Boost Your Java Skills: Building a Fast Expression Evaluator
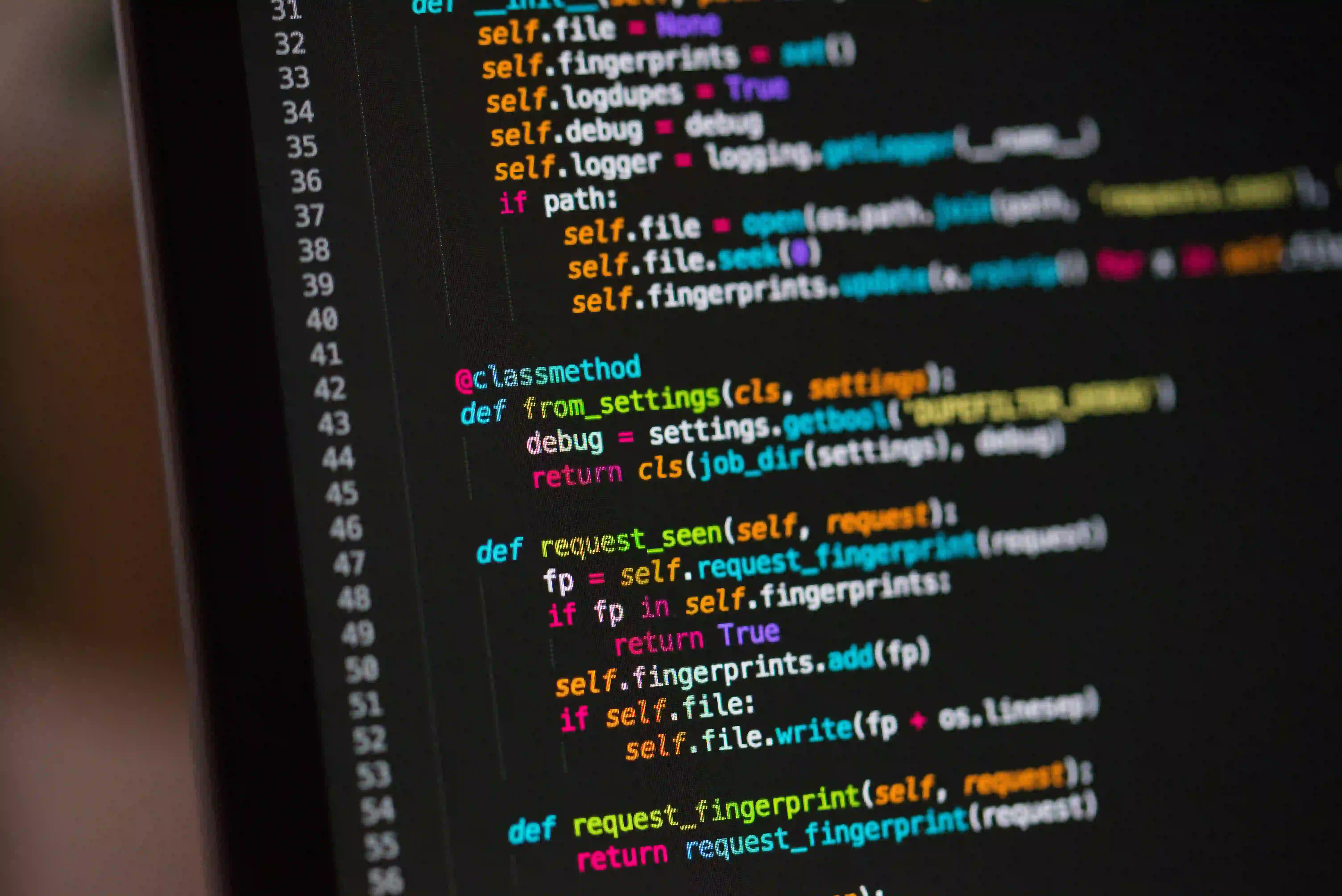
Boost Your Java Skills: Building a Fast Expression Evaluator
In the world of programming, skill enhancement is a continual journey. One of the exciting projects that can help elevate your Java skills is building a Fast Expression Evaluator. This project not only strengthens core programming concepts but also introduces you to advanced topics such as data structures and algorithms.
In this blog post, we will explore the fundamental concepts involved in creating an expression evaluator. We will cover:
- What an Expression Evaluator is
- Key components to consider
- Step-by-step implementation
- Performance considerations
- Conclusion and next steps
Let’s get started!
What Is an Expression Evaluator?
An expression evaluator is a tool that computes the result of a mathematical expression represented as a string. For example, if we have a string "3 + 5", our evaluator should return 8
.
However, the real challenge lies in handling various operators, precedence rules (like multiplication before addition), and parentheses.
Core Components
Before diving into the implementation, let's outline the key components we'll need to create our evaluator:
-
Tokenization: Breaking down the input string into manageable tokens (numbers, operators, parentheses).
-
Parsing: Understanding the hierarchical structure of the tokens, which dictates how operations are performed.
-
Evaluation: Computing the mathematical results based on the parsed structure.
