How to Overcome Delays in Sustainable Software Projects
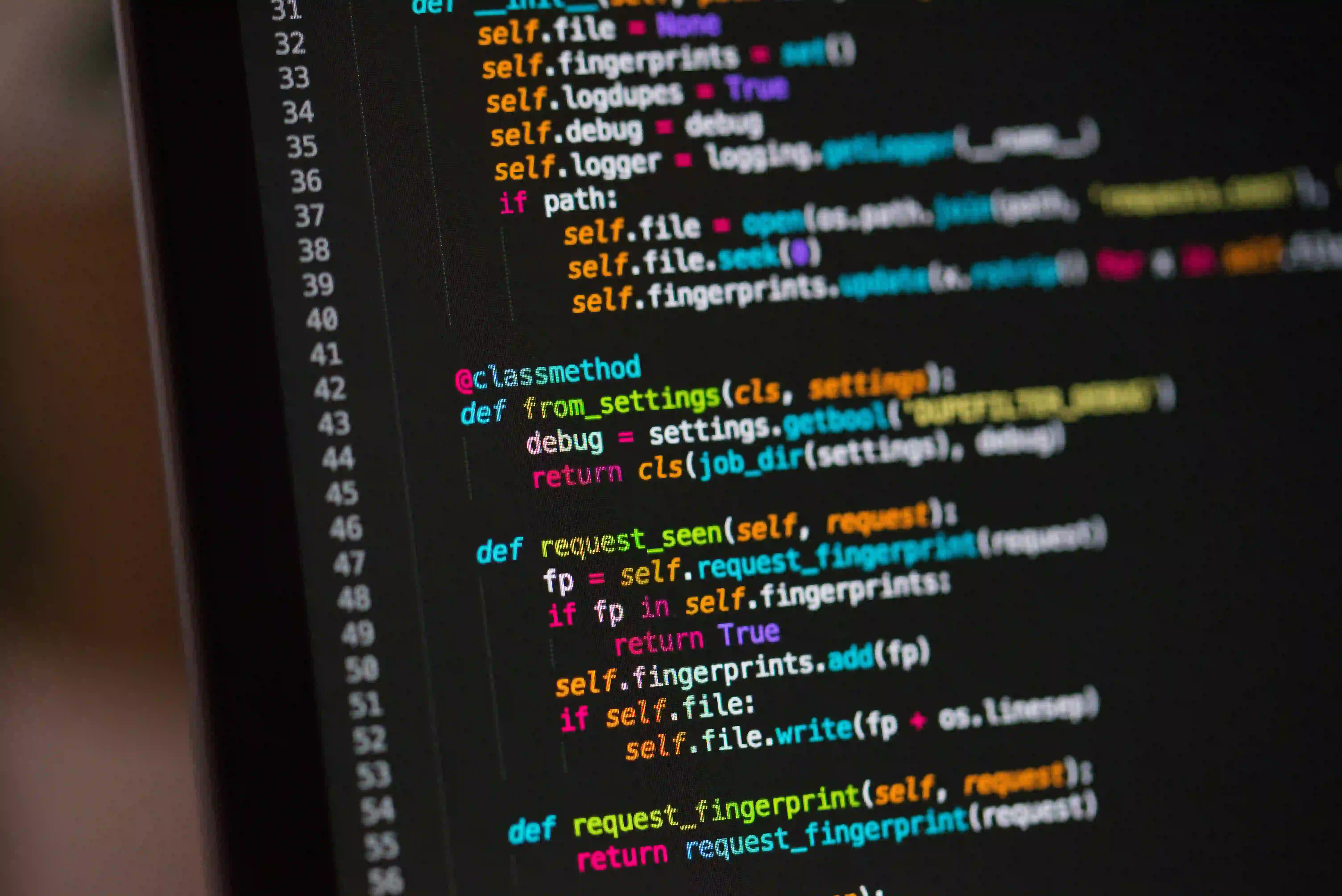
How to Overcome Delays in Sustainable Software Projects
In the ever-evolving landscape of technology, sustainable software development is becoming increasingly vital. However, many teams encounter significant delays in their projects. It's essential to understand the reasons behind these delays and how to overcome them effectively.
In this blog post, we will explore common causes of delays in sustainable software projects and provide actionable strategies to mitigate them. We will also delve into how Java, with its robust ecosystem, can play a pivotal role in enhancing project sustainability.
Understanding the Delays
Delays in software projects can stem from several factors:
- Scope Creep: This occurs when new features are added without proper assessment of their impact on the timeline.
- Technical Debt: Shortcuts taken during earlier phases of development can lead to more significant issues in later stages.
- Ineffective Communication: Poor communication among team members can result in misunderstandings and misaligned goals.
- Team Skill Gaps: Lack of expertise in critical areas can hinder progress.
- Unrealistic Deadlines: Setting impractical timelines is a common pitfall.
