Common Pitfalls When Decompiling Java 7 Project Coin
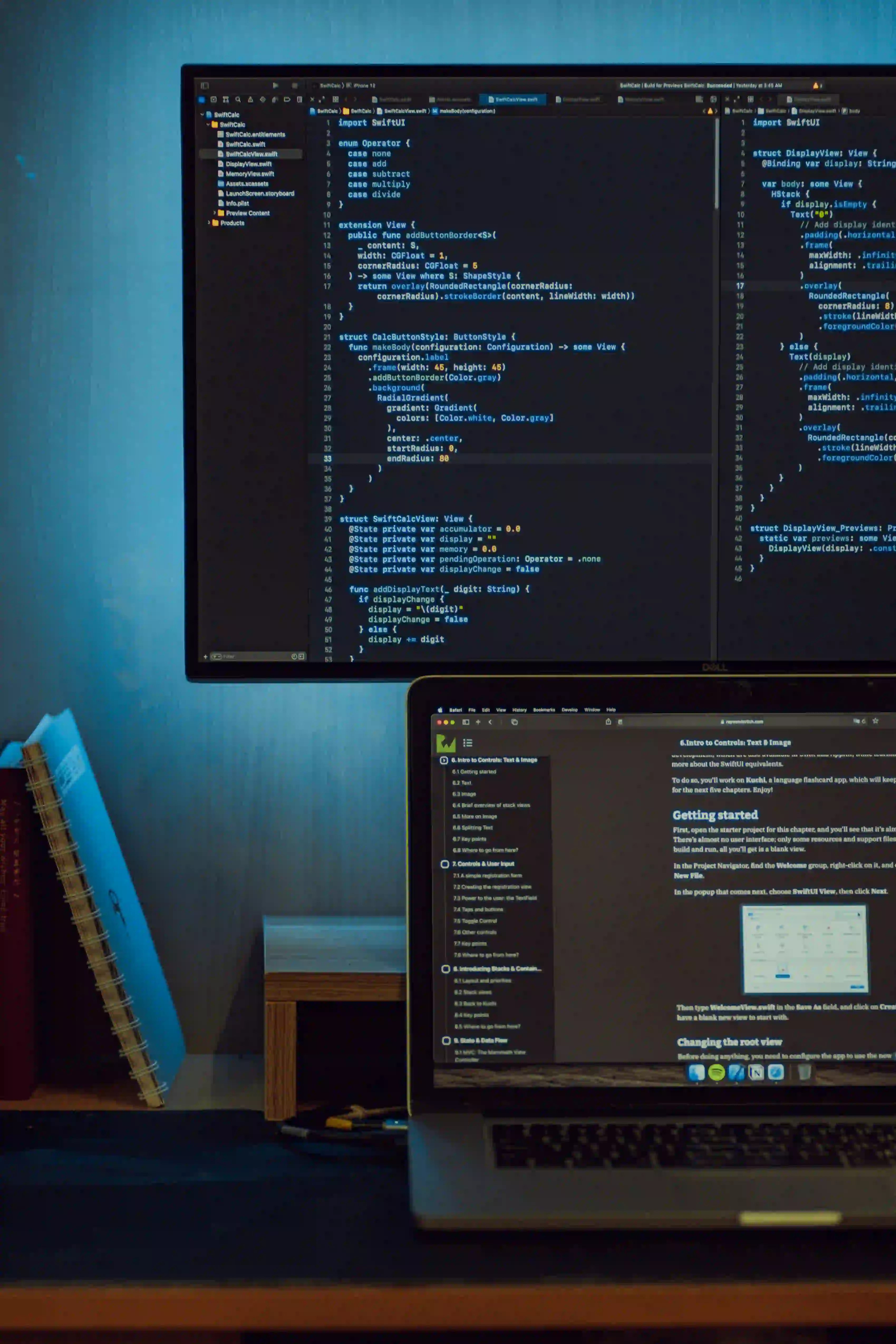
Common Pitfalls When Decompiling Java 7 Project Coin
Decompiling Java code can sometimes feel like solving a jigsaw puzzle where several pieces are lost. When it comes to Java 7, which was introduced with a variety of enhancements popularly dubbed as Project Coin, decompilation can reveal certain pitfalls that developers should be aware of. In this blog post, we will explore common issues encountered when decompiling Java 7 Project Coin features, illustrate them with code snippets, and offer insights into how to handle these challenges effectively.
