Why Tests-as-Documentation Often Fails Teams
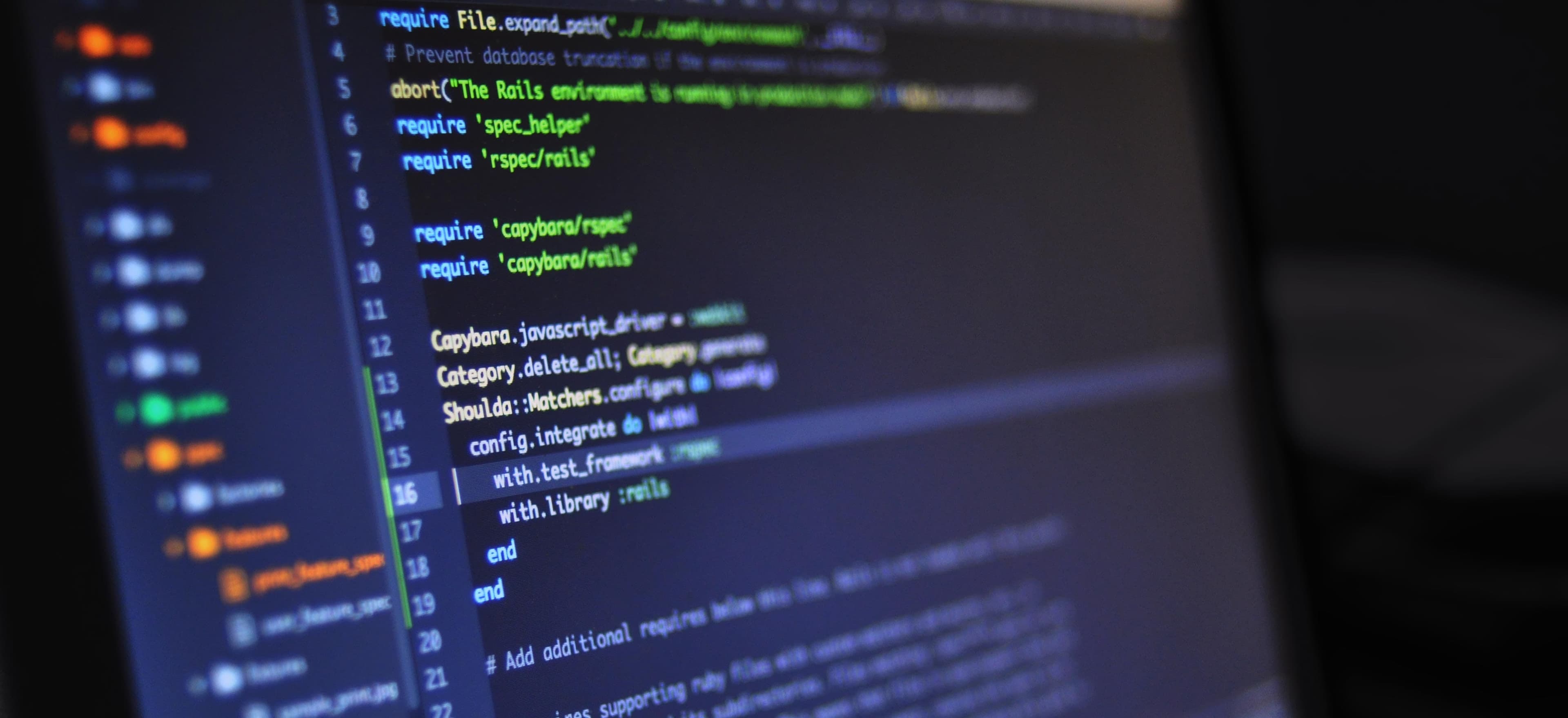
- Published on
Why Tests-as-Documentation Often Fails Teams
In the realm of software development, tests play a critical role. They ensure quality, enforce functionality, and ultimately contribute to a seamless user experience. However, an intriguing topic arises around the concept of "Tests-as-Documentation." While many teams lean heavily on this approach, it's worth exploring why it often falls short of expectations.
Understanding the Concept of Tests-as-Documentation
What is Tests-as-Documentation?
The idea behind Tests-as-Documentation is simple: tests should serve dual purposes. Not only do they verify the correctness of the code, but they also act as living documentation for the system. A well-written test case can inform future developers about the intent and functionality of the code base.
The Ideal Scenario
In a perfect world, tests would serve as comprehensive guides. Developers could write a feature, create a robust suite of tests, and those tests would then explain how the feature works. This would theoretically reduce the need for separate documentation, streamline onboarding, and improve overall team efficiency.
