Mastering Relationships: First-Class Citizens in OOP
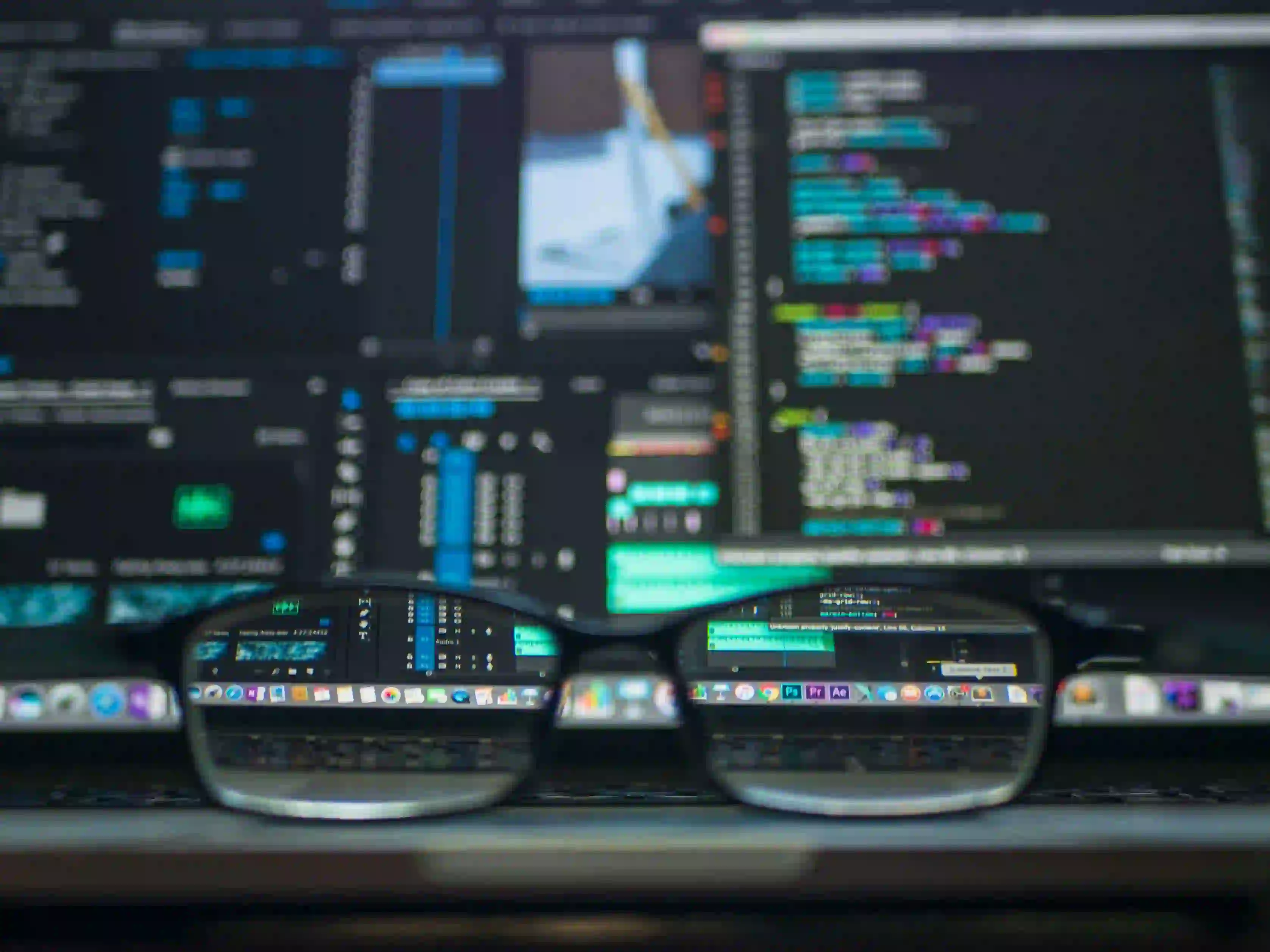
Mastering Relationships: First-Class Citizens in OOP
Object-Oriented Programming (OOP) is renowned for its ability to model complex systems through the use of classes and objects. One of the cornerstones of OOP is the concept of relationships among these objects. Understanding and mastering the different types of relationships can greatly enhance your programming skills and the architecture of your applications. In this blog post, we will explore the various relationships in OOP, particularly in Java, and how they can be used effectively in your designs.
