Common Pitfalls in Spring 3.1 Cache Implementation
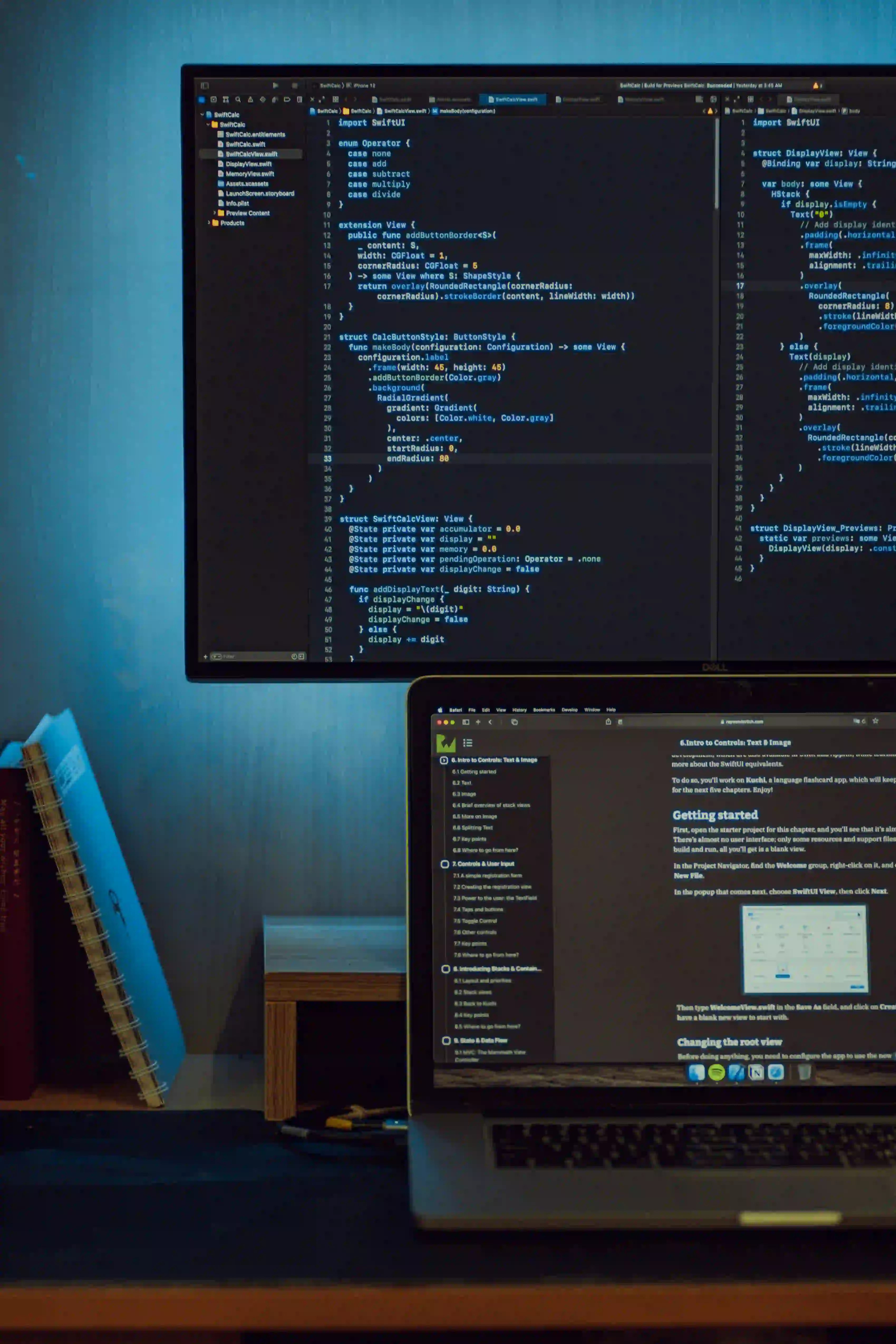
Common Pitfalls in Spring 3.1 Cache Implementation
Spring Framework has made caching easier with its built-in caching abstractions. However, as with any technology, developers can run into common pitfalls, particularly when using advanced features like caching in Spring 3.1. In this blog, we will explore those pitfalls, discuss how to avoid them, and provide you with code snippets illustrating best practices.