However, reality often tells a different story.
Common Pitfalls of Tests-as-Documentation
While the concept sounds appealing, there are several reasons why teams struggle to implement it effectively.
1. Lack of Clarity
In many cases, tests may be written in a way that isn’t obvious. Consider the following code snippet:
@Test
public void shouldReturn200WhenRequestIsValid() {
Response response = apiCall("/valid-endpoint");
assertEquals(200, response.getStatus());
}
While the test checks for a successful response, it does little to explain the context behind the /valid-endpoint
. New developers might find it challenging to understand what makes the endpoint valid, or what inputs are acceptable.
2. Overwhelming Testing Frameworks
The proliferation of testing frameworks and tools can lead to confusion. Developers might become so focused on syntax and structure that they overlook the purpose of the tests. For instance, using Mockito might lead to tests like this:
@Test
public void testSendEmail() {
EmailService mockEmailService = mock(EmailService.class);
User user = new User("test@example.com");
// Perform the action
user.sendEmail(mockEmailService);
// Verification
verify(mockEmailService).send("test@example.com");
}
Although the purpose is clear to those familiar with Mockito, it may be less so to newcomers, particularly if they don't understand dependency injection.
3. Tests Become Outdated
As developers modify the code base, tests can frequently become outdated or misaligned. This can happen for multiple reasons:
- Changes in functionality without corresponding updates to tests.
- The addition of complexity without clear motivational documentation in the tests themselves.
Here’s an example:
@Test
public void shouldCalculateTotalPrice() {
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("Apple", 1.00));
cart.addItem(new Item("Banana", 0.50));
assertEquals(1.50, cart.getTotalPrice(), 0.001);
}
If the pricing model changes, unless clearly indicated, the purpose of the test may be lost, leading to potential confusion about the functionality it was initially documenting.
4. Lack of Narrative
Tests often fail to tell a story. They may verify functionality but do not contextualize it within the domain of the application. A test should be more than a simple assertion; it should convey why the functionality exists.
For instance, instead of:
@Test
public void shouldNotDeleteUserWhenNotFound() {
boolean result = userService.deleteUser(999);
assertFalse(result);
}
A better approach would be:
@Test
public void shouldNotDeleteUserWhenNotFound() {
// Attempting to delete a user that does not exist should return false.
boolean result = userService.deleteUser(999);
assertFalse("Expected deletion of non-existent user to return false", result);
}
This version provides additional clarity and intent, improving the test's capability as documentation.
A Better Approach to Documentation
Given these pitfalls, what can teams do? Here are several strategies to improve the effectiveness of tests as documentation:
1. Clear Naming Conventions
Ensuring tests have descriptive names can significantly enhance their readability. By using a naming convention that reflects the expected outcome and purpose, teams can provide clarity. For instance:
@Test
public void shouldReturnValidationErrorWhenEmailIsInvalid() { ... }
2. Align Tests with User Stories
Testing should relate back to user stories. This involves drawing a direct line between what a feature is supposed to do and how it's tested. Utilizing a method such as Behavior-Driven Development (BDD) can provide significant benefits in this area as it facilitates collaboration among stakeholders.
3. Maintain Documentation
Having all tests write clear descriptions of what they expect helps future developers. However, as functionality evolves, regular audits of tests help ensure they remain relevant. This means not just running tests, but reviewing their intent and context.
4. Combine Tests with Traditional Documentation
While tests can serve as documentation, they should not be the sole resource. Complementing them with additional documentation—like API documentation or architecture diagrams—provides a more holistic view of the system.
5. Adopt Code Comments Judiciously
While code comments should not be overused, relevant comments explaining particularly complex test logic can bridge gaps that pure test cases leave. This helps to provide insights into why a test exists and what issues it’s solving.
Wrapping Up
While the idea of Tests-as-Documentation has merit, teams must remain aware of its limitations. Clarity, narrative, and alignment with business objectives are crucial aspects that cannot be overlooked. By cultivating a culture of rigorous testing combined with thoughtful documentation practices, teams can foster an environment where both tests and traditional documentation coexist to enhance understanding and facilitate a seamless development process.
For further insights on testing and software quality assurance, consider these useful resources:
- Testing on the JVM
- Effective Unit Testing
By deliberately addressing these common pitfalls, teams can better utilize tests as an invaluable documentation tool—ensuring that both current and future developers can navigate the code and its purpose with confidence.