Unauthorized Access: Troubleshooting Google Analytics API Issues
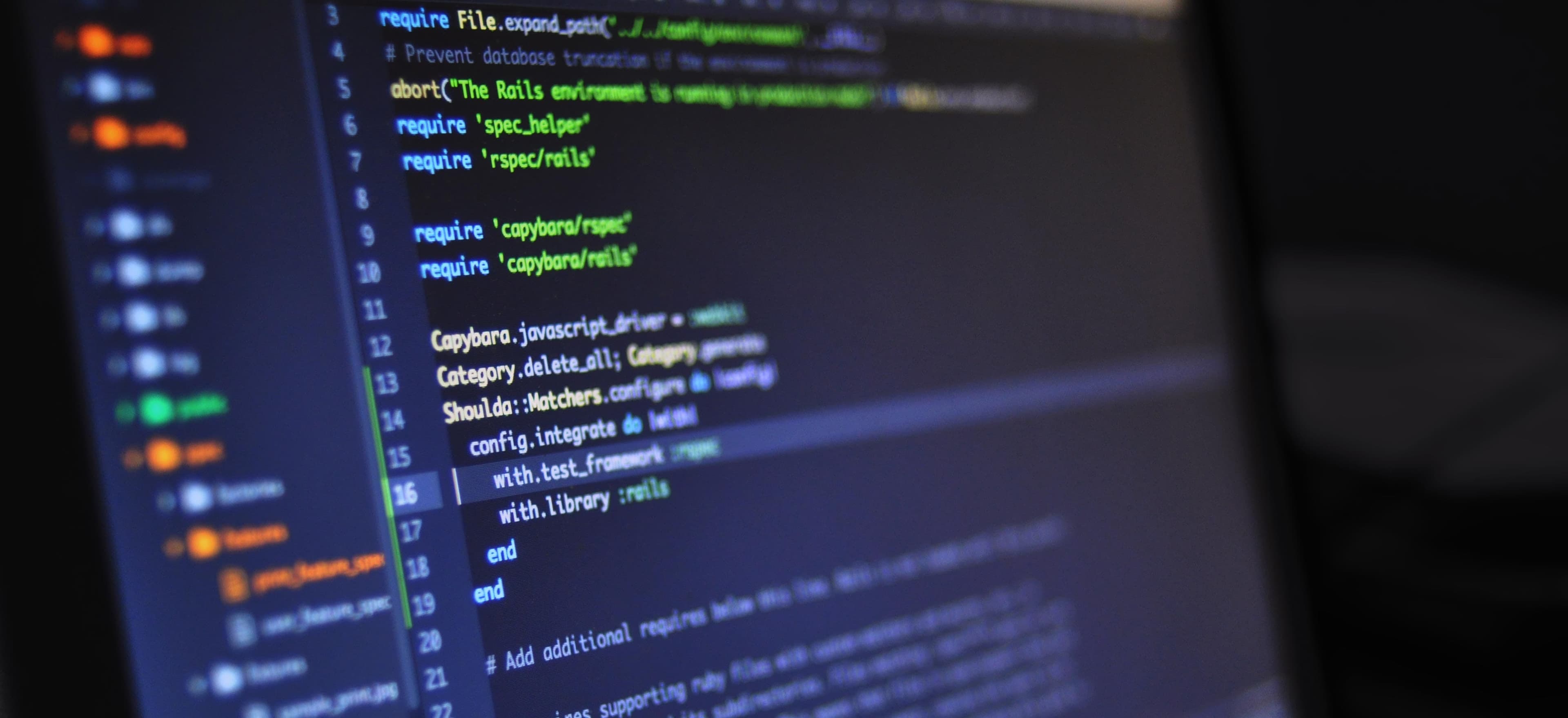
- Published on
Unauthorized Access: Troubleshooting Google Analytics API Issues
The Google Analytics API is a powerful tool that allows developers to programmatically access data from their Google Analytics account. However, many users encounter challenges when trying to access their data due to authorization issues. In this blog post, we will dive into common problems related to unauthorized access when using the Google Analytics API and provide detailed troubleshooting steps.
