Enhance SQL Logging in Spring Hibernate with Log4jdbc
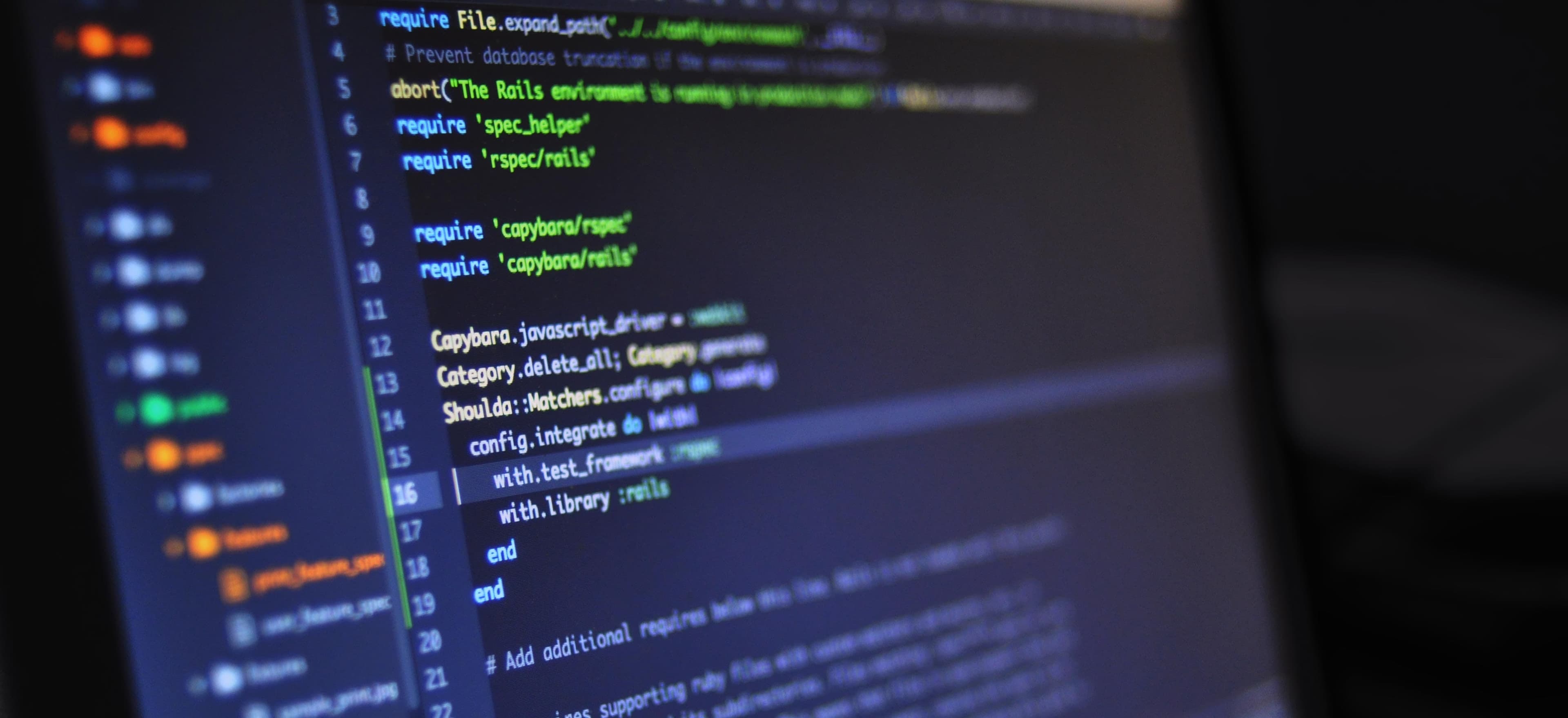
- Published on
Enhance SQL Logging in Spring Hibernate with Log4jdbc
When working with databases in a Spring application, especially one that uses Hibernate as its ORM (Object-Relational Mapping) framework, efficient logging of SQL statements is essential. Proper logging gives insight into the data access layer's behavior, assists in troubleshooting, and enhances the overall development process. One effective way to achieve this is by integrating Log4jdbc, which provides an easy way to intercept JDBC calls, log SQL statements, and visualize query performance.
