Common Vaadin Integration Issues in Spring Boot
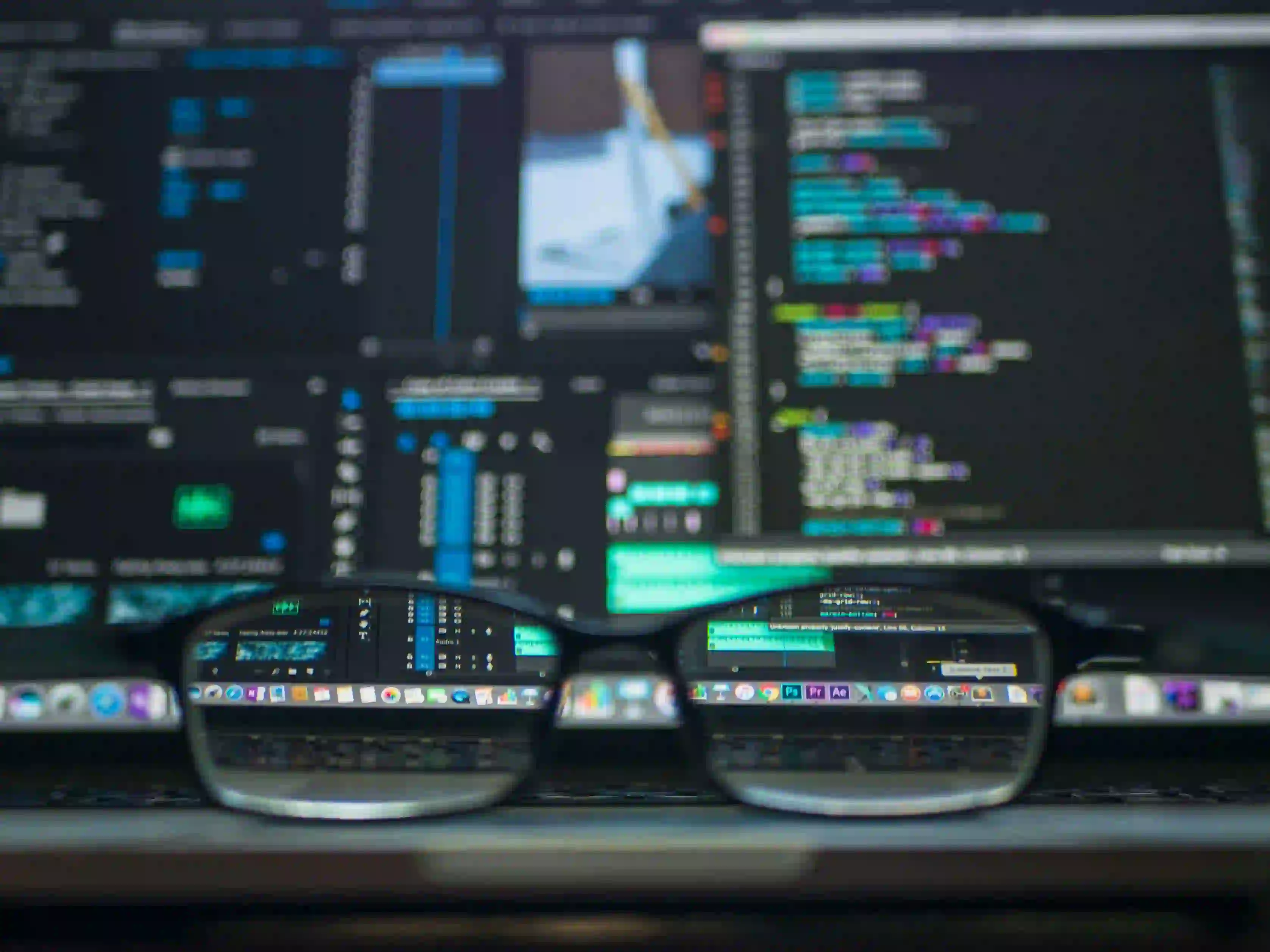
Common Vaadin Integration Issues in Spring Boot
Vaadin is a powerful Java framework for building modern web applications. When combined with Spring Boot, it enables developers to create rich user interfaces with minimal hassle. However, integrating Vaadin with Spring Boot may present some challenges. In this blog post, we will explore common issues developers face when integrating Vaadin with Spring Boot and discuss solutions to overcome them.
