Trim Your Logs: Java 8 Tips for Size Management
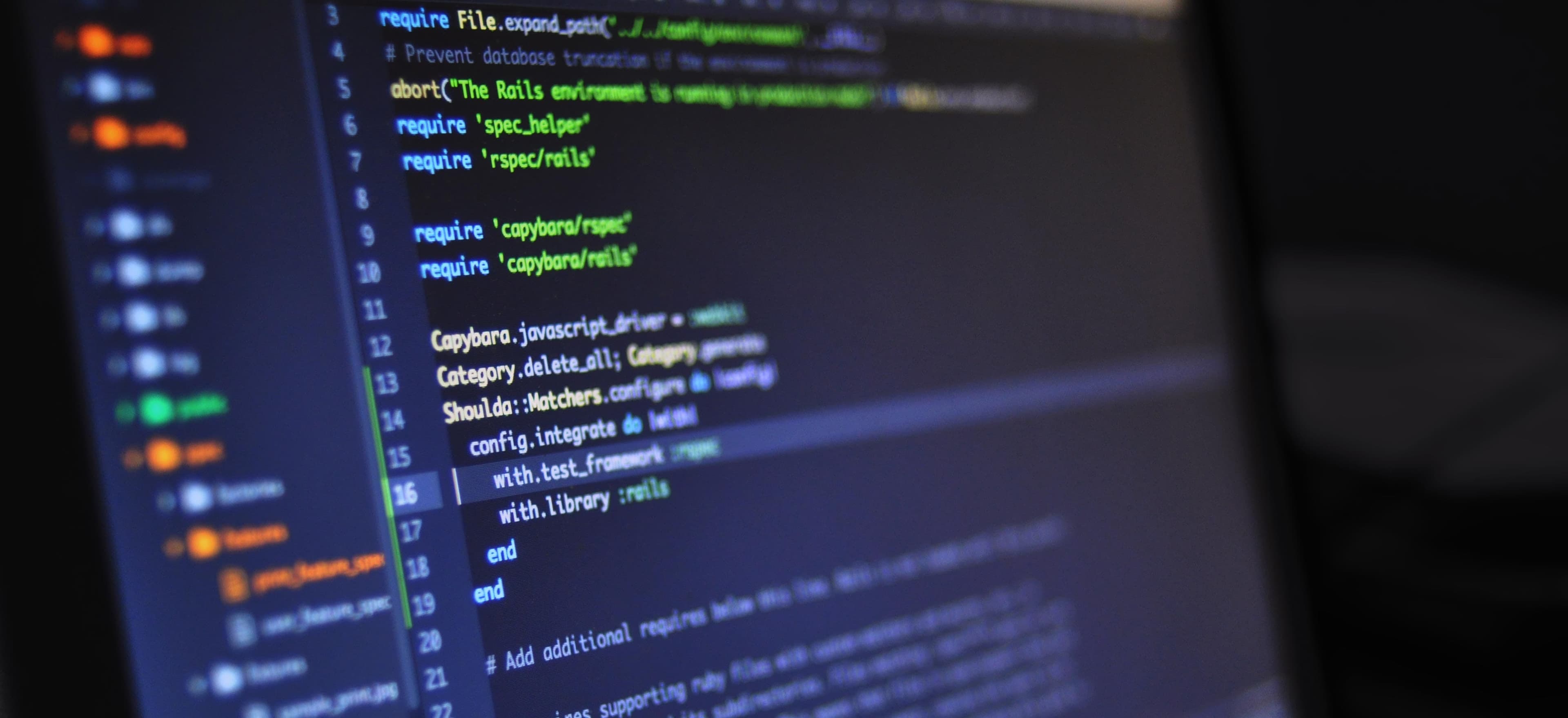
- Published on
Trim Your Logs: Java 8 Tips for Size Management
Logging is a crucial part of any application development process, providing insight into the behavior of the application, aiding debugging, and monitoring performance. However, excessive logging can lead to large log files that consume disk space and potentially degrade application performance. In this blog post, we will explore how to manage log sizes effectively in Java 8 applications, ensuring efficient storage and easy retrieval of logged data.
Understanding Log Management
In the realm of software development, log management refers to the process of overseeing the generation, collection, and storage of log files. While logs provide invaluable information, they can grow rapidly, leading to storage issues and performance bottlenecks. Effective log management involves establishment of strategies to retain meaningful logs while pruning unnecessary data.
Why Java 8?
Java 8 introduced several enhancements, including the Stream API and Lambda expressions, making it easier to handle collections and process data concisely. These features can be leveraged to implement effective logging strategies.
