2000); // Simulate time-consuming task
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Task finished in thread: " + Thread.currentThread().getName());
}
}
In this example, using multithreading would be advantageous, as each thread is executing a long-running task. In contrast, if the task were trivial, spawning multiple threads would only add overhead and potentially slow down execution.
Misconception 3: Synchronization is Always Necessary
Reality: While synchronization is crucial for shared resources, it is not needed for every scenario. Over-synchronizing can lead to bottlenecks and reduced performance.
Safe Operations on Local Variables
Local variables are stored on the stack and are thread-safe by default. For instance:
public class MyRunnable implements Runnable {
public void run() {
int localVariable = 5; // Thread-safe
System.out.println("Local Variable: " + localVariable);
}
}
In this case, it’s unnecessary to synchronize access since localVariable
is not shared between threads.
Using Immutability
Immutable objects are naturally thread-safe. By using these, you can often avoid complex synchronization problems, resulting in cleaner and more readable code.
Example
public class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
// Getters for x and y
public int getX() {
return x;
}
public int getY() {
return y;
}
}
By utilizing immutable objects, you mitigate the need for synchronization altogether, leading to safer and more efficient multithreading.
Misconception 4: All Exceptions Can Be Handled in Threads
Reality: Handling exceptions in threads can be tricky. Uncaught exceptions thrown by a thread can lead to the termination of the thread and may not always be caught in the main method or the parent thread.
Example of Exception Handling in Threads
public class ExceptionHandlingRunnable implements Runnable {
@Override
public void run() {
try {
// Simulates an exception
throw new RuntimeException("Thread exception");
} catch (RuntimeException e) {
System.out.println("Caught an exception in Thread: " + Thread.currentThread().getName());
}
}
}
While the above code handles exceptions locally, any uncaught exception would terminate the thread. To ensure robustness, enable global exception handling through a custom Thread.UncaughtExceptionHandler
.
Setting a Custom Handler
Thread thread = new Thread(new ExceptionHandlingRunnable());
thread.setUncaughtExceptionHandler((t, e) -> {
System.out.println("Uncaught exception in thread " + t.getName() + ": " + e.getMessage());
});
thread.start();
This way, you can handle exceptions more gracefully in multithreaded applications.
Misconception 5: Java's Thread Pool is Just for Reusing Threads
Reality: While reusing threads is a significant benefit, Java’s thread pool (e.g., ExecutorService
) provides a richer feature set that enhances task scheduling and management. It helps in prioritizing tasks, limiting the number of concurrent threads, and handling failed tasks.
Using ExecutorService
ExecutorService executorService = Executors.newFixedThreadPool(3);
for (int i = 0; i < 10; i++) {
executorService.submit(new Task());
}
executorService.shutdown();
In this example, Executors.newFixedThreadPool(3)
creates a pool of three threads. Even if you submit ten tasks, only three will run concurrently, as determined by the pool size. This avoids overwhelming system resources and leads to more stable performance.
For more details on thread pools and their configurations, visit the Java Documentation on ExecutorService.
Closing the Chapter
Understanding multithreading is vital not just for interviews but also for building efficient Java applications. Clearing up misconceptions can significantly improve both your coding skills and your interview responses.
When preparing for multithreaded environments, focus on safe practices, performance opportunities, and the correct use of synchronization. Use Java's built-in capabilities wisely to handle threads, avoid assumptions that could lead to inefficiencies, and always prioritize clarity of implementation.
With this knowledge, you will not only improve your chances in interviews but also create robust, efficient, and maintainable Java applications. Dive deeper into the intricacies of multithreading, and watch your programming skills soar!
For further reading, review materials on Java Concurrency from Java Concurrency in Practice and delve into more advanced topics. Happy coding!