Step-by-Step Implementation
Step 1: Tokenization
Tokenization is essential to simplify the evaluation process. We'll convert the incoming expression into a list of tokens. Here's a simple implementation in Java:
import java.util.ArrayList;
import java.util.List;
public class Tokenizer {
public List<String> tokenize(String expression) {
List<String> tokens = new ArrayList<>();
StringBuilder token = new StringBuilder();
for (char ch : expression.toCharArray()) {
if (Character.isDigit(ch) || ch == '.') {
token.append(ch);
} else {
if (token.length() > 0) {
tokens.add(token.toString());
token.setLength(0); // Resetting token
}
if (ch != ' ') {
tokens.add(String.valueOf(ch)); // Add operator or parenthesis
}
}
}
if (token.length() > 0) {
tokens.add(token.toString());
}
return tokens;
}
}
// Example usage
Tokenizer tokenizer = new Tokenizer();
List<String> tokens = tokenizer.tokenize("3 + 5 * (2 - 8)");
System.out.println(tokens); // [3, +, 5, *, (, 2, -, 8, )]
Explanation
- Why tokenization?: The initial string needs to be parsed into meaningful components. Without this step, the computation becomes complex and error-prone.
- Conditions: We check each character to determine if it belongs to a number or is an operator/parenthesis. This helps us build tokens efficiently.
Step 2: Parsing
Next, we need to handle operator precedence and parentheses. We will implement the Shunting Yard Algorithm by Edsger Dijkstra which simplifies expression parsing.
Here is a basic parser implementation:
import java.util.ArrayDeque;
import java.util.Deque;
import java.util.List;
public class Parser {
private static int precedence(String op) {
switch (op) {
case "+":
case "-":
return 1;
case "*":
case "/":
return 2;
default:
return 0;
}
}
public Deque<String> parse(List<String> tokens) {
Deque<String> output = new ArrayDeque<>();
Deque<String> operators = new ArrayDeque<>();
for (String token : tokens) {
if (Character.isDigit(token.charAt(0))) {
output.add(token);
} else if (token.equals("(")) {
operators.add(token);
} else if (token.equals(")")) {
while (!operators.isEmpty() && !operators.peek().equals("(")) {
output.add(operators.pop());
}
operators.pop(); // remove '('
} else {
while (!operators.isEmpty() && precedence(operators.peek()) >= precedence(token)) {
output.add(operators.pop());
}
operators.add(token);
}
}
while (!operators.isEmpty()) {
output.add(operators.pop());
}
return output;
}
}
// Example usage
Parser parser = new Parser();
Deque<String> parsedExpression = parser.parse(tokens);
System.out.println(parsedExpression);
// Expected output could be: [3, 5, 2, 8, -, *, +]
Explanation
- Why parse?: Parsing allows us to rearrange tokens according to their precedence rules. It translates the infix expression (like
3 + 5 * 2
) into postfix notation (like3 5 2 * +
), which is easier to evaluate programmatically. - Handling parentheses: The implementation respects groupings of operations using a stack structure for the operators.
Step 3: Evaluation
With the expression parsed, the next step is evaluating the postfix expression:
import java.util.Deque;
import java.util.ArrayDeque;
public class Evaluator {
public double evaluate(Deque<String> postfix) {
Deque<Double> stack = new ArrayDeque<>();
while (!postfix.isEmpty()) {
String token = postfix.poll();
if (Character.isDigit(token.charAt(0))) {
stack.push(Double.parseDouble(token));
} else {
double right = stack.pop();
double left = stack.pop();
switch (token) {
case "+":
stack.push(left + right);
break;
case "-":
stack.push(left - right);
break;
case "*":
stack.push(left * right);
break;
case "/":
stack.push(left / right);
break;
}
}
}
return stack.pop();
}
}
// Example usage
Evaluator evaluator = new Evaluator();
double result = evaluator.evaluate(parsedExpression);
System.out.println(result); // Result of evaluation
Explanation
- Why evaluate?: This final step performs the computation using the postfix representation, enabling the evaluator to produce the correct numerical result.
- Stack usage: A stack is employed to handle the operands effectively. This avoids the complexity of tracking the current state of operations during evaluation.
Performance Considerations
While our implementation offers a straightforward approach, certain performance optimizations can significantly enhance the evaluator's speed and efficiency:
- Memoization: If the evaluator will be handling repetitive calculations, caching results can save time on future computations.
- Multi-threading: For high-demand applications, using Java’s concurrency libraries can improve performance by handling multiple evaluations simultaneously.
The Closing Argument and Next Steps
Building a fast expression evaluator in Java offers a practical exercise in mastering crucial programming concepts such as tokenization, parsing, and evaluation. The skills gained from this project can be applied to various real-world applications, including calculators, query interpreters, and data analysis tools.
As your next steps, consider extending the functionality of your evaluator by:
- Supporting additional mathematical functions such as sine, cosine, etc.
- Improving error handling to manage malformed expressions gracefully.
- Creating a graphical user interface (GUI) to enhance user interaction.
Keep enhancing your skills and explore the endless possibilities with Java!
Further Reading
- Java Documentation
- Introduction to Algorithms
- Java Concurrency in Practice
Feel free to share your implementations and further optimizations in the comments below. Happy coding!