Common Pitfalls When Replacing Rules in JUnit 5
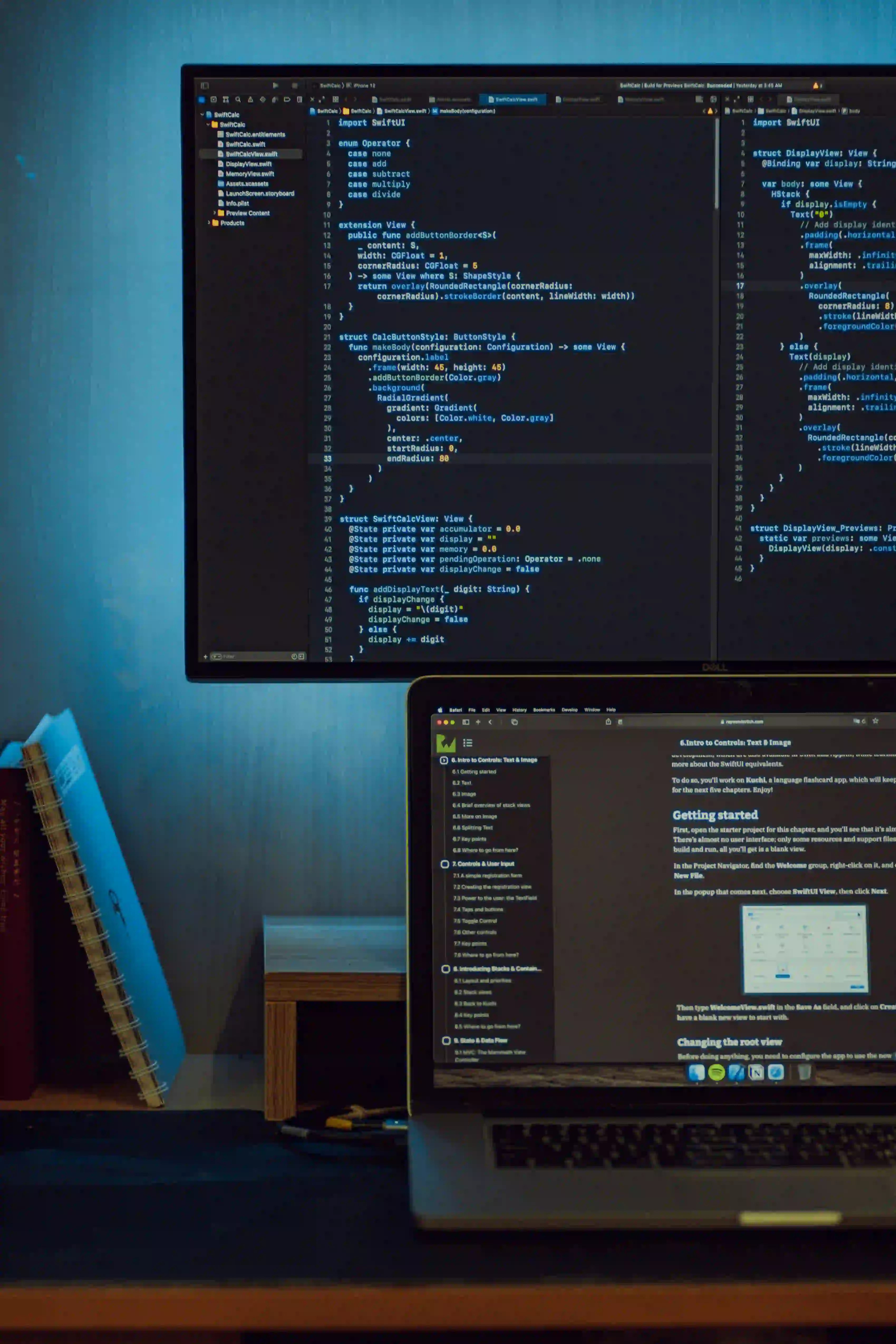
Common Pitfalls When Replacing Rules in JUnit 5
JUnit 5 has introduced a plethora of improvements over its predecessor, JUnit 4, especially when it comes to testing functionality and enhancing maintainability. One significant change is the replacement of the concept of "Rules" in JUnit 4 with "Extensions" in JUnit 5. While this shift brings about more flexibility and power, it can also lead to some common pitfalls, especially for those transitioning from JUnit 4. In this blog post, we’ll explore these pitfalls in detail, all the while highlighting best practices and effective ways to mitigate potential pitfalls.
