Unlocking Password Digest Issues in JMeter Extensions
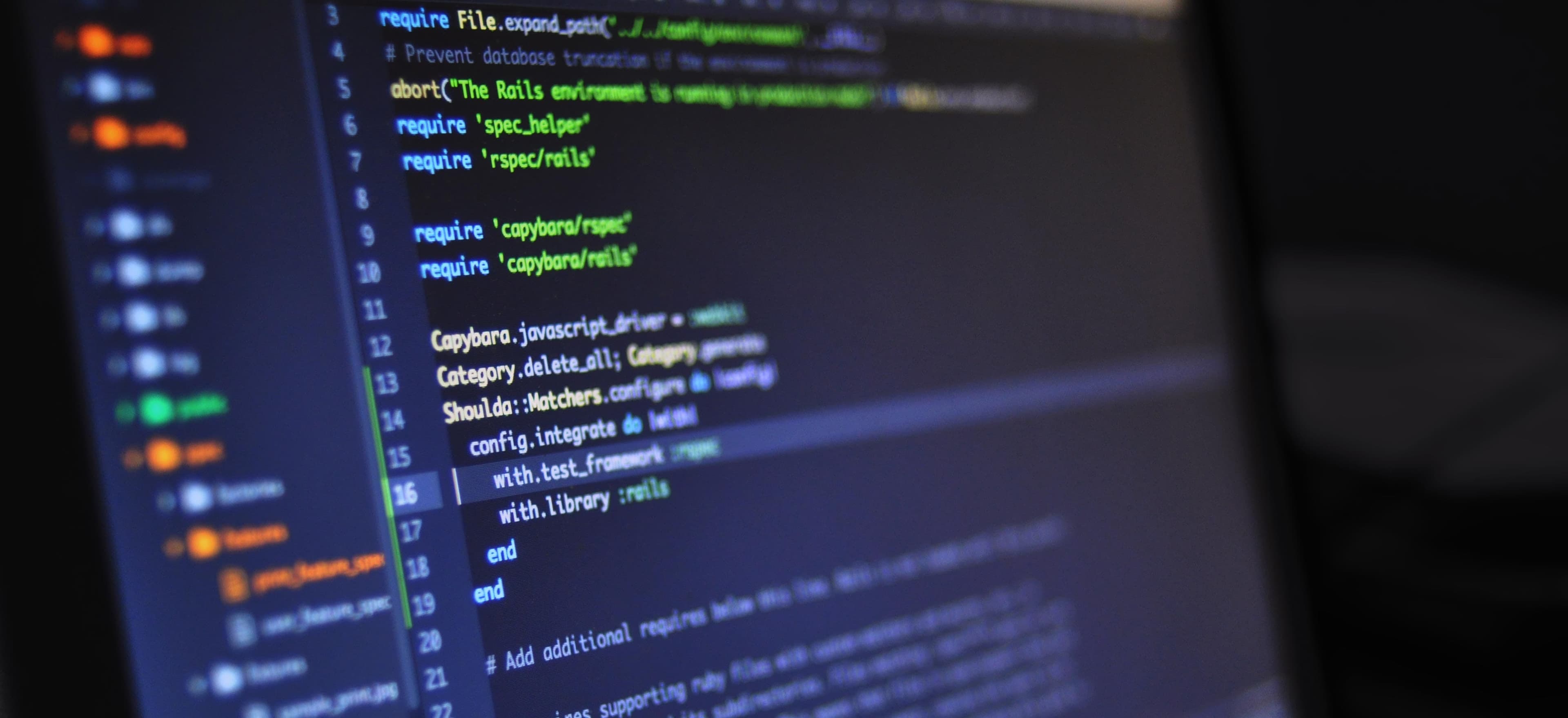
- Published on
Unlocking Password Digest Issues in JMeter Extensions
Apache JMeter is a powerful open-source tool used for performance testing and load testing web applications. Among its many features, the ability to handle security protocols, including password digest authentication, is crucial for testing applications that require secure access. In this blog post, we will delve into the challenges faced when working with password digest issues in JMeter extensions and provide practical solutions.
Understanding Password Digest Authentication
Before we dive into the specifics of JMeter extensions, it's essential to understand what password digest authentication is. This method enhances basic authentication by using a nonce and a hash function to prevent the transmission of a user's plaintext password over the network.
