When Maven Dependency Plugin Gives Incorrect Results
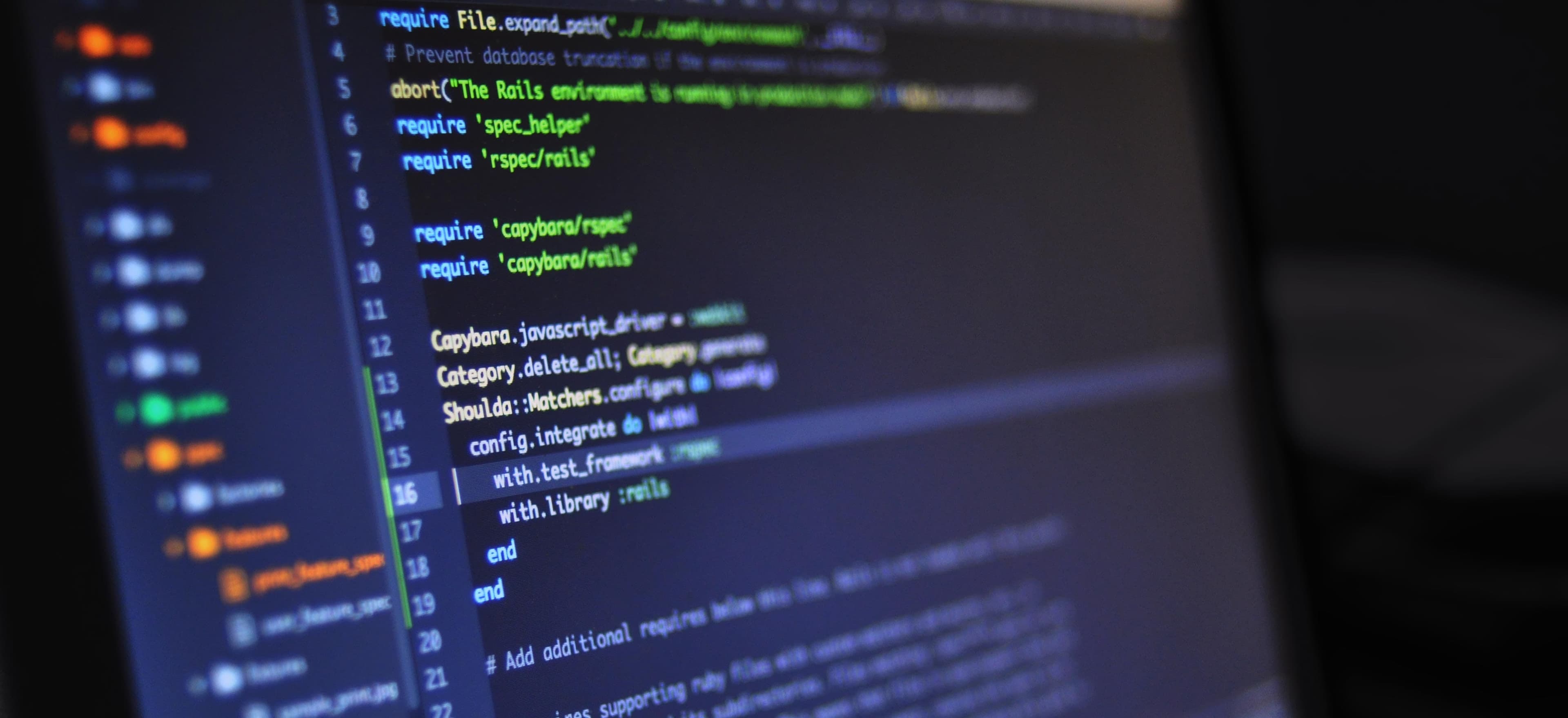
- Published on
When Maven Dependency Plugin Gives Incorrect Results
Maven is an essential tools in the Java ecosystem, widely utilized for build automation and dependency management. Among various Maven plugins, the Maven Dependency Plugin is crucial for analyzing and managing project dependencies. However, it can sometimes yield incorrect results, confusing developers. This post delves into common scenarios where the Maven Dependency Plugin may falter and how to troubleshoot and resolve these issues.
