Struggling to Get Git Commit Data in JSON Format?
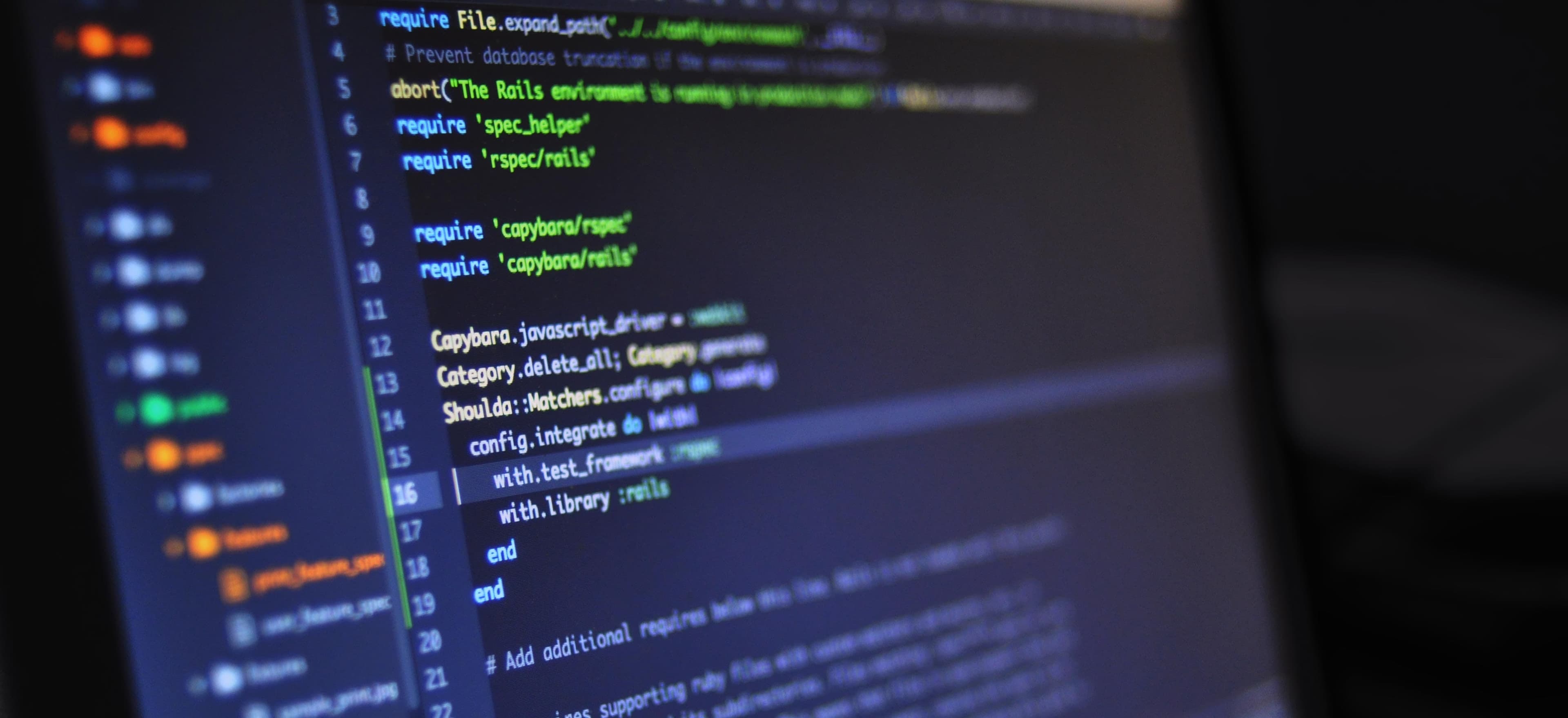
- Published on
Struggling to Get Git Commit Data in JSON Format?
If you've ever needed to access Git commit data programmatically, you may have found it challenging to extract and format that information in JSON. Whether you're building an application that requires commit histories, generating reports, or simply visualizing changes in your codebase, having that data in JSON format can be a game-changer. In this post, we'll explore how to retrieve Git commit data and format it into JSON effectively.
