Common Pitfalls in Groovy Grails Development
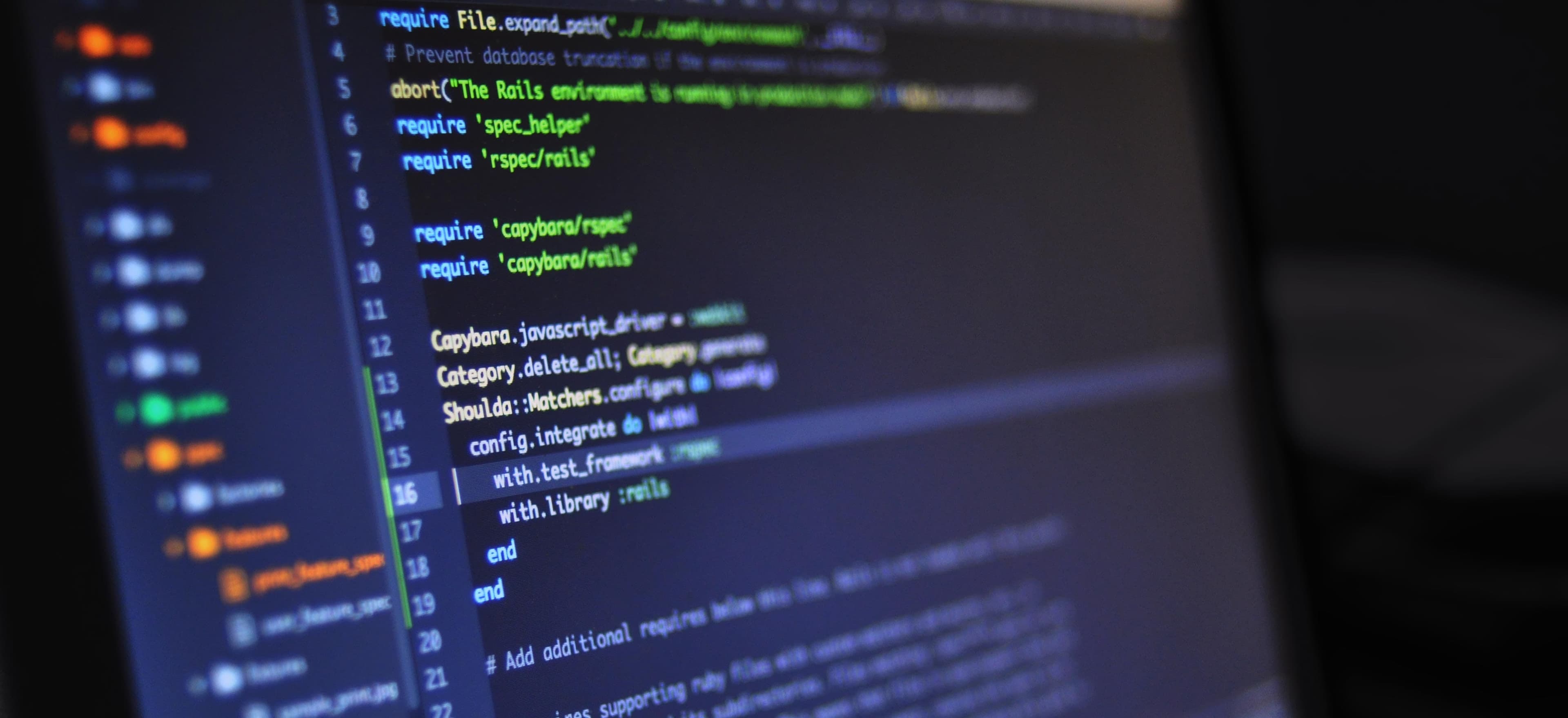
- Published on
Common Pitfalls in Groovy Grails Development
Grails, a web application framework that leverages the Groovy language and the Spring framework, is a powerful tool for developers seeking to create web applications rapidly. However, even seasoned developers can stumble upon various pitfalls when working with Groovy and Grails. In this post, we will explore the common mistakes made during Grails development, why they occur, and how they can be avoided.
1. Misunderstanding Grails Conventions
One of the cornerstones of Grails is its "convention over configuration" philosophy. While this philosophy makes it easy to get started, it can lead to misunderstandings and misconfigurations.
Why This Matters
Grails expects a standard directory structure and naming conventions. Deviating from these can cause unexpected behavior. For example, if you place your domain classes outside the grails-app/domain
directory, Grails won't recognize them.
