Mastering Deadlock Prevention in Java Threads
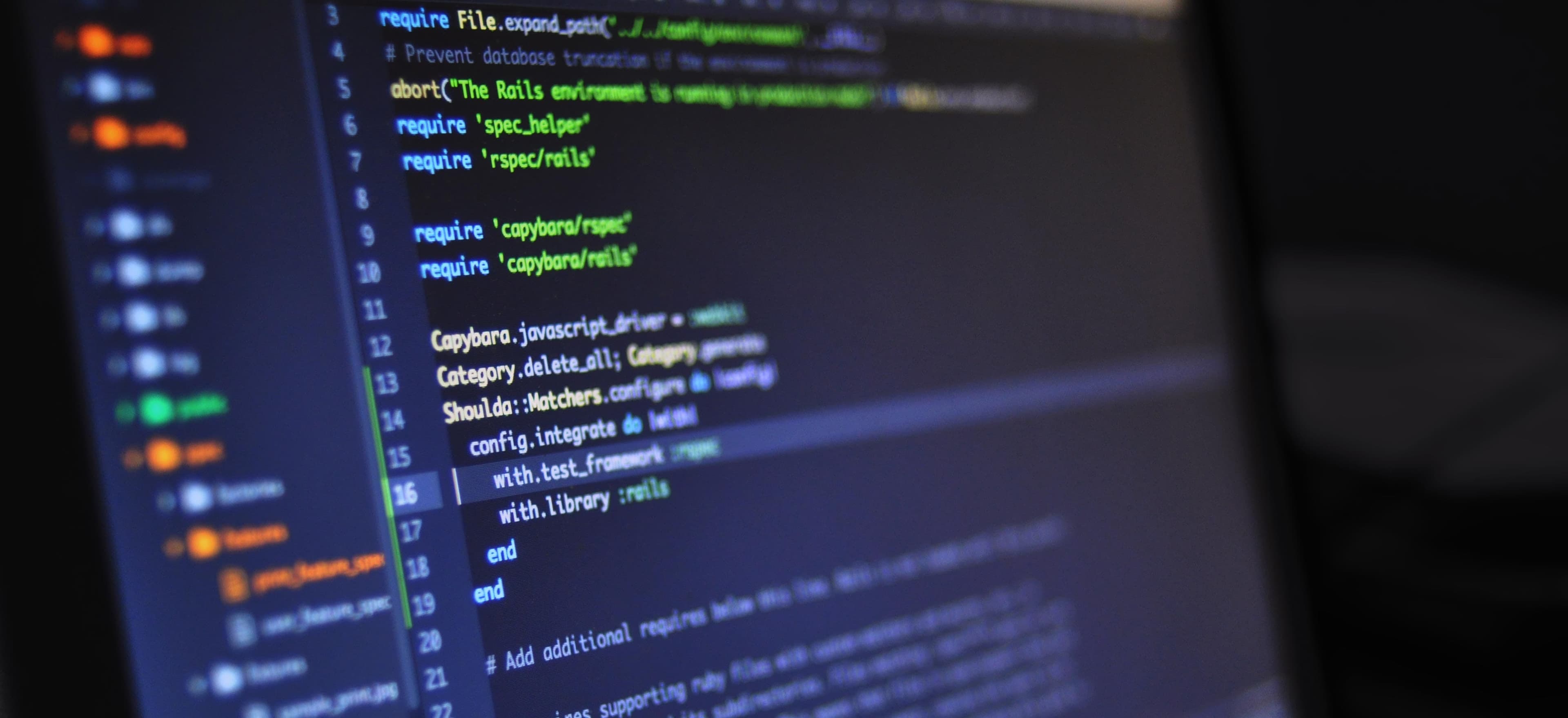
- Published on
Mastering Deadlock Prevention in Java Threads
When building complex multi-threaded applications in Java, developers often encounter challenges related to concurrency, one of which is deadlock. Deadlocks can lead to unresponsive applications and affect the user experience. In this blog post, we’ll delve into deadlocks, explore their causes, and discuss strategies for prevention in Java threads.
What is Deadlock?
A deadlock occurs when two or more threads are unable to continue execution because each thread is waiting for a resource held by one of the others. In simple terms, it's a stand-off where resources are locked up and nothing moves.
