Improving Performance by Optimizing Memory Access Patterns
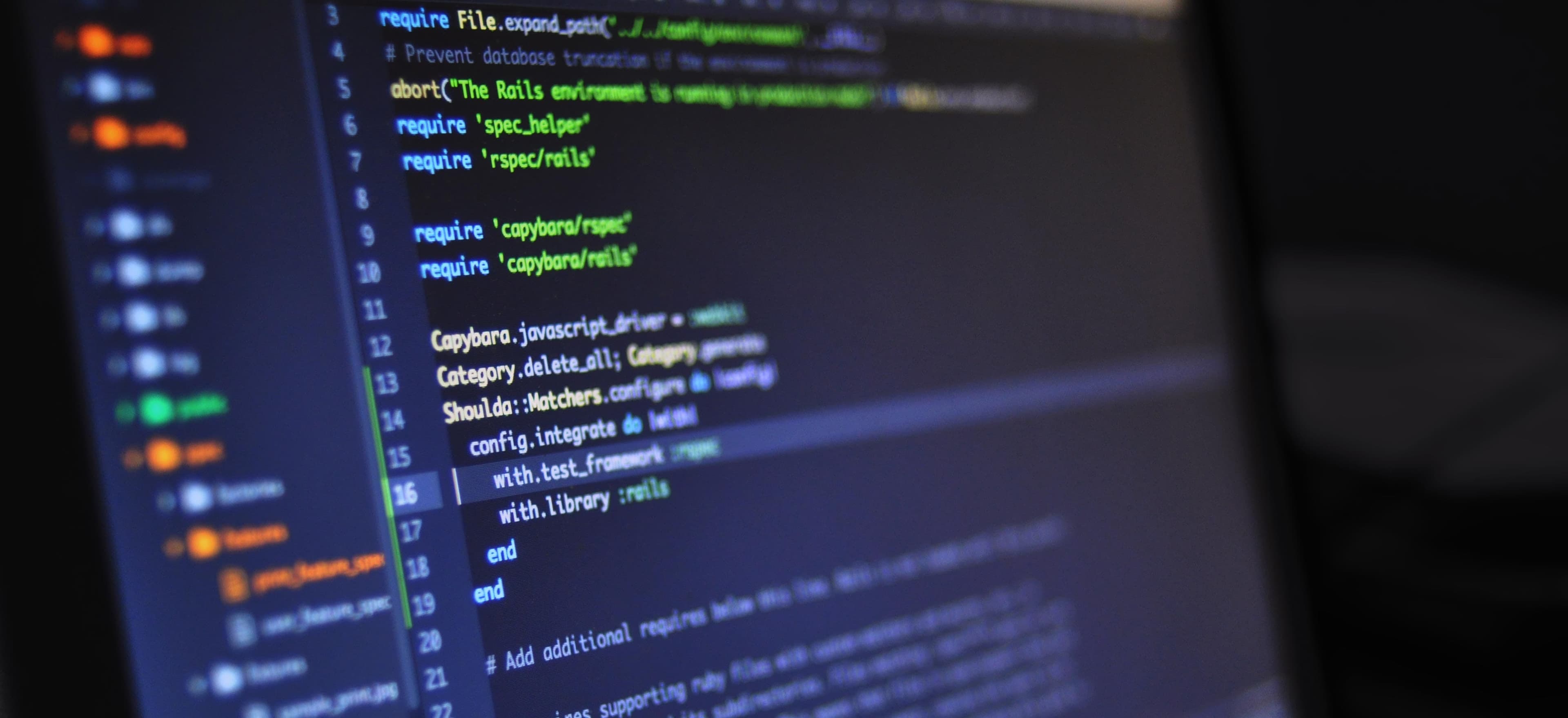
- Published on
Improving Performance by Optimizing Memory Access Patterns in Java
In the realm of programming, particularly in Java, optimizing performance is a key focus for developers looking to create fast and efficient applications. One pivotal area that can significantly influence overall performance is memory access patterns. In this blog post, we will explore how optimizing memory access can enhance performance and provide practical examples that illustrate this concept.
