Common Docker Container Mistakes and How to Avoid Them
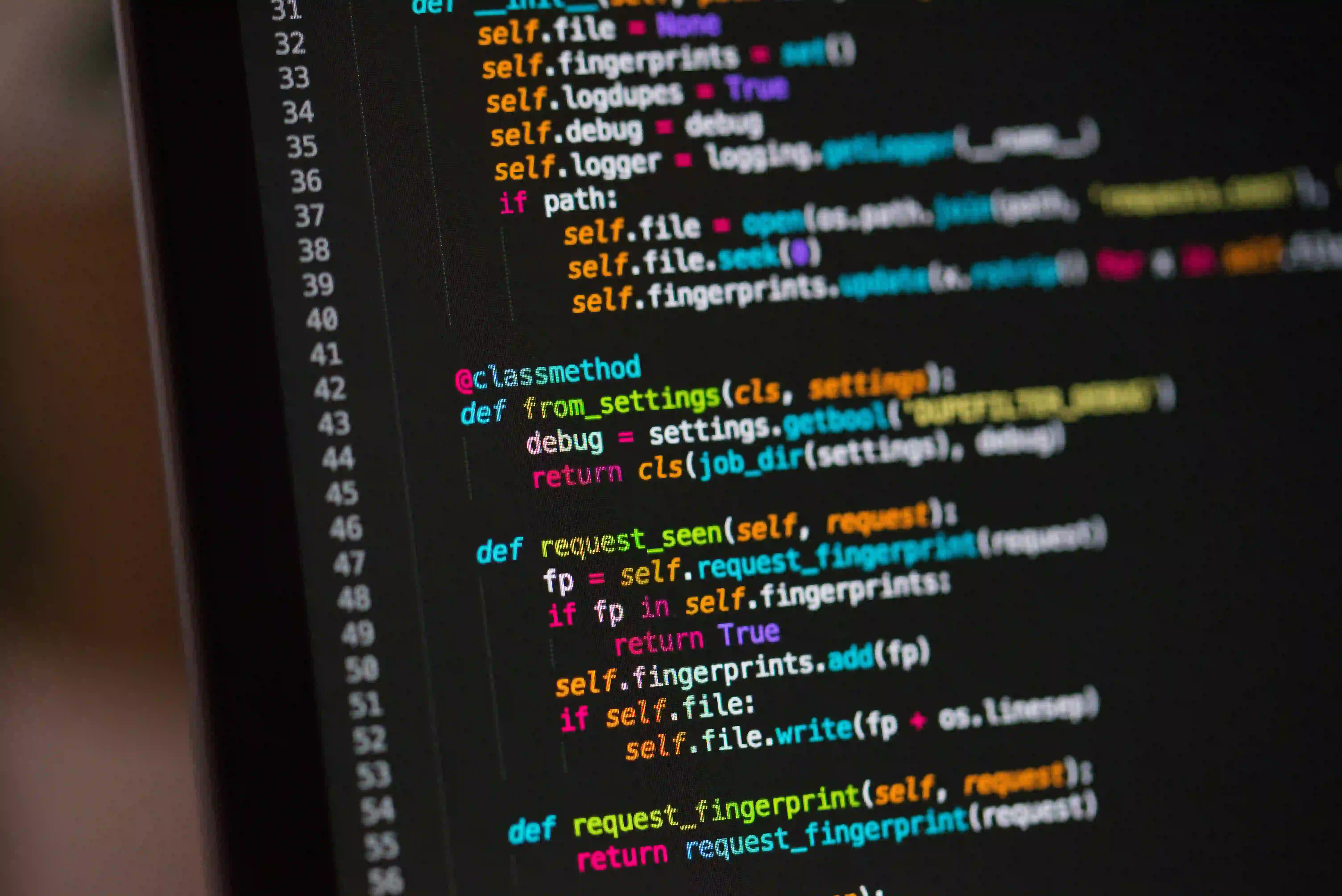
Common Docker Container Mistakes and How to Avoid Them
Docker has revolutionized the way developers build, ship, and run applications. With its containerization capabilities, it simplifies the deployment process and ensures consistency across various environments. However, even seasoned professionals can encounter pitfalls when working with Docker. This blog post explores some common Docker container mistakes and offers insights on how to avoid them, helping you to maintain best practices as you leverage this powerful technology.
