Avoiding the Most Common Java Code Violations
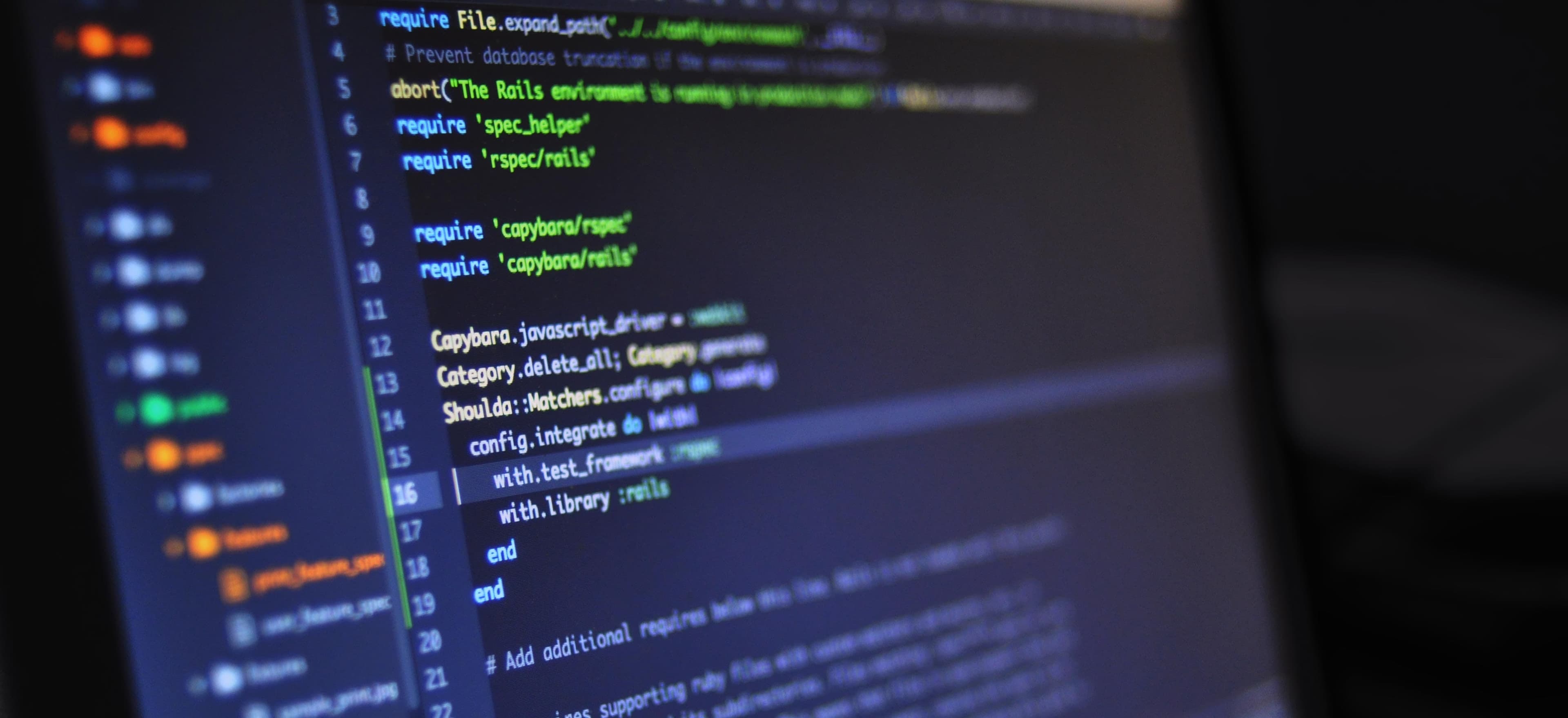
- Published on
Avoiding the Most Common Java Code Violations
Java is a versatile programming language that's widely used for building enterprise applications, Android apps, and various other software systems. Despite its robust features and design principles, developers can easily fall into traps that lead to common code violations. This post seeks to highlight some of these violations and provide best practices to avoid them.
Why Code Quality Matters
Before diving in, it's essential to understand why adhering to coding standards and best practices is crucial. High-quality code is not just about making it work; it's about maintainability, readability, and efficiency. A codebase that’s clean and well-structured is easier to debug, modify, and extend. Moreover, it enhances collaboration among team members who may interact with your code.
