Mastering Percentile Calculation in Java: Common Pitfalls
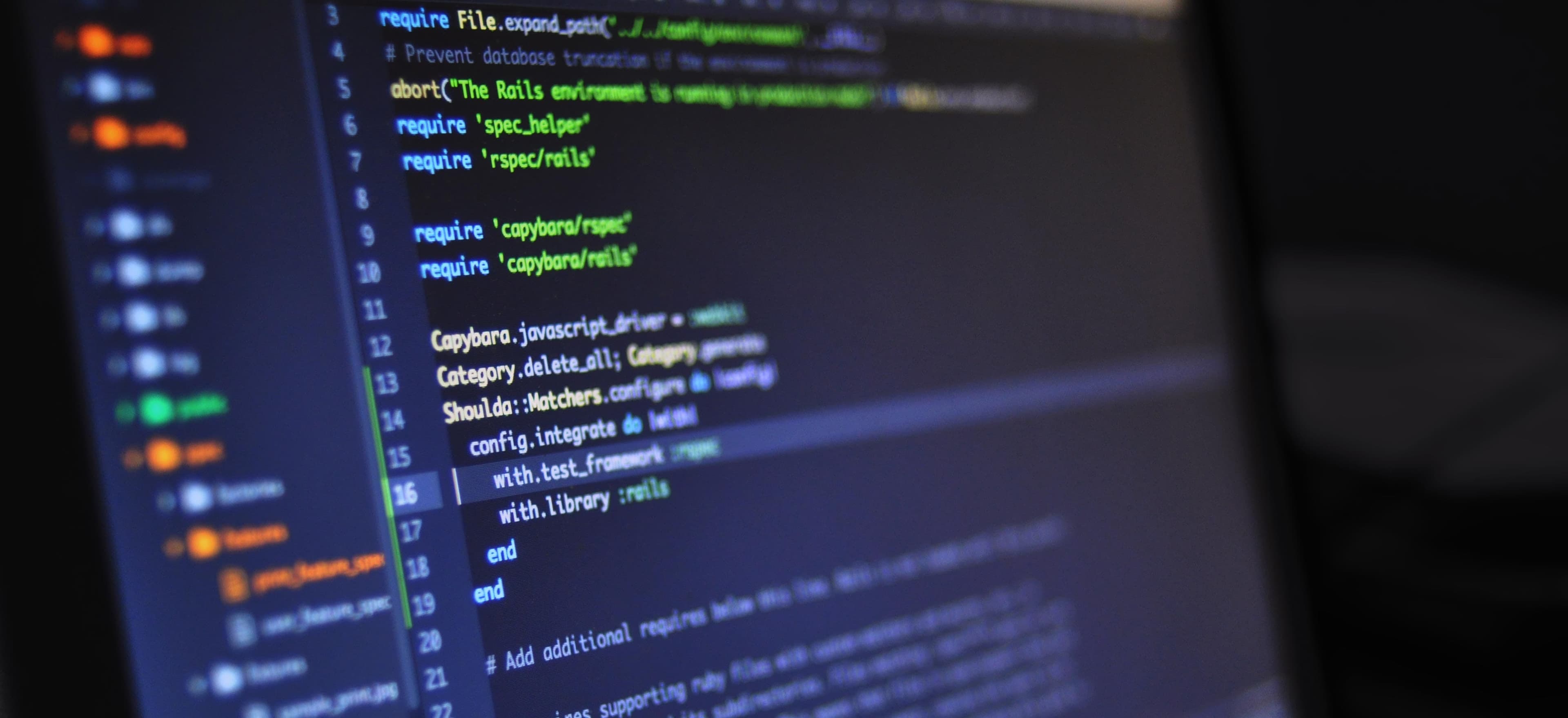
- Published on
Mastering Percentile Calculation in Java: Common Pitfalls
Calculating percentiles can be a foundational skill in data analysis, statistics, and various applications of machine learning. In Java, incorrect implementations can lead to misleading results. This blog post will guide you through the common pitfalls faced while calculating percentiles in Java and help you master the process through effective coding practices.
What Are Percentiles?
Percentiles are numerical values that divide a dataset into 100 equal parts. For instance, the 50th percentile (median) separates the lowest 50% of data from the highest 50%. Understanding percentiles is essential for interpreting large datasets, especially in fields such as data science, finance, and research.
