Streamline Java App Deployment with Terraform for EKS
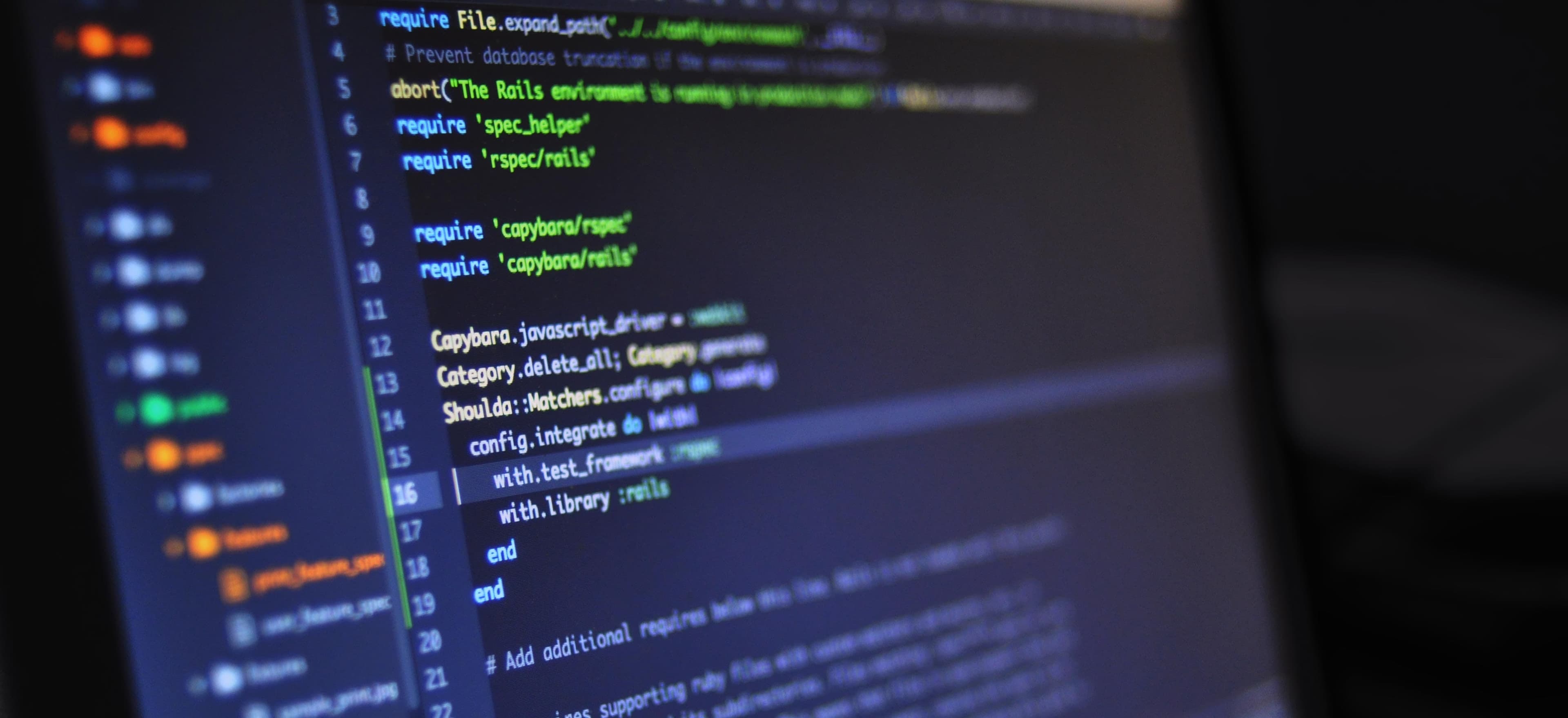
- Published on
Streamline Java App Deployment with Terraform for EKS
Deploying applications in the cloud can often feel like a daunting task, especially in an ecosystem as rich and complex as the AWS Elastic Kubernetes Service (EKS). When working with Java applications, the intricacies of environment configuration, scale, and resource management can introduce further complexity. Fortunately, using Terraform as your Infrastructure as Code (IaC) tool can significantly simplify the deployment process.
In this article, we will explore how to streamline the deployment of your Java app in EKS using Terraform. We'll highlight some best practices and provide you with practical code snippets to help you deploy efficiently. For deeper insights into managing EKS, especially in the context of auto-scaling, check out the article "Auto-Scaling Woes in EKS? Master Terraform Fixes!" at configzen.com/blog/eks-autoscaling-terraform-fixes.
Why Terraform for EKS?
Before diving into the code, it's crucial to understand why Terraform is an excellent choice for deploying applications on EKS:
-
Declarative Syntax: Terraform allows you to describe your infrastructure in simple, human-readable code, making it easy to understand what you are deploying.
-
Version Control: With Terraform, all your infrastructure code can be stored in version control systems like Git. This helps you track changes and collaborate in a team more effectively.
-
Automation: Terraform can automate the steps involved in setting up your EKS cluster and deploying applications, making your deployment pipeline faster and more reliable.
