Preventing XSS Attacks in Java: Key Strategies You Need
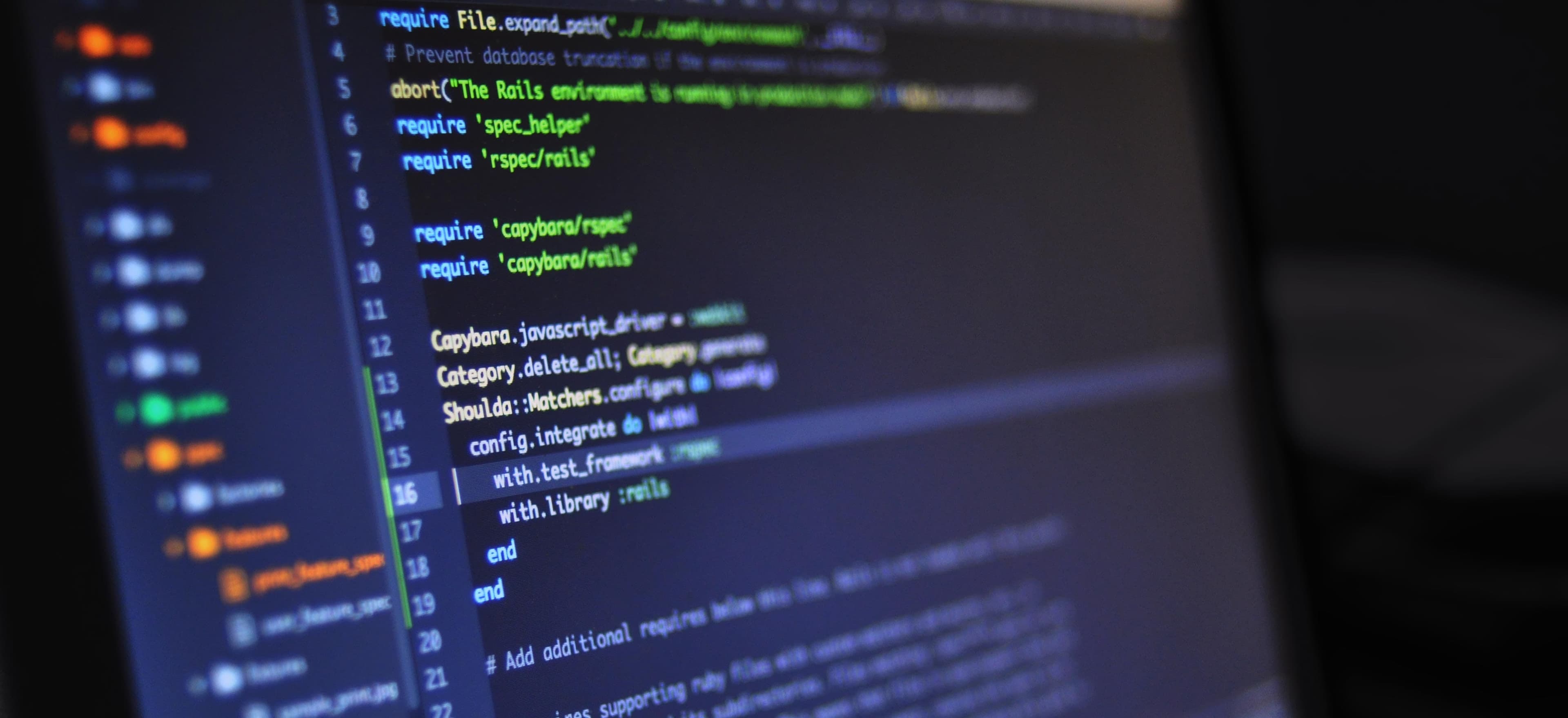
- Published on
Preventing XSS Attacks in Java: Key Strategies You Need
Cross-Site Scripting (XSS) attacks have become one of the most common vulnerabilities in web applications. These attacks allow malicious actors to inject scripts into webpages viewed by other users, potentially compromising sensitive user information or hijacking user sessions. Given the frequency of such attacks, understanding how to prevent them in Java applications is critical for any developer. This blog post explores effective strategies to mitigate XSS vulnerabilities in your Java-based applications.
Understanding XSS Attacks
XSS occurs when an attacker successfully injects malicious scripts into web pages that are delivered to users. There are three main types of XSS attacks:
- Stored XSS: This happens when malicious scripts are stored on a server (e.g., a database) and served to users when they access a webpage.