The key components of password digest authentication include:
- Nonce: A unique, one-time value generated for each session.
- Realm: The protection space to which a user is attempting to authenticate.
- Hash function: Combines the username, password, nonce, and other data to create a secure hashed value.
Why Use Password Digest Authentication?
Using password digest authentication enhances security:
- Prevents Eavesdropping: Passwords are not transmitted in plain text.
- Mitigates Replay Attacks: The nonce ensures that each request is unique.
The Challenge with JMeter Extensions
While JMeter provides robust testing capabilities, handling password digest authentication can present challenges. These challenges often arise from the need to manually generate and send the appropriate digest information with each request.
Common Issues with Password Digest in JMeter
- Nonce Generation: JMeter does not automatically handle nonce values, which can lead to failed authentication attempts.
- Time Sensitivity: Nonces can expire. If your tests run too slowly, the nonce might no longer be valid when you send your request.
- Hashing Implementation: The default method of generating the required hash might not align with the server’s expectations.
Solution: Custom JMeter Sampler
To address these issues, we can create a custom JMeter sampler that handles the password digest authentication seamlessly. Below is an example of how to implement this.
Creating a Custom Sampler
Here’s a simple custom sampler that will manage the password digest authentication.
Step 1: Extension Class
package com.yourcompany.jmeter;
import org.apache.jmeter.protocol.http.sampler.HTTPSamplerBase;
import org.apache.jmeter.samplers.SampleResult;
public class DigestAuthSampler extends HTTPSamplerBase {
private String username;
private String password;
private String realm;
private String nonce;
public DigestAuthSampler(String username, String password, String realm, String nonce) {
this.username = username;
this.password = password;
this.realm = realm;
this.nonce = nonce;
}
@Override
public SampleResult sample() {
SampleResult result = new SampleResult();
try {
String response = sendAuthenticatedRequest();
result.setResponseData(response, "UTF-8");
result.setSuccessful(true);
} catch (Exception e) {
result.setSuccessful(false);
result.setResponseMessage(e.getMessage());
}
return result;
}
private String sendAuthenticatedRequest() {
// Implement the logic to send the request and return the server response
// Here would go the code to calculate the hash
return "Server response";
}
}
Step 2: Handling Digest Calculation
In the sendAuthenticatedRequest()
method, you would implement the logic to create the digest hash required for the authentication. Below is a concise version of how to implement the hashing part.
import java.security.MessageDigest;
public String calculateDigest(String method, String uri) throws Exception {
String A1 = username + ":" + realm + ":" + password;
String A2 = method + ":" + uri;
String ha1 = md5(A1);
String ha2 = md5(A2);
// Repeat the nonce handling, adding the necessary values
return ha1 + ":" + nonce + ":" + ha2; // This needs to be hashed again
}
private String md5(String input) throws Exception {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] messageDigest = md.digest(input.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : messageDigest) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
Commentary on Why This Works
- Secure Hashing: The use of MD5 (or SHA-256 for stronger security) ensures that sensitive credentials are not exposed.
- Separation of Concerns: The method
calculateDigest
cleanly separates the digest computation from the network request, enhancing maintainability.
Testing the Custom Sampler
Once you’ve implemented the custom sampler, you need to test it. Here are some steps to validate the functionality:
- Set Up JMeter: Include your custom sampler in the classpath.
- Configure a Test Plan: Create a thread group and add the custom sampler to it.
- Check for Nonce Expiration: Run your tests at a pace that simulates real-world usage and ensure that the nonce is valid throughout.
Troubleshooting Tips
- Monitor Log Files: JMeter logs can provide insights into failed authentication attempts. Use the Listener like View Results Tree to see the request and response.
- Debugging: Use debug Samplers to check intermediate values, especially the nonces and generated hashes.
- Update Configuration: Ensure you have the server-side configuration correctly set up to listen for digest authentications.
Bringing It All Together
Implementing password digest authentication in JMeter requires an understanding of the underlying security principles as well as custom code to handle nonce and hash generation. By utilizing a custom sampler, you can simplify the testing process, enhance security during testing, and ensure that your application can handle secure access methodologies seamlessly.
For more information on JMeter and performance testing, consider visiting the official Apache JMeter documentation, which offers comprehensive guidance on using this powerful tool.
With the above, you now have the tools to effectively handle password digest authentication challenges in your JMeter extensions. Happy testing!