In this blog post, we will explore how to enhance SQL logging in a Spring application powered by Hibernate using Log4jdbc. We will cover:
- Overview of Log4jdbc
- Setting Up Log4jdbc in Your Spring Application
- Configuring Logging
- Example Implementation
- Conclusion and Best Practices
Overview of Log4jdbc
Log4jdbc is a proxy driver that surrounds the actual JDBC driver, capturing SQL statements and their execution times. This capability allows for comprehensive logging of SQL queries, parameters, and the execution duration without altering your existing code significantly.
Key Features of Log4jdbc:
- SQL Logging: Captures all SQL statements executed against the database.
- Parameter Logging: Logs the parameters passed to queries, ensuring full visibility into data actions.
- Execution Time Logging: Helps in identifying slow queries by logging execution duration.
For more on Log4jdbc, you can check the official documentation here.
Setting Up Log4jdbc in Your Spring Application
Integrating Log4jdbc into your Spring application involves a few straightforward steps. Below, we will detail this process.
Step 1: Add Dependencies
First, you need to add Log4jdbc and the actual JDBC driver you are using (like PostgreSQL, MySQL, etc.) to your pom.xml
if you are using Maven:
<dependency>
<groupId>log4jdbc</groupId>
<artifactId>log4jdbc-spring-boot-starter</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.19</version>
</dependency>
Note: Make sure to use the latest version of both libraries. You can check for updates on Maven Repository.
Step 2: Configure Data Source
Next, you need to configure your data source in your application.properties
or application.yml
. You'll substitute your actual JDBC URL and credentials. Below is an example configuration for a PostgreSQL database in application.properties
:
spring.datasource.url=jdbc:log4jdbc:postgresql://localhost:5432/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
This configuration tells your application to use Log4jdbc as a proxy for the PostgreSQL driver.
Step 3: Enable Logging
In order to see the logs, you will need to configure your logging framework adequately. If you are using logback
, you can configure it in src/main/resources/logback-spring.xml
:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%date %level [%thread] %logger{5} - %msg%n</pattern>
</encoder>
</appender>
<logger name="jdbc.sqlonly" level="DEBUG"/>
<logger name="jdbc.sql" level="DEBUG"/>
<logger name="jdbc.batch" level="DEBUG"/>
<root level="INFO">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
This configuration will enable detailed logging of the SQL statements processed by Log4jdbc.
Example Implementation
Let’s look at an example implementation within a Spring Boot application. Below, we will demonstrate a simple CRUD operation using Hibernate, while showcasing Log4jdbc.
Entity Class
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Repository Interface
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface CustomerRepository extends JpaRepository<Customer, Long> {
}
Service Class
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
public List<Customer> getAllCustomers() {
return customerRepository.findAll();
}
public Customer createCustomer(Customer customer) {
return customerRepository.save(customer);
}
}
Controller
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/customers")
public class CustomerController {
@Autowired
private CustomerService customerService;
@GetMapping
public List<Customer> getCustomers() {
return customerService.getAllCustomers();
}
@PostMapping
public Customer addCustomer(@RequestBody Customer customer) {
return customerService.createCustomer(customer);
}
}
With the above components in place, you can run the application, and you will see log statements related to SQL execution in your console. For instance, when you create a new customer and the application runs the SQL insert statement, you should see debug logs showing the exact SQL generated, the parameters, and the execution time.
Closing Remarks and Best Practices
Enhancing SQL logging with Log4jdbc in a Spring Hibernate application is straightforward and very beneficial. Detailed SQL logs help developers troubleshoot issues faster and optimize queries effectively. Logging execution times can expose performance bottlenecks, making it easier to fine-tune your database interactions.
Best Practices:
- Use Different Log Levels: While DEBUG is great for development, consider changing the log level to INFO or WARN in production environments to avoid performance overhead.
- Filtering Log Output: You can enable filtering based on the SQL types (insert, update, delete, etc.) to focus on what matters most during debugging.
- Review Performance Logs Regularly: Keep an eye on slow queries to ensure optimal performance of your application.
By thoughtfully integrating Log4jdbc into your Spring application, you empower your debugging and performance profiling efforts significantly. For further details, you can always refer to the Log4jdbc documentation.
Happy coding!
Checkout our other articles