- Reflected XSS: In this case, the attacker's script is reflected off a web server and executed immediately. This often happens through URL parameters.
- DOM-based XSS: This variant occurs when the client-side script modifies the DOM on the browser, executing malicious code.
To combat these vulnerabilities effectively, let’s explore pivotal strategies you can implement in your Java applications.
Strategy 1: Input Validation and Sanitization
The first line of defense against XSS attacks is proper input validation and sanitization. Always validate data coming from users, ensuring it matches expected formats.
Example of Input Validation
import java.util.regex.Pattern;
public class InputValidator {
private static final Pattern VALID_NAME_PATTERN = Pattern.compile("^[a-zA-Z0-9-_ ]*$");
public boolean isValidName(String name) {
return VALID_NAME_PATTERN.matcher(name).matches();
}
}
In this example, we validate usernames to ensure they only contain alphanumeric characters, hyphens, underscores, and spaces. By using regex, we effectively restrict input and mitigate risks.
Why It's Important
Validation helps prevent unwanted characters from entering your application, making it harder for attackers to inject scripts. Pair this with a sanitization process that involves encoding characters:
import org.apache.commons.text.StringEscapeUtils;
public class Sanitizer {
public String sanitize(String input) {
return StringEscapeUtils.escapeHtml4(input);
}
}
Utilizing libraries like Apache Commons Text enables you to encode potentially harmful characters, transforming <
into <
, for instance. This is crucial before rendering user input on any webpage.
Strategy 2: Contextual Output Encoding
Even when input is validated and sanitized, there's still a need to focus on how data is outputted. Contextual output encoding involves using different encoding strategies based on where the output is being placed.
For HTML output:
response.getWriter().write(StringEscapeUtils.escapeHtml4(userInput));
If outputting in JavaScript:
response.getWriter().write(StringEscapeUtils.escapeEcmaScript(userInput));
Why Context Matters
By using the appropriate escaping method based on the context, you can prevent attackers from executing scripts. Failing to encode data correctly leaves your application open to exploitation.
Strategy 3: Implementing Content Security Policy (CSP)
Content Security Policy (CSP) is a standard designed to prevent XSS. It operates through specific HTTP headers that allow you to control which resources can load on your site.
Example Header Configuration
You can configure CSP in your Java web application like so:
response.setHeader("Content-Security-Policy", "default-src 'self'; script-src 'self' https://trusted.cdn.com");
Why CSP Is Effective
CSP allows you to specify the sources allowed to load JavaScript, images, and other content types. This minimizes the risk of executing non-approved scripts, acting as another layer of security.
Strategy 4: Use Secure Frameworks and Libraries
Java developers often rely on frameworks to build their web applications, which often come with built-in security features to deal with XSS vulnerabilities.
- Spring Security: This framework provides mechanisms to prevent XSS and continually updates to mitigate newly discovered vulnerabilities.
- JSF (JavaServer Faces): JSF automatically escapes HTML output by default.
Why Choosing the Right Framework Matters
Using robust frameworks helps in avoiding common pitfalls as they are curated by a community of security experts. Relying on well-architected libraries saves time and reduces the chance of overlooking key security practices.
Strategy 5: Regular Security Reviews and Testing
No measure is foolproof. Regular security reviews, including code audits and penetration testing, can help you identify and mitigate potential vulnerabilities.
Tools You Might Consider
- OWASP ZAP: This is a popular open-source tool for finding security vulnerabilities in web applications during development and testing.
- Burp Suite: Another comprehensive tool for security testing of web applications.
Why Testing Is Crucial
Performing regular security assessments ensures that your code remains resilient against emerging attack patterns. Outdated libraries, misconfigurations, or newly discovered vulnerabilities can jeopardize the security of your application if neglected.
The Bottom Line
XSS attacks pose a significant threat to Java web applications, but they are preventable with strategic measures. Through effective input validation and sanitization, implementing contextual output encoding, and utilizing CSP, along with secure frameworks, you can significantly reduce the risk of XSS. Moreover, regular security testing ensures that your defenses remain robust as technology evolves.
For further reading on securing your applications, consider exploring OWASP Top Ten and Java Security Best Practices.
By proactively applying these strategies, you will safeguard your Java applications from XSS attacks, contributing to a more secure and trustworthy web environment for your users.