Uncovering Hidden Layers in Your Docker Images
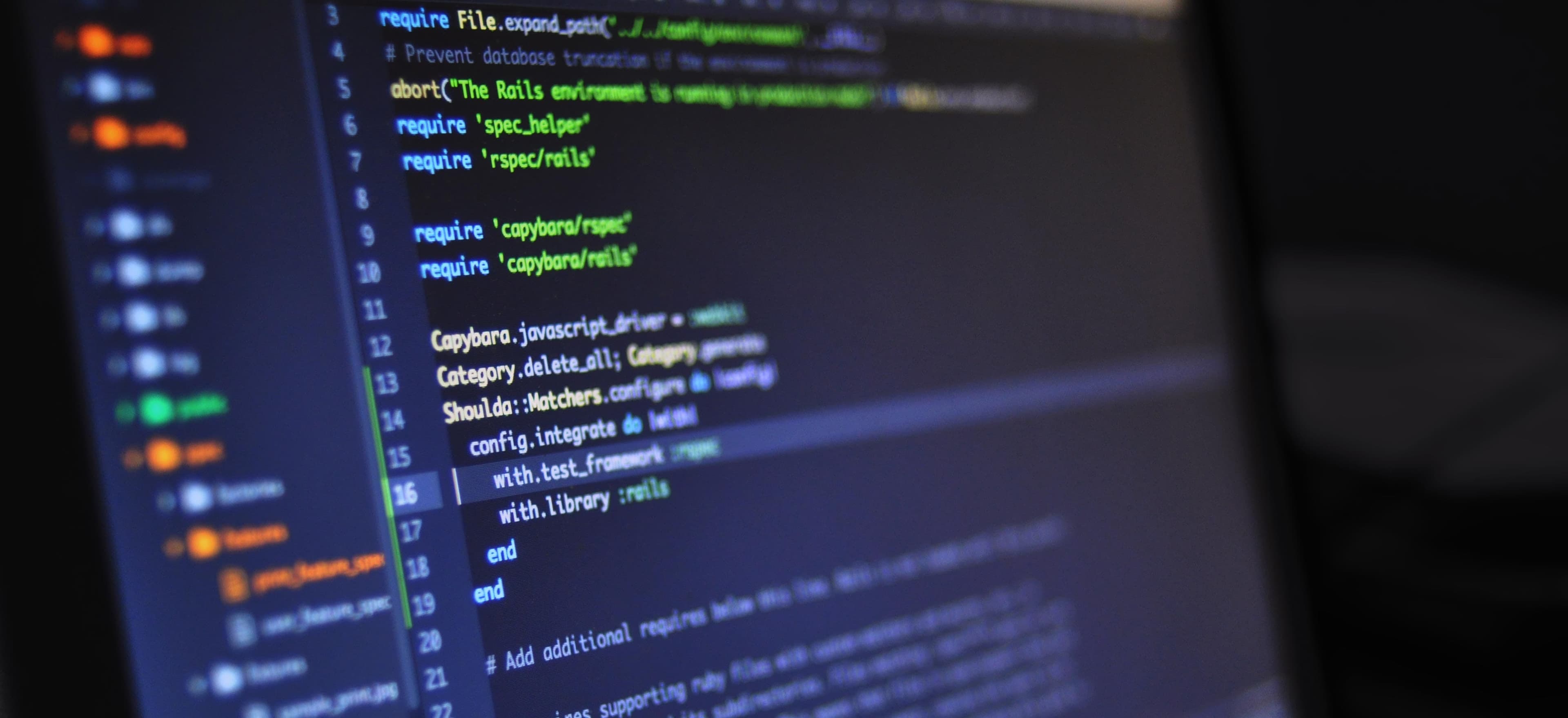
- Published on
Uncovering Hidden Layers in Your Docker Images
Docker has revolutionized the way developers build, share, and deploy applications. It allows you to create isolated environments called containers, which bundle your application with everything it needs to run. However, one aspect that many developers overlook is the architecture of Docker images themselves. Each Docker image consists of multiple layers, and understanding these layers is crucial for optimizing image size, improving performance, and ensuring security.
In this blog post, we will explore the concept of Docker image layers, why they matter, and how to assess and optimize them. By the end, you will have practical tools and techniques to streamline your Docker images effectively.
What Are Docker Image Layers?
A Docker image layer is a set of files that were created during the image build process. Each instruction in a Dockerfile (such as RUN
, COPY
, and ADD
) creates a new layer in the image. Docker layers are stacked on top of each other, forming a unified, read-only image. When you run a container from this image, a writable container layer is added on top.
