Solving Log Management Chaos in Microservices
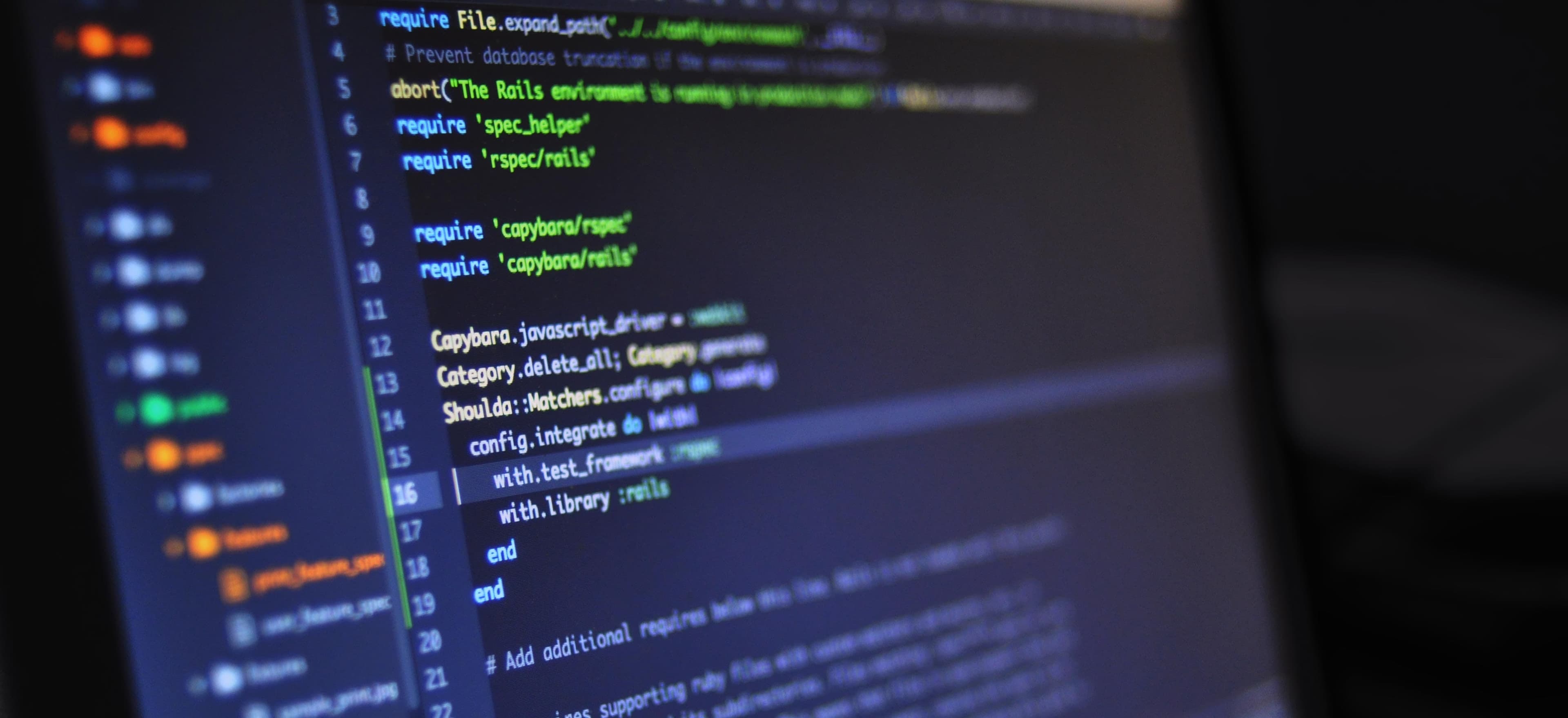
- Published on
Solving Log Management Chaos in Microservices
Microservices architecture has revolutionized the way we build applications, enabling flexibility, scalability, and rapid development. However, this innovation comes with its fair share of challenges, particularly in handling logs effectively. In this post, we will explore how to tackle log management chaos in microservices, providing insights, strategies, and code snippets.
Understanding the Complexity of Microservices Logging
In a microservices environment, an application is broken down into smaller, independent services. Each service can have its own logging mechanism, structure, and storage solutions, leading to a multitude of logs being generated. The chaos arises when:
- Services produce different log formats.
- Logs are scattered across various locations.
- Centralized logging becomes cumbersome.
