Understanding the Hidden Costs of Tight Coupling in OOP
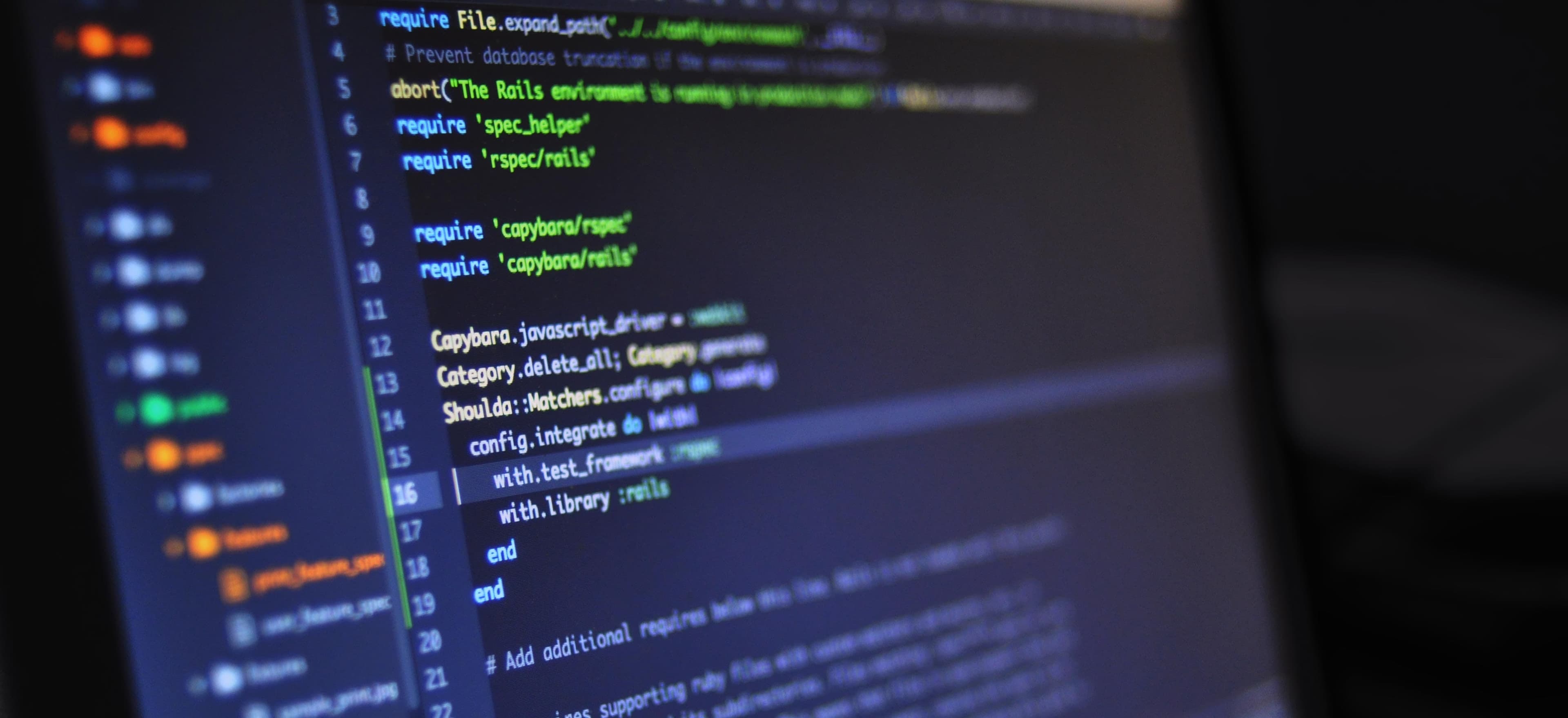
- Published on
Understanding the Hidden Costs of Tight Coupling in OOP
In Object-Oriented Programming (OOP), tight coupling refers to a scenario where classes are highly dependent on one another. While coupling is a necessary aspect of software design, tight coupling can lead to significant complexities and hidden costs that may not be immediately apparent. In this blog post, we will delve into the concept of coupling in OOP, explore the hidden costs associated with tight coupling, and finally, look at practical examples and best practices for achieving loose coupling.
What is Tight Coupling?
Tight coupling occurs when a class relies heavily on another class. This dependency can manifest in several ways, including direct references, method calls, and data exchanges. Tight coupling can create a domino effect; a change in one class may necessitate changes in others, leading to a fragile codebase that is challenging to maintain or extend.
