Decoding Design Patterns: Why Developers Struggle to Apply Them
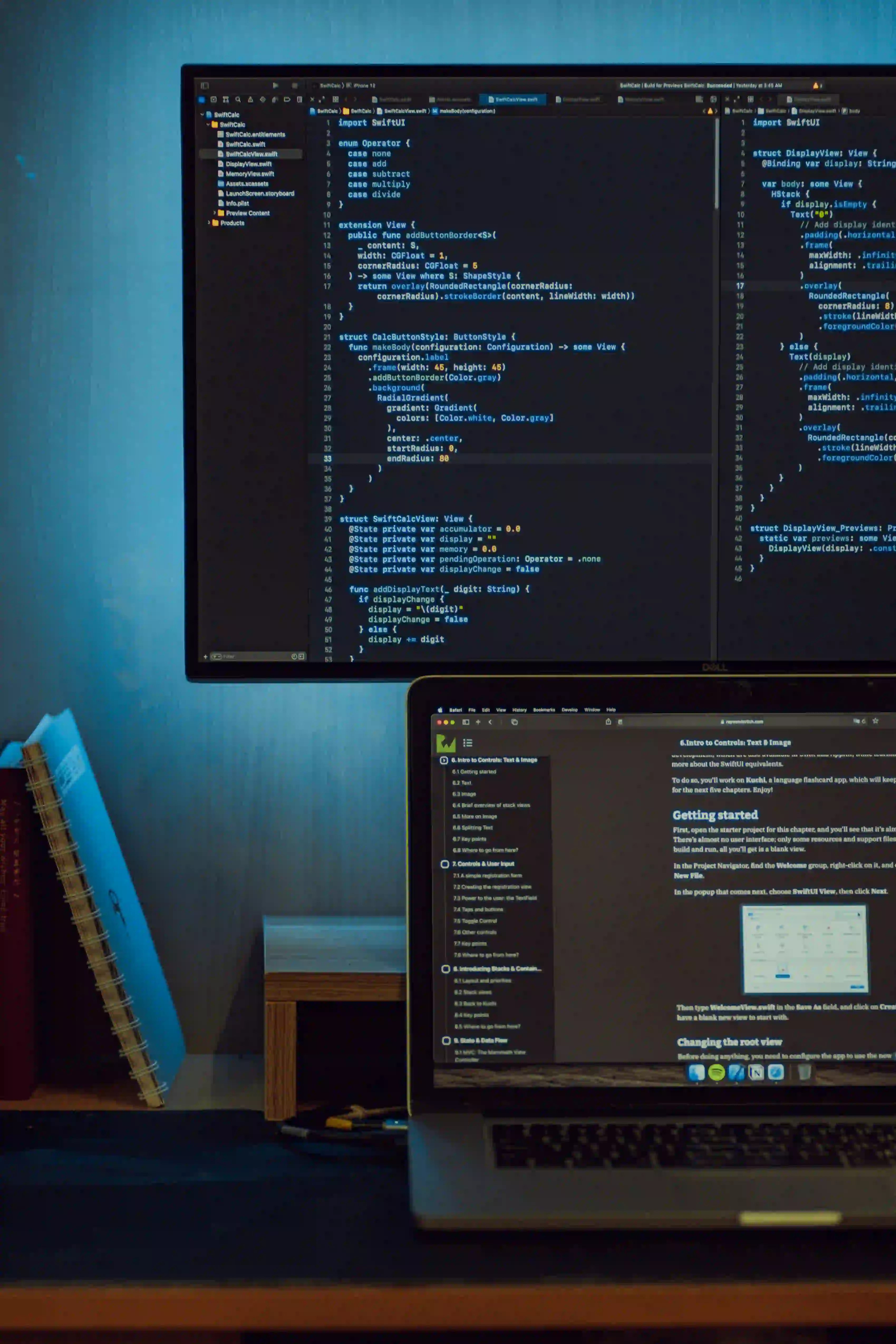
Decoding Design Patterns: Why Developers Struggle to Apply Them
Design patterns are powerful tools in software development. They solve common problems, promote code reuse, and enhance maintainability. However, many developers face difficulties when attempting to implement these patterns. In this post, we will explore why developers struggle with applying design patterns and provide insight into how to overcome these challenges.
Understanding Design Patterns
A design pattern is a reusable solution to a commonly occurring problem in software design. These patterns are categorized into three main types:
- Creational Patterns: Focus on object creation mechanisms. Examples include Singleton, Factory Method, and Abstract Factory.