Understanding Java 7 Project Coin
Java 7, released in July 2011, introduced several language enhancements under the Project Coin initiative. These included:
- Strings in switch statements
- Type inference for generic instance creation
- Try-with-resources statement
- Binary literals
- Underscore in numeric literals
For more details on the enhancements that came with Project Coin, you can visit the Oracle documentation.
What is Decompilation?
Decompilation is the process of converting bytecode (JAR or .class files) back to source code. While decompilers can assist in this process, the output can often be less readable than the original code, especially when the code leverages complex language features.
Common Pitfalls
Let's dive into the common pitfalls when decompiling code utilizing Java 7 features.
1. Loss of Variable Names
One of the significant issues with decompiled code is the loss of meaningful variable names. When code is compiled, variable names become part of the metadata, which can often be lost, particularly in optimization runs.
// Original code
public class Sample {
public void displayMessage(String message) {
System.out.println(message);
}
}
// Decompiled code might look like this
public class Sample {
public void displayMessage(String arg0) {
System.out.println(arg0);
}
}
Why it matters: Understanding the context of code becomes challenging when variables are renamed to generic identifiers. This makes the decompiled code harder to maintain or troubleshoot.
Tip: Always keep track of the original source files if possible. Using version control can help maintain a reference to variable names.
2. Misinterpretation of Try-with-resources
Java 7 introduced the try-with-resources statement that simplifies resource management. However, decompilers may struggle to reflect this accurately.
// Original code
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
// Decompiled code could look like this
BufferedReader br = new BufferedReader(new FileReader("file.txt"));
try {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} finally {
if (br != null) br.close();
}
Why it matters: The absence of the try-with-resources syntax means resources may not be closed properly, leading to memory leaks or issues with resource management.
Tip: Manually refactor any decompiled try-with-resources code to restore the structure for proper resource management.
3. Unreadable Anonymous Classes and Lambdas (With Project Coin)
Although lambdas are not part of Java 7, anonymous classes became prevalent in Java 7 projects before lambda expressions were introduced in Java 8. Decompilers can error in interpreting these constructs.
// Original code
Runnable r = new Runnable() {
@Override
public void run() {
System.out.println("Hello, World!");
}
};
// Decompiled code might produce:
Runnable r = new Runnable() {
public void run() {
System.out.println("Hello, World!");
}
};
Why it matters: Anonymous classes can become convoluted when decompiled, making it difficult to follow the logic.
Tip: Carefully analyze the structure of decompiled anonymous classes and consider converting them back to named classes if necessary.
4. Overly Complicated Binary Literals
Java 7 allows binary literals, which can enhance readability. However, some decompilers may struggle, showing binary representations in complex forms.
// Original code
int mask = 0b101010; // binary literal
// Decompiled code might show:
int mask = Integer.parseInt("101010", 2); // converts from binary to decimal.
Why it matters: This conversion is less readable than using the binary literal directly, making it harder for developers to understand what the code does at a glance.
Tip: Always check if the logic represented by binary literals makes sense in the context of the application. Refactor if needed.
Choosing the Right Decompiler
Not all decompilers handle Java 7 and Project Coin features equally. Some commonly used Java decompilers include:
- JD-GUI: A popular Java decompiler that is user-friendly and straightforward.
- CFR: Known for its accuracy with newer Java features.
- Procyon: Excellent for decompiling Java 8 and later features.
You can find more decompilers listed on GitHub's repository, where you will also discover their capabilities and limitations.
The Bottom Line
Decompiling Java code, especially with enhancements from Java 7's Project Coin, can present several challenges. From the loss of meaningful variable names to misinterpretations of constructs like try-with-resources and complex binary literals, understanding these pitfalls is critical for maintaining the integrity of your Java projects.
By keeping these common issues in mind and choosing the right decompiler, you can mitigate potential problems and ensure your code remains readable and maintainable. Always prioritize preserving original sources, review decompiled code carefully, and be prepared to refactor where necessary.
For more insights on Java's evolution and its decompilation challenges, stay tuned to our upcoming posts!
This blog post not only points out the challenges but also emphasizes the importance of being proactive in your approach to decompilation, promoting better coding practices and understanding of Java's features.