Why Convert Git Commit Data to JSON?
-
Interoperability: JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. This makes it an excellent choice for APIs and data interchange between systems.
-
Ease of Use: Most programming languages have robust libraries for handling JSON, allowing for easier integration and manipulation of data.
-
Structured Data: JSON allows you to structure your commit data in nested objects, which can provide significant context and clarity.
Understanding Git Commit Data
Before diving into the code, it's essential to understand the structure of Git commit data. Each commit in Git typically contains:
- Commit Hash
- Author Name
- Author Email
- Date
- Commit Message
This information is not only vital for tracking changes but, when formatted in JSON, can provide a structured way to interact with the data programmatically.
Extracting Git Commit Data
Using Git Command-Line
If you want to fetch the commit data, you can use the Git command-line interface. Here’s a basic command to get all commit data:
git log --pretty=format:'{"hash": "%H", "author_name": "%an", "author_email": "%ae", "date": "%ad", "message": "%s"}' --date=iso
The Breakdown:
git log
: This command retrieves the commit history.--pretty=format:
: This flag lets us customize output formatting.- JSON Strings: We format each commit detail into JSON key-value pairs.
--date=iso
: Formats the date to become ISO 8601 compliant.
However, this command will output each commit on a new line, and it won't provide a proper JSON array format.
Providing JSON Array Format
To generate a complete JSON array, you can run the following command:
git log --pretty=format:'{"hash": "%H", "author_name": "%an", "author_email": "%ae", "date": "%ad", "message": "%s"}' --date=iso | sed '1s/^/[/' | sed '$s/$/]/'
Explanation:
- The first
sed
command adds an opening bracket[
at the beginning of the output. - The second
sed
command appends a closing bracket]
at the end.
These adjustments ensure you are working with a valid JSON array.
Parsing Git Commit Data with Java
Now that we have a basic understanding of how to extract commit data into JSON format from the Git command line, let's delve into making this more useful by parsing it in a Java application.
Sample Java Code
Below is a Java example that executes the Git command to get commit data, reads the output, and formats it into a JSON structure.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONObject;
public class GitCommitParser {
public static void main(String[] args) {
try {
// Execute Git command
ProcessBuilder processBuilder = new ProcessBuilder("git", "log", "--pretty=format:'{\"hash\": \"%H\", \"author_name\": \"%an\", \"author_email\": \"%ae\", \"date\": \"%ad\", \"message\": \"%s\"}'", "--date=iso");
Process process = processBuilder.start();
// Read command output
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
List<JSONObject> commits = new ArrayList<>();
String line;
while ((line = reader.readLine()) != null) {
// Remove quotations from the start and end
line = line.replaceFirst("^\'", "").replaceFirst("\'$", "");
JSONObject commit = new JSONObject(line);
commits.add(commit);
}
// Create JSON array from the commit list
JSONArray commitArray = new JSONArray(commits);
System.out.println(commitArray.toString(2)); // Pretty print with an indent of 2
} catch (Exception e) {
e.printStackTrace();
}
}
}
The Breakdown:
ProcessBuilder
: This Java class is used to create operating system processes. We execute our Git command here.BufferedReader
: We use this to read the output of our Git command line-by-line.JSONObject
andJSONArray
: Provided by theorg.json
library, these classes help construct and manipulate JSON objects and arrays.
Importing the JSON Library
Make sure to add the following Maven dependency in your pom.xml
if you're using Maven:
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20210307</version>
</dependency>
Final Thoughts
We hope this guide has shed light on how to retrieve and format Git commit data into JSON format. Leveraging both the Git CLI and Java's JSON manipulation capabilities opens new avenues to effectively manage and analyze commit histories.
Whether you're streamlining development workflows or enabling data-driven insights into your repositories, the power of JSON cannot be overstated.
For further reading on Java JSON libraries, you can explore the Maven Repository for various available options or visit the official Git documentation for deeper insights.
Happy coding!