Table of Contents
- Understanding Maven Dependency Plugin
- Common Issues and Challenges
- How to Troubleshoot Dependency Issues
- Best Practices to Avoid Dependency Problems
- Conclusion
Understanding Maven Dependency Plugin
The Maven Dependency Plugin allows developers to analyze and manage project dependencies effectively. It offers commands for:
- Analyzing dependencies
- Copying dependencies
- Resolving dependency trees
- Displaying dependency information
Understanding how this plugin works is key to effectively handling dependencies in a Maven project. Use the following command to list all dependencies in your project:
mvn dependency:list
This command will generate a list of project dependencies, but be cautious. The accuracy of the data depends heavily on the configuration of your project’s pom.xml
file.
Common Issues and Challenges
1. Conflicting Dependencies
Dependency conflicts occur when two or more libraries depend on different versions of the same library. This can lead to runtime errors if the JVM chooses a version incompatible with your application.
To illustrate, consider the following dependencies:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.9.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
In this case, the spring-core
version is outdated compared to the spring-web
version, which may lead to class definition errors at runtime.
Solution: Enforce dependency management to provide a consistent version throughout your application:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
</dependencies>
</dependencyManagement>
2. Scope Issues
Maven supports different scopes for dependencies, such as compile
, provided
, runtime
, and test
. Misunderstanding these scopes can lead to unexpected results.
For example, if a dependency is marked as test
, it will not be included in your application's final build. Consider the following:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.9.0</version>
<scope>test</scope>
</dependency>
If you attempt to use Mockito
in your main application code, you will encounter a ClassNotFoundException
since it's not included in the production build.
Solution: Always verify the scope of your dependencies and ensure they align with your intended use.
3. Transitive Dependencies
When a dependency depends on other libraries, its dependencies are called transitive dependencies. Issues arise when these transitive dependencies are not compatible or not present.
Take the following example:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
The commons-lang3
library may depend on other libraries, and if those are not resolved correctly, you may face issues.
Solution: Use the dependency:tree
command to visualize dependencies and identify any missing or conflicting transitive dependencies:
mvn dependency:tree
4. Plugin Version Mismatches
Another common issue arises when there's a mismatch between the plugin version and the dependencies it manages. For example, if you're using an outdated version of the Dependency Plugin, it may not effectively resolve newer dependencies.
To ensure you are using the latest version, check your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>3.1.2</version>
</plugin>
</plugins>
</build>
Solution: Always keep your Maven plugins updated to minimize incompatibilities. You can refer to the official Maven Dependency Plugin documentation for version details and updates.
How to Troubleshoot Dependency Issues
-
Effective POM: Run
mvn help:effective-pom
to see how your POM looks after Maven applies default settings and manages dependencies. -
Dependency Tree: Use
mvn dependency:tree
to identify dependency conflicts and transitive dependencies. -
Dependency Plugin Report: Generate a report with
mvn dependency:analyze
to check for unused or undeclared dependencies.
Best Practices to Avoid Dependency Problems
- Use Dependency Management: Use
<dependencyManagement>
in your parent POM to manage versions consistently across modules. - Version Ranges: Utilize version ranges carefully to give flexibility to dependency versions.
- Regular Updates: Regularly update dependencies and plugins to the latest stable versions.
- CI/CD Integration: Integrate dependency checks into your CI/CD pipeline to catch issues early.
- Use Tools: Consider tools like Maven Enforcer Plugin or Dependency-Check for enhanced dependency management.
Key Takeaways
The Maven Dependency Plugin is a powerful tool, but it is not without quirks. From conflicting dependencies to scope issues, developers can often encounter roadblocks that hinder smooth development. Understanding common pitfalls and following best practices can aid in efficiently managing your Maven dependencies.
By keeping your project configuration clean, utilizing dependency management features, and actively maintaining your dependencies, you can avoid many of the issues that arise.
For further reading, you might find Maven: The Complete Reference very useful. If you need to explore more advanced concepts, consider delving into the Maven Central Repository documentation that provides insight into maintaining libraries.
Remember, a well-maintained POM file can save significant time and trouble, allowing you to focus on what you do best: writing great Java code.
Checkout our other articles