Recognizing these factors is the first step toward developing strategies to combat them.
Strategies to Overcome Delays
1. Clearly Define Scope and Objectives
Having a well-defined project scope is crucial. Ensure that all stakeholders agree on the project's objectives. Utilize project management tools like Trello or Jira for better tracking and management.
Example:
Here’s how you can define scope effectively in Java:
public class ProjectScope {
private String feature;
private String description;
private boolean isCompleted;
public ProjectScope(String feature, String description) {
this.feature = feature;
this.description = description;
this.isCompleted = false;
}
public void completeFeature() {
this.isCompleted = true;
System.out.println(feature + " has been completed.");
}
// Getters and Setters
}
Why This Code Matters: This code snippet creates a basic structure to define project scope. Tracking features and their completion status can help mitigate scope creep by visualizing what has been achieved.
2. Prioritize Technical Debt Management
Regularly address technical debt to avoid long-term complications. The practice of code refactoring can significantly enhance maintainability.
Example:
Consider using Java’s updated features to refactor a method:
public class DebtManager {
// Original verbose method
public void manageDebt(List<String> debts) {
for (String debt : debts) {
System.out.println("Managing debt: " + debt);
}
}
// Refactored using streams
public void manageDebtWithStreams(List<String> debts) {
debts.forEach(debt -> System.out.println("Managing debt: " + debt));
}
}
Why This Code Matters: Refactoring old code to utilize Java Streams reduces complexity and enhances performance. Regularly refining code can prevent future issues, promoting a sustainable project environment.
3. Foster Open Communication
Encouraging open lines of communication among team members can reduce misunderstandings. Daily stand-up meetings are an effective strategy for this.
Example:
public class DailyStandup {
private List<TeamMember> members;
public DailyStandup(List<TeamMember> members) {
this.members = members;
}
public void holdMeeting() {
for (TeamMember member : members) {
System.out.println(member.getName() + ": " + member.getStatusUpdate());
}
}
}
Why This Code Matters: This snippet highlights how to manage daily status updates. Consistent communication helps ensure everyone is on the same page and minimizes delays.
4. Upskill the Team
Invest in the continuous improvement of your team. Regular training in Java and software development best practices can bridge the skill gap.
Training Example:
Encourage participation in online courses like Codecademy or Coursera to enhance skills.
5. Set Realistic Timelines
Establish achievable deadlines by understanding the project's demands and team capabilities. Incorporate buffer times to accommodate unexpected delays.
Example:
Utilize Java's Timer
class for scheduling tasks efficiently:
import java.util.Timer;
import java.util.TimerTask;
public class ProjectDeadline {
Timer timer = new Timer();
public void setDeadline(long delay) {
timer.schedule(new TimerTask() {
@Override
public void run() {
System.out.println("Deadline reached! Check project status.");
}
}, delay);
}
}
Why This Code Matters: This code sets reminders for project deadlines. Implementing such practices ensures that your team remains focused and aware of timelines.
Embracing Sustainable Development Practices
Incorporate sustainable development practices into your projects. These practices are not just environmentally friendly; they also encourage efficiency, cost-effectiveness, and resilience.
1. Utilize Agile Methodologies
Agile methodologies, such as Scrum or Kanban, enable teams to adapt quickly to changes and focus on delivering high-quality projects.
2. Continuous Integration/Continuous Deployment (CI/CD)
Implement CI/CD practices to automate and streamline the software delivery process. Tools like Jenkins or GitHub Actions can support this approach.
3. Regular Code Reviews
Conducting regular code reviews ensures quality across the codebase. They help in identifying issues early, reducing the risk of delays later on.
4. Focus on Testing
Invest in automated testing tools. Java offers frameworks like JUnit for unit testing, which can significantly enhance the stability of your software.
Example:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class ProjectScopeTest {
@Test
public void testCompleteFeature() {
ProjectScope scope = new ProjectScope("Feature1", "This is Feature 1");
scope.completeFeature();
assertEquals(true, scope.isCompleted());
}
}
Why This Code Matters: This JUnit test checks if the feature is marked as completed. Proper testing practices can prevent future delays caused by unforeseen bugs.
Closing the Chapter
Overcoming delays in sustainable software projects requires a comprehensive approach that includes clear goal setting, effective communication, and a commitment to reducing technical debt. By leveraging Java's powerful features and tools, teams can enhance their productivity and maintain the quality of their work.
Embracing sustainable practices and continuous improvement is essential. When project teams are aligned, skilled, and equipped with the right tools, not only do they achieve their immediate goals, but they also contribute positively to the broader software development ecosystem.
Understanding and addressing delays means the difference between a successful software project and a failed one. Let us advance towards building sustainable software solutions with efficiency and purpose.
For more insights about sustainable software development, consider reading this comprehensive guide on Agile methodologies.