Choosing the Right Test: A Guide to Common Classifications
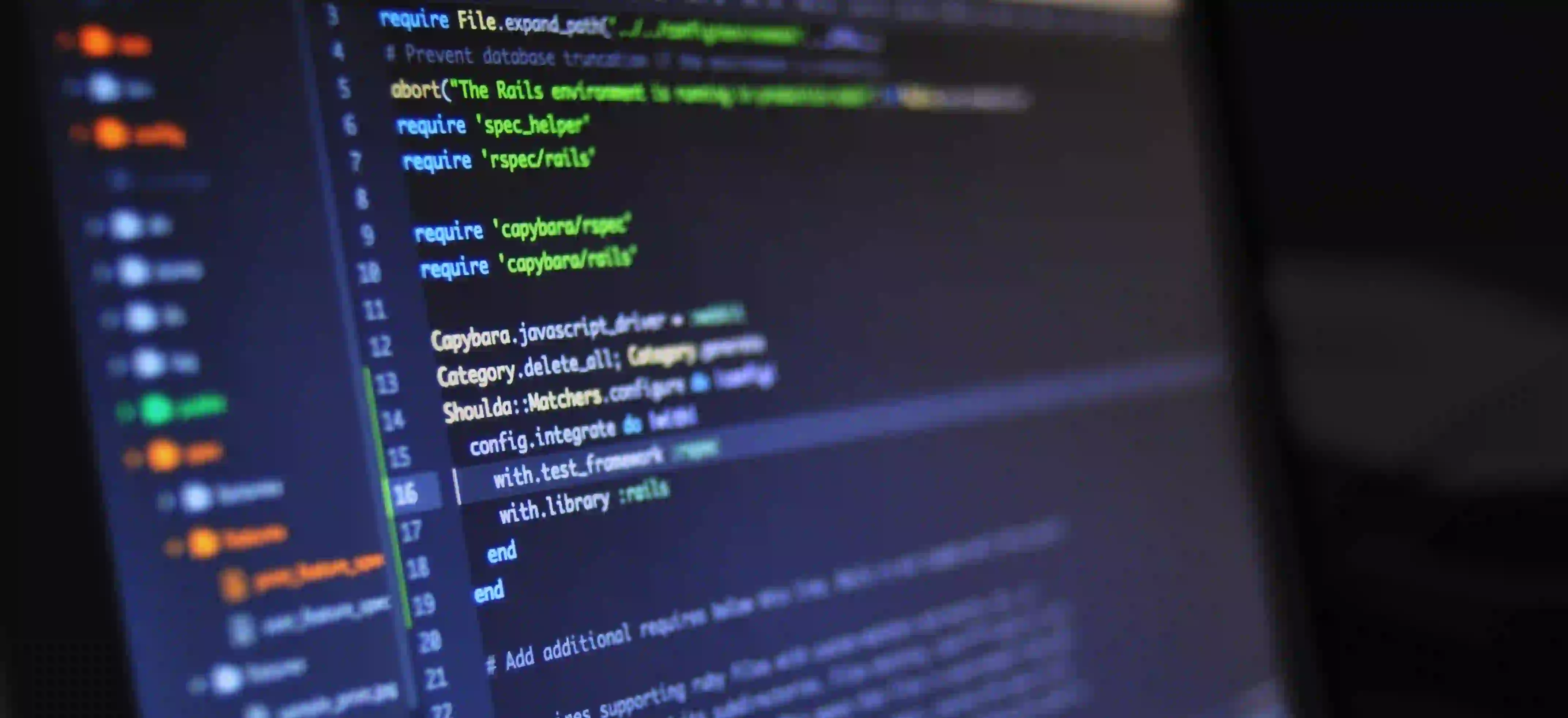
Choosing the Right Test: A Guide to Common Classifications
Testing is an essential component of software development. It ensures that applications function as expected and meet user needs. With numerous testing types available, it can be overwhelming to decide which one to use. This blog post will navigate you through common classifications of tests, their purposes, and best practices. By the end, you will have a clearer understanding of how to choose the right test for your project.