Common Java Code Violations
Here’s a breakdown of the most prevalent Java code violations and how to avoid them:
1. Inefficient Exception Handling
Violation: Overusing exceptions for flow control can lead to performance issues. Exceptions are expensive operations in Java, and using them improperly can degrade application performance.
Solution: Use exceptions for exceptional situations only, not for regular control flow. Implement proper error handling strategies.
Example:
// Incorrect use of exceptions for control flow
public void processOrders(List<Order> orders) {
for (Order order : orders) {
try {
existsInDatabase(order);
} catch (OrderNotFoundException e) {
// Handle exception
}
}
}
In this example, the code checks the existence of orders in a database using exceptions, which is inefficient. Instead, consider using a return value to handle this situation gracefully.
// Correct approach using return values
public void processOrders(List<Order> orders) {
for (Order order : orders) {
if (!existsInDatabase(order)) {
// Handle the case when the order doesn't exist
}
}
}
private boolean existsInDatabase(Order order) {
// Check database and return true/false
}
2. Hardcoding Values
Violation: Hardcoding values can make your code less adaptable and challenging to maintain. It limits flexibility because any change requires altering the code.
Solution: Use constants or configuration files to manage values that might change.
Example:
// Hardcoding values
public static final double TAX_RATE = 0.2;
public static final double DISCOUNT = 0.1;
Instead, define these values in a properties file or use constants as shown below:
// Using constants effectively
public class FinancialConfig {
public static final double TAX_RATE = getTaxRateFromConfig();
public static final double DISCOUNT = getDiscountFromConfig();
private static double getTaxRateFromConfig() {
// Logic to get value from configuration
}
private static double getDiscountFromConfig() {
// Logic to get value from configuration
}
}
3. Not Using Generics
Violation: Failing to use generics can lead to ClassCastException
at runtime and undermines type safety.
Solution: Always leverage generics when working with collections to ensure that the compiler can catch type mismatches.
Example:
// Without generics - risky
List orders = new ArrayList(); // Raw type
orders.add("Order1"); // Adding a String
Order myOrder = (Order) orders.get(0); // Will throw ClassCastException
With generics, you can achieve type safety:
// With generics - type-safe
List<Order> orders = new ArrayList<>();
orders.add(new Order());
Order myOrder = orders.get(0); // Safe, no casting required
4. Overcomplicated Code
Violation: Writing overly complex code can lead to a loss of clarity. This can slow down development, increase bugs, and make maintenance challenging.
Solution: Keep your methods focused on a single task (Single Responsibility Principle). Break down complex logic into smaller methods.
Example:
// Overly complex method
public void handleRequest(Request req) {
if (req.isGetRequest()) {
// Processing GET
} else if (req.isPostRequest()) {
// Processing POST
} else {
// Handle other cases
}
}
Instead, simplify the code:
// Simpler methods
public void handleRequest(Request req) {
switch (req.getMethod()) {
case "GET":
processGet(req);
break;
case "POST":
processPost(req);
break;
default:
handleOther(req);
break;
}
}
private void processGet(Request req) {
// Handle GET request
}
private void processPost(Request req) {
// Handle POST request
}
private void handleOther(Request req) {
// Handle other types of requests
}
5. Ignoring Code Readability
Violation: Writing unreadable code hurts the maintainability of software. Unclear naming conventions, poor formatting, and lack of comments can create barriers for understanding.
Solution: Maintain clear naming conventions, consistent formatting, and meaningful comments. Follow the Java Code Conventions for guidance.
Example:
// Hard to read and understand
public void f(int a, int b) {
// Some processing
}
Should be rewritten for clarity:
// Improved readability
public void calculateSum(int firstNumber, int secondNumber) {
// Calculate the sum of two numbers
}
Wrapping Up
Improving your Java code involves being vigilant about common violations, adopting best practices, and continuously refining your coding skills. Strive to maintain clear, efficient, and error-free code to enhance collaboration, ensure easier maintenance, and deliver a solid product.
To further deepen your understanding, consider exploring these additional resources:
By mastering these aspects, you'll not only improve your coding ability but also contribute to the quality of the software engineering community at large. Happy coding!
Checkout our other articles