Understanding Rules vs. Extensions
Before diving into specific pitfalls, let’s clarify the differences between "Rules" in JUnit 4 and "Extensions" in JUnit 5.
Rules
In JUnit 4, Rules were used to apply common behavior to test methods without the need to edit the test code directly. For example, you could use a Rule to manage resource cleanup or to apply common setup procedures.
Extensions
JUnit 5 offers a more robust mechanism through Extensions. They provide similar capabilities but allow for more flexibility than Rules. Extensions can be applied at various scopes (method, class, or even globally), and there are various types of extensions, including BeforeEach
, AfterEach
, BeforeAll
, AfterAll
, etc.
Common Pitfalls When Transitioning to JUnit 5
1. Ignoring the Lifecycle Annotations
One of the most significant shifts is the lifecycle management through annotations. JUnit 5 offers several new lifecycle annotations (@BeforeEach
, @AfterEach
, @BeforeAll
, @AfterAll
) that must be implemented correctly.
Common Mistake:
Developers often misplace these annotations, particularly @BeforeAll
and @AfterAll
, which need to be declared as static methods in non-static test classes.
Best Practice:
Always ensure that methods annotated with @BeforeAll
and @AfterAll
are static unless you're explicitly using @TestInstance(TestInstance.Lifecycle.PER_CLASS)
.
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class MyTests {
@BeforeAll
void setup() {
// This will work without being static
System.out.println("Setting up the tests");
}
@Test
void testSomething() {
// Your test code here
}
}
2. Overusing Extensions
While JUnit 5 Extensions are powerful, overly relying on them can lead to complex tests that are hard to read and maintain.
Common Mistake: Developers may stack multiple extensions, creating test classes that are difficult to comprehend.
Best Practice: Keep your test classes clean and use only essential extensions. If multiple behaviors need to be integrated, consider creating a custom extension that encapsulates the required behaviors, rather than using several disparate extensions.
3. Misunderstanding the Executable Parameter
JUnit 5 allows usage of method references and lambda expressions in its assertions. This might confuse some developers transitioning from JUnit 4.
Common Mistake:
Failing to recognize the need for Executable
parameter in assertThrows.
Best Practice:
Always wrap your test code in the Executable
interface when using assertThrows
.
import static org.junit.jupiter.api.Assertions.assertThrows;
public class MyTests {
@Test
void testException() {
// Using lambda to capture the executable
assertThrows(IllegalArgumentException.class, () -> {
throw new IllegalArgumentException("test");
});
}
}
4. Incorrectly Using Dependency Injection
JUnit 5 supports dependency injection for test instances through the @ExtendWith
annotation. However, misunderstanding its context can lead to confusion.
Common Mistake: Failing to use the correct context for dependency injection, such as not using the correct extension for the test environment.
Best Practice: Ensure that your extension matches the scope of input/output required for your test setup.
5. Neglecting Parameterized Tests
The introduction of parameterized tests is another strong feature in JUnit 5. However, migrating from JUnit 4 to JUnit 5 can leave developers unaware of how to handle parameters effectively.
Common Mistake:
Not utilizing @ParameterizedTest
appropriately leads to repetitive test cases.
Best Practice:
Utilize the built-in sources of parameters, such as @MethodSource
or @CsvSource
.
import static org.junit.jupiter.params.provider.Arguments.arguments;
@ParameterizedTest
@MethodSource("provideStringsForConcatenation")
void testConcatenate(String first, String second, String expected) {
assertEquals(expected, first + second);
}
private static Stream<Arguments> provideStringsForConcatenation() {
return Stream.of(
arguments("Hello", " World", "Hello World"),
arguments("Foo", "Bar", "FooBar")
);
}
My Closing Thoughts on the Matter
Moving from JUnit 4 to JUnit 5 opens up many new possibilities for writing cleaner, more maintainable tests. However, it also introduces certain pitfalls that beginners (and sometimes even seasoned developers) may encounter.
Understanding the fundamental differences between Rules and Extensions, paying attention to lifecycle annotations, avoiding unnecessary complexities from too many extensions, and practicing effective parameterized testing are all essential practices for successful test transition.
For more detailed reading, you might find the official JUnit 5 User Guide invaluable as you navigate these changes. Additionally, insights into parameterized tests can enhance your testing strategy.
By recognizing these common pitfalls and applying best practices, you'll be well on your way to mastering JUnit 5. Happy testing!