Best Practices for Logging in Java
- Choose the Right Logging Framework
- Log at the Appropriate Level
- Implement Rolling Log Files
- Limit Log Size and Retention Duration
- Utilize Log Filters and Formatters
- Monitor and Audit Logs Regularly
1. Choose the Right Logging Framework
Various logging frameworks are available for Java, including Log4j, Logback, and java.util.logging. Choosing the right framework is crucial. For example, Log4j 2 provides asynchronous logging, which can dramatically improve performance in high-load scenarios. Here's a simple setup for Log4j 2:
<!-- Log4j 2 configuration (log4j2.xml) -->
<Configuration status="WARN">
<Appenders>
<RollingFile name="RollingFile" fileName="app.log" filePattern="app-%d{yyyy-MM-dd}-%i.log.gz">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
<Policies>
<OnStartupTriggeringPolicy/>
<SizeBasedTriggeringPolicy size="10MB"/>
</Policies>
</RollingFile>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="RollingFile"/>
</Root>
</Loggers>
</Configuration>
Why Use Log4j 2? It supports rolling files, which means that when the log file reaches a predefined size, it creates a new one and compresses the old file, thus conserving space.
2. Log at the Appropriate Level
Appropriate logging levels (TRACE, DEBUG, INFO, WARN, ERROR, FATAL) should be enforced. During production, higher levels, such as WARN or ERROR, should be prioritized over DEBUG or TRACE to reduce excessive logging noise.
private static final Logger logger = LogManager.getLogger(YourClass.class);
public void process() {
logger.info("Starting process...");
if (someCondition) {
logger.warn("This might cause issues: {}", someValue);
}
try {
// Your logic here
} catch (Exception e) {
logger.error("Error encountered: {}", e.getMessage());
}
}
Why Control Logging Levels? Logging too much can flood your log files and obscure important messages. Filtering out low-priority logs preserves meaningful data.
3. Implement Rolling Log Files
Rolling log files manage log sizes by creating new files when specified conditions are met (such as time or file size). This allows older log entries to be archived without user intervention.
logback.xml
<configuration>
<appender name="ROLLING" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>myapp.log</file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>myapp.%d{yyyy-MM-dd}.%i.log</fileNamePattern>
<maxHistory>30</maxHistory>
</rollingPolicy>
<encoder>
<pattern>%date %level %logger{10} [%thread] %msg%n</pattern>
</encoder>
</appender>
</configuration>
Why Use Rolling Log Files? This practice mitigates disk space issues while ensuring compliance with storage retention policies.
4. Limit Log Size and Retention Duration
Setting up size limits for logs and specifying retention policies keeps logs concise and manageable. This entails retaining only a fixed number of past log files and deleting older ones.
Here's an example in Log4j 2:
<SizeBasedTriggeringPolicy size="5MB"/>
<DefaultRolloverStrategy max="5"/>
Why Limit Log Size? It prevents a single application from monopolizing disk space, helping maintain operational efficiency.
5. Utilize Log Filters and Formatters
Log filters can exclude certain log messages based on various parameters (e.g., log level, logger name) and formatters help remain consistent in the logged output.
logger.debug("Debugging information here...");
logger.info("Important process information.");
logger.warn("Warning message that should catch attention.");
logger.error("An error has occurred.", throwable);
Why Apply Filters and Formatters? Consistent formatting and filtering can simplify log analysis later. Easily interpretable logs can expedite troubleshooting.
6. Monitor and Audit Logs Regularly
Regularly auditing logs for anomalies or patterns can provide insights into potential application issues or security risks. Tools like ELK Stack (Elasticsearch, Logstash, Kibana) can help analyze logs effectively.
Why Monitor Logs? Continuous monitoring helps to quickly identify and address issues, improving overall system health.
Using Java 8 Features for Log Management
Java 8 introduced lambdas and streams, which can enhance the efficiency of data handling, including logging.
Example: Filtering Logs with Streams
Imagine you have a list of log entries and you want to determine which logs deserve attention.
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class LogFilter {
public static void main(String[] args) {
List<String> logs = Arrays.asList(
"INFO: User login successful",
"ERROR: Unable to connect to database",
"WARN: Low disk space",
"DEBUG: User action tracked"
);
List<String> urgentLogs = logs.stream()
.filter(log -> log.startsWith("ERROR") || log.startsWith("WARN"))
.collect(Collectors.toList());
urgentLogs.forEach(System.out::println);
}
}
Why Use Streams for Log Management? Streams offer a concise way to filter and process log statements, making management operations more efficient.
Bringing It All Together
Effective log management is a foundational aspect of successful Java application development. By leveraging features of Java 8 alongside best practices, you can keep log sizes manageable while retaining the ability to monitor application behavior effectively.
Taking the time to implement a robust logging strategy today can save you from headaches downstream. Regular audits, combined with the right frameworks and practices, ensure you have meaningful data to aid in your decision-making.
For further reading on advanced logging techniques, consider checking out Log4j 2 Documentation or Logging Best Practices.
Happy logging!
Checkout our other articles