Common RabbitMQ Issues in Play Framework and Fixes
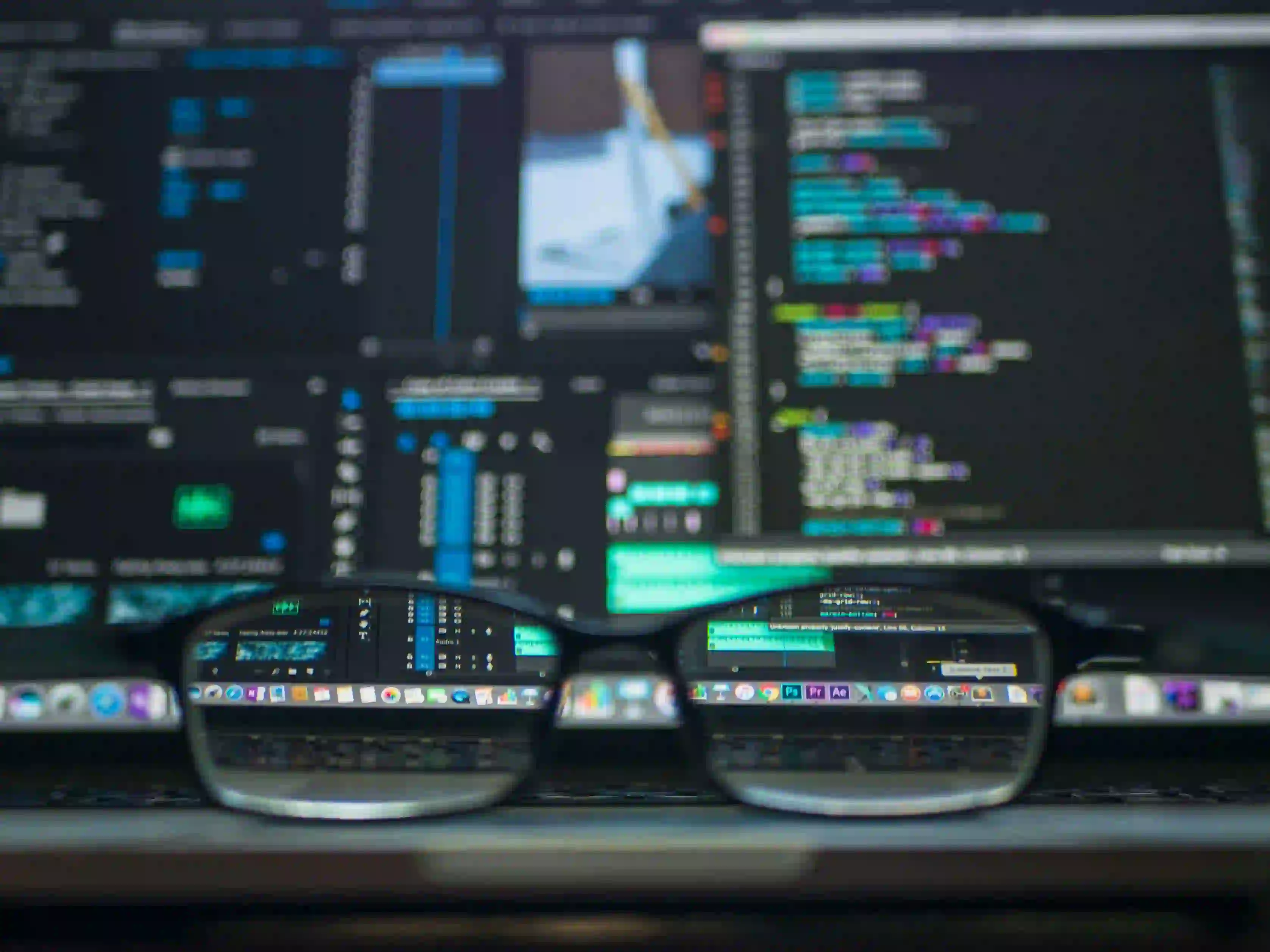
Common RabbitMQ Issues in Play Framework and Fixes
When integrating RabbitMQ with the Play Framework, developers often encounter a range of common issues. Understanding these pitfalls and their resolutions can significantly enhance the reliability and performance of your application. This blog post aims to provide insights into frequent RabbitMQ challenges within the Play Framework ecosystem and suggest effective solutions.