Example of Tight Coupling
Consider the following basic example of tight coupling:
class Engine {
public void start() {
System.out.println("Engine started");
}
}
class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Direct dependency on Engine
}
public void start() {
engine.start();
}
}
In this code snippet, the Car
class is tightly coupled to the Engine
class. Any change to the Engine
class (for example, changing the method signature or adding additional logic) will result in changes in the Car
class. This creates unnecessary complexity and limits the flexibility of your application.
Hidden Costs of Tight Coupling
Tight coupling introduces several hidden costs in software development:
-
Reduced Reusability: When classes are tightly coupled, it becomes difficult to reuse them in other parts of the application or in different applications altogether. For example, if we try to reuse the
Car
class in a different project but without theEngine
class, it would not function, thus defeating the purpose of modular design. -
Increased Maintenance Costs: Making changes to a tightly coupled system often requires understanding the entire interdependency network of classes. This increases the likelihood of introducing bugs and complicates the onboarding of new developers who must navigate through the convoluted dependencies.
-
Rigidity: Tight coupling tends to make systems inflexible. Innovations in software often require changes to existing classes. If classes are tightly integrated, altering one part of the system can lead to significant, unintended cascading effects across other parts, ultimately reducing the velocity of development.
-
Difficulties in Testing: Tight coupling complicates unit testing. When you test a class that has dependencies on other classes, you end up testing multiple classes simultaneously, which goes against the principles of unit testing where each component is tested in isolation.
-
Challenges in Scaling: In a tight coupling scenario, scaling your application to meet increased demand or accommodate new features often requires cascading changes to many interdependent classes. This is especially problematic in larger systems, where the cost of scaling could outweigh the benefits.
Achieving Loose Coupling
The goal in software design should be to strive for loose coupling, where components have minimal dependencies on one another. This can be accomplished using several strategies:
Dependency Injection
One of the most effective techniques for reducing coupling is Dependency Injection (DI). By injecting dependencies rather than hard-coding them, you decouple classes and enhance their reusability.
Here’s a refactored version of our previous example using DI:
class Engine {
public void start() {
System.out.println("Engine started");
}
}
class Car {
private Engine engine;
// Constructor Injection
public Car(Engine engine) {
this.engine = engine; // Injected dependency
}
public void start() {
engine.start();
}
}
// Usage
public class Application {
public static void main(String[] args) {
Engine myEngine = new Engine();
Car myCar = new Car(myEngine); // Now Car is loosely coupled to Engine
myCar.start();
}
}
In this implementation, the Car
class no longer creates an instance of Engine
. Instead, it receives an Engine
instance through the constructor. This approach enhances flexibility, permitting the use of various engines without altering the Car
class.
Interfaces and Abstract Classes
Another way to reduce coupling is to program against interfaces or abstract classes rather than concrete implementations. This abstraction enables you to swap out different implementations without affecting the dependent classes.
interface Engine {
void start();
}
class V6Engine implements Engine {
public void start() {
System.out.println("V6 Engine started");
}
}
class ElectricEngine implements Engine {
public void start() {
System.out.println("Electric Engine started");
}
}
class Car {
private Engine engine;
// Constructor Injection
public Car(Engine engine) {
this.engine = engine;
}
public void start() {
engine.start();
}
}
// Usage
public class Application {
public static void main(String[] args) {
Engine v6 = new V6Engine();
Car sportsCar = new Car(v6);
sportsCar.start(); // Outputs: V6 Engine started
Engine electric = new ElectricEngine();
Car ecoCar = new Car(electric);
ecoCar.start(); // Outputs: Electric Engine started
}
}
With this architecture, you can easily switch between engine types (V6 or Electric) without needing to alter the Car
class. This results in a cleaner, more maintainable codebase.
Using Design Patterns
Various design patterns, such as the Observer pattern or Strategy pattern, can facilitate loose coupling. These patterns promote interactions between classes without tight dependencies.
For understanding more about these concepts, you may find the Gang of Four Design Patterns beneficial. Each design pattern provides a template for building reusable and decoupled systems.
Closing Remarks
Tight coupling may seem manageable in small projects, but as systems grow, the hidden costs can escalate rapidly. By understanding the implications of tight coupling and adopting strategies like Dependency Injection, using interfaces, and applying design patterns, you create a more modular, maintainable, and testable codebase.
Encouraging loose coupling not only enhances the quality of your code but also leads to faster development cycles and reduced technical debt. Remember, the goal of OOP is to create flexible and reusable components. Keep refactoring and revisiting your designs; they should serve you well into the future.
If you're looking to deepen your understanding of OOP and design principles, check out these fantastic resources:
By adopting best practices and principles like loose coupling, you can create robust applications equipped to handle future demands and challenges. Happy coding!
Checkout our other articles