JavaFX vs HTML: Choosing the Right Tool for Your App
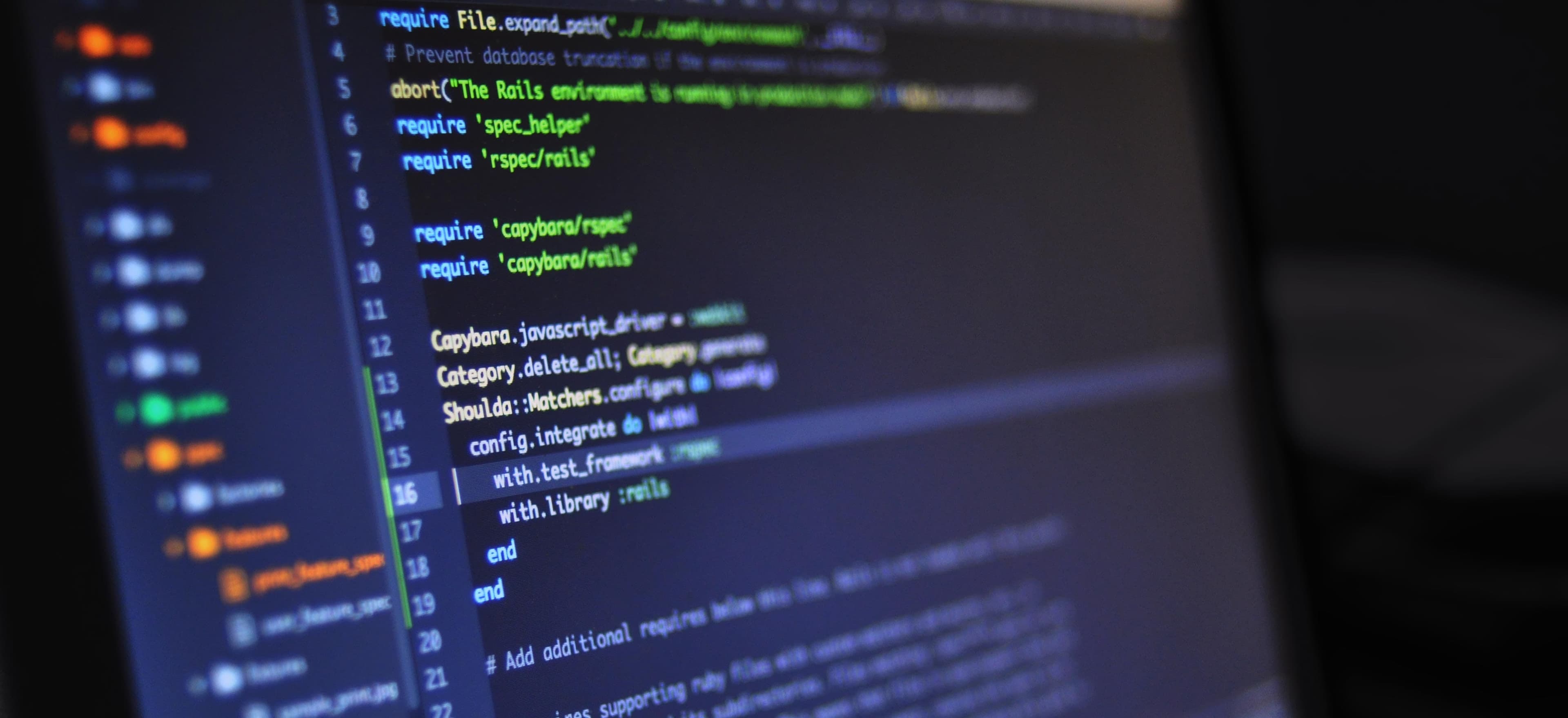
- Published on
JavaFX vs HTML: Choosing the Right Tool for Your App
When it comes to building applications, developers often find themselves at a crossroads. Should they opt for JavaFX or go with the traditional web-based approach using HTML? This decision can significantly influence application performance, user experience, and development efficiency. In this blog post, we'll explore the strengths and weaknesses of both technologies, help you make an informed choice for your project, and provide some practical code examples.