-
Multi-Cloud Compatibility: If you need to deploy across multiple cloud providers like AWS, GCP, or Azure, Terraform supports them out of the box, making your deployment seamless.
Setting Up Your EKS Cluster
To start deploying your Java application, you first need to set up your EKS cluster using Terraform. Below is a simplified example of how to create an EKS cluster:
Prerequisites
Ensure you have the following installed:
- Terraform
- AWS CLI
- kubectl
You will also need IAM permissions to create an EKS cluster.
Terraform Configuration
Create a file named main.tf
and add the following code:
provider "aws" {
region = "us-west-2" # Change to your desired region
}
resource "aws_eks_cluster" "my_cluster" {
name = "my-cluster"
role_arn = aws_iam_role.eks_cluster.arn
vpc_config {
subnet_ids = aws_subnet.my_subnet[*].id
}
}
resource "aws_iam_role" "eks_cluster" {
name = "EKS-Cluster-Role"
assume_role_policy = data.aws_iam_policy_document.eks_assume_role_policy.json
}
data "aws_iam_policy_document" "eks_assume_role_policy" {
statement {
actions = ["sts:AssumeRole"]
principals {
type = "Service"
identifiers = ["eks.amazonaws.com"]
}
}
}
resource "aws_subnet" "my_subnet" {
count = 2 # Use multiple subnets for high availability
cidr_block = "10.0.${count.index}.0/24" # Modify CIDR blocks as needed
vpc_id = aws_vpc.my_vpc.id
}
resource "aws_vpc" "my_vpc" {
cidr_block = "10.0.0.0/16"
}
Commentary
-
Provider Configuration: We specify the AWS region where we want to create our resources. Change the region as per your requirement.
-
EKS Cluster: By defining the EKS cluster resource with its name and IAM role, you set the foundation for your Kubernetes cluster.
-
Networking: We create a virtual private cloud (VPC) and subnets where the cluster will be hosted. The use of multiple subnets enhances availability.
Initial Deployment
To deploy your cluster, run the following commands:
terraform init
terraform apply
Understanding EKS Components
The aforementioned configuration sets up a basic EKS cluster. However, EKS utilizes various components such as nodes, services, and IAM roles. Let's add an EKS node group so that our applications can run:
resource "aws_eks_node_group" "my_node_group" {
cluster_name = aws_eks_cluster.my_cluster.name
node_group_name = "my-node-group"
node_role_arn = aws_iam_role.node_role.arn
subnet_ids = aws_subnet.my_subnet[*].id
scaling_config {
desired_size = 2
max_size = 5
min_size = 1
}
}
resource "aws_iam_role" "node_role" {
name = "EKS-Node-Role"
assume_role_policy = data.aws_iam_policy_document.node_assume_role_policy.json
}
data "aws_iam_policy_document" "node_assume_role_policy" {
statement {
actions = ["sts:AssumeRole"]
principals {
type = "Service"
identifiers = ["ec2.amazonaws.com"]
}
}
}
Why Node Groups?
Node groups manage your worker nodes efficiently, allowing you to scale your application based on demand. The configuration above enables autoscaling, which is crucial for handling varying workloads.
Deploying Your Java Application
Now that your EKS cluster is ready, the next step is deploying your Java application. For this, we'll create a Kubernetes deployment and service YAML file. Create a deployment.yaml
file and add the following code:
apiVersion: apps/v1
kind: Deployment
metadata:
name: java-app
spec:
replicas: 2
selector:
matchLabels:
app: java-app
template:
metadata:
labels:
app: java-app
spec:
containers:
- name: java-app
image: your-docker-repo/your-java-app:latest # Specify your Docker image here
ports:
- containerPort: 8080
Commentary
-
Deployment Resource: This YAML file specifies the deployment of your Java application, defining the number of replicas (or pods) that the Kubernetes will run.
-
Container Configuration: The Docker image needs to be pushed to a container registry (like Elastic Container Registry or Docker Hub). It is vital to ensure that the Java application is containerized properly.
Exposing Your Application
To expose your Java application externally, create a service.yaml
file:
apiVersion: v1
kind: Service
metadata:
name: java-app-service
spec:
type: LoadBalancer
selector:
app: java-app
ports:
- port: 80
targetPort: 8080
Explanation
- Service Resource: By defining a service of type LoadBalancer, Kubernetes automatically provisions an AWS ELB (Elastic Load Balancer) that routes traffic to your Java application.
The Last Word
Deploying a Java application on EKS using Terraform streamlines the complex infrastructure management process. The combination of Terraform's declarative syntax and EKS's auto-scaling capabilities allows you to focus more on application development and less on managing cloud resources.
Remember, effective scaling and deployment are key to achieving a robust architecture. If you encounter auto-scaling issues, refer to the excellent resource titled "Auto-Scaling Woes in EKS? Master Terraform Fixes!" at configzen.com/blog/eks-autoscaling-terraform-fixes.
By implementing the strategies discussed in this article, you can ensure your Java applications are deployed efficiently and can scale as needed in EKS. Happy coding and deploying!