Understanding System.nanoTime: Precision or Overhead?
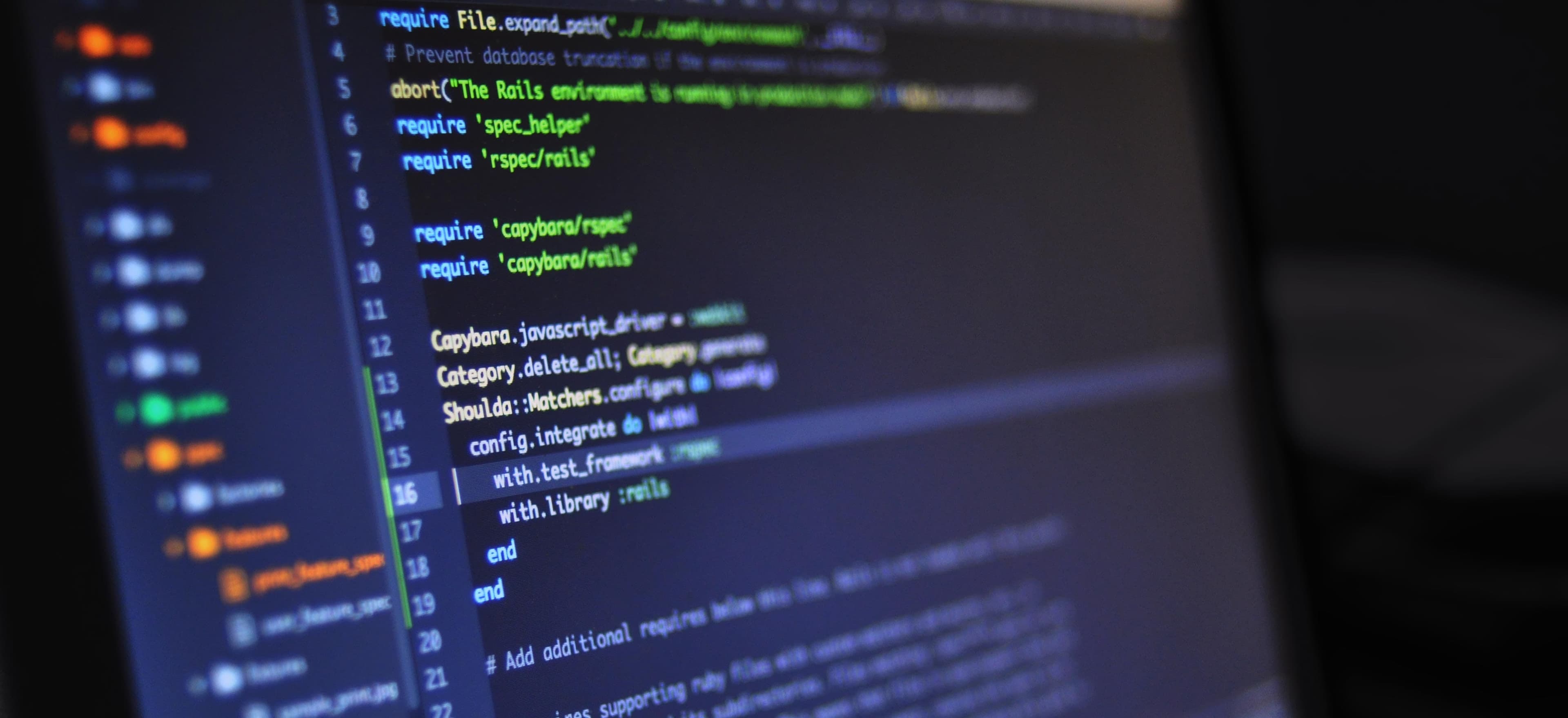
- Published on
Understanding System.nanoTime: Precision or Overhead?
Java provides several ways to measure time, and System.nanoTime() stands out for its precision. Yet, it prompts a significant question: Is it a valuable tool or merely an overhead that should be avoided? In this blog post, we will explore the details of System.nanoTime()
, its use cases, potential overhead, and recommendations for effective implementation.
What is System.nanoTime()?
System.nanoTime()
is a method provided by the Java System
class. It returns the current value of the most precise available system timer in nanoseconds. This makes it an ideal candidate for measuring elapsed time, especially in performance-critical applications.