Table of Contents
The Roadmap to RabbitMQ and Play Framework
RabbitMQ is a robust message broker that enables applications to communicate with one another in a scalable and efficient manner. The Play Framework, on the other hand, is a powerful web development framework for building Java and Scala applications. When combined, these technologies can create highly performant and responsive applications.
In this post, we will explore common RabbitMQ issues developers face when working with the Play Framework, why they occur, and how to address them effectively.
Common Issues and Their Solutions
1. Connection Issues
Problem: One of the most common issues developers face is establishing a connection to RabbitMQ. Factors such as incorrect credentials, incorrect hostnames, or RabbitMQ server downtime can lead to connection failures.
Solution: Ensure the correct configuration settings are in place. For instance, the connection URL, username, and password in your application configuration file should be accurate.
Example Configuration:
rabbitmq {
host = "localhost"
port = 5672
user = "guest"
password = "guest"
}
Why This Works: By ensuring that the host points to the correct RabbitMQ server and that the credentials are accurate, your Play Framework application can successfully establish a connection.
Additional Tip: Use connection pooling to manage RabbitMQ connections efficiently, especially in high-load scenarios. Libraries like spring-amqp can be useful for creating connection factories.
2. Message Loss
Problem: In some scenarios, especially during high traffic, messages may be lost. This often happens when messages are sent but not acknowledged correctly.
Solution: Implement publisher confirms and consumer acknowledgments. This ensures that messages are not lost due to unacknowledged sends.
Example Code:
channel.confirmSelect(); // Enable publisher confirms
channel.basicPublish("", QUEUE_NAME, null, message.getBytes());
boolean confirmed = channel.waitForConfirms(); // Wait for confirmation
if (!confirmed) {
// Handle failed confirmation
}
Why This Works: Enabling publisher confirms allows you to verify that messages are successfully received by RabbitMQ, reducing the chance of message loss.
3. Acknowledgment Errors
Problem: Acknowledgment is crucial for messaging integrity. If you fail to send an acknowledgment after processing a message, RabbitMQ assumes that the message was not processed and may requeue it.
Solution: Always acknowledge messages after they're processed successfully.
Example Code:
channel.basicConsume(QUEUE_NAME, false, (consumerTag, message) -> {
try {
processMessage(message);
channel.basicAck(message.getEnvelope().getDeliveryTag(), false);
} catch (Exception e) {
// Handle processing error
channel.basicNack(message.getEnvelope().getDeliveryTag(), false, true);
}
}, consumerTag -> {});
Why This Works:
Using basicAck
confirms successful processing, while basicNack
requeues the message for eventual processing if there is an error.
4. Performance Problems
Problem: As your application scales, performance can degrade. This might be a result of slow consumers or excessive message convert and serialization times.
Solution:
- Prefetch Count: Adjust the prefetch count to control how many messages are sent before an acknowledgment is received.
- Asynchronous Processing: Utilize asynchronous processing of messages when feasible.
Example Code:
channel.basicQos(10); // Limit the prefetch count
// Use a separate thread pool for processing messages asynchronously
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> {
// message processing logic here
});
Why This Works: By limiting the prefetch count, the consumer can better manage its load, and asynchronous processing can utilize the application's resources more effectively.
5. Serialization Issues
Problem: Serialization errors often occur when converting objects to bytes for RabbitMQ. If the message payload is not correctly serialized and deserialized, it can lead to application failures.
Solution: Use standardized serialization mechanisms such as JSON or Protocol Buffers.
Example Code:
ObjectMapper objectMapper = new ObjectMapper();
String jsonMessage = objectMapper.writeValueAsString(yourObject);
channel.basicPublish("", QUEUE_NAME, null, jsonMessage.getBytes());
Why This Works: Using a consistent serialization mechanism like JSON with libraries such as Jackson ensures that objects are converted to and from a format that retains their structure and meaning, reducing the likelihood of errors.
To Wrap Things Up
Integrating RabbitMQ with the Play Framework can enable powerful messaging capabilities in your applications. However, you may face various common issues, from connection problems to performance bottlenecks. By recognizing these issues and applying the solutions discussed, you can significantly improve the reliability and efficiency of your messaging system.
For further reading, consider exploring the following resources:
By understanding and addressing these common pitfalls, you can ensure a smoother integration process and a more robust application overall. Keep coding, stay curious, and happy coding!