Troubleshooting JMX Issues in Hibernate EHCache with Quartz
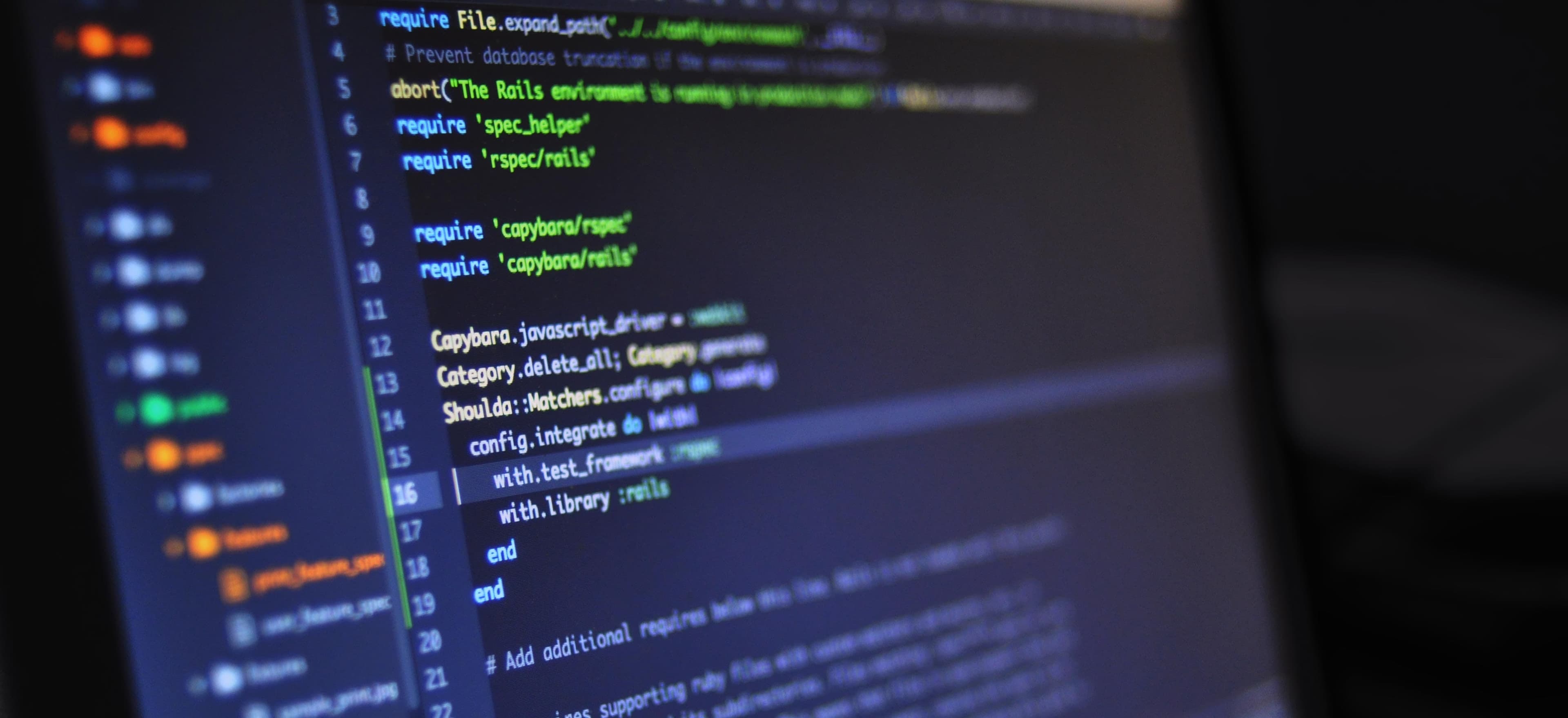
- Published on
Troubleshooting JMX Issues in Hibernate EHCache with Quartz
Using Java Management Extensions (JMX) can be crucial for monitoring and managing Java applications. When combined with Hibernate for ORM (Object-Relational Mapping), EHCache for caching, and Quartz for job scheduling, many developers may run into troubleshooting scenarios. In this post, we’ll explore how to effectively troubleshoot JMX issues in a Hibernate EHCache and Quartz integrated application.
What is JMX?