Why Use System.nanoTime()?
-
High Precision: While
System.currentTimeMillis()
provides time in milliseconds, it lacks the precision required for certain applications such as benchmarking or profiling. -
Relative Timing:
System.nanoTime()
is designed for measuring the time between events rather than obtaining the current time. This makes it suitable for profiling code performance. -
Monotonic Clock: Unlike wall-clock time, the value returned by
System.nanoTime()
is monotonic, meaning it will not go backward. This avoids issues with system clock adjustments.
The Overhead of System.nanoTime()
Despite its advantages, System.nanoTime()
is not without concerns. The overhead associated with calling this method comes from:
- CPU Cycle Consumption: The method requires CPU cycles to fetch the current time, which can add up if used excessively.
- Granularity Differences: On some operating systems or hardware, the granularity of the clock can affect precision, although Java typically manages this well.
- Garbage Collection Impact: In performance-sensitive code, frequent time measurements can introduce noise, especially in a garbage-collected environment.
Example Usage of System.nanoTime()
Let's review a simple benchmark comparing two methods of calculating the sum of integers.
public class Benchmark {
public static void main(String[] args) {
long startTime = System.nanoTime();
// Perform the operation
long sum = calculateSum(1, 1_000_000);
long endTime = System.nanoTime();
long duration = endTime - startTime; // duration in nanoseconds
System.out.println("Sum: " + sum);
System.out.println("Execution time: " + duration + " ns");
}
public static long calculateSum(int start, int end) {
long total = 0;
for (int i = start; i <= end; i++) {
total += i;
}
return total;
}
}
Code Commentary
- Start Timer: We capture the current time before the operation begins using
System.nanoTime()
. - Perform Operation: The
calculateSum
method computes the sum of integers from a starting point to an endpoint. - End Timer: After the operation, we capture the end time and calculate the elapsed time.
- Output: We display the result and the time taken for the operation.
Best Practices for Using System.nanoTime()
To harness the power of System.nanoTime()
effectively while mitigating overhead, here are some best practices:
-
Limit Frequency: Avoid an excessive number of calls within tight loops or critical code paths. Instead, take snapshots at key points.
-
Consider Context: Use
System.nanoTime()
primarily for benchmarking and profiling, rather than for general-purpose timekeeping. -
Use Warmup Iterations: To get more reliable results, run warm-up iterations before recording timing data, which can help stabilize the JVM performance.
-
Compare with Other Timers: When needed, compare results from
nanoTime()
with other timing methods for complementary insights.
Measuring Performance of Multiple Methods
Here’s how you can benchmark two different summing methods:
public class MethodComparison {
public static void main(String[] args) {
final int N = 1_000_000;
long startTime1 = System.nanoTime();
long result1 = sumUsingLoop(N);
long duration1 = System.nanoTime() - startTime1;
long startTime2 = System.nanoTime();
long result2 = sumUsingFormula(N);
long duration2 = System.nanoTime() - startTime2;
System.out.println("Loop Result: " + result1 + " Time: " + duration1 + " ns");
System.out.println("Formula Result: " + result2 + " Time: " + duration2 + " ns");
}
public static long sumUsingLoop(int n) {
long sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
public static long sumUsingFormula(int n) {
return (long) n * (n + 1) / 2;
}
}
Code Explanation
- Two Sum Methods: We define both a loop method and a mathematical formula method for summing up integers.
- Timing: We measure the time taken for each method in nanoseconds.
- Output: Finally, we print out each result along with its execution time.
The Closing Argument: Is System.nanoTime() Worth It?
The choice of using System.nanoTime()
depends largely on your project's requirements. If you need high-precision time measurements, it is an excellent choice—just use it wisely.
When to Use:
- Profiling and benchmarking critical code paths.
- Measuring elapsed time of methods where precise measurements can influence performance optimization.
When to Avoid:
- Frequent calls in high-frequency loops.
- Situations where performance impact from measurement overhead can overshadow the benefits gained from precision.
In summary, System.nanoTime()
provides an accurate means of measuring execution time but should be used judiciously. Always keep the trade-off between precision and overhead in mind, and you’ll be well on your way to optimizing your Java applications effectively.
For further reading on performance tuning in Java, check out Java Performance Tuning. Understanding how the JVM operates can provide critical insights into your benchmarks.