Understanding the Basics of Google Analytics API
Before we get into troubleshooting, it’s essential to understand what the Google Analytics API starts with. The API allows users to query and retrieve data from Google Analytics accounts, making it easier for businesses to leverage their analytics data in various applications.
Key Terms
- Client ID: A unique identifier for an app accessing the API.
- OAuth 2.0: An open standard for access delegation used to grant limited access to users' data without exposing credentials.
- Service Account: A special type of account used by applications to connect and interact with services and APIs.
Common Unauthorized Access Issues
-
Invalid OAuth Credentials
- One of the most frequent issues arises from invalid or incorrectly configured OAuth credentials. This typically occurs when copying the Client ID and Client Secret.
-
Insufficient Permissions
- Even if the credentials are valid, the user may not have the necessary permissions set for the service account or user account.
-
Quota Limits
- Google Analytics imposes quota usage limits. If you exceed these limits, you will receive an unauthorized access error.
-
Scopes Configuration
- The OAuth scopes used during the authentication process may not match what is required by the API.
-
API Access Enabled
- Sometimes, users forget to enable the Google Analytics API for their project in the Google Cloud Platform.
Step-by-Step Troubleshooting
Step 1: Validate OAuth Credentials
Make sure you are using the correct Client ID and Client Secret from your Google Cloud project.
Code Example:
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new FileReader("client_secret.json"));
String clientId = clientSecrets.getDetails().getClientId();
String clientSecret = clientSecrets.getDetails().getClientSecret();
System.out.println("Client ID: " + clientId);
System.out.println("Client Secret: " + clientSecret);
Why? Having the correct credentials is crucial because they authenticate your requests to the API. If they are incorrect, your access will be denied.
Step 2: Check Permissions
To ensure your account has the right permissions, do the following:
- Go to Google Analytics.
- Select Admin > Account > User Management.
- Ensure your user or the service account has the requisite permissions (at least 'Read & Analyze').
Step 3: Monitor Quota Limits
Check your current quota levels. You can use the Google Cloud Console to monitor your quota usage.
Why? If you've exceeded your quota, no further requests will be processed until the next renewal period, leading to unauthorized access errors.
Step 4: Review Scopes Configuration
Ensure that you request appropriate scopes when authenticating. In the case of Google Analytics, you might need:
private static final String ANALYTICS_READONLY_SCOPE = "https://www.googleapis.com/auth/analytics.readonly";
You can add scopes as follows:
ArrayList<String> scopes = new ArrayList<>();
scopes.add(ANALYTICS_READONLY_SCOPE);
// Use these scopes in the authorization process
Why? Scopes define the level of access you are requesting. Incorrect scopes can lead to rejected authentication requests.
Step 5: Enable the Google Analytics API
Sometimes, users overlook enabling the Google Analytics API in their Google Cloud project. Here’s how to check it:
- Go to the Google Cloud Console.
- Select your project.
- Navigate to APIs & Services > Library.
- Search for Google Analytics API and ensure it’s enabled.
Why? If the API isn’t enabled, your application won't be able to make requests, resulting in unauthorized access errors.
Additional Considerations
Here are a few other aspects to consider when troubleshooting unauthorized access.
-
Time Sensitivity: OAuth tokens have expiry times, which means you need to refresh them periodically.
-
Service Account Key File: If you’re using a service account, make sure that you're using a key file that hasn’t expired or been revoked.
-
Testing in Different Environments: Unauthorized access issues can sometimes be environment-specific. Testing in different setups can help identify configuration issues.
Bringing It All Together
Troubleshooting unauthorized access issues in the Google Analytics API can be daunting, particularly if the root cause is not immediately apparent. By following the steps outlined above, from validating your OAuth credentials to ensuring adequate permissions are set, you can often resolve these issues swiftly.
For more information on setting up credentials and permissions, refer to the Google Analytics API documentation and Google Cloud Console.
By ensuring that your configurations are correctly set, you can fully leverage the capabilities of the Google Analytics API and unlock valuable insights from your analytics data. Happy coding!