Understanding Garbage Collection: Common Myths Debunked
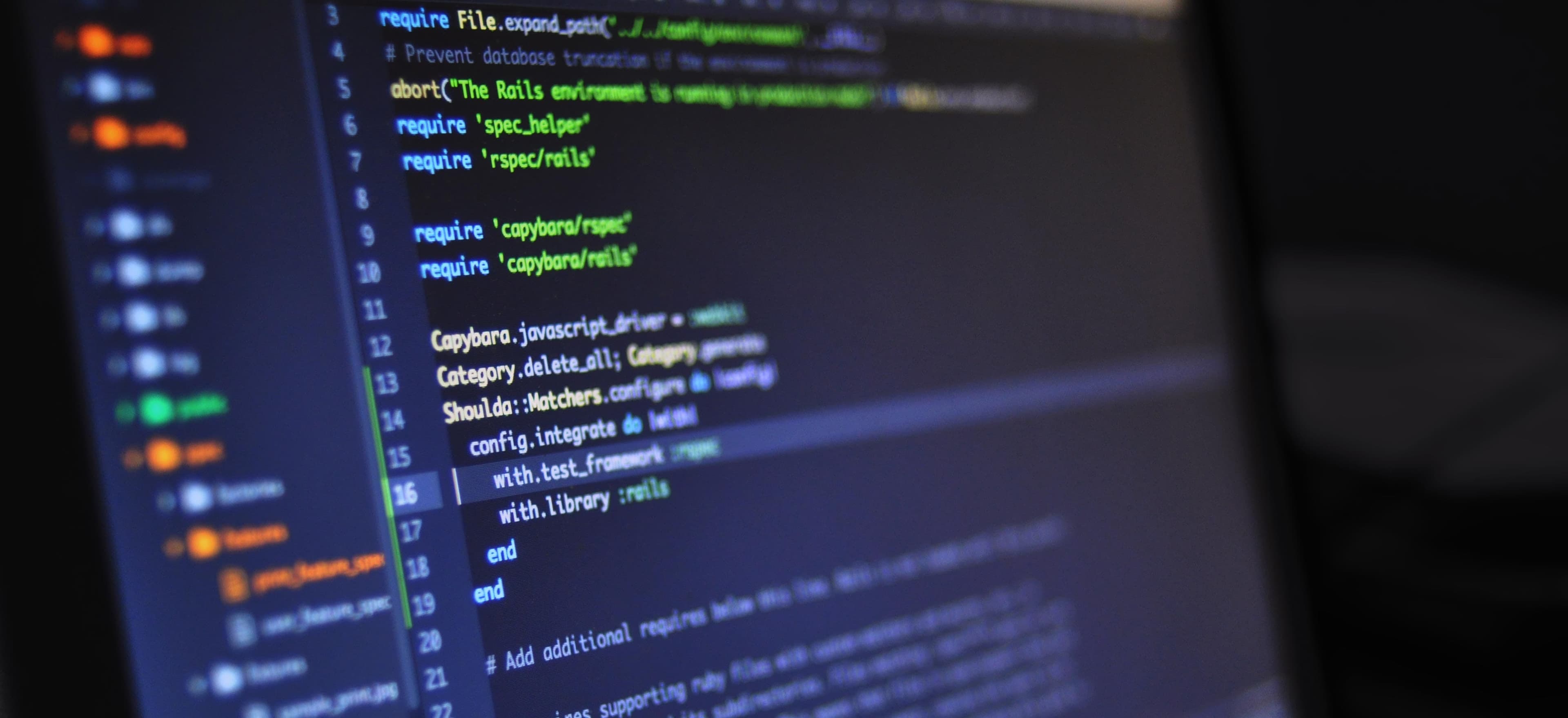
- Published on
Understanding Garbage Collection: Common Myths Debunked
Garbage Collection (GC) is a fundamental part of memory management in Java, playing a pivotal role in ensuring efficient use of memory and improving application performance. Unfortunately, many myths and misconceptions surround it, leading to misunderstandings about how it works, why it's necessary, and how it impacts Java applications. This blog post aims to debunk some of the most prevalent myths about Garbage Collection in Java.
What is Garbage Collection?
Garbage Collection refers to the automated process of deallocating memory that is no longer in use. It identifies and disposes of objects that are no longer reachable from any reference within the application, thus cleaning up memory for reuse. Understanding GC is crucial for performance optimization in Java applications.
Myth 1: Garbage Collection in Java is Slow
The first myth we will address is that Garbage Collection is inherently slow. While it is true that GC can introduce pauses in application execution, advancements in Garbage Collection algorithms have made them remarkably efficient.