Understanding JavaFX
What is JavaFX?
JavaFX is a rich client application platform designed to provide a sophisticated UI for Java desktop applications. It allows developers to create visually stunning applications that leverage the power of Java with modern design principles.
Key Features:
- Rich Graphics Capabilities: JavaFX supports 2D and 3D graphics, making it a strong candidate for applications needing high graphical fidelity.
- CSS Styling: JavaFX allows the use of CSS for styling, enabling cleaner and more maintainable code.
- FXML: An XML-based mark-up language, FXML separates UI design from business logic.
Example of a Simple JavaFX Application:
Here’s a simple JavaFX application that creates a window with a button:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SimpleJavaFXApp extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setOnAction(event -> System.out.println("Hello World"));
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Hello World!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why JavaFX? This code illustrates the simplicity and readability of JavaFX. The UI is defined with a clear structure, separating the business logic from UI components, which is critical for maintainability.
Understanding HTML and Web Technologies
What is HTML?
HTML (HyperText Markup Language) is the foundational language of the web. It allows developers to create structured documents that can be styled with CSS and made interactive with JavaScript.
Key Features:
- Universal Accessibility: HTML applications run in browsers, making them accessible on any device with internet connectivity.
- Rich Ecosystem: JavaScript frameworks like React, Angular, and Vue.js extend HTML's capabilities for building interactive applications.
- Responsive Design: With CSS frameworks like Bootstrap, web applications can be designed to work on any screen size seamlessly.
Example of a Simple HTML Application:
Here’s a basic HTML5 application with a button:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple HTML App</title>
<style>
body { font-family: Arial, sans-serif; }
button { padding: 10px 15px; font-size: 16px; }
</style>
</head>
<body>
<button onclick="sayHello()">Say 'Hello World'</button>
<script>
function sayHello() {
console.log("Hello World");
}
</script>
</body>
</html>
Why HTML? This example shows the straightforward nature of web applications. The functionality is encapsulated in a simple button action, and developers can style and script with ease.
Comparing JavaFX and HTML
Performance
- JavaFX often delivers higher performance for applications that demand rich graphics. This is particularly important in gaming, simulation, or visualization applications.
- HTML, while potentially less performant for heavy graphics, has improved significantly with optimizations like hardware acceleration in modern browsers.
Development Environment
- JavaFX requires a Java development environment along with tools like Scene Builder for designing GUI. This might be a barrier for new developers.
- HTML development can be done with simple text editors, and numerous IDEs support web technologies. The entry barrier is lower, making it easier for novice developers.
User Experience
- JavaFX provides an OS-specific look and feel, which can lead to a more consistent user experience across desktop applications.
- HTML applications, when designed responsibly with frameworks like Bootstrap or Materialize CSS, offer modern UX patterns that are familiar to users.
Use Cases
- Use JavaFX for:
- Desktop applications needing high performance and rich visuals
- Industries like finance, where data graphs and charts are critical
- Use HTML for:
- Web applications needing cross-platform accessibility
- Mobile-first applications, where responsive design is vital
Bringing It All Together: Choosing the Right Tool
Deciding between JavaFX and HTML ultimately comes down to the specific requirements of your project.
If your application is heavily graphical and requires native desktop capabilities, JavaFX may be the better choice. However, if accessibility across various devices and platforms is your priority, then HTML is the way to go.
Considerations Before You Start
- Target Audience: Understand your end-users. Desktop vs. web audiences have different needs.
- Skills: Balance your and your team's proficiency with Java or web technologies.
- Performance Needs: Assess whether performance will be a critical factor in your application.
For additional insights on developing web applications, check out W3Schools for tutorials and JavaFX documentation for in-depth knowledge about building applications with JavaFX.
Choosing the right tool does not end here. Both JavaFX and HTML are evolving technologies with communities actively promoting best practices. Keep an eye on future developments, as they could shift the balance in favor of one technology over the other. In any case, understanding the strengths and limitations of both options will empower you to make informed decisions for your applications.
Checkout our other articles