Understanding Relationships in OOP
In OOP, classes can relate to each other in several ways. The main types of relationships include:
- Association
- Aggregation
- Composition
- Inheritance
Let's delve into each one and see how they play a vital role in building robust and maintainable software.
Association
Association represents a "using" relationship. In this case, an object of one class can use or interact with an object of another class. This relationship is the most general type, and it implies that one class does not have ownership over the other.
Example of Association
class Driver {
private String name;
public Driver(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
class Car {
private String model;
private Driver driver;
public Car(String model) {
this.model = model;
}
public void assignDriver(Driver driver) {
this.driver = driver;
}
public String getDriverName() {
return driver != null ? driver.getName() : "No driver assigned.";
}
}
In this example, the Car
class associates with the Driver
class. A car can have a driver assigned to it, but it does not own the driver. This establishes a flexible relationship where a driver can also switch between cars if needed.
Aggregation
Aggregation is a specialized form of association where an object is made up of one or more objects but does not own them strictly. The lifetime of the part does not depend on the lifetime of the whole. This means that objects can exist independently.
Example of Aggregation
class Library {
private List<Book> books;
public Library() {
this.books = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
}
class Book {
private String title;
public Book(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
}
In this example, the Library
class aggregates Book
objects. A library can have multiple books, but it does not necessarily own them. Thus, the books can exist without the library — for instance, if they are removed from the library's collection.
Composition
Composition is a stronger form of association where an object is composed of one or more objects, and the lifetime of the contained objects is tied to the lifetime of the container. If the container is destroyed, so are the contained objects.
Example of Composition
class House {
private List<Room> rooms;
public House() {
this.rooms = new ArrayList<>();
this.rooms.add(new Room("Bedroom"));
this.rooms.add(new Room("Bathroom"));
}
public void showRooms() {
for (Room room : rooms) {
System.out.println("Room: " + room.getName());
}
}
}
class Room {
private String name;
public Room(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In the House
example, the House
class owns Room
objects. If a house is destroyed, the rooms cease to exist. This tight coupling is crucial in scenarios where contained objects should not outlive their parent.
Inheritance
Inheritance is a fundamental OOP concept that establishes an "is-a" relationship between classes. One class can inherit attributes and behaviors from another class, which promotes code reusability and establishes a hierarchical relationship.
Example of Inheritance
class Vehicle {
public void start() {
System.out.println("Vehicle is starting");
}
}
class Truck extends Vehicle {
public void loadCargo() {
System.out.println("Loading cargo");
}
}
public class Main {
public static void main(String[] args) {
Truck myTruck = new Truck();
myTruck.start();
myTruck.loadCargo();
}
}
Here, the Truck
class inherits from the Vehicle
class. This allows Truck
to use the start
method defined in the parent class while also adding its own functionality through the loadCargo
method.
The Importance of Relationships in OOP Design
Mastering relationships is essential for several reasons:
-
Code Reusability: Proper use of relationships like inheritance allows for better code reuse. You can create new classes based on existing ones without reinventing the wheel.
-
Maintainability: Clear relationships make it easier to understand and maintain your code. Changes in one class can naturally propagate if relationships are defined correctly.
-
Modularity: With proper relationships, your code is more modular and flexible, allowing for easier updates and enhancements over time.
-
Readability: A well-defined relationship structure can improve code readability, making it easier for other developers to understand your logic.
To Wrap Things Up
In this blog post, we explored the various relationships in Object-Oriented Programming, focusing on association, aggregation, composition, and inheritance. Understanding these relationships can significantly improve your design and implementation strategies in Java and other OOP languages.
If you're looking to further your expertise in OOP concepts, consider exploring the sophisticated design patterns found in the Gang of Four book. These patterns demonstrate how relationships between classes can be utilized to solve common design problems effectively.
Master these relationships and witness how your code evolves into an elegant, efficient, and maintainable solution. Happy coding!