Efficient Multi-Generational Garbage Collection
Java employs a multi-generational approach to Garbage Collection. The heap is divided into different generations (Young, Old, and Permanent). Most objects are short-lived, and young objects undergo frequent Garbage Collection, which is optimized for performance.
public class GCDemo {
public static void main(String[] args) {
// Creating a large number of temporary objects
for (int i = 0; i < 100000; i++) {
String tempStr = new String("Temporary String " + i);
}
// Suggest garbage collection (not guaranteed)
System.gc();
System.out.println("Garbage Collection requested.");
}
}
Why This Matters: By collecting young objects quickly, GC minimizes the time taken to reclaim memory. Hence, while it might induce pauses, modern Garbage Collection in Java is optimized to keep these to a minimum.
Myth 2: You Can't Control Garbage Collection
Another prevalent myth is that Garbage Collection in Java is entirely out of a developer's control. While it is managed by the JVM, developers do have some options to influence it.
GC Tuning Options
Java provides various command-line options to tune Garbage Collection. For example, you can specify the size of memory pools or select different GC algorithms.
Here’s how you might set options for the JVM:
java -Xms512m -Xmx4g -XX:+UseG1GC -jar YourApp.jar
-Xms
sets the initial heap size.-Xmx
sets the maximum heap size.-XX:+UseG1GC
specifies the use of the G1 Garbage Collector.
Why This Matters: Understanding these parameters helps developers configure the application memory settings to better fit their needs. This can lead to significantly improved performance in terms of memory management.
Myth 3: Finalizers are a Reliable Way to Manage Resources
Finalizers in Java were once considered a way to ensure that important cleanup code runs when an object becomes unreachable. However, relying on them has been proven to be a bad practice.
Performance Overhead and Unpredictability
The invocation of finalizers is unpredictable. The JVM decides when (or if) to run them. This unpredictability can lead to resource leaks and can introduce performance overhead.
public class Resource {
// Resource allocation
public Resource() {
// Allocate resources
}
// Finalizer (not recommended)
protected void finalize() throws Throwable {
try {
// Cleanup resource
} finally {
super.finalize();
}
}
}
Why This Matters: Relying on finalizers can lead to undefined behavior. Instead, developers should use try-with-resources statements or explicit resource management to ensure that resources are released promptly.
Myth 4: Long-Lived Objects Are Collected Immediately
There's a misconception that long-lived objects are collected quickly. In reality, the lifecycle of objects in Java can be more complex.
Promotion to Old Generation
Long-lived objects undergo a process known as "promotion." When an object survives several rounds of GC in the Young generation, it is moved (or promoted) to the Old generation. This means that they are collected less frequently.
public class LongLivedObject {
static LongLivedObject obj = new LongLivedObject();
public static void main(String[] args) {
// obj will be promoted since it is static and long-lived
System.out.println("Long-lived object created.");
System.gc();
}
}
Why This Matters: Understanding the promotion process can help developers design their data models with memory management in mind, preventing unnecessary memory bloat.
Myth 5: Garbage Collection is a Panacea for Memory Leaks
A prevalent belief is that using Garbage Collection nullifies the risk of memory leaks. However, this is misleading.
Understanding Memory Leaks
In Java, memory leaks can still occur. When objects are still referenced in some way, they will not be collected, leading to memory bloat. Common culprits include static collections that grow indefinitely or listeners that are not properly unregistered.
import java.util.*;
public class MemoryLeakDemo {
private static List<Object> list = new ArrayList<>();
public static void addObject() {
list.add(new Object());
}
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
addObject(); // Adds objects to the static list
}
System.gc(); // Request garbage collection
System.out.println("Requested garbage collection.");
}
}
Why This Matters: Being aware of memory leaks is crucial, as relying solely on GC can lead to performance degradation and application failure. Proper memory management practices must still be employed.
The Bottom Line
Understanding Garbage Collection is essential for Java developers. The myths surrounding GC can lead to poor coding practices and suboptimal applications.
- Remember that GC is not inherently slow; rather, it has become an efficient mechanism.
- You can control certain aspects of GC through JVM parameters.
- Finalizers should not be relied upon for resource management.
- Long-lived objects are not collected immediately and are promoted to longer-lived areas.
- Garbage Collection alone does not eliminate memory leaks.
By debunking these myths, developers can harness the full power of Java's Garbage Collection, leading to better performance and cleaner applications. To deepen your understanding of Garbage Collection, you can explore resources such as the Java Tutorials or Oracle's Garbage Collection Tuning Guide.
Understanding these concepts will not only improve your code but also help maintain high performance, making you a more proficient Java developer.