Solution
Always adhere to the standard Grails project structure. Utilize the conventions effectively to streamline your development process. Familiarize yourself with the Grails documentation to better understand the framework's expectations.
2. Ignoring Grails Plugins
Grails has a rich ecosystem of plugins that can simplify your work, yet many developers either overlook them or try to recreate functionality from scratch.
Why This Matters
Reinventing the wheel not only wastes time but can also lead to buggy implementations. For instance, using the Spring Security Core Plugin can expedite user authentication setup significantly.
Solution
Before starting your implementation, explore available plugins. Many common functionalities, such as user authentication, pagination, and file uploads, already exist as plugins. Spend some time reviewing the Grails Plugin Portal.
// Example of applying Spring Security plugin in build.gradle
plugins {
compile "grails-plugin-spring-security-core:4.0.0"
}
3. Not Utilizing GORM Efficiently
GORM (Grails Object Relational Mapping) simplifies database operations, but inefficient usage can lead to performance issues, such as lazy loading pitfalls.
Why This Matters
Many developers may find themselves fetching large datasets without considering the implications, leading to LazyInitializationExceptions
or significant memory overhead.
Solution
Always be cautious with your queries. Use withCriteria
and dynamic finders
effectively to limit data fetched from the database.
// Efficiently fetching users who are active
def activeUsers = User.createCriteria().list {
eq('enabled', true)
}
By filtering results when querying, you minimize performance hit and memory usage.
4. Poor Error Handling
Grails applications can fail without proper error handling. Developers often forget to manage exceptions adequately, leading to unhelpful application responses.
Why This Matters
Uncaught exceptions can expose internal application details or result in poor user experience. You might come across a white screen of death if errors aren't handled appropriately.
Solution
Implement global exception handling. Create a grailsApplication
handler for centralized management of errors.
// Global exception handler
class GlobalExceptionHandler {
@ExceptionHandler(Exception)
def handleException(Exception e) {
log.error("An error occurred: ${e.message}", e)
render(view: 'error', model: [message: e.message])
}
}
5. Failing to Optimize GSP Pages
GSP (Groovy Server Pages) files are integral to Grails applications, yet many overlook optimization opportunities.
Why This Matters
Heavy GSP files with extensive logic can lead to slow performance. The view layer should contain minimal logic.
Solution
Keep your GSPs clean and leverage tags and templates. Move business logic to controllers or services, allowing GSPs to serve as presentation layers.
<!-- GSP Example with a tag -->
<g:each in="${userList}" var="user">
<p>${user.username}</p>
</g:each>
This keeps the presentation layer clean and improves performance.
6. Not Utilizing Unit Tests
Testing is a critical aspect of any development process, and Grails makes it easy to implement unit and integration tests. Yet, many developers bypass this crucial step.
Why This Matters
Neglecting tests can lead to fragile applications and costly bugs, especially in larger projects where changes can inadvertently break existing functionality.
Solution
Use Grails and Spock Framework to your advantage. Set up unit tests for your services and integration tests for your controllers.
// Example of a Spock unit test for a service
class UserServiceSpec extends Specification {
UserService userService = new UserService()
def "test user creation"() {
when:
def user = userService.createUser(username: 'jdoe')
then:
user.username == 'jdoe'
User.count() == 1
}
}
This not only catches errors early but ensures that added functionalities don't break existing features.
7. Failing to Monitor and Log
Many developers overlook application monitoring and logging, which is crucial for understanding application health and debugging issues.
Why This Matters
Without proper logging, it becomes challenging to diagnose issues in production. You may face delayed responses to critical problems.
Solution
Leverage Grails' built-in logging capabilities. Utilize the Log4j or SLF4J for flexible logging options.
// Example of logging within a service
class UserService {
private final Logger log = LoggerFactory.getLogger(UserService.class)
def createUser(String username) {
log.info("Creating user with username: {}", username)
// Your user creation logic
}
}
Final Thoughts
While Grails provides an excellent framework for rapid application development, being aware of its common pitfalls is essential. By adhering to conventions, utilizing plugins, optimizing GORM, managing errors, optimizing GSPs, implementing testing frameworks, and establishing robust logging, you can create more efficient, maintainable, and scalable applications.
For further reading and intricate discussions, check out the official Grails documentation or the Groovy documentation.
Happy coding!
Checkout our other articles