Understanding Test Classifications
When it comes to software testing, classifications can be broken down into a few key categories:
- Static vs. Dynamic Testing
- Manual vs. Automated Testing
- Functional vs. Non-Functional Testing
We will explore each type, providing clarity on its use and implementation.
Static vs. Dynamic Testing
Static Testing involves examining the code or documentation without executing the program. Techniques such as code reviews, inspections, or static analysis fall under this category.
Dynamic Testing, on the other hand, requires executing the code and observing its behavior under various conditions. This includes unit testing, integration testing, and system testing.
Why Use Static Testing?
Static Testing is beneficial for detecting specific types of errors early in the development process. According to a study by IBM, early detection can reduce development costs significantly.
Example of Static Testing
Here’s a simple example of a static analysis tool in Java using Checkstyle:
<module name="Checker">
<module name="TreeWalker">
<module name="LineLength">
<property name="max" value="80"/>
</module>
</module>
</module>
In the configuration above, Checkstyle checks if each line of Java code is no longer than 80 characters. This prevents code from becoming cluttered and enhances readability.
Manual vs. Automated Testing
Manual Testing is when tests are executed manually by testers without automated tools. It is indispensable for exploratory, usability, and ad-hoc testing.
Automated Testing uses scripts and tools to run tests automatically, allowing for efficient and repeatable testing processes. It is ideal for regression testing and performance testing.
Why Automated Testing?
Automated testing can significantly enhance efficiency. The Time vs. Cost Study suggests that automation can cut down on testing time by up to 90% for repeatable tests.
Example of Automated Testing
Here’s a simple JUnit test in Java for a hypothetical class, Calculator
:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTests {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals("Addition of 1 and 2 should be 3", 3, calc.add(1, 2));
}
}
In this example, we are testing the add
method of the Calculator
class. The @Test
annotation signals that this method is a test case. The assertEquals
method checks if the actual result equals the expected result. This setup ensures that small units function as intended, leading to less debugging later in the process.
Functional vs. Non-Functional Testing
Functional Testing assesses the software against functional requirements/specifications. It's mainly concerned with what the system does. Techniques include unit testing, system testing, and acceptance testing.
Non-Functional Testing, on the other hand, evaluates non-functional aspects such as performance, reliability, or usability. Common forms include load testing, stress testing, and security testing.
Why Both Matter
Both functional and non-functional testing are vital to delivering a reliable product. While functional tests ensure the system does what it is supposed to do, non-functional tests guarantee that it performs well under various situations.
Choosing the Right Test
Having defined different classifications, how do you choose the right tests?
-
Determine Objectives: Identify what you want to achieve with your tests. Are you looking to ensure functionality, assess performance, or verify security?
-
Consider Resources: Evaluate your resources—both in terms of tools and team skills. Manual testing can be resource-intensive, while automated testing requires an initial investment in tools.
-
Evaluate Application Stage: The stage of development impacts testing choices. For earlier stages, static analysis and manual tests may be more beneficial, while automated tests are excellent for established applications.
Best Practices for Effective Testing
To maximize the effectiveness of your testing strategy, consider these best practices:
-
Test Early and Often: Implement testing early in the software lifecycle. This allows for identifying defects sooner.
-
Maintain Test Clarity: Ensure your tests are easy to understand and maintain. Use clear descriptions for what each test is supposed to do.
-
Integrate with CI/CD: Use Continuous Integration/Continuous Deployment (CI/CD) pipelines to automate the execution of tests. Tools like Jenkins or Travis CI can help integrate testing seamlessly into your development workflow.
-
Review and Revise: Regularly review your testing strategy and adapt based on feedback and new requirements. This ensures your approach remains relevant.
Key Takeaways
Choosing the right test is pivotal to the success of your software project. By understanding the different classifications—static vs. dynamic, manual vs. automated, functional vs. non-functional—you can tailor your testing strategy to fit your needs effectively. Engaging in best practices will ensure your testing remains efficient and productive.
For further reading, explore these resources:
- ISTQB Syllabus
- Principles of Testing
Remember, a well-tested application not only meets user expectations but also enhances overall productivity and quality in the long run. Happy testing!