Top 5 Java Resources Every Developer Should Know About
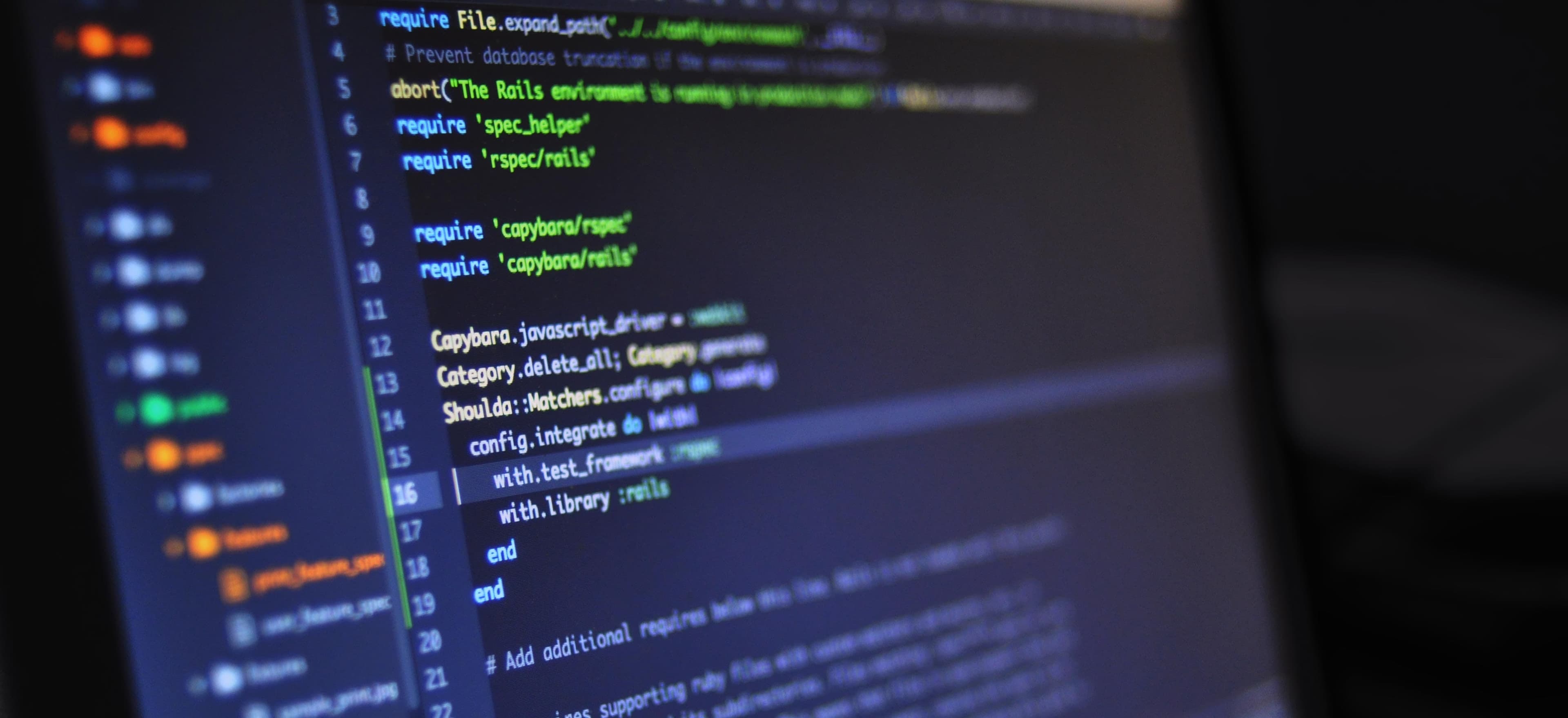
- Published on
Top 5 Java Resources Every Developer Should Know About
Java is one of the most widely used programming languages in the world. Whether you're a seasoned programmer or just getting started, having the right resources at your disposal can significantly accelerate your development skills and project success. In this blog post, we'll explore the top five resources every Java developer should know about and leverage to excel in their careers.
1. Oracle's Official Java Documentation
Oracle's Java Documentation is a staple for any Java developer. It offers comprehensive and detailed insights into all aspects of the Java programming language.