Understanding Spring Cache Abstraction
Spring's caching abstraction allows you to easily cache method results. By doing so, you can significantly enhance the performance of your applications by reducing unnecessary computations and database calls. Before jumping into the common pitfalls, let’s take a look at how to set up caching in Spring 3.1.
Basic Setup of Spring Caching
To utilize Spring's caching feature, you need to first enable caching in your configuration class. Here's a simple example:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.cache.CacheManager;
import org.springframework.cache.concurrent.ConcurrentMapCacheManager;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("items");
}
}
In this example, we use a ConcurrentMapCacheManager
to manage our cache. The @EnableCaching
annotation is critical as it enables the cache support in your Spring application.
Common Pitfalls
Let's dive into the common pitfalls developers face when implementing caching in Spring 3.1.
1. Ignoring Cache Eviction Strategies
One major oversight is neglecting to implement cache eviction strategies. When the underlying data changes, the cached data may become stale. Without proper eviction, users may receive outdated information.
Solution
Make use of eviction annotations like @CacheEvict
. Here’s an example:
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.stereotype.Service;
@Service
public class ItemService {
@CacheEvict(value = "items", allEntries = true)
public void updateItem(Item item) {
// Update the item in the database
}
}
In this snippet, the @CacheEvict
annotation is utilized to clear all entries whenever an item is updated. This effectively prevents stale data from being served.
2. Overusing Caching
Caching can be performance-enhancing but overusing it can lead to increased memory consumption and inefficient memory management. Developers may be tempted to cache everything, but it's essential to choose wisely.
Solution
Cache only what is necessary. Consider the nature of the data:
- Data that is expensive to compute should be cached.
- Frequently accessed data.
- Data that does not change often.
This focused approach helps in resource optimization.
3. Not Considering Thread Safety
Concurrency issues may arise if cache management is not handled thread-safely. This is especially important in multi-threaded environments.
Solution
Ensure that you utilize thread-safe cache managers like ConcurrentMapCacheManager
, as shown earlier. If necessary, consider using distributed cache solutions like Redis or Hazelcast.
4. Misconfiguration of Cache Names
A common pitfall is misconfiguring cache names. If the cache names specified in the annotations do not match the cache names in the CacheManager
, caching simply won't work.
Solution
Double-check cache names in both your configuration and annotations. Consistency is key:
@Cacheable(value = "items")
public Item getItemById(String id) {
// method to fetch item from the database
}
Here, "items" should match the cache we've defined in the CacheConfig
.
5. Ignoring Cache Hit Ratio
Developers often overlook monitoring cache performance. If the cache hit ratio is low, the cache implementation may not be effective.
Solution
Use tools like Spring Actuator to gain insights into cache statistics. Set up monitoring and logging to analyze cache performance regularly.
Example with Spring Actuator:
management:
endpoints:
web:
exposure:
include: '*'
6. Not Using Spring's Conditional Caching
Another powerful feature of Spring's caching abstraction is the ability to conditionally cache results using the condition
attribute. However, not utilizing this can lead to unnecessary cache entries.
Solution
Make use of the condition
attribute in caching annotations:
@Cacheable(value = "items", condition = "#id > 0")
public Item getItemById(Long id) {
// fetch from database
}
Here, the cache entry will only be created if the ID is greater than zero, thereby reducing unnecessary cache usage.
7. Failing to Handle Serialization
In distributed caching scenarios, data needs to be serialized. Failing to consider object serialization can lead to runtime exceptions.
Solution
Ensure that all cached objects implement Serializable
. Here's an example:
public class Item implements Serializable {
private static final long serialVersionUID = 1L;
private String id;
private String name;
// getters and setters
}
This allows Java serialization to correctly handle the object when caching.
The Bottom Line
Implementing caching in Spring 3.1 can greatly improve the performance of your applications if done correctly. However, pitfalls such as ignoring eviction strategies, misconfigurations, and improper handling of concurrency can hinder its effectiveness. By adhering to best practices such as monitoring cache usage, using thread-safe managers, and selectively caching data, you can leverage Spring's caching capabilities effectively.
To familiarize yourself with Spring's caching abstraction, you can refer to the Spring Documentation on Caching. By being aware of these common pitfalls and addressing them, you will be better equipped to implement caching in your applications efficiently.
Happy coding!