Example of a Deadlock Scenario
Consider two threads, Thread A and Thread B.
- Thread A locks Resource 1 and needs Resource 2 to proceed.
- Thread B locks Resource 2 and needs Resource 1 to proceed.
Neither thread can make progress, resulting in a deadlock.
Why Deadlocks Matter
Deadlocks are detrimental to application performance and user experience. Identifying and resolving them can be complex. Thus, prevention is often the best strategy.
Common Causes of Deadlocks
- Lock Ordering: If multiple threads acquire locks in different orders.
- Resource Contention: Multiple threads competing for shared resources.
- Improper Lock Management: Disabling timeouts or failing to release locks properly.
Strategies for Deadlock Prevention
1. Lock Ordering
One effective method of avoiding deadlocks is to enforce a strict order on acquiring locks. Each thread will lock resources in a well-defined sequence.
public class LockOrderingExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void thread1() {
synchronized (lock1) {
System.out.println("Thread 1 acquired lock 1");
synchronized (lock2) {
System.out.println("Thread 1 acquired lock 2");
// Perform task within the locks
}
}
}
public void thread2() {
synchronized (lock1) { // Thread 2 tries to acquire lock1 first
System.out.println("Thread 2 acquired lock 1");
synchronized (lock2) { // Then lock2
System.out.println("Thread 2 acquired lock 2");
// Perform task within the locks
}
}
}
}
Commentary
In this example, if both threads attempt to acquire lock1
and lock2
in the same order, it reduces the chance of a deadlock. Establish a locking hierarchy that's strictly followed by all threads.
2. Using Timeout for Locks
Java’s ReentrantLock
supports the tryLock
method, allowing threads to attempt to acquire locks without blocking indefinitely.
import java.util.concurrent.locks.ReentrantLock;
public class TimeoutExample {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void thread1() {
try {
if (lock1.tryLock()) {
System.out.println("Thread 1 acquired lock 1");
try {
if (lock2.tryLock()) {
System.out.println("Thread 1 acquired lock 2");
// Perform task
} else {
System.out.println("Thread 1 could not acquire lock 2");
}
} finally {
lock1.unlock();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
public void thread2() {
try {
if (lock2.tryLock()) {
System.out.println("Thread 2 acquired lock 2");
try {
if (lock1.tryLock()) {
System.out.println("Thread 2 acquired lock 1");
// Perform task
} else {
System.out.println("Thread 2 could not acquire lock 1");
}
} finally {
lock2.unlock();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
Here, both thread1
and thread2
attempt to acquire locks without getting blocked if the lock is not available. If one thread cannot acquire a lock, it can release its current lock and try later, thus preventing a deadlock.
3. Thread Coordination
Utilize higher-level concurrency utilities from the java.util.concurrent
package. These abstractions handle a lot of lock management for you, significantly reducing the chances of a deadlock.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadCoordinationExample {
public void executeTasks() {
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.execute(() -> {
// Task 1 logic
});
executor.execute(() -> {
// Task 2 logic
});
executor.shutdown();
}
}
Commentary
Executor services manage the lifecycle of threads and manage resources more effectively. By allowing the framework to handle threading, you're mitigating the risks of manual lock management leading to deadlocks.
4. Adding More Granular Locks
Instead of locking whole resources, lock smaller components where possible. By reducing the scope of locking, you decrease the chance of contention which helps in avoiding deadlocks.
public class GranularLockExample {
private final Object lockA = new Object();
private final Object lockB = new Object();
public void processA() {
synchronized (lockA) {
// Critical section for resource A
}
}
public void processB() {
synchronized (lockB) {
// Critical section for resource B
}
}
}
Commentary
Granular locking means fewer resources locked at any given time. This allows other threads to continue processing without being hindered by a lock held elsewhere.
Key Takeaways
Deadlock prevention in Java is critical for maintaining the performance and responsiveness of applications. Employing techniques such as lock ordering, using timeouts, leveraging concurrency utilities, and refining lock granularity can greatly aid in mitigating deadlock risks.
For further exploration of Java’s threading capabilities and comparisons between different concurrency strategies, consider checking out the Java Concurrency in Practice guide.
Always remember, a solid understanding of threading and effective resource management will lead to smoother and more efficient Java applications. Happy coding!
Additional Resources
Feel free to leave your comments or questions below!