- Structural Patterns: Deal with object composition and simplify relationships. Examples include Adapter, Composite, and Decorator.
- Behavioral Patterns: Emphasize communication between objects. Examples include Observer, Strategy, and Command.
Understanding these classifications is imperative for recognizing when and how to use design patterns effectively.
Why Developers Struggle with Design Patterns
1. Complexity
Design patterns can often appear complex, especially for beginners. For instance, consider the Decorator Pattern:
// Component interface
public interface Coffee {
double cost();
}
// Concrete component
public class SimpleCoffee implements Coffee {
public double cost() {
return 2.0;
}
}
// Decorator abstract class
public abstract class CoffeeDecorator implements Coffee {
protected Coffee coffee;
public CoffeeDecorator(Coffee coffee) {
this.coffee = coffee;
}
public abstract double cost();
}
// Concrete decorator
public class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public double cost() {
return coffee.cost() + 0.5;
}
}
In the above example, the decorator pattern adds functionality (milk) to a simple coffee object without altering its structure. The layers of abstraction can overwhelm new developers, making it challenging to grasp its importance and utility.
2. Overengineering
Developers often misapply design patterns by overengineering solutions. The elegant simplicity of patterns is diluted when they're applied unnecessarily. For instance, adding a complex pattern for a simple task:
// A simple object that doesn't need the complexity of a Builder pattern
public class SimpleUser {
private String name;
// Constructor, getters, and setters omitted for brevity
}
// Using a Builder pattern unnecessarily
public class UserBuilder {
private String name;
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public SimpleUser build() {
return new SimpleUser(this.name);
}
}
Using the Builder pattern when creating a user object is excessive, particularly if there are no complex construction operations. This results in code bloat, making the codebase harder to maintain.
3. Lack of Practical Experience
Theoretical knowledge of design patterns is not sufficient for successful implementation. Developers may read about a pattern and understand its mechanics yet fail to recognize when a pattern is necessary in a real-world scenario.
Developing practical experience can demystify the application of design patterns.
4. Misunderstanding the Intent
The real power of design patterns lies in understanding their intent. It’s crucial to grasp what problem a pattern is solving, rather than merely replicating code snippets.
For instance, the Observer Pattern is intended for situations where an object's state changes and other dependent objects need to be notified. Here’s a simple implementation:
// Observer interface
public interface Observer {
void update(String message);
}
// Concrete observer
public class EmailSubscriber implements Observer {
private String email;
public EmailSubscriber(String email) {
this.email = email;
}
@Override
public void update(String message) {
System.out.println("Email sent to " + email + ": " + message);
}
}
// Subject class
import java.util.ArrayList;
import java.util.List;
public class EmailService {
private List<Observer> subscribers = new ArrayList<>();
public void subscribe(Observer observer) {
subscribers.add(observer);
}
public void notifySubscribers(String message) {
for (Observer subscriber : subscribers) {
subscriber.update(message);
}
}
}
In this example, the EmailService
maintains a list of subscribers and notifies them whenever there's a new message. The key takeaway here is understanding that the purpose of this pattern is to separate the object that changes (subject) from objects that listen to changes (observers).
How to Effectively Apply Design Patterns
1. Start Small
Begin with small projects, where you can incorporate design patterns naturally. Refactoring existing code to use patterns can also deepen your understanding without overwhelming you.
2. Focus on the Problem
Always keep the problem at the forefront. Ask yourself: "Does this pattern solve a specific problem?" If it doesn’t, you may want to reconsider its use.
3. Learn from Examples
Studying real-world implementations and case studies can illuminate the practical use of design patterns. Resources include:
4. Collaborate with Peers
Discussing design patterns with peers or mentors can lead to rich insights. Group coding sessions can also encourage the practice of applying patterns correctly.
5. Keep Improving Your Skills
Design patterns are not static. Continuous learning and practice lead to mastery. Keep yourself updated with patterns relevant to modern programming practices.
Wrapping Up
Design patterns are invaluable for effective software design. Despite the common struggles of complexity, misapplication, and theoretical knowledge gaps, understanding the underlying problems they solve can bridge the gaps in implementation.
By starting small, focusing on specific problems, learning from others, collaborating, and maintaining a growth mindset, developers can become adept at effectively applying design patterns.
Always remember: It’s not about how many patterns you know, but about knowing when and how to apply them effectively in your codebase.
By following these principles, developers can navigate the landscape of design patterns with confidence, leading to cleaner, more maintainable, and readable code.
Further Reading
Make sure to experiment and explore this vast topic in more depth as you continue your programming journey!