Why Docker Layers Matter
-
Caching: When making changes to your Dockerfile, Docker optimizes the build process by reusing unchanged layers. This dramatically speeds up build times.
-
Performance: Smaller images lead to faster downloads and reduced deployment times. Optimizing layers can improve overall performance.
-
Security: Each layer carries its own security implications. For example, vulnerable software in a layer can affect the entire image.
Understanding these layers helps you build more efficient, secure, and manageable Docker images.
Analyzing Docker Image Layers
To gain insight into the layers of your Docker images, you can use the docker history
command. This command displays a breakdown of the layers in an image, along with their sizes and command history. Here's an example:
docker history <image_name>
Example Output
When you run this command on a sample image, the output may look something like this:
IMAGE CREATED CREATED BY SIZE COMMENT
abc123def456 2 days ago /bin/sh -c apt-get update && apt-get install... 150MB
ghi789jkl012 2 days ago /bin/sh -c build_deps.sh 100MB
mno345pqr678 2 days ago /bin/sh -c npm install 50MB
Each entry corresponds to a step in the Dockerfile that created a new layer.
Analyzing Layer Size
When looking at the output, pay attention to the SIZE
column. A high size may indicate that the layer contains unnecessary files or actions that could be optimized. For instance, if a single RUN command installs numerous packages, consider breaking them down into smaller, more focused RUN commands.
Example of Optimization
Initial Dockerfile Snippet:
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y package1 package2 package3
Optimized Dockerfile Snippet:
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y package1
RUN apt-get install -y package2
RUN apt-get install -y package3
In this example, the size of individual layers is reduced because the install commands are broken up. However, this may not always be the best approach; there is a balance between readability and size which must be navigated based on specific needs.
Best Practices for Layer Optimization
-
Minimize the Number of Layers: Combine commands when possible. Grouping multiple RUN commands into a single command can minimize layer count.
-
Use .dockerignore: Similar to
.gitignore
, this file allows you to exclude files from the build context. Minimizing context size helps reduce layer sizes. -
Structure Your Dockerfile Logically: Place frequently changed commands at the bottom of the Dockerfile. This will help cache unchanged layers effectively.
-
Remove Unnecessary Files: Ensure that temporary files created during the build process are deleted in the same RUN command. This ensures they don't persist to the resulting image.
Example of Cleaning Up
Here is an example of how to remove temporary files created during the build process:
FROM node:14
WORKDIR /app
COPY package.json ./
RUN npm install && npm cache clean --force
COPY . .
RUN rm -rf /var/cache/apk/*
In this case, we install our dependencies and clean up the npm cache in a single RUN command to ensure that temporary files do not persist to our image.
Tools for Analyzing Docker Layers
Several tools help visualize and analyze Docker image layers. Here are a couple of noteworthy ones:
-
Dive: Dive is a tool for exploring the content of Docker images and analyzing layer sizes. It provides a detailed view of what each layer contains and helps you identify potential savings.
To install Dive, you can use Homebrew or download binaries from GitHub.
brew install dive
Run it using:
dive <image_name>
-
Docker Slim: Docker Slim is another tool that automatically analyzes your Docker images and optimizes them by removing unnecessary files and layers.
To start using Docker Slim, you can follow the instructions in their GitHub repository.
Security Considerations
Security in Docker images is paramount. Each layer has the potential to introduce vulnerabilities. Regularly scan your images for known vulnerabilities using tools such as Docker Bench Security. The earlier you identify potential weaknesses, the better your chances of augmenting your security practices.
docker run --privileged --pid=host --net=host \
--cap-add=ALL --rm --volume /usr/bin/docker:/usr/bin/docker:ro \
--volume /var/lib/docker:/var/lib/docker:ro \
--volume /etc:/etc:ro \
--volume /var/run/docker.sock:/var/run/docker.sock \
docker/bench-security
This command runs the Docker Bench Security tool, helping you identify and address vulnerabilities in your Docker setups.
To Wrap Things Up
By uncovering hidden layers in your Docker images, you can achieve a wide range of benefits, from better performance to enhanced security. Understanding and optimizing these layers can significantly impact your development workflows. Dive deeper into your images, analyze them with tools like Dive or Docker Slim, and put best practices into play to create efficient, secure Docker images.
For more comprehensive documentation on Docker, visit Docker's official documentation. Using these insights will help you create optimized Docker images that not only perform well but also adhere to best security practices. Happy coding!