These challenges can significantly impede troubleshooting and monitoring, making it critical to have a robust logging strategy in place.
Why Logging Matters
Logging serves as the foundation for monitoring, debugging, and analyzing application performance. Effectively managing logs helps in the following ways:
- Issue Identification: Rapidly locate and diagnose issues.
- Performance Optimization: Analyze logs to identify performance bottlenecks.
- Audit Trails: Keep track of changes and access within the application.
Key Considerations for Microservices Logging
When strategizing your logging approach, consider the following:
- Log Structure: Use a structured logging format like JSON to facilitate easier parsing and analysis.
- Log Levels: Implement different log levels (INFO, DEBUG, ERROR, etc.) to control the verbosity of logs.
- Centralized Logging: Utilize centralized logging tools to aggregate logs from all microservices.
Recommended Strategies for Log Management
1. Centralized Logging with ELK Stack
One effective solution for managing logs in a microservices architecture is the ELK Stack (Elasticsearch, Logstash, Kibana). This setup allows you to centralize logs from different services.
Why Use ELK Stack?
- Searchable Logs: Elasticsearch helps in indexing and searching logs quickly.
- Data Pipelines: Logstash supports a wide variety of inputs, including service logs.
- Visualization: Kibana provides an interface for visualizing logs.
Code Snippet: Sample Logstash Configuration
input {
file {
path => "/var/log/my_microservice/*.log"
start_position => "beginning"
}
}
filter {
json {
source => "message"
}
}
output {
elasticsearch {
hosts => ["http://localhost:9200"]
index => "microservice-logs-%{+YYYY.MM.dd}"
}
}
Commentary: In this configuration, Logstash watches for log files generated by your microservices. When it detects a new log file, it processes the log entries as JSON and sends them to Elasticsearch for indexing.
2. Structured Logging
Adopting structured logging means you log events in a serialized format, typically JSON. This structure facilitates various tools to easily parse logs and extract meaningful data.
Code Snippet: Structured Logging in Java with SLF4J
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static com.fasterxml.jackson.databind.ObjectMapper;
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
private static final ObjectMapper objectMapper = new ObjectMapper();
public void processOrder(Order order) {
try {
logger.info("Processing order: {}", objectMapper.writeValueAsString(order));
// Processing logic...
logger.info("Order processed successfully");
} catch (Exception e) {
logger.error("Error processing order: {}", e.getMessage());
}
}
}
class Order {
private String id;
private String customer;
// Getters and Setters
}
Commentary: In this code snippet, we log the order data in a structured format using SLF4J and Jackson for JSON serialization. This practice ensures that all the needed information about the order is captured in a readable format that can be easily parsed later.
3. Centralized Logging Services
Beyond ELK, consider using managed logging services such as Loggly or Splunk. These platforms provide out-of-the-box solutions for collecting, analyzing, and retrieving logs.
4. Implementing Correlation IDs
In a distributed architecture, tracing requests across multiple services can be a challenge. Implementing correlation IDs allows you to track the lifecycle of a request throughout your application.
Code Snippet: Adding Correlation IDs
import org.slf4j.MDC;
public void handleRequest() {
String correlationId = UUID.randomUUID().toString();
MDC.put("correlationId", correlationId);
logger.info("Received request");
try {
// Process request and call other services...
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage());
} finally {
MDC.clear();
}
}
Commentary: Using the Mapped Diagnostic Context (MDC), we associate a unique correlation ID with each log entry. This allows for easier tracking and correlation of logs across services.
5. Setting Appropriate Log Levels
Managing the granularity of logging is vital. Set levels appropriately to avoid log flooding. Choose DEBUG during development and INFO or WARN in production.
6. Rotate and Archive Logs
To prevent disk space issues, implement log rotation and archiving strategies. Tools like logrotate
can help manage log file sizes and delete old log files.
To Wrap Things Up
Navigating the complexities of log management in microservices can be daunting, but with the right strategies in place, you can transform chaos into clarity. By leveraging centralized logging tools, adopting structured logging practices, implementing correlation IDs, and carefully managing log levels, you can create a robust logging framework that enhances your application's observability.
For further reading, consider exploring The Twelve-Factor App: Logging as it outlines best practices for logging in cloud-native applications. Additionally, check out the official documentation of ELK Stack for deeper insights into setting up an effective logging infrastructure.
Remember, effective log management not only aids in troubleshooting but also provides invaluable insights for improvement and growth. Happy logging!
Checkout our other articles