Table of Contents
- Understanding Vaadin and Spring Boot
- Common Integration Issues
- Dependency Conflicts
- UI Initialization Problems
- Server Configuration Issues
- Vaadin Version Mismatch
- Debugging Tips
- Conclusion
Understanding Vaadin and Spring Boot
Before tackling the integration issues, it's essential to understand the basics of both technologies. Vaadin is a Java framework designed for building user interfaces. It provides an extensive set of UI components and tools for developing responsive web applications. Spring Boot, on the other hand, is a framework that simplifies the process of developing and deploying Spring applications with built-in features for configuration, dependency management, and embedded servers.
When these two frameworks are combined, they can effectively deliver powerful web applications. But as with any technology stack, understanding common challenges can significantly enhance the development experience.
Common Integration Issues
1. Dependency Conflicts
One of the most common issues developers encounter while integrating Vaadin with Spring Boot is dependency conflict. This often occurs when there are incompatible versions of libraries in the classpath.
Solution: Exclude Unnecessary Dependencies
To avoid dependency conflicts, you can use the exclude
directive in your Maven pom.xml
or Gradle build.gradle
files. For example, if you're using Maven, you can exclude a transitive dependency like this:
<dependency>
<groupId>com.vaadin</groupId>
<artifactId>vaadin-spring-boot-starter</artifactId>
<version>14.6.0</version>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
This helps ensure that unnecessary libraries are not included, thereby minimizing conflicts.
2. UI Initialization Problems
Another issue developers face is the improper initialization of UI components. Sometimes, components may not render as expected, leading to errors or broken layouts.
Solution: Properly Define the Main View
Make sure your main UI class is annotated with @Route
and is properly set up to initiate the user interface components. Here’s a basic setup:
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import com.vaadin.flow.router.Route;
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
add(new Text("Welcome to Vaadin with Spring Boot!"));
}
}
In this example, the MainView
class serves as the entry point to your application. Make sure all your UI components are properly encapsulated within the correct layout.
3. Server Configuration Issues
Integrating Vaadin with Spring Boot often yields server configuration issues. This may refer to servlet-related problems, such as incorrect servlet mappings or contexts.
Solution: Use Spring Boot’s Default Configuration
To simplify server configurations, utilize Spring Boot's embedded server mechanisms. Ensure your application.properties
file contains the necessary configurations:
vaadin.servlet.urlMapping=/*
vaadin.productionMode=false
This setup allows Vaadin to leverage Spring Boot’s properties for correct servlet mapping.
4. Vaadin Version Mismatch
Using incompatible Vaadin and Spring Boot versions can lead to various issues, from reliance on deprecated functions to complete application failures.
Solution: Stick to Compatible Versions
Always ensure that the versions of Vaadin and Spring Boot you are using in your pom.xml
or build.gradle
are compatible. You can find the compatible version matrix in the Vaadin documentation.
Here is how to specify versions in your Maven pom.xml
:
<properties>
<vaadin.version>14.6.0</vaadin.version>
<spring-boot.version>2.4.5</spring-boot.version>
</properties>
Code Snippet: A Sample Application
To illustrate how everything comes together, here is a simplified version of a Spring Boot application that uses Vaadin.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyVaadinApp {
public static void main(String[] args) {
SpringApplication.run(MyVaadinApp.class, args);
}
}
In this example, the MyVaadinApp
class initializes a basic Spring Boot application. When the application is run, it will serve the Vaadin interface seamlessly.
Debugging Tips
- Consult Logs: Always check your server logs for errors that may indicate what went wrong during the initialization process.
- Test Compatibility: Utilize tools like Maven or Gradle to check for dependency versions and ensure compatibility.
- Use Development Mode: When developing, use Vaadin's development mode to enable hot-reloading, which can help quickly identify UI issues.
My Closing Thoughts on the Matter
Integrating Vaadin with Spring Boot can be straightforward, but you may encounter challenges such as dependency conflicts, UI initialization problems, server configuration issues, and version mismatches. Understanding these common issues and their solutions will help streamline your development process and improve overall application stability.
For more information on Vaadin and Spring Boot, check out the official documentation at Vaadin Docs and Spring Boot.
Happy coding!