Understanding Memory Access Patterns
Memory access patterns refer to the way a program reads from and writes to memory. A memory access pattern can be sequential, where subsequent memory locations are accessed in order, or random, involving accesses made to arbitrary memory locations. Sequential access is generally faster than random access, due to how modern computer architectures, including CPU caches, work.
Importance of Cache Awareness
Modern CPUs utilize a hierarchy of cache levels (L1, L2, L3) to reduce the time it takes to access data. When we access data that is not in the cache, a cache miss occurs, leading to significantly slower performance. Thus, it is essential to write code that utilizes memory efficiently and avoids unnecessary cache misses.
Keys to Optimizing Memory Access Patterns in Java
1. Use Arrays for Continuous Memory
Java arrays provide a contiguous block of memory for storing data. This layout allows for better cache locality compared to collections like ArrayList
, which may cause additional overhead during operations.
int[] data = new int[1000000];
for (int i = 0; i < data.length; i++) {
data[i] = i;
}
In the example above, all integers are stored sequentially in memory, making it cache-friendly. This contrast starkly with instances where we rely on ArrayList
, as internal resizing and pointers could lead to scattered data access.
2. Prefer Primitive Types
Using primitive types like int
, float
, or char
can effectively reduce memory footprint and improve performance, as they do not involve additional reference overhead from object wrappers.
Integer[] numbers = new Integer[100]; // Boxed Integer
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i; // Wrapping incurs overhead
}
In the example above, Integer
objects require additional memory for the object header. Instead, after switching to primitive arrays:
int[] numbers = new int[100]; // Primitive int
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i; // Less overhead
}
You can see that the use of primitive types results in better memory efficiency and access speed.
3. Optimize Data Structures
Choosing the right data structure plays a crucial role in memory access performance. For instance, try to use homogeneous structures when possible, as they aid in better locality.
class Employee {
int id;
String name;
}
// Using a list of employees
List<Employee> employees = new ArrayList<>();
for (int i = 0; i < 100; i++) {
employees.add(new Employee(i, "Employee" + i));
}
In the above example, if we had retained a list with varied types or types that store additional metadata, accessing each element may introduce unwanted overhead. A potential improvement would involve using an array of objects or simply primitives when feasible.
4. Access Patterns: Sequential vs. Random
When looping over data, always aim for sequential access rather than random access. For instance, consider these two access patterns:
// Inefficient Random Access
for (int i = 0; i < 100; i++) {
int randomIndex = (int) (Math.random() * 100);
System.out.println(data[randomIndex]);
}
// Efficient Sequential Access
for (int i = 0; i < 100; i++) {
System.out.println(data[i]);
}
The second example demonstrates a linear traverse of the array, which leads to better utilization of the CPU cache compared to randomly accessing the elements.
5. Efficient Looping Techniques
Additionally, when dealing with large datasets, consider using advanced looping techniques. For example, instead of traditional for-loops:
// Traditional For Loop
int sum = 0;
for (int i = 0; i < data.length; i++) {
sum += data[i];
}
// Enhanced For Loop
sum = 0;
for (int value : data) {
sum += value;
}
The enhanced for loop is usually more readable and can optimize performance in certain JVM architectures based on how the loops are compiled and executed.
Lessons Learned
Optimizing memory access patterns in Java can yield substantial performance improvements. It requires thoughtful consideration about how data is stored, accessed, and manipulated. By restructuring code to favor cache-friendly patterns, choosing the right data structures, and utilizing primitives where possible, developers can ensure that applications run efficiently.
For further exploration of optimizing performance in Java, I recommend diving into resources like Java Performance: The Definitive Guide for deeper insights.
Final Thoughts
As you build your Java applications, always keep memory optimization in the back of your mind. Understanding and improving memory access patterns can lead to distinct benefits, ensuring that your applications not only work correctly but also run at their optimal performance. Happy coding!