Table of Contents
- 1. Ignoring Dockerfile Best Practices
- 2. Not Using .dockerignore File
- 3. Running Containers as Root
- 4. Not Monitoring Container Resources
- 5. Ignoring Layer Caching
- 6. Misconfiguring Networking
- 7. Failure to Manage Volumes Properly
- 8. Not Keeping Containers Up-to-Date
- 9. Conclusion
1. Ignoring Dockerfile Best Practices
Writing an efficient Dockerfile is crucial. Misconfiguration can lead to larger image sizes and longer build times. Here are some best practices to follow:
- Minimize the Number of Layers: Each command in a Dockerfile creates a new layer. Combine commands to minimize the number of layers.
# Before: Multiple layers:
RUN apt-get update
RUN apt-get install -y python
# After: Single layer
RUN apt-get update && apt-get install -y python
This approach not only reduces the image size but also speeds up the build process.
- Use Official Base Images: Start from a trusted base image to avoid vulnerabilities. For instance,
python:3.9-slim
ensures you're using a lightweight version of Python.
2. Not Using .dockerignore File
Just as .gitignore
helps manage your git repository, the .dockerignore
file prevents unnecessary files from being included in your container images. Not utilizing it can lead to bloated images, increasing the time it takes to build and push images.
To create a .dockerignore
file, simply list out the files and directories you want to ignore:
node_modules
*.log
*.tmp
3. Running Containers as Root
Running applications inside containers as root can lead to significant security vulnerabilities. If an attacker gains access, they may have full access to your system.
To run a container as a non-root user, specify the USER
directive in your Dockerfile:
# Create a user
RUN useradd -m myuser
# Set user
USER myuser
This creates a user named myuser
for your application to run under, significantly tightening security.
4. Not Monitoring Container Resources
Without proper monitoring tools, your containers can exhaust system resources, leading to application downtime. Use monitoring tools like Prometheus or Grafana to track key metrics such as memory and CPU usage.
You can monitor resource usage by running:
docker stats
Additionally, integrate tools like cAdvisor to gain deeper insights.
5. Ignoring Layer Caching
Docker's layer caching system is a powerful feature that can drastically improve the build time of images. Changes in a single layer don’t invalidate the entire cache.
When writing Dockerfiles, place the most static components (like package installations) at the top and more dynamic components (like application code) at the bottom:
# Install dependencies
COPY requirements.txt ./
RUN pip install -r requirements.txt
# Copy the application code
COPY . .
This setup ensures that layers that rarely change are cached effectively.
6. Misconfiguring Networking
Docker networking can be tricky. Using the wrong network type can lead to connectivity issues between containers or between a container and the external environment.
For simple applications, the default bridge network might suffice. However, for more complex applications, consider using overlay networks. Here’s how to create an overlay network:
docker network create -d overlay my_overlay
This command establishes a network that spans multiple Docker hosts, allowing containers to communicate seamlessly.
7. Failure to Manage Volumes Properly
Data persistence is critical in containerized applications. Many developers neglect the management of volumes, leading to data loss on container removal.
Always define and manage named volumes properly:
# Create a volume for persistent data
VOLUME /data
You can also create named volumes when starting containers:
docker run -d -v mydata:/data myapp
This way, data is retained even when you remove the container.
8. Not Keeping Containers Up-to-Date
Outdated images can introduce security vulnerabilities. Regularly check for updates to your base images and libraries.
Use the following command to pull the latest versions of Docker images:
docker pull myimage:latest
Consider running tools like Docker Hub's Automatic Builds to ensure your images are always up-to-date.
9. Conclusion
Docker has become a cornerstone for modern development and deployment workflows. However, avoiding common Docker container mistakes is paramount to leverage its full potential. By adhering to best practices around Dockerfile management, resource monitoring, security, and volume management, you can create a robust and secure environment for your applications.
Staying informed about these challenges is essential to avoid pitfalls that could disrupt your development workflow. By integrating these tips into your development practice, you will not only optimize your projects but also enhance collaboration with your team.
For more in-depth reading on Docker best practices, check out the official Docker documentation.
By implementing these strategies and remaining vigilant against common errors, you're not just writing better Docker containers; you're also building a more robust, performant, and secure software application. Happy coding!