Java Management Extensions (JMX) provide a way to manage and monitor applications, system objects, and devices from a Java environment. With JMX, Java applications expose resources called MBeans (Managed Beans) which can be queried and manipulated at runtime. This capability allows developers to dynamically gather information about application performance and health.
Setting Up JMX with Hibernate and EHCache
Before diving into troubleshooting, let’s ensure you have a clear understanding of how to set up JMX in your project using Hibernate and EHCache.
Maven Dependencies
First, ensure you have the following dependencies added to your pom.xml
:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.30.Final</version>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.1</version>
</dependency>
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz</artifactId>
<version>2.3.2</version>
</dependency>
Configuring JMX in your application
Most configurations can be handled in the hibernate.cfg.xml
file. To enable JMX, add the following properties:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.jcache.JCacheRegionFactory</property>
<property name="javax.cache.CacheManager">org.ehcache.jcache.JCacheManager</property>
<property name="hibernate.jmx.enabled">true</property>
<property name="hibernate.jmx.entityManagerFactory">sessionFactory</property>
Here, the key attributes enable Second-Level Caching, configure EHCache as the cache provider, and enable JMX management for the Hibernate session factory.
Common Issues with JMX
When using JMX with Hibernate EHCache and Quartz, issues can arise. Here we will cover the common pitfalls and how to resolve them.
Issue 1: MBeans Not Registering
Symptoms: You may not see the expected MBeans in your JMX console.
Solution
This issue often arises due to JMX not being properly enabled. Ensure that your hibernate.jmx.enabled
property is set to true
and that you’re using the correct port. Here's how to enable the JMX port:
-Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=9090
-Dcom.sun.management.jmxremote.authenticate=false
-Dcom.sun.management.jmxremote.ssl=false
This allows you to connect to the JMX console at localhost:9090
.
Issue 2: EHCache Configuration Errors
Symptoms: JMX MBeans appear, but they don’t represent correct cache statistics.
Solution
Check your EHCache configuration file (usually ehcache.xml
) to ensure it's set up properly. For instance:
<ehcache>
<cache name="myCache"
maxEntriesLocalHeap="1000"
eternal="false"
timeToLiveSeconds="120"
timeToIdleSeconds="60">
<persistence strategy="none"/>
</cache>
</ehcache>
Make sure you've defined the cache correctly and that it integrates with Hibernate. Incorrect settings in either Hibernate or EHCache can lead to discrepancies in cache management and monitoring.
Issue 3: Quartz Jobs Not Triggering
Symptoms: Scheduled jobs in Quartz are not executing as expected.
Solution
Verify the job’s configuration and trigger. For instance:
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(40)
.repeatForever())
.build();
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
scheduler.start();
scheduler.scheduleJob(jobDetail, trigger);
This code snippet demonstrates how to correctly instantiate a Quartz job with a trigger. Be vigilant: if your job does not execute, verify that it is correctly registered with the scheduler and is not blocked by other jobs or misconfigured instances.
Issue 4: Memory Leaks from JMX
Symptoms: High memory usage or application crashes.
Solution
Memory leaks can occur when MBeans are not properly deregistered. When dynamically generating MBeans, ensure they are unregistered when they are no longer needed:
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("my.package.type:name=myMBean");
if (mbs.isRegistered(name)) {
mbs.unregisterMBean(name);
}
This ensures that no lingering MBeans are tied up in memory, which may cause leaks and degrade your application's performance.
Best Practices for Using JMX with EHCache and Quartz
- Enable Management Features: Always make sure to enable JMX and ensure that your configuration parameters are correct.
- Use JBoss or Other Management Tools: For large applications, consider using tools like JBoss for better MBean management.
- Regularly Monitor Performance: Use JMX to probe performance at regular intervals and adjust cache settings based on observed patterns.
- Log JMX Events: Integrate logging mechanisms to log significant JMX events for debugging purposes.
Final Considerations
Setting up and troubleshooting JMX issues in a project using Hibernate for ORM, EHCache for caching, and Quartz for scheduling can be daunting. Following the suggestions and solutions outlined above will help you navigate through common challenges. Remember to validate your configurations, leverage MBeans appropriately, and monitor your application's performance actively.
For more insights on leveraging JMX effectively, consider visiting the official JMX documentation.
By adhering to the best practices and troubleshooting techniques outlined in this post, you can ensure the reliability and efficiency of your enterprise Java applications. Happy coding!
Checkout our other articles