Why It’s Essential:
- Comprehensive Reference: The documentation covers everything from the Java API to language specifications and best practices.
- Always Up-to-Date: Oracle maintains this documentation, ensuring it reflects the latest updates and features in Java.
- Code Examples: Many sections provide clear code snippets, which help to understand complex concepts better.
Code Snippet Example:
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("C++");
System.out.println("Programming Languages: " + list);
}
}
In this example, we create an ArrayList
to store programming languages. The simplicity of creating collections in Java encourages developers to manage data efficiently.
2. Stack Overflow
Stack Overflow is the go-to forum for developers to ask questions, share knowledge, and find solutions to coding challenges.
Why It’s Essential:
- Community Support: Developers can get answer from experienced peers and professionals, making it easier to debug issues.
- A Vast Repository of Knowledge: With countless topics already covered, there’s a high chance someone has addressed your specific issue.
- Real-world Scenarios: The answers often include code examples and practical implementations, showcasing how concepts apply in real projects.
Code Snippet Example:
// Checking for null in Java
public static void checkNull(Object obj) {
if (obj == null) {
System.out.println("Object is null");
} else {
System.out.println("Object is not null");
}
}
This simple method demonstrates how to safely handle null values in Java, a crucial aspect of preventing NullPointerExceptions.
3. GitHub
GitHub is not only a platform for version control but also an extensive resource for Java developers to discover open-source projects and collaborate with others.
Why It’s Essential:
- Access to Libraries and Frameworks: Many popular Java libraries and frameworks are hosted here. You can contribute or simply observe how they work.
- Version Control: GitHub helps track changes in your projects, enabling better collaboration and code management.
- Learning from Code: Browsing through repositories lets developers study real-world applications, improving their own coding practices.
Code Snippet Example:
// Simple demonstration of a singleton pattern
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is crucial in scenarios where a single point of control is necessary like configuration settings.
4. Java Design Patterns
The concept of design patterns can be a game-changer in writing maintainable and efficient code. Familiarizing yourself with Java Design Patterns should be a priority.
Why It’s Essential:
- Proven Solutions: Design patterns are tried-and-true methods for solving common software design issues.
- Improved Communication: Using design patterns helps streamline communication among developers through a shared vocabulary.
- Maintainability: Code that uses well-known patterns can be easier to maintain and extend over time.
Code Snippet Example:
// Example of a Factory Pattern
public class ShapeFactory {
public Shape getShape(String shapeType) {
if (shapeType == null) {
return null;
}
if (shapeType.equalsIgnoreCase("CIRCLE")) {
return new Circle();
} else if (shapeType.equalsIgnoreCase("RECTANGLE")) {
return new Rectangle();
}
return null;
}
}
The Factory pattern encapsulates the creation logic, making the code more flexible and easier to adapt. If a new shape is required, you won't need to modify existing code but only add a new class.
5. Java Blogs and Tutorials
There are multitude of blogs and tutorials that cover a wide range of Java topics. Some notable ones include Baeldung, Javatpoint, and Java Code Geeks.
Why It’s Essential:
- Up-to-Date Information: Blogs often provide insights into current trends, libraries, and best practices in the Java community.
- Diverse Content: From beginner to advanced topics, you're likely to find something that fits your learning curve.
- Detailed Tutorials: Most blogs feature step-by-step guides, making it easier to follow along, especially for complex implementations.
Code Snippet Example:
// Example of a stream operation
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> languages = Arrays.asList("Java", "Python", "JavaScript");
languages.stream()
.filter(lang -> lang.startsWith("J"))
.forEach(System.out::println);
}
}
This snippet uses Java Streams API to filter out programming languages that start with 'J'. This showcases the power of functional programming style available in Java 8 and above.
The Bottom Line
Being a Java developer today means staying informed and using the best resources available. The five resources discussed in this post will not only aid you in your day-to-day programming tasks but also enhance your overall understanding of the language and its ecosystem. Remember that continuous learning is vital in the tech industry – embrace these resources and watch your development skills soar!
For further reading and more resources, consider visiting Java Official Documentation, GitHub Guides, and exploring other programming languages and frameworks. Happy coding!