The Importance of Proper Percentile Calculation
Before diving into the code, let's clarify why accurate percentile calculations matter:
- Decision-Making: Percentiles often inform business and policy decisions.
- Data Analysis: They provide insights into data distributions.
- Anomaly Detection: Identifying outliers can improve data integrity.
Common Pitfalls in Percentile Calculation
- Wrong Formula Implementation
- Data Sorting Inaccuracies
- Off-by-One Errors in Array Indices
- Using Integer Division
- Not Handling Edge Cases
Understanding these pitfalls will enhance your programming skill set and avoid errors in your implementations.
How to Calculate Percentiles
Before showcasing the code, let's establish the basic formula:
Given a sorted dataset of size N
and a desired percentile P
:
-
Calculate the rank R:
R = P/100 * (N + 1) -
If R is an integer, the Pth percentile is the value at the Rth position.
-
If R is not an integer, round R down to the nearest whole number
k
and find the value at this position. Letf
be the fractional part (R - k). Then, the Pth percentile is calculated as:
Percentile = X[k] + (f * (X[k+1] - X[k]))
Implementing Percentile Calculation in Java
Now let’s implement percentile calculations in Java while keeping potential pitfalls in mind.
import java.util.Arrays;
public class PercentileCalculator {
// Method to calculate percentile
public static double calculatePercentile(double[] data, double percentile) {
// Step 1: Sort the data
Arrays.sort(data);
// Step 2: Calculate the rank
int N = data.length;
double rank = (percentile / 100.0) * (N + 1);
// Step 3: Handle edge cases
if (rank < 1 || rank > N) {
throw new IllegalArgumentException("Percentile must be between 0 and 100.");
}
// Step 4: Use floor and ceil for calculations
int lowerIndex = (int) Math.floor(rank) - 1;
int upperIndex = (int) Math.ceil(rank) - 1;
// If rank is an exact integer
if (lowerIndex == upperIndex) {
return data[lowerIndex];
}
// If rank is not an exact integer
double weight = rank - lowerIndex - 1;
return data[lowerIndex] + weight * (data[upperIndex] - data[lowerIndex]);
}
public static void main(String[] args) {
double[] data = {3.5, 2.1, 8.6, 4.9, 5.0, 7.0, 1.2, 6.5};
double percentileToCalculate = 50; // Median
double result = calculatePercentile(data, percentileToCalculate);
System.out.println("The " + percentileToCalculate + "th percentile is: " + result);
}
}
Commentary on the Code:
- Data Sorting: Sorting is critical as percentiles depend on order. The
Arrays.sort(data);
line ensures data is in ascending order. - Rank Calculation: We calculate the rank using
double rank = (percentile / 100.0) * (N + 1);
. This line maintains precision. - Index Handling: The method checks edge cases like
rank < 1
orrank > N
, throwing anIllegalArgumentException
for invalid percentiles. - Proper Indexing: Indices in Java start at zero, creating a common pitfall if handled incorrectly; thus,
lowerIndex
andupperIndex
help navigate this. - Weighting Values: The weighting process allows for accurate interpolation between two values when the rank doesn’t map directly to an integer position.
Testing the Functionality
Always validate your calculations with assertions or sample datasets. Here’s how you can conduct simple tests:
public static void runTests() {
double[] data1 = {10, 20, 30, 40, 50};
assert calculatePercentile(data1, 50) == 30 : "Test Case 1 Failed";
double[] data2 = {1, 3, 4, 6, 7, 8, 9};
assert calculatePercentile(data2, 25) == 4 : "Test Case 2 Failed";
System.out.println("All test cases passed successfully.");
}
public static void main(String[] args) {
runTests();
}
The Closing Argument
Mastering percentile calculations is a crucial skill for any Java developer involved in data analysis or statistics. By avoiding common pitfalls such as incorrect formulas, indexing errors, and failing to handle edge cases, you can ensure your percentile calculations yield accurate and valuable insights.
Further Reading
To deepen your understanding, you may find value in exploring the following topics:
- Java Arrays Documentation
- Java Math Class
- Statistical Analysis in Data Science
Feel free to share your experiences or concerns regarding percentile calculations in Java in the comments below! Your insights can help others in their journey to mastering data analysis.
Checkout our other articles